Install React with NPM | Quick Node.js How-to Guide
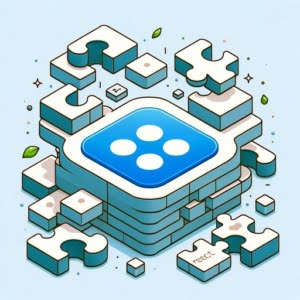
While optimizing our Node.js setup processes for IOFLOOD, we’ve found that installing React comes with numerous benefits and ensures compatibility with other Node.js packages and dependencies. As we believe React can be helpful for building dynamic web applications, especially to our dedicated cloud hosting customers, we’ve centered today’s article around its installation and usage.
This guide will walk you through the process of using npm to install React, making sure you’re well-equipped to take on the vast and exciting world of modern web development. With React, you’re not just learning a library; you’re stepping into an ecosystem that will empower your projects like never before.
Let’s dive into the process of installing React with npm, empowering you to utilize its powerful capabilities in your projects.
TL;DR: How Do I Install React with npm?
To install React, run the
npm install react
and (optional)npm install react-dom
commands in your terminal, while in your project directory.
Here’s an example:
npm install react react-dom
This simple command initiates the download and installation of React and ReactDOM into your project, setting the stage for you to start building with one of the most popular JavaScript libraries today.
Stick around for a deeper dive into the prerequisites, detailed steps, and advanced options available for a seamless React setup.
Table of Contents
Installing React: A Beginner’s Guide
Before diving into the world of React, it’s essential to ensure your development environment is ready. This means having both Node.js and npm (Node Package Manager) installed on your computer. Node.js is the runtime environment that runs JavaScript code outside a web browser, and npm is the package manager that allows you to install JavaScript libraries, such as React.
Checking Node.js and npm Installation
First, let’s verify if Node.js and npm are installed on your system. Open your terminal or command prompt and enter the following commands:
node -v
npm -v
# Output:
# v14.17.0 (or your version of Node.js)
# 6.14.13 (or your version of npm)
These commands check the versions of Node.js and npm installed on your machine. It’s crucial to have them installed before proceeding with React installation, as React and its ecosystem rely on Node.js and npm.
Installing React
Once you’ve confirmed the presence of Node.js and npm, you’re ready to install React. While the TL;DR section provided the basic command, let’s delve a bit deeper into what this command does and why it’s important.
To install React and ReactDOM (the package for working with the DOM in React), run the following command in your terminal:
npm install react react-dom --save
# Output:
# + [email protected]
# + [email protected]
This command not only installs the latest versions of React and ReactDOM but also adds them to your project’s package.json
file under dependencies
. This is crucial for documenting which packages your project depends on, allowing others (or future you) to install these dependencies easily with just an npm install
command.
By following these steps, you’ve taken your first giant leap into React development. Next, we’ll explore more advanced npm commands and how to manage React versions in your project.
Advanced npm Commands for React
When working on more complex projects or when specific versions of libraries are needed, understanding how to manage React versions and dependencies becomes crucial. npm provides a set of advanced commands that allow for precise control over the packages in your project.
Installing a Specific Version of React
Sometimes, a project may require a specific version of React, not necessarily the latest. To install a particular version, you use the npm install
command followed by react@
and the version number. For example, to install React version 16.13.1, you would use the following command:
npm install [email protected] [email protected]
# Output:
# + [email protected]
# + [email protected]
This command specifically fetches and installs version 16.13.1 of React and ReactDOM, ensuring your project aligns with the required dependencies. It’s a critical step for maintaining consistency and avoiding potential conflicts in projects with multiple contributors or specific version dependencies.
Updating React in Your Project
Keeping your React version up-to-date is important for accessing new features and security patches. To update React to the latest version within the range specified in your package.json
, you can use the npm update
command. However, if you want to upgrade to a newer major version outside of your current range, you might need the npm install
command again. For example:
npm install react@latest react-dom@latest
# Output:
# + [email protected] (or newer)
# + [email protected] (or newer)
This command replaces the current versions of React and ReactDOM in your project with the latest versions available, ensuring you have access to the latest improvements and fixes.
