Creating an Infinite Bash Loop: Linux Shell Script Syntax
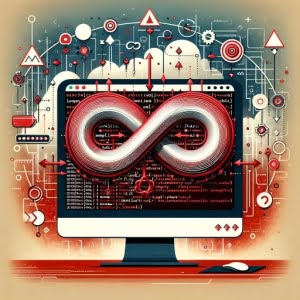
Ever found yourself in a bind trying to create an infinite loop in Bash? You’re not alone. Like a never-ending roller coaster, a Bash infinite loop can keep your code running until you decide to stop it. This can be a powerful tool when used correctly, but also a source of confusion for many.
Think of Bash infinite loops as a marathon race – they keep going until they’re explicitly told to stop. They can be used for a variety of tasks, from monitoring system processes, to keeping a script running indefinitely.
This guide will walk you through the steps to create an infinite loop in Bash, from the basics to more advanced techniques. We’ll cover everything from the simple ‘while’ loop method to alternative approaches, as well as troubleshooting common issues and considerations.
So, let’s dive in and start mastering Bash infinite loops!
TL;DR: How Do I Create an Infinite Loop in Bash?
To create an infinite loop in Bash, you can use the
'while'
loop with the condition set to'true'
,while true
. This will keep the loop running indefinitely until it’s explicitly stopped.
Here’s a simple example:
while true
do
echo 'This is an infinite loop'
done
# Output:
# 'This is an infinite loop'
# 'This is an infinite loop'
# 'This is an infinite loop'
# ...
In this example, we’ve used the ‘while’ loop with the condition set to ‘true’. This means the loop will keep running and echoing ‘This is an infinite loop’ until it’s explicitly stopped by the user.
But Bash’s infinite loop capabilities go far beyond this. Continue reading for more detailed examples and advanced infinite loop techniques.
Table of Contents
- Understanding the ‘While’ Loop: The Foundation of Infinite Loops
- Advanced Infinite Loop Techniques in Bash
- Exploring Alternative Infinite Loop Methods
- Troubleshooting Common Infinite Loop Issues
- Bash Scripting and Loop Structures: A Deeper Dive
- Expanding Infinite Loop Applications
- Wrapping Up: Mastering Infinite Loop Creation in Bash
Understanding the ‘While’ Loop: The Foundation of Infinite Loops
The ‘while’ loop is a key component in creating infinite loops in Bash. It’s a control flow statement that allows code to be executed repeatedly based on a given condition. The loop will continue until the condition is no longer met.
Let’s look at how we can use the ‘while’ loop to create a basic infinite loop. In this example, we will create a loop that continuously prints a countdown from 10.
counter=10
while [ $counter -ge 0 ]
do
echo 'Countdown:' $counter
((counter--))
done
# Output:
# 'Countdown: 10'
# 'Countdown: 9'
# 'Countdown: 8'
# ...
# 'Countdown: 0'
In this code block, we start by setting a counter to 10. The ‘while’ loop then checks if the counter is greater than or equal to 0. If it is, it prints the countdown and decreases the counter by 1. This loop will continue until the counter is no longer greater than or equal to 0, at which point it will stop.
This is a simple example of how a ‘while’ loop works. However, to make this loop infinite, we can set the condition to ‘true’. This will keep the loop running indefinitely, as the condition will always be met.
While infinite loops can be useful in certain scenarios, they also have potential pitfalls. For example, if not handled properly, they can consume system resources and slow down your computer. Therefore, it’s important to use them with caution and ensure you have a way to stop the loop when needed.
Advanced Infinite Loop Techniques in Bash
As you become more comfortable with Bash scripting, you’ll discover that infinite loops can be used in more complex ways. Let’s delve into some of these techniques.
Nested Loops and Infinite Loops
A nested loop is a loop within a loop. When used with infinite loops, nested loops can provide powerful functionality. Here’s an example of a nested infinite loop that prints out a sequence of numbers indefinitely:
outer_counter=1
while true
do
inner_counter=1
while [ $inner_counter -le 5 ]
do
echo "$outer_counter.$inner_counter"
((inner_counter++))
done
((outer_counter++))
done
# Output:
# '1.1'
# '1.2'
# '1.3'
# '1.4'
# '1.5'
# '2.1'
# '2.2'
# '2.3'
# '2.4'
# '2.5'
# ...
In this example, we have an outer loop that runs indefinitely, and an inner loop that runs five times for each iteration of the outer loop. This results in a sequence of numbers that repeats indefinitely.
