Adding Elements to ArrayList in Java: How-To Guide
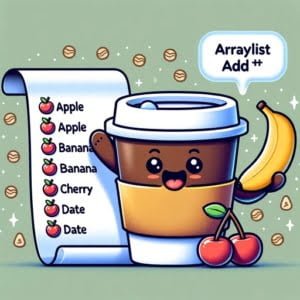
Are you finding it challenging to add elements to an ArrayList in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling dynamic data structures in Java, but we’re here to help.
Think of Java’s ArrayList as a dynamic container – allowing us to store data on the fly, providing a versatile and handy tool for various tasks.
This guide will walk you through the process of adding elements to an ArrayList in Java, from basic usage to advanced techniques. We’ll cover everything from the basics of the add()
method to more advanced techniques, as well as alternative approaches.
Let’s get started and master the art of adding elements to an ArrayList in Java!
TL;DR: How Do I Add Elements to an ArrayList in Java?
To add elements to an ArrayList in Java, you can use the
.add()
method of the ArrayList class, with the syntaxarrayListName.add(valueToAdd);
. This method allows you to add elements to the end of an ArrayList.
Here’s a simple example:
ArrayList<String> list = new ArrayList<String>();
list.add("Hello");
// Output:
// [Hello]
In this example, we create an ArrayList of type String and add the string ‘Hello’ to it using the add()
method. The output shows that the string ‘Hello’ has been successfully added to the ArrayList.
This is a basic way to add elements to an ArrayList in Java, but there’s much more to learn about ArrayLists and their manipulation. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Adding Elements to ArrayList: The Basics
When dealing with ArrayLists in Java, the add()
method is our go-to tool for inserting elements. This method allows us to add elements to the end of an ArrayList, thereby expanding its size dynamically. Let’s delve into how this works.
The add()
Method in Action
Here’s a simple example of adding elements to an ArrayList using the add()
method:
ArrayList<String> fruits = new ArrayList<String>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Cherry");
System.out.println(fruits);
// Output:
// [Apple, Banana, Cherry]
In this example, we create an ArrayList named ‘fruits’ and add three elements to it. The add()
method appends the elements to the end of the ArrayList, which we can see from the output.
Advantages and Pitfalls of add()
The add()
method is powerful and easy to use, but it’s important to understand its behavior to use it effectively.
Advantages:
- Dynamic Size: Unlike arrays, ArrayLists can dynamically resize, allowing you to add elements without worrying about the size of the ArrayList.
Ease of Use: The
add()
method is straightforward and simple, making it easy to add elements to an ArrayList.
Pitfalls:
- Performance: If an ArrayList is near its maximum capacity and we add an element, it needs to increase its size. This resizing operation can be a performance hit if it happens frequently.
Null Elements: The
add()
method allows null elements. If not handled correctly, this can lead to NullPointerExceptions.
Understanding these aspects of the add()
method will help you better manipulate ArrayLists in Java.
Inserting Elements at Specific Indexes
As you become more comfortable with using ArrayLists in Java, you might find yourself needing to insert elements at specific positions, rather than just appending them to the end. For this, Java provides an overloaded version of the add()
method, which accepts an index parameter.
Using add(int index, E element)
Method
This method allows us to add an element at a specific index in the ArrayList, shifting the existing elements to the right. Here’s an example of how it works:
ArrayList<String> fruits = new ArrayList<String>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add("Cherry");
fruits.add(1, "Orange");
System.out.println(fruits);
// Output:
// [Apple, Orange, Banana, Cherry]
In this example, we insert ‘Orange’ at index 1 of the ArrayList. The existing elements (from index 1 onwards) are shifted to the right, and ‘Orange’ takes its place at index 1.
This version of the add()
method provides more control over where elements are inserted within the ArrayList. However, it’s important to note that the index provided must be within the current size of the ArrayList (or equal to it), or an ‘IndexOutOfBoundsException’ will be thrown.
Exploring Alternative Methods to Add Elements
While the add()
method is a fundamental tool for manipulating ArrayLists, Java provides other methods that offer more flexibility and convenience when adding multiple elements or collections. Let’s delve into a couple of these alternatives.
The addAll()
Method
The addAll()
method allows you to add all elements from a collection to an ArrayList. This is particularly useful when you want to merge two ArrayLists.
Here’s an example:
ArrayList<String> fruits = new ArrayList<String>();
fruits.add("Apple");
fruits.add("Banana");
ArrayList<String> moreFruits = new ArrayList<String>();
moreFruits.add("Cherry");
moreFruits.add("Orange");
fruits.addAll(moreFruits);
System.out.println(fruits);
// Output:
// [Apple, Banana, Cherry, Orange]
In this example, we have two ArrayLists: ‘fruits’ and ‘moreFruits’. We use the addAll()
method to add all elements of ‘moreFruits’ to ‘fruits’. The output shows that ‘fruits’ now contains all elements from both ArrayLists.
The Collections.addAll()
Method
The Collections.addAll()
method is another alternative. This static method allows you to add multiple elements to an ArrayList in a single line.
Here’s how it works:
ArrayList<String> fruits = new ArrayList<String>();
Collections.addAll(fruits, "Apple", "Banana", "Cherry", "Orange");
System.out.println(fruits);
// Output:
// [Apple, Banana, Cherry, Orange]
In this example, we use Collections.addAll()
to add four elements to the ‘fruits’ ArrayList in a single line. The output shows that all four elements have been successfully added.
These alternative methods provide more flexibility and convenience when adding elements to an ArrayList, particularly when dealing with multiple elements or collections.
While working with ArrayLists in Java, you might encounter a few common issues. Understanding these issues and knowing how to solve them can save you a lot of time and frustration.
