How to Exit Bash Scripts Effectively: Linux Shell Guide
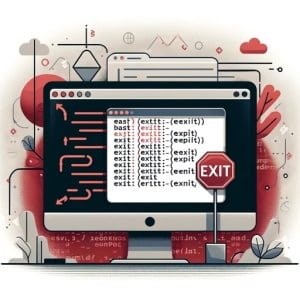
Ever found yourself in a maze while trying to exit a Bash script? You’re not alone. Many developers find themselves puzzled when it comes to gracefully terminating a Bash script. Think of Bash’s exit command as a secret door – a door that allows you to leave the script when you want.
The exit command is a powerful tool to control the flow of your Bash scripts, making it extremely popular for managing script execution.
In this guide, we’ll walk you through the process of exiting a Bash script, from the basics to more advanced techniques. We’ll cover everything from using the simple ‘exit’ command, understanding exit statuses, to dealing with common issues and even exploring alternative approaches.
Let’s jump right in and learn to exit Bash scripts effectively!
TL;DR: How Do I Exit a Bash Script?
To exit a Bash script, you use the
'exit'
command. This command allows you to terminate a script at any point and optionally return a status.
Here’s a simple example:
#!/bin/bash
# This is a simple bash script
echo 'Hello, World!'
# Exit the script
exit
# Output:
# 'Hello, World!'
In this example, we’ve created a simple Bash script that prints ‘Hello, World!’ to the console. After printing the message, the ‘exit’ command is used to terminate the script. If there were any commands after ‘exit’, they would not be executed.
This is just a basic way to use the ‘exit’ command in Bash, but there’s much more to learn about managing script execution. Continue reading for more detailed understanding and advanced usage scenarios.
Table of Contents
- Understanding the ‘Exit’ Command in Bash Scripts
- Leveraging Exit Statuses in Bash Scripts
- Exploring Alternatives: ‘Return’ and ‘Kill’ Commands
- Troubleshooting Common Issues with Bash Exit Scripts
- The Basics of Bash Scripting and Process Termination
- The Impact of Exit Commands in Larger Projects
- Wrapping Up: Mastering Bash Exit Commands
Understanding the ‘Exit’ Command in Bash Scripts
The ‘exit’ command is a built-in command in Bash that allows you to end a script at any point. When the ‘exit’ command is executed, the script stops running, and control is returned to the command line. This command is particularly useful when you want to stop the script due to an error condition.
Here’s a simple example of how the ‘exit’ command works:
#!/bin/bash
# This is a bash script that checks if a file exists
FILE=/tmp/somefile
if [[ -e $FILE ]]; then
echo 'File exists!'
else
echo 'File does not exist!'
exit 1
fi
# Output:
# 'File does not exist!'
In this script, we’re checking if a file exists at a specific location. If the file doesn’t exist, we print a message and then use the ‘exit’ command to terminate the script. The ‘1’ after ‘exit’ is an exit status. In this case, ‘1’ indicates that an error occurred.
The ‘exit’ command is a powerful tool in Bash scripting as it provides a way to manage the flow of scripts. However, it’s essential to use it wisely. Overuse of the ‘exit’ command can make a script difficult to read and debug. It’s usually best to use ‘exit’ sparingly and only when necessary, such as when an error occurs that the script cannot recover from.
Leveraging Exit Statuses in Bash Scripts
In Bash scripting, the ‘exit’ command can also return a status when the script ends. This exit status, also known as a return status or exit code, is an integer number that represents the success or failure of the last executed command or script.
By convention, an exit status of ‘0’ indicates success, while a non-zero status signifies an error or abnormal termination. You can specify the exit status as an argument to the ‘exit’ command, like ‘exit 1’, ‘exit 2’, etc.
