Exploring Java 8 Features: A Detailed Walkthrough
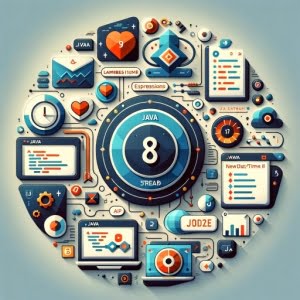
Are you curious about what’s new in Java 8? Just like a new season of your favorite TV show, Java 8 brought a host of exciting new features to the Java programming language. These features have not only improved the efficiency of Java but also made it more user-friendly.
This guide will walk you through each feature, from lambda expressions to the new date and time API. We’ll explore the core functionality of these features, delve into their advanced usage, and even discuss common issues and their solutions. So, let’s dive in and start exploring the features of Java 8!
TL;DR: What Are the Features of Java 8?
Java 8
introduced several new features, includinglambda expressions, functional interfaces, stream API, new date and time API
, and more. For instance, lambda expressions, used with the syntaxage -> System.out.println(age)
, allow for a concise representation of methods in functional programming.
Here’s a simple example of a lambda expression:
List<Integer> ages = Array.asList(12, 22, 33, 10);
ages.forEach(age -> System.out.println(age));
/* Output:
12
22
33
10
*/
In this example, we’ve used a lambda expression to print each name in the list. The lambda expression name -> System.out.println(name)
is a shorter and more readable way to iterate over the list compared to traditional for-each loops.
This is just a glimpse of what Java 8 has to offer. Continue reading for a more detailed explanation of each feature and how to use them effectively.
Table of Contents
- Unpacking the Power of Lambda Expressions and Functional Interfaces
- Stream API: Simplifying Data Processing
- New Date and Time API: Making Date and Time Manipulations Easier
- Java 8 vs Previous Versions: A Comparative Analysis
- Troubleshooting Java 8 Features: Common Issues and Solutions
- Evolution of Java: The Journey to Java 8
- Real-World Applications of Java 8 Features
- Wrapping Up: Java 8 Features
Unpacking the Power of Lambda Expressions and Functional Interfaces
Lambda Expressions
One of the most significant enhancements in Java 8 is the introduction of lambda expressions. Lambda expressions are a new syntax element that enable you to treat functionality as a method argument, or code as data. They provide a clear and concise way to represent one method interface using an expression. Lambda expressions also improve the collection library making it easier to iterate through, filter, and extract data from a collection.
Here’s a simple example of a lambda expression:
List<String> names = Arrays.asList("John", "Jane", "Adam", "Eve");
names.forEach(name -> System.out.println(name));
/* Output:
John
Jane
Adam
Eve
*/
In the above example, we’ve used a lambda expression to print each name in the list. The lambda expression name -> System.out.println(name)
is a shorter and more readable way to iterate over the list compared to traditional for-each loops.
Functional Interfaces
Along with lambda expressions, Java 8 introduced functional interfaces. A functional interface is an interface that contains only one abstract method. They are a perfect candidate for lambda expressions. In fact, you can use any interface with a SAM (Single Abstract Method) as a functional interface.
Here’s an example of a functional interface and its usage with a lambda expression:
@FunctionalInterface
interface Greeting {
void sayHello(String name);
}
public class Main {
public static void main(String[] args) {
Greeting greeting = (name) -> {
System.out.println("Hello, " + name);
};
greeting.sayHello("John");
}
}
/* Output:
Hello, John
*/
In this example, Greeting
is a functional interface with a single abstract method sayHello()
. We’ve used a lambda expression (name) -> { System.out.println("Hello, " + name); }
to provide the implementation of the sayHello()
method.
Lambda expressions and functional interfaces have greatly simplified the syntax and improved the readability of Java code. They have opened up possibilities for functional programming in Java and have made parallel processing more efficient.
Stream API: Simplifying Data Processing
The Stream API in Java 8 is another powerful feature that simplifies data processing. It can be used in combination with lambda expressions to provide a more declarative and composable approach to iterating over and processing data.
Here’s an example of how you can use the Stream API to filter and process data:
List<String> names = Arrays.asList("John", "Jane", "Adam", "Eve");
List<String> result = names.stream()
.filter(name -> name.startsWith("J"))
.collect(Collectors.toList());
System.out.println(result);
/* Output:
[John, Jane]
*/
In this example, we’re using the Stream API to filter the list of names and collect only those that start with ‘J’. The filter()
method takes a lambda expression that specifies the condition to filter the data.
