How To Check a Key Exists in a Python Dictionary
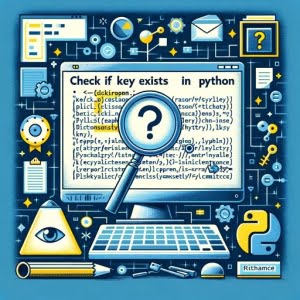
Ever found yourself in a situation where you needed to check if a key exists in a Python dictionary? Just like a detective searching for clues, Python offers several ways to investigate if a specific key is tucked away in a dictionary.
Whether you’re a novice programmer or someone with a bit more experience under your belt, this guide is designed to walk you through the process. We’ll start from the basics and gradually delve into more advanced techniques.
So, if you’re ready to become a Python dictionary sleuth, let’s get started!
TL;DR: How Do I Check if a Key Exists in a Python Dictionary?
You can use the
in
keyword to check if a key exists in a Python dictionary. Let’s look at a simple example:
my_dict = {'apple': 1, 'banana': 2}
if 'apple' in my_dict:
print('Key exists')
else:
print('Key does not exist')
# Output:
# Key exists
In this example, we create a dictionary named my_dict
with two key-value pairs. We then use the in
keyword to check if the key ‘apple’ exists in our dictionary. If it does, ‘Key exists’ is printed. If not, ‘Key does not exist’ is printed. In this case, since ‘apple’ is a key in our dictionary, ‘Key exists’ is printed.
Stay tuned for more detailed explanations and advanced usage scenarios. We’ll be diving deeper into the world of Python dictionaries and how to effectively check for key existence.
Table of Contents
- Key Existence Check: The Basic Use
- Diving Deeper: Advanced Key Checks
- Exploring Alternatives: The has_key() Method and More
- Troubleshooting Common Issues and Considerations
- Python Dictionaries and Keys: The Fundamentals
- Beyond Key Checks
- Further Resources for Dictionaries in Python
- Key Checks in Python Dictionaries: A Recap
Key Existence Check: The Basic Use
One of the simplest and most common ways to check if a key exists in a Python dictionary is by using the in
keyword. The in
keyword in Python is used to check if a value exists in a sequence like a list, tuple, etc., or as in our case, if a key exists in a dictionary. Let’s take a look at a basic example:
# Define a simple dictionary
my_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
# Check if 'banana' is a key in the dictionary
if 'banana' in my_dict:
print('Key exists')
else:
print('Key does not exist')
# Output:
# Key exists
In this example, we have a dictionary my_dict
with three key-value pairs. We’re using the in
keyword to check if the key ‘banana’ exists in our dictionary. If it does, ‘Key exists’ is printed, and if it doesn’t, ‘Key does not exist’ is printed. As ‘banana’ is indeed a key in our dictionary, the output is ‘Key exists’.
The in
keyword is a fast and efficient way to check for key existence in a dictionary. It’s simple to use and easy to read, making it a popular choice among Python developers.
Diving Deeper: Advanced Key Checks
While using the in
keyword is straightforward for checking a single key, what if we need to check for multiple keys? Or perhaps we need to employ other dictionary methods like get()
or items()
. Let’s explore these scenarios.
Checking for Multiple Keys
Suppose we have a list of keys, and we want to check if all these keys exist in our dictionary. Here’s how we can do it:
# Define a dictionary and a list of keys
my_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
keys_to_check = ['apple', 'banana', 'dragonfruit']
# Check if all keys in the list exist in the dictionary
if all(key in my_dict for key in keys_to_check):
print('All keys exist')
else:
print('Not all keys exist')
# Output:
# Not all keys exist
In this example, we’re using the all()
function along with a generator expression to check if all keys in keys_to_check
exist in my_dict
.
The all()
function returns True
if all elements in the passed iterable are true. Otherwise, it returns False
. Since ‘dragonfruit’ is not a key in our dictionary, the output is ‘Not all keys exist’.
