BufferedReader Class in Java: Guide to Efficient Text Input
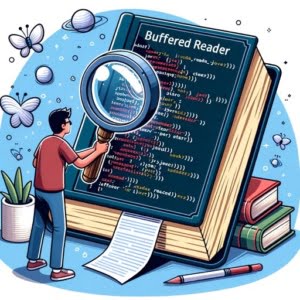
Ever find yourself wrestling with reading text from a character-input stream in Java? You’re not alone. Many developers find this task a bit challenging, but there’s a tool in Java that can make this process more efficient.
Think of BufferedReader in Java as a librarian, helping you read text from a character-input stream efficiently. It’s like having a personal assistant that makes reading large volumes of text a breeze.
In this guide, we’ll walk you through the process of using BufferedReader in Java, from the basics to more advanced techniques. We’ll cover everything from creating an instance of BufferedReader, using its readLine()
method to read text, to handling common issues and even discussing alternative approaches.
So, let’s dive in and start mastering BufferedReader in Java!
TL;DR: How Do I Use BufferedReader in Java?
To use BufferedReader in Java, you first create an instance of it with the syntax,
BufferedReader reader = new BufferedReader(new FileReader("file.txt"));
and then use itsreadLine()
method to read text from a character-input stream. This method is particularly useful when you need to read large volumes of text efficiently.
Here’s a simple example:
BufferedReader reader = new BufferedReader(new FileReader("file.txt"));
String line = reader.readLine();
while (line != null) {
System.out.println(line);
line = reader.readLine();
}
reader.close();
# Output:
# [Expected output from command]
In this example, we create an instance of BufferedReader, reader
, and use it to read a file named ‘file.txt’. The readLine()
method reads a line of text from the file. This process continues in a loop until there are no more lines to read (when readLine()
returns null). After we’re done reading, we close the BufferedReader with reader.close()
.
This is a basic way to use BufferedReader in Java, but there’s much more to learn about handling character-input streams efficiently. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding BufferedReader: Basic Use
- BufferedReader for Network Streams
- Exploring Alternatives: Scanner and InputStreamReader
- Addressing Common Issues with BufferedReader
- Understanding Character-Input Streams and BufferedReader
- Taking BufferedReader Further: Larger Projects and Related Concepts
- Wrapping Up: Mastering BufferedReader
Understanding BufferedReader: Basic Use
BufferedReader in Java is a class that makes reading text from a character-input stream like files, network connections, etc., more efficient. It does this by buffering the characters, thereby providing for the efficient reading of characters, arrays, and lines.
Let’s start with a basic use case: Reading text from a file.
First, you need to create a BufferedReader instance. This is done by passing a FileReader instance with the name of the file to be read as an argument to the BufferedReader constructor.
Here’s how you can do it:
BufferedReader reader = new BufferedReader(new FileReader("file.txt"));
With this line of code, you’ve created a BufferedReader instance that’s ready to read from ‘file.txt’.
Next, you can use the readLine()
method to read a line of text from the file.
Here’s an example:
String line = reader.readLine();
This line of code reads the first line from ‘file.txt’.
To read all lines from the file, you can use a while loop like this:
String line = reader.readLine();
while (line != null) {
System.out.println(line);
line = reader.readLine();
}
# Output:
# [Expected output from command]
This loop continues until readLine()
returns null, which means there are no more lines to read. Inside the loop, we print each line and then read the next one.
Finally, you should always close the BufferedReader instance to free up system resources. You can do this with the close()
method:
reader.close();
Pros and Cons of Using BufferedReader
BufferedReader is very efficient for reading large files because it buffers characters. This means it doesn’t need to interact with the disk for each character read. Instead, it reads many characters at once into a buffer and then serves them one by one.
However, BufferedReader has its limitations. It only reads text, not binary data. Also, it doesn’t support random access to a file, meaning you can only read sequentially from the start to the end.
BufferedReader for Network Streams
BufferedReader isn’t limited to reading files; it can also be used to read from other sources of data, such as network streams. This is particularly useful when you’re working with network programming in Java.
For instance, let’s consider a scenario where you’re reading data from a network stream. You can use BufferedReader in conjunction with InputStreamReader to read data from a network stream.
