numpy.transpose() Function Guide (With Examples)
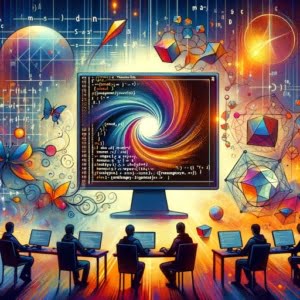
Struggling to transpose arrays in Python? You’re not alone. Many programmers find themselves in a bind when trying to flip a matrix on its diagonal.
With the numpy transpose function, you can rearrange your data in a snap. This function is a powerful tool in the numpy library that can simplify your coding life.
In this comprehensive guide, we will walk you through the process of using numpy’s transpose function. We’ll start with the basics and gradually move on to more advanced techniques. Whether you’re a beginner or an intermediate programmer, this guide has something for you.
So, let’s dive in and start transposing those arrays!
TL;DR: How Do I Transpose a Numpy Array in Python?
You can use the
numpy.transpose()
function to transpose a numpy array. Here’s a simple example:
import numpy as np
a = np.array([[1, 2], [3, 4]])
b = np.transpose(a)
print(b)
# Output:
# array([[1, 3],
# [2, 4]])
In this example, we first import the numpy library. We then create a 2×2 numpy array ‘a’. The numpy.transpose()
function is then used to transpose the array ‘a’, and the result is stored in ‘b’. When we print ‘b’, we see that the rows and columns of ‘a’ have been swapped.
Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the Basics of Numpy Transpose
- Transposing Multi-Dimensional Arrays with Numpy
- Exploring Alternative Methods for Array Transposition
- Troubleshooting Common Issues with Numpy Transpose
- Understanding Numpy and Array Data Structures
- The Relevance of Array Transposition in Data Analysis and Machine Learning
- Wrapping Up: Numpy Transpose and Array Manipulation
Understanding the Basics of Numpy Transpose
The numpy.transpose()
function is a versatile tool in Python that allows you to rearrange the axes of an array. At its simplest, it flips the axes of your array, effectively swapping rows with columns. This operation is particularly useful when working with matrices in data analysis or machine learning.
Consider the following example:
import numpy as np
# Create a 2D array
a = np.array([[1, 2, 3], [4, 5, 6]])
print('Original array:')
print(a)
# Transpose the array
b = np.transpose(a)
print('
Transposed array:')
print(b)
# Output:
# Original array:
# [[1 2 3]
# [4 5 6]]
#
# Transposed array:
# [[1 4]
# [2 5]
# [3 6]]
In this example, we create a 2D array ‘a’ with two rows and three columns. When we apply the numpy.transpose()
function, it returns a new array where the original rows become columns and the original columns become rows. This is the basic operation of the numpy.transpose()
function.
One of the key advantages of the numpy.transpose()
function is that it does not physically rearrange the data in memory. It simply changes the way the array is indexed, making it a highly efficient operation.
However, one potential pitfall to be aware of is that transposing will not necessarily give you the result you expect if your original array is not a 2D array. This is because numpy.transpose()
operates on the axes of your array, and the concept of ‘rows’ and ‘columns’ does not necessarily apply in the same way for arrays of higher dimensions.
Transposing Multi-Dimensional Arrays with Numpy
While the numpy.transpose()
function is straightforward to use with 2D arrays, its real power becomes apparent when you work with multi-dimensional arrays. For such arrays, the transpose operation involves a permutation of the axes, and understanding this can offer greater flexibility and control over your data.
Consider a 3D array, for instance. When transposing a 3D array, the numpy.transpose()
function will by default reverse the order of the axes. This means that if your original array has shape (i, j, k), the transposed array will have shape (k, j, i).
Let’s illustrate this with an example:
import numpy as np
# Create a 3D array
a = np.array([[[1, 2], [3, 4]], [[5, 6], [7, 8]]])
print('Original array:')
print(a)
# Transpose the array
b = np.transpose(a)
print('
Transposed array:')
print(b)
# Output:
# Original array:
# [[[1 2]
# [3 4]]
#
# [[5 6]
# [7 8]]]
#
# Transposed array:
# [[[1 5]
# [3 7]]
#
# [[2 6]
# [4 8]]]
In this example, we create a 3D array ‘a’ with shape (2, 2, 2). When we apply the numpy.transpose()
function, it returns a new array where the original axes have been reversed. This is why the first and second matrices of ‘a’ now appear as the first and second columns of ‘b’.