Managing Dependencies and devDependencies
Your package.json
file not only tracks the versions of dependencies but also distinguishes between dependencies needed for running your app (dependencies
) and those needed for development and testing (devDependencies
). For example, React is a runtime dependency, while tools like ESLint or Babel may be development dependencies. To save a package as a devDependency, you would use the --save-dev
flag:
npm install eslint --save-dev
# Output:
# + [email protected]
Understanding and utilizing these distinctions in your package.json
can optimize your development workflow and ensure that your production environment is as lean and efficient as possible.
Exploring Beyond npm: Yarn & CRA
While npm install react
is a widely recognized method for adding React to your project, the JavaScript ecosystem offers alternative tools that can enhance your development workflow. Two notable tools are Yarn and Create React App (CRA). Understanding when and why to use these alternatives can streamline your project setup and development process.
Yarn: A npm Alternative
Yarn is a fast, reliable, and secure dependency management tool, introduced by Facebook to address some of npm’s shortcomings, such as performance and security. It works in a similar way to npm but offers a different set of features and commands. To add React to your project using Yarn, you would use:
yarn add react react-dom
# Output:
# success Saved 1 new dependency.
# info Direct dependencies
# └─ [email protected]
# info All dependencies
# └─ [email protected]
This command, like its npm counterpart, adds React and ReactDOM to your project’s dependencies. Yarn’s lock file (yarn.lock
) ensures that the same versions of the dependencies are installed across different environments, enhancing consistency and reliability.
Create React App: Simplifying Setup
Create React App (CRA) is another powerful tool created by Facebook. It offers a zero-config setup for React projects, bundling a modern build setup with no configuration effort. To bootstrap a new React project with CRA, run:
npx create-react-app my-react-app
# Output:
# Success! Created my-react-app at /path/to/my-react-app
# Inside that directory, you can run several commands:
# ...
CRA sets up your development environment so that you can use the latest JavaScript features, providing a solid foundation for your React application. It includes everything from React and ReactDOM to a live development server and a build script, all pre-configured.
When to Use Yarn or CRA
Choosing between npm, Yarn, and CRA depends on your project needs and personal preferences. Yarn is ideal if you’re looking for faster installations and enhanced security features. CRA, on the other hand, is perfect for developers who want to jump straight into coding without worrying about setup configurations. Both tools complement npm and can significantly improve your development experience with React.
Understanding these alternatives to npm install react
equips you with the knowledge to select the best tool for your project’s needs, ensuring a smooth and efficient development process.
Even with a straightforward command like npm install react
, developers can encounter issues that halt their progress. Understanding these common pitfalls and how to address them ensures a smoother React setup process.
Handling Version Conflicts
One common issue is version conflicts, either between React and other libraries or within React’s own dependencies. This can lead to errors that are tricky to debug. To specify a version range that avoids conflicts, you can use the npm install
command with a version range. For example, to install React but avoid version 17 due to a known conflict, you might use:
npm install react@"<17.0.0"
# Output:
# + [email protected]
This command installs the latest version of React that is less than 17.0.0, helping to sidestep known issues with that version. It’s a practical approach to managing dependency versions and ensuring compatibility.
Solving Installation Failures
Installation failures can occur for various reasons, from network issues to corrupted npm cache. If you encounter an error during the installation, a good first step is to clear your npm cache with npm cache clean --force
. Then, retry the installation. If the problem persists, ensure your network connection is stable and that your npm version is up to date by running npm install -g npm@latest
.
npm cache clean --force
npm install react react-dom
# Output:
# + [email protected]
# + [email protected]
Clearing the cache and ensuring you’re using the latest version of npm can resolve many common installation issues. This step is crucial for troubleshooting and can save time spent on seeking external help.
Best Practices for Smooth Setup
To minimize the chances of encountering issues when installing React with npm, follow these best practices:
- Always verify your Node.js and npm versions before starting.
- Use the
--save
flag to ensure dependencies are recorded in yourpackage.json
. - Regularly update npm to the latest version to benefit from improvements and fixes.
- Consider using version control for your project to easily revert to a working state if an update causes issues.
By being proactive and employing these strategies, you can mitigate common issues and focus on building your React application.
npm & Node.js: React’s Backbone
Before we delve into the specifics of using npm install react
, it’s crucial to understand the fundamental tools that make this command possible: npm and Node.js. npm stands for Node Package Manager, a tool that allows developers to share and consume JavaScript packages. Node.js, on the other hand, is a runtime environment that executes JavaScript code outside of a browser.
