Log4j2 in Java: Configuration Guide for Java Logging
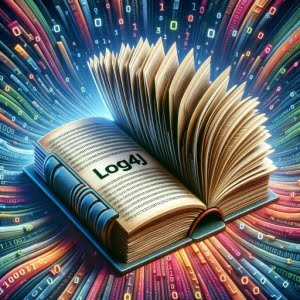
Are you finding it challenging to manage logging in your Java application? You’re not alone. Many developers find themselves in a maze when it comes to handling logs, but there’s a tool that can make this process a breeze.
Think of Log4j2 as a vigilant watchman, always keeping an eye on your application’s behavior. It’s a powerful tool that can seamlessly integrate with your Java application, providing you with detailed and customizable logs.
This guide will walk you through everything you need to know about Log4j2, from basic setup to advanced configurations. We’ll explore Log4j2’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Log4j2!
TL;DR: How Do I Configure Log4j2 in Java?
To use Log4j2 in Java, you first need to add the Log4j2 dependencies to your project with the import statements:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
Then create a Log4j2 configuration file with the syntax,
private static final Logger logger = LogManager.getLogger(HelloWorld.class);
You can then create a logger instance and use it to log messages.
Here’s a simple example:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class MyApp {
private static final Logger logger = LogManager.getLogger(MyApp.class);
public static void main(String[] args) {
logger.info("Hello, Log4j2!");
// Your application logic here
}
}
# Output:
# INFO HelloWorld - Hello, Log4j2!
This basic setup uses the LogManager
to create a Logger
instance. The logger.info()
method logs a simple message. Ensure log4j-api
and log4j-core
are in your classpath. For detailed configuration, use a log4j2.xml
or .properties
file in your project’s classpath.
Remember, Log4j2
provides enhanced performance and flexibility over its predecessor Log4j
, making it a robust choice for modern Java applications.
This is just a basic way to use
Log4j2
in Java, but there’s much more to learn about configuring and using this powerful logging library. Continue reading for more detailed instructions and advanced usage examples.
Table of Contents
- Setting Up Log4j2 in Your Java Project
- Exploring Advanced Log4j2 Features
- Comparing Log4j2 with Other Logging Frameworks
- Tackling Common Log4j2 Issues
- The Importance of Logging in Software Development
- Log4j2: Enhancing Java Logging
- Log4j2 in Larger Projects: Enterprise Applications and Microservices
- Wrapping Up: Using Log4j2 for Effective Java Logging
Setting Up Log4j2 in Your Java Project
To get started with Log4j2, you need to first add the necessary dependencies to your project. If you’re using Maven, you can add the following dependency to your pom.xml
file:
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>2.14.1</version>
</dependency>
This will include the core Log4j2 library in your project. The next step is to create a Log4j2 configuration file. This file defines how Log4j2 should log messages from your application. Here’s a simple example configuration file:
<Configuration status="INFO">
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"/>
</Console>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="Console"/>
</Root>
</Loggers>
</Configuration>
This configuration file tells Log4j2 to log messages at the INFO
level or higher to the console. The PatternLayout
defines the format of the log messages.
Now you’re ready to start logging messages with Log4j2. Here’s how you can log messages at different levels:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class HelloWorld {
private static final Logger logger = LogManager.getLogger(HelloWorld.class);
public static void main(String[] args) {
logger.info('This is an INFO message');
logger.debug('This is a DEBUG message');
logger.error('This is an ERROR message');
}
}
# Output:
# INFO HelloWorld - This is an INFO message
# ERROR HelloWorld - This is an ERROR message
In this example, we log messages at the INFO
, DEBUG
, and ERROR
levels. However, because our configuration file is set to log messages at the INFO
level or higher, the DEBUG
message does not appear in the output.
And there you have it! You’ve set up Log4j2 in your Java project and logged messages at different levels. But there’s much more to learn about Log4j2, so let’s move on to some of its more advanced features.
Exploring Advanced Log4j2 Features
After mastering the basic use of Log4j2, it’s time to delve into some of its more advanced features. These include custom log formats, filtering log messages, and using different appenders.
