Java 11 Features: Summary of New Development Tools
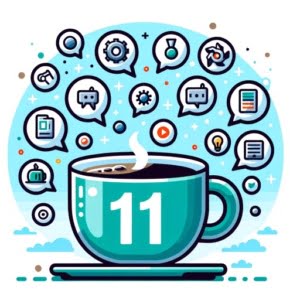
Are you eager to discover the new features in Java 11? You’re not alone. Many developers are excited to explore the new territories that Java 11 has to offer.
Think of Java 11 as a treasure chest – packed with a plethora of new features that can enhance your programming capabilities. From the HttpClient API to the Epsilon garbage collector, there’s a lot to uncover.
This guide will provide an in-depth look at each feature introduced in Java 11, from basic usage to advanced techniques. We’ll cover everything from the basics of the HttpClient API, the workings of the Epsilon garbage collector, to the ability to launch single-file source-code programs.
So, let’s embark on this exciting journey and start exploring Java 11!
TL;DR: What are the new features in Java 11?
Java 11 introduced several new features such as the new HttpClient API, the Epsilon garbage collector, and the ability to launch single-file source-code programs. For instance, you can now use the HttpClient API like this:
HttpClient.newHttpClient().send(request, BodyHandlers.ofString())
.
Here’s a simple example:
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("http://example.com/"))
.build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
// Output:
// The HTML content of the http://example.com page
In this example, we’ve used the new HttpClient API to send a GET request to http://example.com
and print the response body. This is just a basic usage of HttpClient API, one of the new features in Java 11.
But Java 11’s new features go far beyond this. Continue reading for more detailed examples and advanced usage scenarios.
Table of Contents
- Exploring HttpClient API: The Basic Use
- Epsilon Garbage Collector: An Advanced Insight
- Launching Single-File Source-Code Programs: An Alternative Approach
- Navigating Java 11: Troubleshooting and Considerations
- Journey Through Java: A Brief History
- Applying Java 11 Features: Real-World Scenarios and Further Reading
- Wrapping Up: Java 11 Features
Exploring HttpClient API: The Basic Use
Java 11 introduced a brand new HttpClient API that replaces the older, less efficient HttpUrlConnection. The HttpClient API is a significant improvement, offering a more modern and intuitive approach to handling HTTP requests.
Let’s examine a simple example of using the HttpClient API:
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("http://example.com/"))
.build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
// Output:
// The HTML content of the http://example.com page
In this example, we’re creating an instance of HttpClient
using the newHttpClient()
method. We’re then building an HttpRequest
to http://example.com/
and sending it using the send()
method. The response is handled by HttpResponse.BodyHandlers.ofString()
, which returns the body of the response as a string.
HttpClient API: Advantages and Pitfalls
The HttpClient API has several advantages over the previous HttpUrlConnection. It supports HTTP/2 and WebSocket protocols, which can improve the performance of your applications. It’s also easier to use, with a more intuitive and fluent API.
However, there are a few potential pitfalls to be aware of. For example, the HttpClient API is immutable, which means you can’t modify its state after it’s been created. This can lead to unexpected behavior if you’re not careful. Additionally, while the HttpClient API is more powerful and flexible than HttpUrlConnection, it’s also more complex and can be harder to learn for beginners.
Epsilon Garbage Collector: An Advanced Insight
Java 11 introduces a new garbage collector called Epsilon. This is a ‘no-op’ (no operation) garbage collector, meaning it allocates memory but does not actually reclaim it.
Let’s understand this with an example. Consider this simple Java program:
public class EpsilonDemo {
public static void main(String[] args) {
for (int i = 0; i < 1000; i++) {
byte[] b = new byte[1024 * 1024];
}
}
}
To run this program using the Epsilon garbage collector, you would use the following command:
java -XX:+UnlockExperimentalVMOptions -XX:+UseEpsilonGC EpsilonDemo
This program simply allocates a block of memory (1MB) in a loop. If you run this program with a standard garbage collector, it will run without any problems because the garbage collector will periodically free up memory that is no longer in use.
However, if you run this program with the Epsilon garbage collector, it will eventually throw an OutOfMemoryError
because Epsilon does not reclaim any memory.
When to Use Epsilon Garbage Collector
You might be wondering why you would ever want to use a garbage collector that doesn’t actually collect garbage. The answer is that Epsilon is useful for performance testing. It can help you understand the memory requirements of your application and identify potential memory leaks.
However, Epsilon is not intended for general use. In a production environment, you would typically use a garbage collector that actually reclaims memory, such as G1 or Shenandoah.
Launching Single-File Source-Code Programs: An Alternative Approach
Java 11 introduced the ability to execute single-file source-code programs, a feature that simplifies Java application development. This feature allows you to run a Java program without compiling it first, making it faster and easier to test and debug your code.
Let’s explore this with a simple example. Consider this Java program saved in a file named HelloWorld.java
:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
In previous versions of Java, you would need to compile this program using javac
before you could run it with java
. But with Java 11, you can run it directly from the source file:
java HelloWorld.java
# Output:
# Hello, World!
The ability to run single-file source-code programs can significantly speed up the development process. It’s particularly useful for scripting, prototyping, and other tasks that require rapid feedback.
However, it’s important to note that this feature is not a replacement for traditional Java application deployment. For larger applications with multiple source files, you’ll still want to compile your code into a JAR or WAR file for deployment.
While exploring the new features in Java 11, you might encounter a few bumps along the way. Here, we’ll discuss some common issues and provide tips for resolving them.
HttpClient API: Handling Exceptions
When using the HttpClient API, you might come across exceptions like IOException
or InterruptedException
. These exceptions are thrown when an I/O error occurs or the send operation is interrupted.