Controlling Infinite Loops with ‘break’ and ‘continue’
The ‘break’ and ‘continue’ statements provide greater control over your infinite loops. The ‘break’ statement can be used to exit the loop prematurely, while the ‘continue’ statement can be used to skip the remainder of the current loop iteration.
Here’s an example of an infinite loop that uses the ‘break’ statement to exit when a certain condition is met:
counter=1
while true
do
if [ $counter -gt 5 ]
then
break
fi
echo "Counter: $counter"
((counter++))
done
# Output:
# 'Counter: 1'
# 'Counter: 2'
# 'Counter: 3'
# 'Counter: 4'
# 'Counter: 5'
In this example, the loop breaks (stops) when the counter is greater than 5. This demonstrates how you can use the ‘break’ statement to control when your infinite loop should stop.
Handling Signals in Infinite Loops
In Bash, you can handle signals using trap commands. This can be useful in infinite loops to ensure that your script behaves as expected when it receives a signal. For example, you might want your script to clean up temporary files or reset terminal settings when it receives an interrupt signal (Ctrl+C).
Here’s an example of an infinite loop that uses a trap command to handle the interrupt signal:
trap 'echo "Loop interrupted"; exit' INT
while true
do
echo "Running..."
sleep 1
done
# Output:
# 'Running...'
# 'Running...'
# 'Running...'
# ...
# 'Loop interrupted'
In this example, the trap command specifies that when the script receives an interrupt signal (INT), it should print ‘Loop interrupted’ and then exit. This way, you can control what happens when your infinite loop is interrupted.
Exploring Alternative Infinite Loop Methods
While the ‘while’ loop is a go-to for creating infinite loops in Bash, there are other methods you can use, such as ‘for’ and ‘until’ loops. Let’s explore these alternatives.
Infinite Loops Using ‘For’ Loops
Though not as common as ‘while’ loops, ‘for’ loops can also be used to create infinite loops. Here’s an example:
for (( ; ; ))
do
echo 'This is an infinite loop using for loop'
done
# Output:
# 'This is an infinite loop using for loop'
# 'This is an infinite loop using for loop'
# 'This is an infinite loop using for loop'
# ...
In this example, we’ve left the condition for the ‘for’ loop empty, which makes it run indefinitely. This is a less common approach to creating infinite loops, but it’s useful to know that it’s possible.
Infinite Loops Using ‘Until’ Loops
The ‘until’ loop is the opposite of the ‘while’ loop. It runs until the condition is true. By setting the condition to false, we can create an infinite loop. Here’s an example:
until false
do
echo 'This is an infinite loop using until loop'
done
# Output:
# 'This is an infinite loop using until loop'
# 'This is an infinite loop using until loop'
# 'This is an infinite loop using until loop'
# ...
In this example, the ‘until’ loop will keep running because the condition (false) will never be true.
Each of these methods has its own benefits and drawbacks. The ‘while’ loop is straightforward and easy to understand, making it a good choice for beginners. The ‘for’ loop is more flexible and can be used in more complex scenarios, while the ‘until’ loop can be more intuitive in certain situations. Ultimately, the method you choose will depend on your specific needs and the nature of your script.
Troubleshooting Common Infinite Loop Issues
Infinite loops can be a powerful tool in Bash scripting, but they can also lead to some common issues. Let’s explore these problems and how to solve them.
Issue: Loop Running Indefinitely
One common issue is that your loop might run indefinitely, consuming system resources. This is often due to a condition that never becomes false. Here’s an example of a problematic infinite loop:
counter=1
while [ $counter -gt 0 ]
do
echo "Counter: $counter"
((counter++))
done
# Output:
# 'Counter: 1'
# 'Counter: 2'
# 'Counter: 3'
# 'Counter: 4'
# 'Counter: 5'
# ...
In this example, the loop will run indefinitely because the counter will always be greater than 0. To solve this issue, you could add a ‘break’ statement to exit the loop when the counter reaches a certain value.
Issue: Infinite Loop Not Running
Another common issue is that your infinite loop might not run at all. This could be due to a condition that is always false. Here’s an example of an infinite loop that won’t run:
while false
do
echo 'This loop will never run'
done
In this example, the loop will never run because the condition (false) is never true. To solve this issue, you would need to adjust the condition so that it can be true.
Best Practices and Optimization Tips
When working with infinite loops, it’s important to follow some best practices and optimization tips:
- Always make sure you have a way to stop the loop, either by including a ‘break’ statement or by ensuring you can manually interrupt the loop (e.g., with Ctrl+C).