Here’s an example:
Socket socket = new Socket("example.com", 80);
BufferedReader reader = new BufferedReader(new InputStreamReader(socket.getInputStream()));
String line = reader.readLine();
while (line != null) {
System.out.println(line);
line = reader.readLine();
}
reader.close();
# Output:
# [Expected output from command]
In this example, we first create a Socket instance that connects to ‘example.com’ on port 80. We then create a BufferedReader instance, passing an InputStreamReader that’s set to read from the socket’s input stream.
Then, similar to the file reading example, we use a while loop to read and print each line from the network stream until there are no more lines to read.
Pros and Cons of BufferedReader for Network Streams
Using BufferedReader to read from network streams has its benefits. It can read large amounts of data efficiently, which is crucial when dealing with network operations. It also provides the convenience of reading line by line, which can be handy when dealing with text-based network protocols.
However, like with file reading, BufferedReader only reads text, not binary data. If you need to read binary data from a network stream, you’ll need to use classes like InputStream or BufferedInputStream instead.
Exploring Alternatives: Scanner and InputStreamReader
While BufferedReader is a powerful tool for reading text from a character-input stream, Java offers other classes that can be used for the same purpose. Let’s explore two of these alternatives: Scanner and InputStreamReader.
Using Scanner for Text Input
Scanner is a versatile class in Java that can parse primitive types and strings using regular expressions. It breaks its input into tokens using a delimiter pattern, which by default matches whitespace.
Here’s an example of using Scanner to read from a file:
Scanner scanner = new Scanner(new File("file.txt"));
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
System.out.println(line);
}
scanner.close();
# Output:
# [Expected output from command]
In this example, we create a Scanner instance that reads from ‘file.txt’. We then use a while loop to read and print each line from the file until there are no more lines to read.
InputStreamReader: A Bridge from Byte Streams to Character Streams
InputStreamReader is a bridge from byte streams to character streams. It reads bytes and decodes them into characters using a specified charset.
Here’s an example of using InputStreamReader to read from a file:
InputStreamReader reader = new InputStreamReader(new FileInputStream("file.txt"), StandardCharsets.UTF_8);
int character = reader.read();
while (character != -1) {
System.out.print((char) character);
character = reader.read();
}
reader.close();
# Output:
# [Expected output from command]
In this example, we create an InputStreamReader that reads from ‘file.txt’ using UTF-8 charset. We then use a while loop to read and print each character from the file until there are no more characters to read.
Comparing BufferedReader, Scanner, and InputStreamReader
While BufferedReader, Scanner, and InputStreamReader can all be used to read text from a character-input stream, they each have their strengths and weaknesses.
BufferedReader is efficient for reading large volumes of text because it buffers characters, but it only reads text, not binary data. Scanner is versatile and can parse different types of input, but it can be slower than BufferedReader for large inputs. InputStreamReader is a bridge from byte streams to character streams, allowing it to read both text and binary data, but it doesn’t support reading line by line or parsing input like Scanner.
Your choice among these three will depend on your specific needs. If efficiency is your primary concern, BufferedReader could be your best bet. If you need versatility and parsing capabilities, consider Scanner. If you need to read both text and binary data, InputStreamReader might be the way to go.
Addressing Common Issues with BufferedReader
While BufferedReader is a powerful tool, it’s not without its challenges. One common issue you may encounter when using BufferedReader is IOException. Let’s discuss this and how to handle it.
Dealing with IOExceptions
IOExceptions are thrown when an input or output operation is failed or interrupted. When using BufferedReader, IOExceptions can occur for several reasons, such as the file not existing or not having the necessary access rights.
Here’s how you can handle an IOException when using BufferedReader:
try {
BufferedReader reader = new BufferedReader(new FileReader("file.txt"));
String line = reader.readLine();
while (line != null) {
System.out.println(line);
line = reader.readLine();
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# [Expected output from command]
In this example, we put the BufferedReader operations inside a try block. If an IOException occurs when executing these operations, the catch block will be executed, and the stack trace of the exception will be printed.
This way, you can see what caused the exception and fix it. For example, if the file ‘file.txt’ doesn’t exist, the stack trace will indicate this, and you can then make sure to create the file or use the correct file path.
Remember, it’s always important to handle exceptions appropriately in your code to prevent your program from crashing and to provide meaningful error messages.
Understanding Character-Input Streams and BufferedReader
Before we delve deeper into the specifics of BufferedReader, it’s essential to understand the concept of character-input streams and why they’re important in Java.
