Bash Basename Command | Extracting Linux Filenames
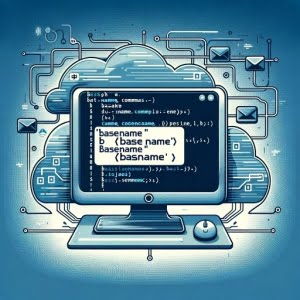
Are you finding it challenging to manage file paths in Bash? You’re not alone. Many developers find themselves in a maze when it comes to handling file paths in Bash, but there’s a tool that can help you navigate this labyrinth.
Like a skilled navigator, the ‘basename’ command in Bash is a powerful tool that can guide you through the maze of directories. It is adept at extracting the last part of a file path, providing you with the filename you need.
In this guide, we’ll walk you through the process of using the ‘basename’ command in Bash, from the basics to more advanced techniques. We’ll cover everything from simple usage of ‘basename’ to handling complex file paths, as well as alternative approaches.
Let’s dive in and start mastering the ‘basename’ command in Bash!
TL;DR: How Do I Use the ‘basename’ Command in Bash?
The
'basename'
command in Bash is used to strip directory and suffix from filenames, for examplebasename /path/to/file
, leaving you with just the base name of the file. Here’s a simple example:
basename /usr/bin/sort
# Output:
sort
In this example, we use the ‘basename’ command on the file path ‘/usr/bin/sort’. The ‘basename’ command strips the directory, ‘/usr/bin/’, from the file path, leaving us with just the base name of the file, ‘sort’.
This is just a basic use of the ‘basename’ command in Bash, but there’s much more to learn about managing file paths efficiently. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with ‘basename’ in Bash
The ‘basename’ command is a fundamental tool in Bash that’s used to extract the last part of a file path, essentially the file name. Let’s break down how to use this command in a basic scenario.
Consider you have a file located at ‘/home/user/documents/file.txt’. If you want to extract just the file name ‘file.txt’ from this path, you can use the ‘basename’ command.
basename /home/user/documents/file.txt
# Output:
file.txt
In this example, ‘basename’ strips away the ‘/home/user/documents/’ part of the path, leaving us with ‘file.txt’. This simple yet powerful command can be incredibly useful when working with file paths in Bash.
One advantage of using ‘basename’ is its simplicity and straightforwardness. It’s a quick and easy way to get the file name from a file path. However, one potential pitfall to be aware of is that ‘basename’ doesn’t actually check if the file or directory exists. It simply processes the text string you provide it.
So, if you were to run the command ‘basename /this/path/does/not/exist’, it would still return ‘exist’ as the output, even though there’s no such file or directory.
basename /this/path/does/not/exist
# Output:
exist
This is something to keep in mind when using ‘basename’ in your scripts. In the next section, we’ll delve into more complex uses of the ‘basename’ command.
Advanced Usage of ‘basename’ in Bash
As we delve deeper into the ‘basename’ command, you’ll find it’s capable of more than just extracting the file name from a file path. It can also remove file extensions, making it a versatile tool for file path manipulation.
Let’s say you have a file named ‘report.txt’ and you want to remove the ‘.txt’ extension. Here’s how you can do it with ‘basename’:
basename /home/user/documents/report.txt .txt
# Output:
report
In this example, we provide two arguments to the ‘basename’ command. The first is the file path, and the second is the extension we want to remove. The ‘basename’ command strips away both the directory and the ‘.txt’ extension, leaving us with ‘report’.
This feature of ‘basename’ can be particularly useful when you’re working with a lot of files of the same type and you want to perform operations on them without their extensions.
Another advanced use of ‘basename’ involves managing file paths that include special characters. Let’s consider a file path that includes spaces:
basename '/home/user/my documents/report.txt' .txt
# Output:
report
Even with the space in the file path, ‘basename’ successfully extracts the file name without the extension.
These examples illustrate the versatility and power of the ‘basename’ command in Bash. It’s not just about getting the file name; it’s about managing and manipulating file paths effectively.
Alternative Methods to Extract File Names
While the ‘basename’ command is an efficient tool for extracting the last part of a file path, there are other approaches in Bash that offer similar functionality. Two such alternatives are ‘awk’ and ‘sed’. Let’s explore these methods and how they can be used to manipulate file paths.
Using ‘awk’ to Extract File Names
‘awk’ is a powerful text-processing command in Unix-like operating systems. You can use it to extract file names from file paths. Here’s an example:
echo '/home/user/documents/report.txt' | awk -F/ '{print $NF}'
# Output:
report.txt
In this example, we use ‘awk’ with the -F option to specify ‘/’ as the field separator. The ‘{print $NF}’ command prints the last field, which is the file name.
Extracting File Names with ‘sed’
‘sed’ stands for Stream EDitor. It’s another tool you can use to manipulate text, which includes extracting file names from file paths. Here’s how you can do it:
echo '/home/user/documents/report.txt' | sed 's|.*/||'
# Output:
report.txt
In this example, we use ‘sed’ with the ‘s’ command for substitution. The ‘.*/’ pattern matches everything up to the last ‘/’, and it’s replaced with nothing, effectively leaving us with the file name.
Both ‘awk’ and ‘sed’ provide powerful alternatives to ‘basename’, but they come with a steeper learning curve. If you’re comfortable with regular expressions and text processing in Bash, these tools offer great flexibility and can handle more complex scenarios than ‘basename’. However, for simple file name extraction, ‘basename’ is often the easiest and most straightforward choice.
