Using Deque in Python | Python Queues and Stacks Guide
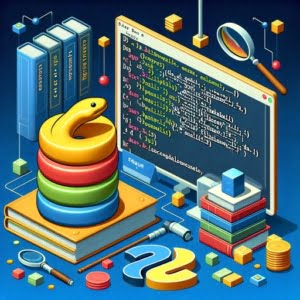
Are you familiar with the concept of queues and stacks in data handling? If so, you’ve likely used lists in Python to manage these structures. Lists are simple, intuitive, and highly flexible, making them the go-to choice for many programmers. However, there are situations where lists may not be the most efficient tool for the job.
Imagine you’re at a bustling theme park. There are two types of lines you can join – one for the roller coaster, where the first person in line gets to ride first (a queue), and another for the Ferris wheel, where the last person who joined the line gets to ride first (a stack). Now, imagine if you could have a line that could function as both a queue and a stack, efficiently handling people coming in and out from both ends. This is where Python’s deque() function comes into play.
The deque() function, part of Python’s collections module, offers significant advantages over lists when it comes to implementing queues and stacks. This article will delve into the power of deque in Python, exploring its efficiency, versatility, and unique traits. Ready to uncover the potential of deque? Let’s get started!
TL;DR: What is deque in Python?
Deque is a Python function for efficient data handling. It’s more versatile than lists, especially for implementing queues and stacks. It allows efficient append and pop operations from both ends with a time complexity of O(1). It’s also thread-safe, making it a safer choice for multithreaded programs. For more advanced methods, background, tips and tricks, read on.
An example of using deque() in Python:
from collections import deque
# Create a deque
queue = deque()
# Enqueue elements
queue.append('A')
queue.append('B')
queue.append('C')
# Dequeue elements
queue.popleft() # 'A'
queue.popleft() # 'B'
queue.popleft() # 'C'
Table of Contents
Basics of Stacks and Queues
As we embark on our journey to explore the deque in Python, it’s essential to first grasp two fundamental concepts in data structures: queues and stacks. These structures are the cornerstone of numerous intricate algorithms and systems in computer science.
What is a Queue?
A queue is a type of data structure where elements are added at one end (termed the ‘rear’) and removed from the other end (the ‘front’). This approach is known as First-in-First-out (FIFO).
Let’s relate it back to our theme park analogy: a queue is like the line for a roller coaster ride. The first person to join the line is the first one to enjoy the ride.
Example of a queue operation using lists:
queue = []
# Enqueue elements
queue.append('A')
queue.append('B')
queue.append('C')
# Dequeue elements
queue.pop(0) # 'A'
queue.pop(0) # 'B'
queue.pop(0) # 'C'
What is a Stack?
Contrarily, a stack is a data structure where elements are both added and removed from the same end. This is the Last-in-First-out (LIFO) approach. Think of it as a stack of pancakes at a breakfast buffet. The last pancake placed on the stack is the first one to be picked up.
Example of a stack operation using lists:
stack = []
# Push elements
stack.append('A')
stack.append('B')
stack.append('C')
# Pop elements
stack.pop() # 'C'
stack.pop() # 'B'
stack.pop() # 'A'
FIFO vs LIFO
The principal difference between a queue (FIFO) and a stack (LIFO) is the removal order of elements. In a queue, the oldest element (the one that has been in the queue the longest) is removed first. In contrast, a stack prioritizes the removal of the newest element (the one most recently added).
To solidify your understanding, let’s consider a couple of real-world examples. A print queue perfectly embodies a queue data structure. The print jobs are processed in the order they were received, adhering to the FIFO approach. Meanwhile, a stack of books is a classic example of a stack data structure. You can only remove the book that was added last, following the LIFO approach.
Concept | Description |
---|---|
FIFO (Queue) | The oldest element (the one that has been in the queue the longest) is removed first. |
LIFO (Stack) | The newest element (the one most recently added) is removed first. |
Grasping these concepts is crucial as they form the foundation of the deque data structure in Python, which we will delve into in the subsequent sections.
Lists are a fundamental part of Python programming. They’re versatile, user-friendly, and support a myriad of operations. However, when it comes to implementing queues and stacks, lists may not be the most efficient tool. Let’s delve into why this is the case and how deque provides a more efficient alternative.
Lists vs Deque
The Limitations of Lists
The first limitation of using lists for queues and stacks arises in a multithreaded environment. Lists are not thread-safe, meaning if two threads try to modify the same list simultaneously, it could lead to unexpected behavior or errors.
Imagine two people trying to rearrange a line of books simultaneously. They might end up bumping into each other, dropping books, or even duplicating efforts.
Furthermore, lists are inefficient when inserting or deleting an element from the left end (the 0th index). This operation requires shifting all other elements by one position, leading to a time complexity of O(n), where n is the length of the list.
This can be quite costly, especially for large lists, much like trying to insert a person at the front of a long queue in our theme park analogy.