Let’s see an example:
#!/bin/bash
# This script checks if a directory exists
DIR=/tmp/somedir
if [[ -d $DIR ]]; then
echo 'Directory exists!'
exit 0
else
echo 'Directory does not exist!'
exit 1
fi
# Output:
# 'Directory does not exist!'
In this script, we’re checking if a directory exists at a specific location. If the directory exists, we print a message and then use the ‘exit’ command with a status of ‘0’ to indicate success. If the directory doesn’t exist, we print a message and exit with a status of ‘1’ to signal an error.
These exit statuses can be particularly useful when your script is part of a larger system where other scripts or processes need to know whether your script completed successfully or not. By using different exit statuses, you can communicate a variety of outcomes to whatever process invoked your script.
Exploring Alternatives: ‘Return’ and ‘Kill’ Commands
While the ‘exit’ command is the most common way to terminate a Bash script, there are alternative methods that you can use depending on your specific needs. Two such alternatives are the ‘return’ and ‘kill’ commands.
Using the ‘Return’ Command
The ‘return’ command is generally used to terminate a function and return a value to the caller. If it’s used outside a function, it behaves like ‘exit’. Here’s an example:
#!/bin/bash
# Define a function
function test {
echo 'Inside the function'
return 1
}
test
echo 'Outside the function'
# Output:
# 'Inside the function'
# 'Outside the function'
In this script, we define a function ‘test’. Inside the function, we print a message and then use the ‘return’ command to end the function and return a value of ‘1’. After calling the function, we print another message.
Using the ‘Kill’ Command
The ‘kill’ command is used to send a signal to a process. By default, it sends the TERM (terminate) signal, which requests a process to stop. However, you can use it with the -9 option (KILL signal) to forcefully stop a process. Here’s an example:
#!/bin/bash
# Start a background process
sleep 60 &
# Get its PID
PID=$!
# Kill the process
kill -9 $PID
# Output:
# No output, but the sleep process is terminated
In this script, we start a ‘sleep’ process in the background, which simply pauses for 60 seconds. We then get its process ID (PID) using the special variable ‘$!’. Finally, we use the ‘kill’ command with the -9 option to forcefully stop the sleep process.
Both the ‘return’ and ‘kill’ commands offer alternative ways to manage script execution. However, they should be used judiciously as they can make scripts more complex and harder to debug.
Troubleshooting Common Issues with Bash Exit Scripts
When working with Bash scripts, you may encounter a few issues that can prevent your script from exiting correctly. Let’s discuss some of the common issues and how to troubleshoot them.
‘Command Not Found’ Error
This error typically occurs when Bash cannot locate the command you’re trying to execute. It could be due to a typo in the command name or the command might not be installed on your system.
For instance, consider the following script:
#!/bin/bash
# This script tries to use a non-existent command
non_existent_command
# Output:
# 'non_existent_command: command not found'
In this script, we’re trying to use a command that doesn’t exist, so Bash throws a ‘command not found’ error.
Problems with Exit Statuses
Exit statuses can sometimes be misleading. For instance, if a command in your script fails but the last command before the ‘exit’ command succeeds, the exit status will be ‘0’, indicating success.
Consider the following script:
#!/bin/bash
# This script has a failing command but still exits with status 0
non_existent_command
echo 'This is the end of the script'
# Output:
# 'non_existent_command: command not found'
# 'This is the end of the script'
In this script, the ‘non_existent_command’ fails, but the ‘echo’ command succeeds, causing the script to exit with a status of ‘0’. To avoid this, you can use the ‘set -e’ command at the beginning of your script, which causes the script to exit immediately if any command exits with a non-zero status.
#!/bin/bash
# This script will exit immediately if any command fails
set -e
non_existent_command
echo 'This is the end of the script'
# Output:
# 'non_existent_command: command not found'
In this modified script, the ‘set -e’ command causes the script to exit as soon as the ‘non_existent_command’ fails, and the ‘echo’ command is never executed.
The Basics of Bash Scripting and Process Termination
Bash (Bourne Again SHell) is a popular command-line interpreter or shell. It provides a robust and powerful environment for executing commands and scripts on Unix-like operating systems, including Linux and macOS.