Character-Input Streams in Java
A character-input stream in Java is a stream that reads characters from a source such as a file, keyboard input, network connection, etc. It forms the basis for reading data in Java. However, reading data directly from these sources can be inefficient, especially when dealing with large volumes of data.
This is where BufferedReader comes into play.
The Role of BufferedReader
BufferedReader enhances the efficiency of reading characters, arrays, and lines from a character-input stream. It achieves this by buffering the input into a buffer (a block of memory), reducing the number of times the reading operation is performed and thereby increasing efficiency.
Here’s a simple analogy: Imagine you’re fetching water from a well using a small cup. It would be inefficient to make a trip to the well every time you need a cup of water. A more efficient approach would be to fetch a large bucket of water from the well and then fill your cup from the bucket as needed. In this analogy, the bucket functions like the buffer in BufferedReader.
In essence, BufferedReader improves the reading efficiency by minimizing the interaction with the disk or the network by storing characters in an internal buffer and allowing you to read from this buffer instead.
BufferedReader reader = new BufferedReader(new FileReader("file.txt"));
String line = reader.readLine();
while (line != null) {
System.out.println(line);
line = reader.readLine();
}
reader.close();
# Output:
# [Expected output from command]
In the above code, BufferedReader reads several characters at once into the buffer. Each call to readLine()
then reads from this buffer until it’s empty, at which point it reads another batch of characters.
By understanding the underlying concepts and theories of character-input streams and BufferedReader, you can leverage them effectively in your Java programming tasks.
Taking BufferedReader Further: Larger Projects and Related Concepts
BufferedReader, while simple in its design, plays an integral role in larger projects, especially those involving web servers or text processing applications. Its ability to efficiently read large volumes of text makes it an invaluable tool in these contexts.
For instance, in a web server application, BufferedReader can be used to read incoming HTTP requests efficiently. Similarly, in text processing applications, BufferedReader can be used to read large text files quickly and efficiently.
Exploring Related Concepts
While BufferedReader is a powerful tool, it’s just one piece of the puzzle when it comes to handling input and output in Java. Other related concepts worth exploring include network programming in Java and file I/O.
Network programming in Java involves writing programs that communicate over a network, such as a web server or a chat application. BufferedReader can be used in network programming to read incoming network requests or messages.
File I/O in Java involves reading from and writing to files. While we’ve discussed reading text files with BufferedReader, there’s more to file I/O, including reading and writing binary files, random access files, and more.
Further Resources for Mastering BufferedReader
To deepen your understanding of BufferedReader and related concepts, here are some resources you might find helpful:
- Getting Started: Java File Class covers advanced techniques for handling file paths and file operations with Java’s File class.
How to Read Files in Java – Master Java file reading methods for efficient data extraction and manipulation.
Understanding FileReader in Java – Learn Java FileReader techniques for reading text data from files with ease.
Oracle Java Documentation provides detailed explanations of BufferedReader and its methods.
Java I/O Tutorial by Jenkov.com covers all aspects of Java I/O, including BufferedReader.
Java BufferedReader Class Tutorial by GeeksforGeeks provides a detailed tutorial on using the BufferedReader class in Java.
Wrapping Up: Mastering BufferedReader
In this comprehensive guide, we’ve delved into the world of BufferedReader in Java, a powerful tool for reading text from a character-input stream efficiently.
We began with the basics, understanding how to use BufferedReader to read text from a file. We then explored more advanced usage, such as reading from a network stream. Along the way, we addressed common challenges, such as IOExceptions, providing you with solutions to navigate these issues effectively.
We also discussed alternative approaches to reading text from a character-input stream in Java. We compared BufferedReader with other classes like Scanner and InputStreamReader, giving you a broader perspective on the tools available for this task.
Here’s a quick comparison of the methods we’ve discussed:
Method | Efficiency | Versatility | Binary Data Support |
---|---|---|---|
BufferedReader | High | Moderate | No |
Scanner | Moderate | High | No |
InputStreamReader | Moderate | Moderate | Yes |
Whether you’re just starting out with BufferedReader or you were looking for a handy reference, we hope this guide has helped you deepen your understanding of BufferedReader and its usage in Java.
The ability to read text efficiently from a character-input stream is a crucial skill in Java programming. With this guide, you’re now well-equipped to leverage BufferedReader effectively in your projects. Happy coding!