Learn Python: How To Add to Dictionary (With Examples)
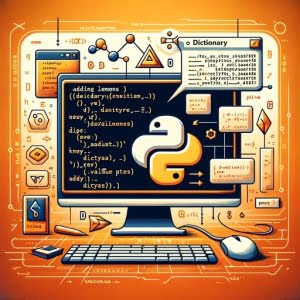
Are you finding it difficult to add items to a dictionary in Python? Don’t fret. Just like adding a new word to a physical dictionary, Python allows you to add new key-value pairs to your digital dictionary.
This guide is designed to simplify the process for you, walking you through the basics and gradually leading up to more advanced techniques.
So, let’s dive right in and master the art of adding items to dictionaries in Python.
TL;DR: How Do I Add an Item to a Dictionary in Python?
You can add an item to a dictionary in Python by assigning a value to a new key. Here’s a simple example:
my_dict = {}
my_dict['new_key'] = 'new_value'
print(my_dict)
# Output:
# {'new_key': 'new_value'}
In this example, we first created an empty dictionary with {}
. Then, we added a new key-value pair to the dictionary by using the syntax my_dict['new_key'] = 'new_value'
. When we print the dictionary, we can see that the new key-value pair has been added.
If you’re interested in more detailed explanations, advanced usage scenarios, or alternative approaches to adding items to a dictionary in Python, keep reading. We’ve got a lot more in store for you.
Table of Contents
- Adding a Single Item to a Python Dictionary
- Adding Multiple Items and Updating a Python Dictionary
- Exploring Alternative Methods for Dictionary Addition in Python
- Troubleshooting Common Issues in Python Dictionary Addition
- Understanding Python’s Dictionary Data Type
- Expanding Your Python Dictionary Skills
- Wrapping Up: Python Dictionary Addition
Adding a Single Item to a Python Dictionary
To add an item to a dictionary in Python, you assign a value to a new key. Let’s break this down with a simple example:
my_dict = {}
my_dict['fruit'] = 'apple'
print(my_dict)
# Output:
# {'fruit': 'apple'}
In this code block, we first create an empty dictionary called my_dict
using the {}
syntax. We then add a new key-value pair to the dictionary with the line my_dict['fruit'] = 'apple'
. Here, ‘fruit’ is the key and ‘apple’ is the value. When we print my_dict
, we see that it now contains the key-value pair {'fruit': 'apple'}
.
This method of adding items to a dictionary is straightforward and efficient. However, it’s important to note that if you use a key that already exists in the dictionary, the old value will be replaced with the new one. This is because keys in a Python dictionary are unique. So, when using this method, make sure the key you’re using does not already exist in the dictionary, unless your intention is to update the value.
Adding Multiple Items and Updating a Python Dictionary
Python makes it easy to add multiple items to a dictionary or update an existing item. Let’s explore how to do this with a code example:
my_dict = {'fruit': 'apple', 'vegetable': 'carrot'}
new_items = {'animal': 'dog', 'fruit': 'banana'}
my_dict.update(new_items)
print(my_dict)
# Output:
# {'fruit': 'banana', 'vegetable': 'carrot', 'animal': 'dog'}
In this example, we start with a dictionary my_dict
that already has a couple of key-value pairs. We then create a second dictionary new_items
with new key-value pairs to add to my_dict
. The update()
method is used to add these items to my_dict
. Note that one of the keys in new_items
(‘fruit’) is the same as a key in my_dict
. The update()
method replaces the value of ‘fruit’ in my_dict
with the value of ‘fruit’ in new_items
.
The update()
method is a powerful tool for adding multiple items to a dictionary or updating an existing item. However, it’s important to remember that if a key in the dictionary you’re updating matches a key in the dictionary you’re adding, the value of the key in the original dictionary will be replaced with the value from the new dictionary. This behavior is consistent with the rule that keys in a Python dictionary must be unique.
Exploring Alternative Methods for Dictionary Addition in Python
Python offers more than one way to add items to a dictionary. Besides the basic method and the update()
method, there are a few more advanced techniques that Python pros often use. Let’s take a look at these alternative methods.
Dictionary Comprehension
Dictionary comprehension is a concise and efficient way to create a new dictionary or add items to an existing one. Here’s an example:
my_dict = {f'key{i}': f'value{i}' for i in range(1, 4)}
print(my_dict)
# Output:
# {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'}
In this code block, we create a new dictionary using dictionary comprehension. The expression f'key{i}': f'value{i}'
generates key-value pairs, and for i in range(1, 4)
repeats the process three times. The result is a dictionary with three key-value pairs.
The setdefault()
Method
The setdefault()
method is another way to add items to a dictionary. This method only adds a new item if the key does not already exist in the dictionary. Here’s how it works:
my_dict = {'fruit': 'apple'}
my_dict.setdefault('fruit', 'banana')
my_dict.setdefault('vegetable', 'carrot')
print(my_dict)
# Output:
# {'fruit': 'apple', 'vegetable': 'carrot'}
In this example, the setdefault()
method attempts to add ‘banana’ to the key ‘fruit’ and ‘carrot’ to the key ‘vegetable’. However, since ‘fruit’ already exists in the dictionary, ‘banana’ is not added. On the other hand, since ‘vegetable’ does not exist, ‘carrot’ is added.
Method | Advantages | Disadvantages |
---|---|---|
Basic | Simple, direct | Overwrites existing values |
update() | Adds multiple items, updates existing items | Overwrites existing values |
Dictionary comprehension | Efficient, concise | Can be complex for beginners |
setdefault() | Does not overwrite existing values | Only adds one item at a time |
Each method has its advantages and disadvantages, and the best one to use depends on your specific needs. The basic method and update()
method are straightforward and work well for most tasks, but they will overwrite existing values. Dictionary comprehension is efficient and concise, but it might be complex for beginners. The setdefault()
method won’t overwrite existing values, but it can only add one item at a time.