In more advanced scenarios, you can specify a tuple for the axes
parameter to determine the order of the axes after the transpose.
Exploring Alternative Methods for Array Transposition
While numpy.transpose()
is a powerful tool for transposing arrays, it’s not the only method available in numpy. Two notable alternatives are the numpy.ndarray.T
property and the numpy.swapaxes()
function.
The numpy.ndarray.T
Property
The numpy.ndarray.T
property is a simple and fast way to transpose a numpy array. It works just like numpy.transpose()
, but can be used in a more concise and readable way. Here’s an example:
import numpy as np
# Create a 2D array
a = np.array([[1, 2, 3], [4, 5, 6]])
print('Original array:')
print(a)
# Transpose the array using the T property
b = a.T
print('
Transposed array:')
print(b)
# Output:
# Original array:
# [[1 2 3]
# [4 5 6]]
#
# Transposed array:
# [[1 4]
# [2 5]
# [3 6]]
In this example, the numpy.ndarray.T
property is used to transpose the array ‘a’. The result is identical to using numpy.transpose(a)
.
The numpy.swapaxes()
Function
For more control over the transposition process, you can use the numpy.swapaxes()
function. This function allows you to specify which two axes you want to swap. Here’s an example:
import numpy as np
# Create a 3D array
a = np.array([[[1, 2], [3, 4]], [[5, 6], [7, 8]]])
print('Original array:')
print(a)
# Transpose the array using the swapaxes function
b = np.swapaxes(a, 0, 1)
print('
Transposed array:')
print(b)
# Output:
# Original array:
# [[[1 2]
# [3 4]]
#
# [[5 6]
# [7 8]]]
#
# Transposed array:
# [[[1 2]
# [5 6]]
#
# [[3 4]
# [7 8]]]
In this example, the numpy.swapaxes()
function is used to swap the first and second axes of the array ‘a’. The result is a transposed array where the first and second matrices of ‘a’ are now the first and second rows of ‘b’.
Each method has its own advantages and disadvantages. The numpy.transpose()
function and the numpy.ndarray.T
property are simple and straightforward, but they offer less control over the transposition process. The numpy.swapaxes()
function is more flexible, but it can be a bit more complex to use. Choose the method that best suits your needs.
Troubleshooting Common Issues with Numpy Transpose
While numpy’s transpose function is robust and versatile, you might encounter some issues during its use. One common issue is dimension mismatch errors. These errors occur when you try to perform operations between arrays that have incompatible shapes.
Dimension Mismatch Errors
Dimension mismatch errors are common when you try to perform operations between a transposed array and another array. For instance, consider the following example:
import numpy as np
# Create two 2D arrays
a = np.array([[1, 2, 3], [4, 5, 6]])
b = np.array([[7, 8], [9, 10], [11, 12]])
# Transpose 'a'
a_transposed = np.transpose(a)
# Try to add 'a_transposed' and 'b'
c = a_transposed + b
# Output:
# ValueError: operands could not be broadcast together with shapes (3,2) (2,3)
In this example, we try to add a transposed array ‘a_transposed’ with shape (3, 2) and another array ‘b’ with shape (2, 3). This results in a ValueError because the shapes of the arrays are incompatible for addition.
Solutions and Workarounds
To fix this issue, you need to ensure that the shapes of your arrays are compatible for the operation you’re trying to perform. In the case of addition, this means that the arrays need to have the same shape. You could, for instance, transpose ‘b’ as well before performing the addition:
# Transpose 'b'
b_transposed = np.transpose(b)
# Now, adding 'a_transposed' and 'b_transposed' works fine
c = a_transposed + b_transposed
print(c)
# Output:
# [[ 8 12]
# [11 15]
# [14 18]]
In this revised example, we transpose ‘b’ to get ‘b_transposed’ with shape (3, 2). Now, ‘a_transposed’ and ‘b_transposed’ have the same shape, and adding them together works without any errors.
Remember, numpy’s transpose function is a powerful tool, but it needs to be used with care. Always make sure your array shapes are compatible when performing operations between them.
Understanding Numpy and Array Data Structures
To fully grasp the power of numpy’s transpose function, it’s crucial to understand the numpy library and its array data structure. Numpy, short for ‘Numerical Python’, is a library that provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays.