Using the get()
Method
The get()
method is another way to check if a key exists in a dictionary. This method returns the value for the given key if it exists. If not, it returns a default value that you can specify. Here’s an example:
# Define a dictionary
my_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
# Use get() to check if 'banana' is a key in the dictionary
result = my_dict.get('banana', 'Key does not exist')
print(result)
# Output:
# 2
In this case, ‘banana’ is a key in our dictionary, so the get()
method returns its associated value, which is 2. If ‘banana’ was not a key in the dictionary, the method would return ‘Key does not exist’.
These advanced techniques give you more flexibility when working with Python dictionaries and key checks. They allow you to handle more complex situations and make your code more efficient and readable.
Exploring Alternatives: The has_key()
Method and More
While the in
keyword and get()
method are popular choices for checking if a key exists in a Python dictionary, there are other alternatives that offer different advantages.
One such method is has_key()
. However, it’s important to note that has_key()
is not available in Python 3 and later versions. It was used in Python 2.x versions and is now considered outdated. Here’s how it worked:
# This is a Python 2.x example
my_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
if my_dict.has_key('banana'):
print('Key exists')
else:
print('Key does not exist')
# Output:
# Key exists
In Python 2.x, has_key()
was a built-in method of dictionary objects. It took a key as an argument and returned True
if the key existed in the dictionary and False
otherwise.
Despite its simplicity,
has_key()
was removed in Python 3 due to its redundancy with thein
keyword. Thein
keyword is more Pythonic, readable, and versatile, as it can be used with any iterable, not just dictionaries. Therefore, it’s recommended to use thein
keyword or theget()
method for checking if a key exists in a Python dictionary.
Troubleshooting Common Issues and Considerations
While checking if a key exists in a Python dictionary is a straightforward task, it’s not without its potential pitfalls. Here, we’ll discuss some common issues you may encounter and their solutions.
KeyError
One of the most common issues when working with Python dictionaries is the KeyError
. This error occurs when you try to access a dictionary key that doesn’t exist. For example:
# Define a dictionary
my_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
# Try to access a non-existent key
print(my_dict['dragonfruit'])
# Output:
# KeyError: 'dragonfruit'
In this example, we’re trying to access the key ‘dragonfruit’, which doesn’t exist in our dictionary. As a result, Python raises a KeyError
. To avoid this, always check if a key exists in the dictionary before trying to access it.
Checking for Keys in Nested Dictionaries
Another common scenario is checking for keys in nested dictionaries. The in
keyword only checks for keys in the top-level dictionary, not in any nested dictionaries. Here’s an example:
# Define a nested dictionary
my_dict = {'fruits': {'apple': 1, 'banana': 2}, 'vegetables': {'carrot': 1, 'peas': 2}}
# Check if 'apple' is a key in the dictionary
if 'apple' in my_dict:
print('Key exists')
else:
print('Key does not exist')
# Output:
# Key does not exist
In this case, even though ‘apple’ is a key in the nested dictionary under ‘fruits’, the in
keyword doesn’t find it because it’s not a key in the top-level dictionary. To check for keys in nested dictionaries, you’ll need to write additional code to iterate over the nested dictionaries.
Checking if a key exists in a Python dictionary is a common operation, but it’s important to be aware of the potential issues and how to handle them. With these troubleshooting tips and considerations, you’ll be better equipped to deal with any challenges that come your way.
Python Dictionaries and Keys: The Fundamentals
Before we delve further into how to check if a key exists in a Python dictionary, let’s take a step back and understand the basics of Python dictionaries and keys.
A Python dictionary is an unordered collection of items. Each item is stored as a key-value pair. The keys in a dictionary are unique and immutable. This means that once a key is assigned a value, it can’t be changed. Values, on the other hand, can be of any type and can be modified. Here’s an example of a Python dictionary:
# Define a dictionary
my_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
# Print the dictionary
print(my_dict)
# Output:
# {'apple': 1, 'banana': 2, 'cherry': 3}
In this example, ‘apple’, ‘banana’, and ‘cherry’ are keys, and 1, 2, and 3 are their respective values.