Consider this example:
try {
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("http://example.com/"))
.build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
// Output:
// Exception in thread "main" java.net.ConnectException: Connection timed out: no further information
In this example, we’ve added a try-catch block to handle any potential exceptions. If an exception is thrown, it’s caught and the stack trace is printed.
Epsilon Garbage Collector: Managing Memory
When using the Epsilon garbage collector, you might run into OutOfMemoryError
if your program uses more memory than is available. To avoid this, you should carefully monitor your program’s memory usage and ensure it doesn’t exceed the available memory.
Single-File Source-Code Programs: Ensuring Correct Java Version
If you’re trying to run single-file source-code programs and encountering errors, ensure you’re using the correct Java version. This feature is only available in Java 11 and later versions. You can check your Java version with the following command:
java -version
// Output:
// java version "11.0.1" 2018-10-16 LTS
// Java(TM) SE Runtime Environment 18.9 (build 11.0.1+13-LTS)
// Java HotSpot(TM) 64-Bit Server VM 18.9 (build 11.0.1+13-LTS, mixed mode)
In this example, the output shows that the Java version is 11.0.1, which supports single-file source-code programs.
Journey Through Java: A Brief History
Java, a versatile and widely-used programming language, has seen numerous updates since its inception by Sun Microsystems in 1995. Each version brought new features, enhancements, and improvements, making Java more efficient and user-friendly.
The journey from Java’s first version to Java 11 has been significant. Let’s take a quick look at some of the major milestones:
- Java 5 (2004): Introduced generics, autoboxing/unboxing, and annotations, among other features.
- Java 7 (2011): Brought a new file I/O library (java.nio), support for dynamic languages, and the try-with-resources statement.
- Java 8 (2014): Introduced lambda expressions, streams, and functional interfaces, marking a major shift towards functional programming.
- Java 9 (2017): Added the Java Platform Module System (JPMS) and jshell, the interactive Java REPL.
- Java 10 (2018): Introduced local-variable type inference (var), allowing the type of local variables to be inferred.
And then came Java 11 in 2018, the subject of our exploration. This version introduced several new features like the HttpClient API, the Epsilon garbage collector, and the ability to run single-file source-code programs.
Here’s a simple example of using the new HttpClient API introduced in Java 11:
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("http://example.com/"))
.build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
// Output:
// The HTML content of the http://example.com page
In this example, we’ve used the new HttpClient API to send a GET request to http://example.com
and print the response body. This is just a glimpse of what Java 11 has to offer. As we delve deeper into Java 11 features, we’ll uncover more about its capabilities and how it can enhance your Java programming experience.
Applying Java 11 Features: Real-World Scenarios and Further Reading
The new features introduced in Java 11 aren’t just theoretical—they have practical applications that can make a real impact on your coding efficiency and the performance of your applications.
HttpClient API: Enhancing Web Communication
The HttpClient API, for instance, is a powerful tool for communicating with web servers. It can be used to build web clients, scrapers, or bots. Its support for HTTP/2 can improve the performance of your applications by reducing latency and saving bandwidth.
Epsilon Garbage Collector: Performance Testing
The Epsilon garbage collector, while not intended for general use, can be a valuable tool for performance testing. By not reclaiming any memory, it allows you to see the ‘raw’ memory usage of your application, helping you identify potential memory leaks or areas for optimization.
Single-File Source-Code Programs: Rapid Prototyping
The ability to run single-file source-code programs can be a game-changer for rapid prototyping. By eliminating the need for separate compilation, it allows you to quickly test and debug your code, speeding up the development process.
Further Resources for Java 11 Features
To deepen your understanding of Java 11 features and their applications, consider exploring the following resources:
- Installing Java Runtime Environment (JRE) on Ubuntu – Explore different methods to install Java on Ubuntu.
How to Update Java – Explore methods for updating Java Development Kit (JDK) and Java Runtime Environment (JRE).
Exploring Java 8 Updates – Explore the enhancements to Date and Time API and Optional class in Java 8.
Oracle’s Java 11 Documentation is a comprehensive resource covering all aspects of Java –
Baeldung’s Guide to Java 11 provides a detailed look at the new features in Java 11.
InfoQ’s Java 11 Features, Migration, and More offers info on Java 11 and a discussion on migration from older versions.
Wrapping Up: Java 11 Features
In this comprehensive guide, we’ve delved into the new features introduced in Java 11 and how they can enhance your programming experience.
We began with the basics, exploring the new HttpClient API and its advantages over the previous HttpUrlConnection. From there, we dove into more advanced territory, examining the Epsilon garbage collector and its unique ‘no-op’ behavior. Finally, we delved into the ability to run single-file source-code programs, a feature that simplifies Java application development and debugging.
Throughout our journey, we’ve tackled common issues that you might encounter when using these new features, providing solutions and tips to help you navigate these challenges. We’ve also discussed the broader context of Java’s evolution, giving you a deeper understanding of where these new features fit in the grand scheme of Java’s development.
Here’s a quick comparison of the features we’ve discussed:
Feature | Basic Use | Advanced Use | Troubleshooting Tips |
---|---|---|---|
HttpClient API | Send HTTP requests | Handle HTTP/2 and WebSocket protocols | Handle exceptions like IOException and InterruptedException |
Epsilon Garbage Collector | Understand ‘no-op’ behavior | Use for performance testing | Monitor memory usage to avoid OutOfMemoryError |
Single-File Source-Code Programs | Run Java code without compiling | Use for rapid prototyping and debugging | Ensure correct Java version |
Whether you’re just starting out with Java 11 or looking to deepen your understanding of its features, we hope this guide has been a valuable resource. With its mix of basic and advanced features, Java 11 is a powerful tool that can enhance your Java programming experience. Happy coding!