The Efficiency of Deque
Now, let’s turn our attention to deque. Unlike lists, deque is designed to allow efficient append and pop operations from both ends, with a time complexity of O(1). This makes it a much more efficient alternative for implementing queues and stacks. It’s like having a special pass in our theme park that allows you to join or leave the line from both ends.
Example of deque operations being more efficient than list operations:
from collections import deque
from time import time
# List
start_time = time()
list_ = []
for i in range(1000000):
list_.insert(0, i)
end_time = time()
print('List time:', end_time - start_time)
# Deque
start_time = time()
deque_ = deque()
for i in range(1000000):
deque_.appendleft(i)
end_time = time()
print('Deque time:', end_time - start_time)
The deque class in Python is implemented as a doubly-linked list. This means that each element holds references to the next and previous elements, allowing for efficient insertion and removal of elements.
In a multithreaded environment, deque operations are atomic, meaning they can be completed without being interrupted by other thread operations. This makes deque a safer choice for multithreaded programs.
It’s like having a dedicated staff member at our theme park ensuring people join and leave the line in an orderly manner, even when it’s crowded.
Using deque() in Python
Having established why deque is a more efficient alternative to lists for implementing queues and stacks, let’s delve into the specifics of the deque() function in Python’s collections module and its operations.
Introduction to the deque() Function
The deque() function is housed within Python’s collections module. It allows you to create a new deque object that can be populated with elements from an iterable, such as a list or a tuple. Let’s look at how you can create a new deque object:
from collections import deque
d = deque()
In the above code, d
becomes an empty deque object. If you wish to create a deque with some initial elements, you can pass an iterable to the deque() function in the following way:
from collections import deque
d = deque([1, 2, 3, 4, 5])
Now, d
is a deque object containing the elements 1, 2, 3, 4, and 5.
Features of deque()
One of the standout features of deque() that gives it an edge over lists for implementing queues and stacks is its capability to efficiently handle operations at both ends. Operations such as append(), appendleft(), pop(), and popleft() have a time complexity of O(1), making them extremely efficient.
But that’s not all. The deque class supports a range of other operations as well. Let’s take a closer look at some of them.
Deque Operations
Operation | Description |
---|---|
extend(iterable) | Appends elements from an iterable to the right end of the deque. |
extendleft(iterable) | Appends elements from an iterable to the left end of the deque. The elements are added in reverse order. |
reverse() | Reverses the elements of the deque in-place. |
rotate(n) | Rotates the deque n steps to the right. If n is negative, it rotates to the left. |
Consider a scenario where you’re managing a line of people at a theme park. The extend() function allows you to add a group of people to the end of the line, while extendleft() lets you insert a group at the front.
The reverse() function would flip the order of the line, and the rotate() function would shift people to the right or left in the line.
By supporting these operations, deque offers a high level of flexibility and control over your data structures, making it a robust tool for efficient data handling in Python.
Implementing a Queue with deque()
Implementing a queue using deque() is as straightforward as getting on a roller coaster ride at our theme park. Here, we’ll create a simple queue that supports enqueue and dequeue operations.
from collections import deque
# Create a deque
queue = deque()
# Enqueue elements
queue.append('A')
queue.append('B')
queue.append('C')
# Dequeue elements
queue.popleft() # 'A'
queue.popleft() # 'B'
queue.popleft() # 'C'
In the above code, we first create an empty deque named queue. We then enqueue elements ‘A’, ‘B’, and ‘C’ using the append() method. Finally, we dequeue elements using the popleft() method, which removes and returns an element from the left end of the deque. It’s like letting people into the ride one by one in the order they arrived.
Handling Empty Queue Errors
But what happens if we try to dequeue an element from an empty queue? Let’s find out:
queue.popleft() # Raises an 'IndexError: pop from an empty deque' error
As you can see, trying to dequeue an element from an empty queue raises an error. To handle this, we can use a try-except block:
try:
queue.popleft()
except IndexError:
print('Cannot dequeue from an empty queue.')
Now, instead of raising an error, the program prints a message when trying to dequeue from an empty queue. It’s like having a staff member at the ride entrance to inform people when the ride is closed.
Thread-Safe Atomic Operations
In a multithreaded environment, deque is a better choice than list due to its support for thread-safe atomic operations. This means that operations like append() and popleft() can be completed in a single step without being interrupted by other thread operations. To demonstrate this, let’s imagine a scenario at our theme park where multiple staff members are managing the ride queue. Even if they’re letting people in and out simultaneously, the order of operations is maintained thanks to deque’s thread-safe operations.
Respecting User-Defined Order in a Multithreading Environment
One of the key advantages of using deque in a multithreading environment is that it respects the order of data defined by the user. This means that even when multiple threads are enqueuing and dequeuing elements simultaneously, the deque maintains the order in which the operations were initiated. This is crucial in scenarios where the order of operations matters, such as processing tasks from a task queue or managing a ride queue at a theme park.
In the next section, we’ll explore the versatility and unique traits of deque, which further enhance its utility in data handling.