Checking if a key exists in a dictionary is a common operation in Python. It’s used in various scenarios, such as updating the value of an existing key, adding a new key-value pair if the key doesn’t exist, or performing some action if the key exists.
Understanding the role of keys in a dictionary will help you better grasp the methods and techniques we’ve discussed for checking if a key exists in a Python dictionary.
Beyond Key Checks
Ddictionaries are often used to represent real-world data in applications, such as a user’s profile in a social networking app. In these scenarios, checking for key existence becomes vital when updating a user’s profile information or retrieving it.
In addition to key checks, there are several related concepts that you might find interesting. Dictionary manipulation in Python, for instance, includes tasks like adding or updating key-value pairs, deleting keys, merging dictionaries, and more.
Here’s an example of adding a new key-value pair to a dictionary:
# Define a dictionary
my_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
# Add a new key-value pair
my_dict['dragonfruit'] = 4
# Print the updated dictionary
print(my_dict)
# Output:
# {'apple': 1, 'banana': 2, 'cherry': 3, 'dragonfruit': 4}
In this example, we’re adding the key ‘dragonfruit’ with the value 4 to our dictionary. The updated dictionary now includes this new key-value pair.
Exploring data structures in Python, such as lists, tuples, and sets, can also enhance your understanding of Python programming. Each data structure has its own characteristics and use cases, making Python a versatile language for data manipulation and analysis.
Further Resources for Dictionaries in Python
For a deeper understanding of these concepts, here are a handful of resources available online.
- Exploring the Power of Python Dictionaries – Discover how Python dictionaries can simplify your code and enhance data manipulation in this IOFlood tutorial.
Building Blocks of Python Code – How to Create Dictionaries – Master the process of creating dictionaries in Python.
Python Dictionary Append – Expanding Your Data Sets – Dive into Python dictionary updates and learn how to add key-value pairs.
Documentation on Data Structures – This is Python.org’s comprehensive guide to data structures, covering lists, sets, dictionaries, and more.
The keys(), values(), and items() methods in Python Dictionary – This Medium blog post explains the usage of keys(), values(), and items() methods in Python dictionaries, complete with code examples.
Reference on Dictionary items() Method – This tutorial by W3schools explains the ‘items()’ method for Python dictionaries, providing clear explanations and examples.
Continual learning and exploration will help you become a more proficient Python programmer.
Key Checks in Python Dictionaries: A Recap
We’ve journeyed through the process of checking if a key exists in a Python dictionary, starting from the basics and moving on to more advanced techniques.
We explored how the in
keyword allows you to easily check for a single key, and how it can be combined with the all()
function for checking multiple keys.
We also delved into the get()
method, which not only checks for a key’s existence, but also fetches its value, providing a two-in-one functionality.
We even took a detour into the past with the has_key()
method from Python 2.x, which despite being outdated, offered a glimpse into the evolution of Python.
Recap of functions:
# Recap of key-check methods
my_dict = {'apple': 1, 'banana': 2, 'cherry': 3}
# Using 'in'
print('apple' in my_dict) # Output: True
# Using 'get()'
print(my_dict.get('banana')) # Output: 2
# Python 2.x 'has_key()' method
# print(my_dict.has_key('cherry')) # Output: True (only in Python 2.x)
We also addressed common issues like KeyError
and the limitations of the in
keyword with nested dictionaries, offering solutions and considerations to avoid these pitfalls.
Whether you’re a beginner just starting out with Python or an intermediate programmer looking to solidify your understanding, knowing how to check if a key exists in a Python dictionary is a valuable skill in your programming arsenal. Remember, continual learning and exploration is the key to growing as a Python programmer.