Troubleshooting Common Issues in Python Dictionary Addition
When adding items to a dictionary in Python, you might encounter a few common issues. Let’s discuss these problems and how to solve them.
Key Errors
A Key Error occurs when you try to access a key that does not exist in the dictionary. Here’s an example:
my_dict = {'fruit': 'apple'}
print(my_dict['animal'])
# Output:
# KeyError: 'animal'
In this code block, we try to print the value of the key ‘animal’, but ‘animal’ does not exist in my_dict
. This results in a KeyError. To avoid this, you can check if a key exists in the dictionary before trying to access its value.
my_dict = {'fruit': 'apple'}
if 'animal' in my_dict:
print(my_dict['animal'])
else:
print('Key not found')
# Output:
# Key not found
Type Errors
A Type Error can occur if you try to use an unhashable type, like a list or a dictionary, as a dictionary key. Here’s an example:
my_dict = {}
my_dict[['fruit']] = 'apple'
# Output:
# TypeError: unhashable type: 'list'
In this code block, we try to use a list ['fruit']
as a key, which results in a TypeError. To avoid this, only use hashable types (like numbers, strings, and tuples) as dictionary keys.
When working with dictionaries in Python, it’s important to understand these common issues and how to solve them. This will help you write more robust code and troubleshoot any problems that arise.
Understanding Python’s Dictionary Data Type
Before we delve further into adding items to a Python dictionary, it’s crucial to understand what a dictionary in Python is and how it works.
A dictionary in Python is a mutable, or changeable, data type that stores data in key-value pairs. Here’s a simple example of a Python dictionary:
my_dict = {'fruit': 'apple', 'vegetable': 'carrot'}
print(my_dict)
# Output:
# {'fruit': 'apple', 'vegetable': 'carrot'}
In this example, ‘fruit’ and ‘vegetable’ are keys, and ‘apple’ and ‘carrot’ are their corresponding values. The keys in a dictionary are unique and immutable, meaning they cannot be changed. The values, on the other hand, can be of any data type and can be modified.
A Python dictionary is an unordered collection, which means the items are not stored in any particular order. This is unlike other data types like lists or tuples, which are ordered collections.
The dictionary data type in Python is incredibly versatile and powerful. Its ability to store key-value pairs makes it ideal for data structures that require a logical association between a key (identifier) and a value. Understanding how Python dictionaries work is fundamental to effectively adding items to a dictionary.
Expanding Your Python Dictionary Skills
Adding items to a Python dictionary is an essential skill, but it’s just the tip of the iceberg. Dictionaries play a crucial role in larger scripts or projects, often serving as the backbone for data storage and retrieval.
Consider, for example, a web application that needs to store user data. Each user could be represented as a dictionary, with keys representing attributes like ‘username’, ’email’, and ‘password’, and values representing the user’s actual data. As the application grows and more users are added, you’ll find yourself frequently adding items to these dictionaries.
Exploring Related Concepts
Once you’re comfortable with adding items to a dictionary, there are plenty of related concepts to explore. For instance, dictionary comprehension is a powerful tool that allows you to create new dictionaries or add items to existing ones in a single, concise line of code.
Nested dictionaries are another interesting topic. These are dictionaries that contain other dictionaries as values, allowing for complex data structures that can handle a wide range of real-world scenarios.
Dig Deeper into Python Dictionaries
Click Here for a Complete Guide on Python Dictionaries, which includes a step-by-step tutorial on advanced usage examples, syntax, and practical applications.
If you’re interested in learning more about specific Python dictionary topics, here are some available resources.
- Python Dictionary: How to Check If a Key Exists by IOFlood – Learn how to check for the existence of keys in Python dictionaries.
Updating Python Dictionaries: Best Practices and Tips – Learn how to update Python dictionaries effectively.
Python Documentation: Dictionaries – A comprehensive guide to dictionaries straight from Python’s official documentation.
Geek for Geek’s Dictionaries in Python Tutorial – This Guide discusses the Uses, Syntax, and Creation of Dictionaries.
Tutorial on Accessing Dictionary elements – This tutorial by W3schools shows methods to access elements in Python dictionaries.
Online platforms like StackOverflow and GitHub also have a wealth of information, as do numerous Python-focused blogs and tutorials. With a bit of research and practice, you’ll soon be a Python dictionary expert!
Wrapping Up: Python Dictionary Addition
Throughout this guide, we’ve explored the process of adding items to a Python dictionary, starting from the basic usage to more advanced techniques. We’ve learned that adding an item is as simple as assigning a value to a new key, like so:
my_dict = {}
my_dict['new_key'] = 'new_value'
We’ve also discussed how to add multiple items or update an existing item using the update()
method. Moreover, we’ve delved into alternative approaches, such as dictionary comprehension and the setdefault()
method.
Method | Advantages | Disadvantages |
---|---|---|
Basic | Simple, direct | Overwrites existing values |
update() | Adds multiple items, updates existing items | Overwrites existing values |
Dictionary comprehension | Efficient, concise | Can be complex for beginners |
setdefault() | Does not overwrite existing values | Only adds one item at a time |
We also covered common issues like Key Errors and Type Errors and provided solutions to these problems. Understanding these issues will help you troubleshoot any problems that may arise when adding items to a dictionary in Python.
As we’ve seen, Python dictionaries are incredibly versatile and powerful, serving as the backbone for data storage and retrieval in many larger scripts or projects. As you continue to hone your Python skills, remember to keep exploring and practicing. The more you work with dictionaries, the more comfortable you’ll become with them. Happy coding!