Custom Log Formats
Log4j2 allows you to customize the format of your log messages using PatternLayout
. This is done in the configuration file. Here’s an example:
<PatternLayout pattern="%d{HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"/>
This pattern includes the timestamp, thread, log level, logger name, and the log message itself. You can customize this pattern to include whatever information you find useful.
Filtering Log Messages
Sometimes, you might want to filter out certain log messages. Log4j2 provides several ways to do this. For example, you can use the ThresholdFilter
to only log messages above a certain level:
<ThresholdFilter level="ERROR"/>
This will only log messages at the ERROR
level or higher.
Using Different Appenders
Appenders define where your log messages go. By default, Log4j2 logs messages to the console, but you can change this. For example, you can use the FileAppender
to log messages to a file:
<File name="MyFile" fileName="logs/app.log" immediateFlush="false" append="false">
<PatternLayout pattern="%d{yyy-MM-dd HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"/>
</File>
This will log messages to a file named app.log
in the logs
directory. The immediateFlush
attribute controls whether each log message is immediately written to disk, and the append
attribute controls whether new log messages are appended to the existing file or overwrite it.
These are just a few examples of the advanced features of Log4j2. By leveraging these features, you can create a powerful and flexible logging system for your Java applications.
Comparing Log4j2 with Other Logging Frameworks
While Log4j2 is a powerful tool for logging in Java, it’s not the only option. Let’s compare Log4j2 with other popular logging frameworks like Logback and Java’s built-in logging utility (JUL).
Logback
Logback is considered the successor to Log4j and offers several improvements, such as faster performance and a more flexible configuration system. However, Log4j2 has caught up in many of these areas and even surpasses Logback in some aspects, such as support for asynchronous logging.
Here’s an example of how to log a message with Logback:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class HelloWorld {
private static final Logger logger = LoggerFactory.getLogger(HelloWorld.class);
public static void main(String[] args) {
logger.info('Hello, Logback!');
}
}
# Output:
# INFO HelloWorld - Hello, Logback!
As you can see, the code is very similar to the Log4j2 example. The main difference is that Logback uses the SLF4J API for logging.
Java’s Built-in Logging (JUL)
Java also has a built-in logging utility known as java.util.logging
(JUL). While it’s not as feature-rich as Log4j2 or Logback, it’s built into the Java platform and doesn’t require any additional dependencies.
Here’s an example of how to log a message with JUL:
import java.util.logging.Logger;
public class HelloWorld {
private static final Logger logger = Logger.getLogger(HelloWorld.class.getName());
public static void main(String[] args) {
logger.info('Hello, JUL!');
}
}
# Output:
# INFO: Hello, JUL!
Again, the code is similar to the Log4j2 and Logback examples, but there are some differences in the API.
In conclusion, while Log4j2, Logback, and JUL all provide logging capabilities for Java applications, they each have their own strengths and weaknesses. The best choice depends on your specific needs and circumstances.
Tackling Common Log4j2 Issues
While Log4j2 is a robust logging framework, like any tool, you might encounter a few hiccups along the way. Let’s look at common issues and their solutions when using Log4j2.
Configuration Problems
One common issue is configuration problems. For instance, if Log4j2 cannot find your configuration file, it will default to a basic configuration and log an error message.
ERROR StatusLogger No log4j2 configuration file found. Using default configuration...
To fix this, ensure that your configuration file is in the correct location and that its name matches the one specified in your code. If you’re using an XML configuration file, also ensure that it’s well-formed and valid.
Logging Performance Issues
Another common issue is logging performance. If logging is slowing down your application, you might want to consider asynchronous logging. Log4j2 provides built-in support for asynchronous logging, which can significantly improve logging performance.
To enable asynchronous logging, you need to include the Log4j2-async
dependency in your project and update your configuration file:
<Appenders>
<Async name="Async">
<AppenderRef ref="Console"/>
</Async>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="Async"/>
</Root>
</Loggers>
This configuration tells Log4j2 to log messages asynchronously, which can help alleviate any logging performance issues.
Remember, troubleshooting is an integral part of working with any new tool. The more you familiarize yourself with Log4j2, the easier it will be to diagnose and resolve any issues that arise.