While ‘basename’ is a powerful tool, like any command, it has its quirks and potential pitfalls. This section will discuss some common issues you may encounter when using ‘basename’ and provide solutions and workarounds.
Handling Special Characters in File Paths
One common issue arises when dealing with file paths that include special characters. For instance, consider a file path with a space:
basename /home/user/my documents/report.txt
# Output:
my documents/report.txt
In this case, ‘basename’ doesn’t correctly identify the base name due to the space in the file path. To handle this, you can enclose the file path in quotes:
basename "/home/user/my documents/report.txt"
# Output:
report.txt
By using quotes, we ensure that ‘basename’ treats the entire string as a single argument, correctly identifying ‘report.txt’ as the base name.
Dealing with Paths that End with a Slash
Another issue arises when the file path ends with a ‘/’. ‘basename’ treats the trailing ‘/’ as part of the file path, which can lead to unexpected results:
basename /home/user/documents/
# Output:
documents
In this case, ‘basename’ returns ‘documents’ as the base name, which might not be what you expect. To avoid this, you can trim the trailing ‘/’ before passing the path to ‘basename’.
These are just a couple of the potential issues you might encounter when using the ‘basename’ command. By being aware of these quirks and knowing how to handle them, you can use ‘basename’ more effectively in your scripts.
Understanding File Paths in Bash
To fully grasp the utility of the ‘basename’ command, it’s important to understand the concept of file paths in Bash. A file path in Bash is a string that specifies the location of a file or a directory in a file system.
Absolute vs Relative Paths
There are two types of file paths: absolute and relative.
An absolute path starts with the root directory (‘/’) and provides a complete path to the file or directory. For instance:
echo /home/user/documents/report.txt
# Output:
/home/user/documents/report.txt
A relative path, on the other hand, starts from the current directory. It doesn’t provide the complete path from the root directory. For example:
cd /home/user/documents
pwd
# Output:
/home/user/documents
Here, if you want to refer to ‘report.txt’, you can simply use its name since it’s in the current directory.
Navigating Directories with ‘..’ and ‘.’
In Bash, ‘.’ refers to the current directory, while ‘..’ refers to the parent directory. For instance, if you’re in ‘/home/user/documents’ and want to go back to ‘/home/user’, you can use ‘..’.
cd ..
pwd
# Output:
/home/user
Understanding these fundamentals of file paths in Bash can help you better understand how the ‘basename’ command works and why it’s so useful in scripting and everyday command line usage.
The ‘basename’ Command in the Larger Context
The ‘basename’ command in Bash, while simple, plays a vital role in larger scripts or projects. Its ability to extract the last part of a file path makes it an essential tool in file manipulation and directory navigation in Bash.
‘basename’ in Scripting
In scripting, ‘basename’ can be used to handle file paths dynamically. For instance, if a script takes a file path as an input, ‘basename’ can be used to extract just the file name, regardless of the directory structure. This allows the script to work with just the file name, making it more flexible and adaptable.
Exploring Related Concepts
While ‘basename’ is a powerful tool, it’s just one piece of the puzzle. To effectively manage file paths in Bash, you should also familiarize yourself with related concepts such as directory navigation (cd, pwd), file manipulation (cp, mv, rm), and other commands like ‘dirname’ that work with file paths.
Further Resources for Bash Mastery
To deepen your understanding of Bash and its various commands, check out these resources:
- GNU Bash Manual: This is the official manual for Bash. It’s comprehensive and detailed, making it a great resource for anyone looking to master Bash.
GeeksforGeeks – basename command in Linux with examples: This guide explains the usage of the
basename
command in Linux with examples.Bash Scripting Guide: This guide offers a deep dive into Bash scripting, covering everything from beginner to advanced topics.
Bash, with its extensive set of commands and features, offers a powerful platform for system administration and scripting. By mastering commands like ‘basename’ and understanding the fundamentals of file paths, you can navigate the Bash command line with ease and confidence.
Wrapping Up: Mastering the ‘basename’ Command in Bash
In this comprehensive guide, we’ve explored the ‘basename’ command in Bash, a powerful tool for extracting the last part of a file path, essentially giving you the file name.
We kicked off with the basics, learning how to use ‘basename’ to strip directory and suffix from filenames. We then took a deep dive into more advanced usage, exploring how ‘basename’ can handle complex file paths and even remove file extensions.
Along the way, we tackled common challenges you might encounter when using ‘basename’, such as dealing with special characters in file paths or paths that end with a ‘/’. We provided solutions and workarounds to help you navigate these potential pitfalls.
We also looked at alternative approaches to handling file paths in Bash, comparing ‘basename’ with powerful text-processing commands like ‘awk’ and ‘sed’. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
basename | Simple and straightforward | Doesn’t check if file or directory exists |
awk | Powerful text-processing tool | Steeper learning curve |
sed | Great for text manipulation | Requires understanding of regular expressions |
Whether you’re a Bash beginner or an experienced scripter looking for a refresher, we hope this guide has given you a deeper understanding of the ‘basename’ command and its capabilities.
With its simplicity and versatility, ‘basename’ is a valuable tool in your Bash toolkit. It’s not just about getting the file name; it’s about managing and manipulating file paths effectively. Happy scripting!