The Numpy Array
At the heart of numpy is the array object. Arrays in numpy are grids of values, all of the same type, and are indexed by a tuple of non-negative integers. The number of dimensions is the rank of the array, and the shape of an array is a tuple of integers giving the size of the array along each dimension.
Consider the following example:
import numpy as np
# Create a 2D array
a = np.array([[1, 2, 3], [4, 5, 6]])
print('Array:')
print(a)
print('
Rank:', a.ndim)
print('Shape:', a.shape)
# Output:
# Array:
# [[1 2 3]
# [4 5 6]]
#
# Rank: 2
# Shape: (2, 3)
In this example, ‘a’ is a 2D array (or matrix) with two rows and three columns. The rank of ‘a’ is 2 (since it has two dimensions), and its shape is (2, 3).
Array Dimensions and the Transpose Operation
The concept of array dimensions is crucial when it comes to the transpose operation. As we’ve seen, the numpy.transpose()
function rearranges the axes of an array. For a 2D array, this means swapping rows and columns. For a multi-dimensional array, it involves a more complex permutation of the axes.
Understanding the dimensions of your array and how the numpy.transpose()
function operates on them is key to using this function effectively. As we explore more advanced uses of numpy’s transpose function, keep these fundamentals in mind.
The Relevance of Array Transposition in Data Analysis and Machine Learning
Array transposition, as facilitated by numpy.transpose()
, is not just a mathematical curiosity. It has practical applications in several areas, particularly in data analysis and machine learning. For instance, in machine learning, you often need to transpose matrices when performing operations between features and weights.
Consider a simple linear regression model that predicts a target variable based on multiple features. The weights of the model are represented as a vector, and the features of a data point are represented as another vector. To calculate the predicted value for the target variable, you need to take the dot product of the two vectors. This operation requires that the vectors align correctly, which is where transposition comes in.
import numpy as np
# Features and weights
features = np.array([[1, 2, 3]]) # 1x3 matrix
weights = np.array([[2], [3], [4]]) # 3x1 matrix
# Calculate predicted value
predicted_value = np.dot(features, weights)
print(predicted_value)
# Output:
# [[20]]
In this example, we have a 1×3 matrix for the features and a 3×1 matrix for the weights. The numpy.dot()
function calculates the dot product of the two matrices, which gives us the predicted value for the target variable.
Exploring Related Concepts
Transposing arrays is just one of many operations you can perform with numpy. If you’re interested in diving deeper, consider exploring the following resources:
- Numpy for Data Science: An In-Depth Overview – Learn about NumPy’s role in data analysis, machine learning, and scientific research.
numpy.reshape(): Transforming Data Structures in NumPy – Dive into the world of reshaping NumPy arrays and achieve data restructuring flexibility
Data Stacking with NumPy’s numpy.vstack() – Master the art of using “numpy vstack” for merging arrays along the vertical axis.
Numpy Arrays Tutorial on LearnPython.org – An interactive tutorial from LearnPython.org exploring the functionalities of Numpy arrays.
Numpy Questions on StackOverflow – A collection of StackOverflow questions and answers focused on Numpy, proving a useful resource for troubleshooting and learning.
Numpy Book PDF from MIT – A downloadable PDF from MIT offering comprehensive knowledge about Numpy.
As always, the best way to learn is by doing, so don’t hesitate to experiment with these functions and see what you can create.
Wrapping Up: Numpy Transpose and Array Manipulation
In this guide, we’ve delved into the numpy.transpose()
function, a powerful tool for rearranging the axes of numpy arrays. We’ve explored its basic use, where it swaps the rows and columns of 2D arrays, and its advanced use, where it operates on multi-dimensional arrays in a more complex manner.
We’ve also discussed common issues, such as dimension mismatch errors, and provided solutions and workarounds. Remember, compatibility of array shapes is key when performing operations between them.
In addition, we’ve examined alternative methods for transposing arrays, namely the numpy.ndarray.T
property and the numpy.swapaxes()
function. Each method has its own strengths and weaknesses, and the best one to use depends on your specific needs.
Method | Simplicity | Flexibility |
---|---|---|
numpy.transpose() | High | Medium |
numpy.ndarray.T | High | Low |
numpy.swapaxes() | Medium | High |
Finally, we’ve touched on the relevance of array transposition in fields like data analysis and machine learning, and encouraged you to explore related concepts such as array reshaping and array manipulation.
As with any tool, the key to mastering numpy’s transpose function is practice. Don’t hesitate to experiment with it and see what you can achieve. Happy coding!