- Be mindful of the system resources your loop might consume. If your loop includes resource-intensive tasks, consider adding a delay (e.g., with the ‘sleep’ command) to prevent the loop from running too quickly.
- Test your loop with a limited number of iterations before letting it run indefinitely. This can help you identify and fix any issues before they cause problems.
Bash Scripting and Loop Structures: A Deeper Dive
Bash scripting is a powerful tool that allows you to automate tasks in a Unix-based system. A key element of Bash scripting is the use of loop structures, which allow code to be executed multiple times. There are several types of loop structures in Bash, including ‘while’, ‘for’, and ‘until’ loops.
In the context of infinite loops, it’s important to understand the concept of ‘truthiness’. In Bash, conditions are evaluated in terms of their ‘truthiness’ or ‘falseness’. A condition is considered ‘true’ if it evaluates to zero, and ‘false’ if it evaluates to anything other than zero.
This concept is crucial when creating infinite loops. For example, in a ‘while’ loop, the loop will continue to run as long as the condition is ‘true’ (i.e., evaluates to zero). By setting the condition to ‘true’ or a non-zero value, you can create a loop that runs indefinitely.
Here’s an example of an infinite loop that uses the ‘truthiness’ concept:
while [ 1 ]
do
echo 'This is an infinite loop'
done
# Output:
# 'This is an infinite loop'
# 'This is an infinite loop'
# 'This is an infinite loop'
# ...
In this example, the condition is set to 1, which is considered ‘true’ in Bash. Therefore, the loop will continue to run indefinitely, printing ‘This is an infinite loop’ until it’s explicitly stopped.
Understanding the fundamentals of Bash scripting and the concept of ‘truthiness’ can help you create more effective infinite loops and troubleshoot any issues that may arise.
Expanding Infinite Loop Applications
Infinite loops are not standalone concepts but rather integral parts of larger scripts or projects. Their applications are diverse and can be found in various real-world scenarios.
Infinite Loops in Larger Projects
In larger projects, infinite loops can be used to continuously monitor system processes, keep a script running indefinitely, or handle user input in interactive scripts. They can also be used in server scripts to keep the server running and listening for incoming connections.
Process Management and Signal Handling
Infinite loops often go hand-in-hand with process management and signal handling. For instance, you might have an infinite loop that runs a certain process and needs to handle signals to stop or restart the process as needed.
Further Resources for Bash Infinite Loop Mastery
To deepen your understanding of infinite loops and related topics, here are some resources you might find helpful:
- GNU Bash Reference Manual: This is the official reference for Bash, and it covers everything from basic syntax to advanced features like arrays and regular expressions.
Advanced Bash-Scripting Guide: This guide offers in-depth information about Bash scripting, including detailed explanations and examples of loops, conditionals, and other key concepts.
Bash Academy: This interactive guide offers a hands-on approach to learning Bash. It covers a wide range of topics, including loops, variables, and functions.
Remember, mastering infinite loops in Bash is not just about understanding the syntax but also about knowing when and how to use them effectively in your scripts.
Wrapping Up: Mastering Infinite Loop Creation in Bash
In this comprehensive guide, we’ve explored the ins and outs of creating infinite loops in Bash, a fundamental concept in Bash scripting that can be a powerful tool when used correctly.
We began with the basics, understanding how to create a simple infinite loop using the ‘while’ loop. We then delved into more advanced applications, exploring nested loops, the use of ‘break’ and ‘continue’ statements, and signal handling. Along the way, we also tackled common issues you might encounter when creating infinite loops, offering solutions and best practices to avoid pitfalls.
We didn’t stop at the ‘while’ loop. We looked at alternative approaches, such as using ‘for’ and ‘until’ loops to create infinite loops. Each method has its own benefits and drawbacks, and understanding these can help you choose the right one for your specific needs.
Here’s a quick comparison of the methods we’ve discussed:
Method | Simplicity | Flexibility | Intuitiveness |
---|---|---|---|
‘while’ loop | High | Moderate | High |
‘for’ loop | Moderate | High | Moderate |
‘until’ loop | Moderate | Moderate | High |
Whether you’re just starting out with Bash scripting or you’re looking to deepen your knowledge, we hope this guide has given you a clearer understanding of how to create and control infinite loops in Bash.
Mastering infinite loops is a key step towards becoming a proficient Bash scripter. With this knowledge, you’re well equipped to tackle more complex scripting tasks. Keep exploring, keep learning, and happy scripting!