New Date and Time API: Making Date and Time Manipulations Easier
Java 8 also introduced a new Date and Time API that is more intuitive and easier to use than the older java.util.Date
and java.util.Calendar
.
Here’s an example of how you can use the new Date and Time API:
LocalDate date = LocalDate.now();
LocalTime time = LocalTime.now();
System.out.println("Today's date: " + date);
System.out.println("Current time: " + time);
/* Output:
Today's date: 2022-09-24
Current time: 10:15:30.123
*/
In this example, LocalDate.now()
and LocalTime.now()
methods are used to get the current date and time. The new Date and Time API provides a more readable and simpler way to work with date and time in Java.
These advanced features of Java 8, the Stream API and the new Date and Time API, when used correctly, can greatly improve the efficiency and readability of your Java code.
Java 8 vs Previous Versions: A Comparative Analysis
Java 8 introduced several new features that made coding in Java easier and more efficient. However, it’s important to understand that these new features were not introduced to replace the old ones, but rather to provide more efficient and readable alternatives. Let’s compare some of these features with their counterparts in previous versions of Java.
Lambda Expressions vs Anonymous Classes
Before the introduction of lambda expressions in Java 8, anonymous classes were often used to implement interfaces with a single method. Here’s an example of how this was done:
List<String> names = Arrays.asList("John", "Jane", "Adam", "Eve");
names.forEach(new Consumer<String>() {
@Override
public void accept(String name) {
System.out.println(name);
}
});
/* Output:
John
Jane
Adam
Eve
*/
In this example, we’re using an anonymous class to implement the Consumer
interface and print each name in the list. This same functionality can be achieved more concisely with a lambda expression, as shown in the earlier examples.
Stream API vs Iterative Approach
Before the Stream API was introduced in Java 8, you would have to use iterative constructs like for-each loops to process collections. Here’s an example:
List<String> ages = Arrays.asList("John", "Jane", "Adam", "Eve");
List<String> result = new ArrayList<>();
for (String name : names) {
if (name.startsWith("J")) {
result.add(name);
}
}
System.out.println(result);
/* Output:
[John, Jane]
*/
In this example, we’re using a for-each loop to iterate over the list of names and add those that start with ‘J’ to the result list. The same functionality can be achieved more intuitively with the Stream API, as shown in the earlier examples.
These comparisons show that while the new features introduced in Java 8 provide more efficient and readable ways to achieve certain functionalities, the same functionalities can still be achieved with the features available in previous versions of Java.
Troubleshooting Java 8 Features: Common Issues and Solutions
While Java 8 has brought a host of new features that have simplified coding, there are common issues that developers often encounter when using these features. Let’s discuss some of these issues and their solutions.
Null Pointer Exceptions with Lambda Expressions
Lambda expressions can sometimes lead to Null Pointer Exceptions (NPEs) if not used carefully. Consider the following example:
List<String> names = Arrays.asList("John", null, "Adam", "Eve");
names.forEach(name -> System.out.println(name.length()));
/* Output:
4
Exception in thread "main" java.lang.NullPointerException
*/
In this example, we’re trying to print the length of each name in the list. However, since one of the names is null, a Null Pointer Exception is thrown. To avoid this, we can add a null check before calling any methods on the name:
names.forEach(name -> {
if (name != null) {
System.out.println(name.length());
}
});
/* Output:
4
4
3
*/
Misusing Stream API
The Stream API is a powerful tool, but it can be misused. For instance, calling stream()
on a null collection will result in a NullPointerException:
List<String> names = null;
long count = names.stream().count();
/* Output:
Exception in thread "main" java.lang.NullPointerException
*/
To avoid this, always ensure that the collection is not null before calling stream()
:
if (names != null) {
long count = names.stream().count();
}
These are just a few examples of the issues you might encounter when using Java 8 features. Always remember to handle null values and ensure that collections are not null before using the Stream API. By following these best practices, you can avoid common pitfalls and make the most of the new features introduced in Java 8.
Evolution of Java: The Journey to Java 8
Java, a class-based, object-oriented programming language, has evolved significantly since its inception in 1995. Its journey has been marked by the introduction of new features and enhancements that have made it one of the most popular programming languages in the world.