The Importance of Logging in Software Development
Logging is a critical aspect of software development. It serves as a diagnostic tool that allows developers to track the flow of execution and monitor the state of an application. Logging provides valuable information about the system’s behavior and helps identify and troubleshoot issues that may arise.
Why is Logging Important?
Imagine trying to solve a mystery without any clues. That’s what debugging an application without logs is like. Logs provide context and detail about what the application was doing at a given time. They can provide insights into the state of the system before an error occurred, making it easier to identify and rectify issues.
Log4j2: Enhancing Java Logging
Log4j2 enhances the logging capabilities of Java applications. It’s not just about recording messages; Log4j2 provides a flexible and configurable system for controlling where these messages go (console, file, database, etc.), what they contain, and when they’re written.
Principles of Effective Logging with Log4j2
Effective logging is an art. Here are some principles to guide you when using Log4j2:
- Appropriate Log Levels: Use the appropriate level for logging messages. Log4j2 provides several levels, such as
ERROR
,WARN
,INFO
,DEBUG
, andTRACE
. Choose the level that best matches the importance and severity of the message. Meaningful Messages: Log messages should be informative and provide context. A good log message can save hours of debugging.
Consider Performance: Logging can impact the performance of your application. Use features like asynchronous logging to minimize this impact.
Secure Sensitive Data: Be careful not to log sensitive information. Log4j2 provides features like
Layouts
andFilters
to help control what gets logged.
In conclusion, logging is a vital tool for software development, and Log4j2 is a powerful ally in this task. By understanding and applying the principles of effective logging, you can maximize the benefits of Log4j2 in your Java applications.
Log4j2 in Larger Projects: Enterprise Applications and Microservices
Log4j2 isn’t just for small projects; it’s a robust logging framework capable of handling larger projects like enterprise applications and microservices. With its advanced features like asynchronous logging, Log4j2 can handle high-volume logging scenarios that are common in these types of applications.
Distributed Logging with Log4j2
In a microservices architecture, logs are generated by many different services. Managing these logs can be a challenge, but Log4j2 can help. It supports distributed logging out of the box, making it easier to aggregate and analyze logs from different services.
Integrating Log4j2 with Monitoring Tools
Log4j2 can also be integrated with popular monitoring tools like Splunk or ELK Stack. This allows you to centralize your logs and gain insights into your application’s behavior through data visualization.
Further Resources for Mastering Log4j2
If you are interested in learning more about documentation and logging, Click Here for an in-depth guide on the Javadoc tool.
Additionally, here are some other resources to help you on your journey:
- Log4j: Overview – Understand Log4j for robust logging in Java.
Understanding Annotations in Java – Learn to define and use custom annotations in Java.
Apache Log4j2 Official Documentation covers everything from basic setup to advanced features.
Baeldung’s Guide to Log4j2 provides a comprehensive guide to Log4j2, complete with code examples and explanations.
Log4j2 Tutorial by JournalDev provides a good overview of Log4j2’s features and how to use them.
With these resources and the principles discussed in this guide, you’re well on your way to mastering Log4j2. Happy logging!
Wrapping Up: Using Log4j2 for Effective Java Logging
In this comprehensive guide, we’ve explored the ins and outs of Log4j2, a powerful logging framework for Java applications.
We started with the basics, demonstrating how to set up Log4j2 in a Java project and log messages at different levels. We then ventured into more advanced territory, exploring custom log formats, filtering log messages, and using different appenders.
Along the way, we tackled common issues that you might encounter when using Log4j2, such as configuration problems and logging performance issues, providing you with solutions and workarounds for each issue.
We also compared Log4j2 with other popular logging frameworks like Logback and Java’s built-in logging utility (JUL). Here’s a quick comparison of these logging frameworks:
Framework | Flexibility | Performance | Ease of Use |
---|---|---|---|
Log4j2 | High | High | Moderate |
Logback | Moderate | High | High |
JUL | Low | Moderate | High |
Whether you’re just starting out with Log4j2 or looking to level up your Java logging skills, we hope this guide has given you a deeper understanding of Log4j2 and its capabilities.
With its balance of flexibility, performance, and ease of use, Log4j2 is a powerful tool for logging in Java. Happy coding!