Why npm for React?
npm acts as a bridge between developers and the vast repository of JavaScript libraries, including React. When you run the command npm install react
, here’s what happens behind the scenes:
npm view react version
# Output:
# 17.0.2
This command queries the npm registry for the latest version of React and displays it. It’s a simple yet powerful demonstration of npm’s ability to connect you with the libraries you need. By installing React via npm, you’re tapping into a streamlined workflow that ensures you’re working with the most up-to-date version of the library.
The Role of Node.js
Node.js plays a pivotal role in this process by providing the environment in which npm operates. Without Node.js, npm wouldn’t be able to execute its commands, including npm install react
. Essentially, Node.js and npm work in tandem to manage packages and dependencies in your project, making them indispensable tools in the modern web developer’s toolkit.
Understanding the synergy between Node.js and npm sheds light on why they are foundational to React development. They not only facilitate the installation of React but also ensure that your development environment is aligned with the needs of a dynamic, component-based UI library like React. This background knowledge is essential for any developer looking to navigate the React ecosystem effectively.
Next Steps After Installing React
Successfully installing React with npm is just the beginning of your journey into building dynamic and responsive web applications. The next steps involve diving deeper into React’s ecosystem, exploring its powerful features, and understanding how you can leverage them in your projects.
Starting a New React Project
After installing React, the immediate next step is to start a new project. This can be done by creating a new directory for your project and initializing it with npm init
, followed by installing React as a dependency. Here’s an example:
mkdir my-react-app
cd my-react-app
npm init -y
npm install react react-dom
# Output:
# + [email protected]
# + [email protected]
This sequence of commands creates a new directory for your project, initializes a new npm package, and installs React and ReactDOM. It sets up the basic structure of a React project, preparing you for the exciting development phase.
Understanding the React Ecosystem
React is more than just a UI library; it’s a comprehensive ecosystem that includes state management tools (like Redux or Context API), routing solutions (like React Router), and a vast array of third-party libraries that enhance its functionality. Familiarizing yourself with these tools and concepts is crucial for effective React development.
Further Resources for React Development
To deepen your understanding of React and stay updated with the latest trends and best practices, here are three invaluable resources:
- React Official Documentation – The best place to start, offering a thorough overview of React’s fundamentals and advanced concepts.
React Training / React Router – A comprehensive guide to mastering routing in React applications, directly from the creators of React Router.
FreeCodeCamp – Offers hands-on React projects and exercises, perfect for applying what you’ve learned in a practical setting.
Leveraging these resources can significantly accelerate your learning curve and enhance your skills in React development. They provide a mix of theoretical knowledge and practical examples, ensuring a well-rounded understanding of how to build effective and engaging web applications with React.
Recap: Mastering npm Install React
In this comprehensive guide, we’ve navigated through the process of setting up React in your project using npm. This journey has equipped you with the knowledge to embark on your React development with confidence.
We began with the basics, ensuring you have Node.js and npm installed, followed by running the simple yet powerful command npm install react react-dom
. This command lays the groundwork for your React projects, integrating the React library seamlessly into your development environment.
Moving on, we explored more advanced npm commands that fine-tune your project setup. We delved into installing specific versions of React, updating React within your project, and managing dependencies effectively. These intermediate steps are crucial for maintaining project consistency and leveraging the full potential of React’s ecosystem.
Additionally, we ventured beyond npm, introducing alternative tools like Yarn and Create React App (CRA) for different project needs. These tools offer additional flexibility and efficiency, proving that the React ecosystem is rich with options to suit various development scenarios.
Tool | Purpose | Key Benefit |
---|---|---|
npm | Package management | Direct control over dependencies |
Yarn | Alternative package manager | Speed and consistency |
CRA | Project setup | Zero-config, out-of-the-box setup |
Whether you’re just starting out with React or looking to streamline your development process, this guide has laid out the foundational steps and considerations for using npm to install React. From basic installations to troubleshooting common issues, you’re now better prepared to navigate the challenges and opportunities of React development.
The importance of npm in React development cannot be overstated. It’s the gateway to a vast repository of libraries and tools that enhance your projects. As you move forward, remember the lessons and tips shared in this guide. They will serve as a valuable resource as you continue to explore and master React development. Happy coding!