Java’s evolution can be divided into several key stages, each represented by a major release. Java 8, released in 2014, is one of these significant milestones. It marked a shift towards functional programming and brought several new features and improvements to the Java language.
Embracing Functional Programming with Java 8
One of the most notable changes in Java 8 is the shift towards functional programming. Prior to Java 8, Java was primarily an imperative language. While it did have some elements of object-oriented programming, the language lacked features that would allow developers to fully embrace functional programming.
Java 8 introduced several features that support functional programming, including lambda expressions and functional interfaces. These features have made it possible to write more concise and readable code, and have opened up new possibilities for parallel processing.
For example, consider the following code that uses a lambda expression:
List<String> names = Arrays.asList("John", "Jane", "Adam", "Eve");
names.forEach(name -> System.out.println(name));
/* Output:
John
Jane
Adam
Eve
*/
In this example, the lambda expression name -> System.out.println(name)
is a short and readable way to print each name in the list. Prior to Java 8, you would have to use a for-each loop to achieve the same result.
The introduction of these functional programming features in Java 8 has made Java a more versatile and powerful language. It has allowed developers to write more efficient code and has made it easier to process large amounts of data.
Real-World Applications of Java 8 Features
Java 8 features have found their place in a wide range of real-world applications, from web and mobile app development to machine learning and data analysis. Lambda expressions and Stream API, for instance, have made it easier to write efficient and readable code, thus improving the quality of software products and reducing development time.
The Relevance of Java 8 in Today’s Programming Landscape
Even though newer versions of Java have been released, the features introduced in Java 8 are still relevant and widely used today. Many organizations continue to use Java 8 in their production systems due to its stability and the powerful features it offers. Moreover, understanding these features is crucial for any Java developer as they form the foundation for many of the enhancements introduced in later versions.
Looking Ahead: Exploring Java 9 Features
While Java 8 marked a significant milestone in the evolution of Java, the journey didn’t stop there. Java 9 and later versions introduced several new features and improvements. For example, Java 9 introduced the Java Platform Module System (JPMS) that allows developers to create modular applications. It also introduced improvements to the Stream API and added several new methods to the Optional class.
If you’re comfortable with Java 8 features, it’s worth exploring the features introduced in Java 9 and later versions. This will not only expand your knowledge but also make you a more versatile Java developer.
Further Resources for Java 8
For more in-depth knowledge and understanding of Java 8 features, you can explore the following resources:
- Installing Java on Ubuntu: Complete Tutorial – Learn how to set up Java development environment on Ubuntu.
Understanding Java Versioning – Learn about the key features and improvements introduced in each major Java version.
New Features in Java 11 – Discover how Java 11 enhances developer productivity and application performance.
Java 8 Official Documentation provides comprehensive information about all the new features in Java.
Java 8 in Action provides a deep-dive into Java 8 features, including lambda expressions and the Stream API.
Baeldung’s Guide to Java 8 offers a collection of articles that explain various Java 8 features.
Wrapping Up: Java 8 Features
In this comprehensive guide, we’ve explored the exciting world of Java 8, highlighting its powerful new features and how they have revolutionized the way we code in Java.
We began with the basics, demystifying the usage of lambda expressions and functional interfaces. We then moved on to the more advanced features like the Stream API and the new Date and Time API, providing practical examples to illustrate their usage. We also compared these features with their counterparts in previous versions of Java, highlighting the improvements brought about by Java 8.
Along the way, we tackled common challenges that developers often encounter when using these features, such as Null Pointer Exceptions with lambda expressions and misuse of the Stream API, providing solutions to these issues. We also provided a brief overview of the evolution of Java, showing how the introduction of functional programming features in Java 8 has made it a more versatile and powerful language.
Feature | Pros | Cons |
---|---|---|
Lambda Expressions | Concise and readable code | Can lead to Null Pointer Exceptions if not used carefully |
Functional Interfaces | Enables functional programming in Java | Requires understanding of functional programming concepts |
Stream API | Simplifies data processing | Misuse can lead to errors |
New Date and Time API | More intuitive and easier to use | Different from traditional java.util.Date and java.util.Calendar |
Whether you’re just starting out with Java 8 or you’re an experienced developer looking to brush up on your knowledge, we hope this guide has given you a deeper understanding of Java 8 features and their applications.
With its balance of powerful features and ease of use, Java 8 has set a new standard for coding in Java. Happy coding!