The power of the deque class in Python is truly showcased by its versatility. Unlike other data structures, deque can be used as a queue (FIFO), a stack (LIFO), or a deque (double-ended queue). This flexibility is due to the wide range of methods supported by deque for manipulating data.
Deque as a Queue, Stack, or Deque
As we’ve seen earlier, you can use the append() and popleft() methods to use a deque as a queue. It’s like managing the line for a roller coaster ride at our theme park, where the first person in line is the first to get on the ride. For a stack, you can use append() and pop() methods, similar to stacking and unstacking plates in a cafeteria. And as a deque (double-ended queue), you can use append(), appendleft(), pop(), and popleft() methods to add or remove elements from either end, offering a level of flexibility akin to a double-ended roller coaster that can be boarded from both ends.
Example of using deque as a queue, stack, and deque:
from collections import deque
# Deque as a queue
queue = deque()
queue.append('A') # enqueue
queue.append('B') # enqueue
queue.popleft() # dequeue
# Deque as a stack
stack = deque()
stack.append('A') # push
stack.append('B') # push
stack.pop() # pop
# Deque as a deque
deque_ = deque()
deque_.append('A') # append right
deque_.appendleft('B') # append left
deque_.pop() # pop right
deque_.popleft() # pop left
Restricting Deque’s Length
Another unique trait of deque is its ability to restrict its maximum length. This means that you can limit the number of items a deque can hold.
When the limit is reached, adding a new item to one end automatically discards an item from the opposite end. This feature is useful for creating a ‘recent items’ list that automatically removes the oldest item when a new one is added, much like a ride operator at our theme park keeping track of the last ten rides a visitor has taken.
Example of restricting deque’s length:
from collections import deque
# Create a deque with a maximum length of 3
deque_ = deque(maxlen=3)
# Add elements
deque_.append('A')
deque_.append('B')
deque_.append('C')
deque_.append('D') # 'A' is automatically discarded
print(deque_) # deque(['B', 'C', 'D'], maxlen=3)
Practical Applications
The versatility and unique traits of deque have many practical applications in data handling. For instance, you can use a deque as a sliding window of the last n items in a data stream. This is useful in scenarios like tracking the last n transactions in a banking system, the last n locations visited in a GPS tracking system, or the last n pages visited in a web browsing session.
Real-World Scenario: Maintaining a History of the Last 10 Items Accessed in a Web Application
Let’s consider a real-world scenario where deque’s unique traits can be handy. Suppose you’re developing a web application and you want to maintain a history of the last 10 items accessed by the user. You can use a deque with a maximum length of 10 to achieve this. Here’s how you can do it:
from collections import deque
# Create a deque with a maximum length of 10
history = deque(maxlen=10)
# Add items to the deque
for i in range(15):
history.append(i)
print(history) # deque([5, 6, 7, 8, 9, 10, 11, 12, 13, 14], maxlen=10)
In the above code, we first create a deque named history with a maximum length of 10. We then add 15 items to the deque. Since the deque’s maximum length is 10, it automatically discards the oldest items when the limit is reached. As a result, the deque only contains the last 10 items added, much like a theme park visitor being able to recall their last ten rides.
This is just one example of how deque’s versatility and unique traits can be leveraged in real-world applications. With its efficient operations and flexible nature, deque is truly a powerful tool for data handling in Python, just like a versatile theme park ride that can offer different experiences based on the visitor’s preference.
Further Readings on Python Data Types
To amplify your comprehension on Python Data Types, dig into the following resources that are highly recommended:
- Python Data Types Mastery Simplified – Learn about Python’s mapping data type, the dictionary, for key-value pair storage.
Understanding Sets in Python – Learn how to create and manipulate sets in Python for efficient data filtering and comparison.
Python Dataclass: Fundamentals and Usage Guide – Dive into Python dataclasses for simplifying the creation of structured data types.
Python Stack Guide – Comprehensive guide to Python stack implementation by MyGreatLearning.
Queue in Python Article – Detailed tutorial on Python queues by GeeksforGeeks.
Python’s Official Queue Library Documentation – In-depth look at Python’s queue library from official resources.
By advancing your understanding of Python data types and engaging with related subjects, you can boost your efficiency and effectiveness as a Python developer.
Conclusion
In this comprehensive guide, we’ve taken a thrilling roller coaster ride into the world of deque in Python. We’ve explored the limitations of lists, especially when used to implement queues and stacks, and discovered how deque offers a more efficient and versatile alternative, much like choosing the right ride at a theme park for the ultimate experience.
Perhaps the most compelling feature of deque is its versatility. It can be used as a queue, a stack, or a double-ended queue, providing us with the flexibility to choose the right data structure for our specific needs. Its unique ability to restrict its maximum length allows us to create ‘recent items’ lists that automatically discard the oldest items when new ones are added.
Empower your Python journey with our comprehensive coding blueprint.
In conclusion, deque is a powerful tool in Python’s collections module that offers significant advantages over lists for implementing queues and stacks. So, next time you’re faced with a data handling task in Python, remember to consider deque – it might just be the perfect ride for your data!