Dealing with ‘IndexOutOfBoundsException’
One common issue when using the add(int index, E element)
method is the ‘IndexOutOfBoundsException’. This exception is thrown when you try to add an element at an index that is outside the current size of the ArrayList.
For example, consider the following code:
ArrayList<String> fruits = new ArrayList<String>();
fruits.add("Apple");
fruits.add("Banana");
try {
fruits.add(5, "Cherry");
} catch (IndexOutOfBoundsException e) {
System.out.println("Caught exception: " + e.getMessage());
}
// Output:
// Caught exception: Index: 5, Size: 2
In this example, we try to add ‘Cherry’ at index 5, but the size of ‘fruits’ is only 2. This results in an ‘IndexOutOfBoundsException’.
To avoid this issue, always ensure that the index you’re adding at is within the current size of the ArrayList.
Handling Null Elements
Another consideration when working with ArrayLists is that they allow null elements. If not handled correctly, this can lead to NullPointerExceptions.
Always check if an element is null before calling methods on it to avoid NullPointerExceptions.
These are just a few of the common issues you might encounter when adding elements to an ArrayList in Java. By understanding these issues and how to navigate them, you can work with ArrayLists more effectively.
Understanding Java’s ArrayList Class
Before we delve deeper into the mechanics of adding elements to an ArrayList, it’s essential to understand what an ArrayList is and how it operates in Java.
What is an ArrayList?
An ArrayList in Java is a resizable array, also known as a dynamic array. It implements the List interface and is part of Java’s collection framework. Unlike a standard array, an ArrayList can grow and shrink dynamically as you add or remove elements.
The add()
Method: A Closer Look
The add()
method is a part of the ArrayList class in Java. It is used to add elements to an ArrayList. There are two forms of the add()
method:
boolean add(E e)
: This method appends the specified element to the end of the list. It returns true upon successful addition.void add(int index, E element)
: This method inserts the specified element at the specified position in the list.
Here is an example of using the add()
method:
ArrayList<String> fruits = new ArrayList<String>();
fruits.add("Apple");
fruits.add("Banana");
fruits.add(1, "Cherry");
System.out.println(fruits);
// Output:
// [Apple, Cherry, Banana]
In this example, we first add ‘Apple’ and ‘Banana’ to the ArrayList. Then, we add ‘Cherry’ at index 1. The output shows that ‘Cherry’ has been inserted at index 1, and ‘Banana’ has been shifted to the right.
Understanding these fundamental concepts will help you better grasp the process of adding elements to an ArrayList in Java.
Exploring Further: ArrayLists in Larger Projects
Adding elements to an ArrayList is a fundamental skill in Java programming. However, it’s just the tip of the iceberg. As you work on larger projects, you’ll find that ArrayLists can be used in many more ways and situations.
Diving Deeper into ArrayList Methods
The add()
method is just one of many methods provided by the ArrayList class. Other methods like remove()
, get()
, set()
, clear()
, and size()
offer different ways to manipulate and interact with an ArrayList. Understanding these methods will give you a more comprehensive grasp of ArrayLists and their capabilities.
ArrayLists in the Context of Larger Projects
In larger projects, ArrayLists often serve as dynamic data structures to store and manipulate complex data. They can be used to store objects, making them particularly useful in object-oriented programming. Learning how to effectively use ArrayLists can significantly enhance your efficiency and productivity in Java programming.
Further Resources for Mastering ArrayLists
To deepen your understanding of ArrayLists in Java, here are some resources that you might find helpful:
- Java ArrayList: A Quick Overview – Explore ArrayList’s error handling and exception mechanisms in Java.
Array vs ArrayList: Key Differences – Master choosing between arrays and ArrayLists for Java programs.
Length of ArrayList in Java – Learn how to obtain the size and determine the number of elements of an ArrayList in Java.
Oracle’s Java Documentation on ArrayList provides detailed information about all the ArrayList class methods and functions.
Java ArrayList Tutorial by JavaTpoint provides a comprehensive overview of ArrayLists in Java.
Java ArrayLists by GeeksforGeeks offers a deep dive into ArrayLists.
These resources should provide a solid foundation for mastering the use of ArrayLists in Java.
Wrapping Up: Adding to ArrayLists
In this comprehensive guide, we’ve explored the process of adding elements to an ArrayList in Java. From understanding the basics of ArrayLists to learning how to add elements using different methods, we’ve covered a broad spectrum of topics to help you become proficient at using ArrayLists.
We began with the basics, explaining how the add()
method works and how to use it to add elements to an ArrayList. We then moved on to more advanced usage, discussing how to add elements at specific indexes in an ArrayList and exploring alternative methods for adding elements.
We also delved into common issues you might encounter when working with ArrayLists, such as ‘IndexOutOfBoundsException’ and handling null elements, providing solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Usage | Considerations |
---|---|---|
add(E e) | Adds an element to the end of the ArrayList | Performance can be affected if ArrayList needs to resize frequently |
add(int index, E element) | Inserts an element at a specific index in the ArrayList | ‘IndexOutOfBoundsException’ if index is out of range |
addAll(Collection c) | Adds all elements from a collection to the ArrayList | Convenient for adding multiple elements or merging ArrayLists |
Collections.addAll() | Adds multiple elements to an ArrayList in a single line | Quick and convenient for adding multiple elements |
Whether you’re just starting out with ArrayLists or looking to refine your skills, we hope this guide has provided you with a deeper understanding of how to add elements to an ArrayList in Java.
The ability to manipulate ArrayLists effectively is a crucial skill in Java programming. With the knowledge you’ve gained from this guide, you’re well-equipped to handle ArrayLists in your future projects. Happy coding!