A Bash script is a text file containing a series of commands. When you run the script, Bash reads the file and executes the commands in order.
Now, let’s introduce the concept of a process. In computing, a process is an instance of a program that is being executed. Each process has a unique identifier known as the Process ID (PID).
When a Bash script is running, it’s considered a process. The process continues until the script has executed all the commands, or it encounters an exit command, or an error occurs that it can’t handle.
Here’s a simple Bash script:
#!/bin/bash
# This is a simple bash script
echo 'Hello, World!'
# Output:
# 'Hello, World!'
In this script, we have a single command that prints ‘Hello, World!’. When we run this script, Bash starts a process, executes the ‘echo’ command, and then the process ends because there are no more commands.
Understanding Exit Statuses
An exit status is a numerical value returned by a command or script to indicate its success or failure. By convention, an exit status of ‘0’ means success, and any non-zero value indicates an error.
The exit status is an essential concept in Bash scripting because it allows us to control the flow of execution based on the success or failure of commands. For instance, we can use the exit status to decide whether to continue executing the rest of the script or to exit early.
Here’s an example of using the exit status:
#!/bin/bash
# This script checks if a file exists and prints a message based on the result
FILE=/tmp/somefile
if [[ -e $FILE ]]; then
echo 'File exists!'
exit 0
else
echo 'File does not exist!'
exit 1
fi
# Output:
# 'File does not exist!'
In this script, we’re checking if a file exists at a specific location. If the file exists, we print a message and then use the ‘exit’ command with a status of ‘0’ to indicate success. If the file doesn’t exist, we print a message and exit with a status of ‘1’ to signal an error.
The Impact of Exit Commands in Larger Projects
The ability to control the flow of a Bash script using the ‘exit’ command is a powerful tool, especially when working on larger scripts or projects. In complex scripts, proper use of the ‘exit’ command can help prevent unexpected behavior, simplify debugging, and make the script more maintainable.
In larger projects, scripts often interact with each other. A script might call another script, wait for it to finish, and then act based on its exit status. In such scenarios, the correct use of exit statuses becomes crucial. An incorrect exit status can lead to a cascade of errors and make the project difficult to manage.
Exploring Related Concepts
If you’re interested in diving deeper into Bash scripting, there are several related concepts that you might find intriguing. Signal handling in Bash, for instance, can provide you with greater control over how your scripts respond to various events. Process management is another fascinating topic that can help you understand and manage the various processes that your scripts might spawn.
Further Resources for Bash Scripting Mastery
Here are some resources that can help you further explore Bash scripting and related concepts:
- Advanced Bash-Scripting Guide: An in-depth exploration of Bash scripting, including advanced topics.
Bash Guide for Beginners: A comprehensive guide for those new to Bash scripting.
GNU Bash Manual: The official manual for Bash from the GNU project.
Wrapping Up: Mastering Bash Exit Commands
In this comprehensive guide, we’ve delved into the process of exiting a Bash script, exploring everything from the basic ‘exit’ command to more advanced techniques and alternatives.
We began with the fundamentals, understanding how the ‘exit’ command works in Bash scripts. We then learned how to leverage exit statuses to communicate the outcome of our scripts. We also explored alternative methods for exiting a script, such as using the ‘return’ and ‘kill’ commands.
Along the journey, we tackled common issues you might encounter when working with Bash exit scripts, such as ‘command not found’ errors and problems with exit statuses. We provided solutions and workarounds to help you overcome these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Use Case | Complexity |
---|---|---|
‘exit’ | General purpose, most common | Low |
‘return’ | Within functions or sourced scripts | Medium |
‘kill’ | To terminate other processes | High |
Whether you’re just starting out with Bash scripting or you’re a seasoned developer looking to refine your skills, we hope this guide has given you a deeper understanding of how to effectively manage the termination of your Bash scripts.
The ability to control the flow and termination of a Bash script is a powerful tool in your scripting arsenal. With the knowledge you’ve gained, you’re well equipped to write robust and efficient Bash scripts. Happy scripting!