Python Kafka Integration: Step-by-Step Guide
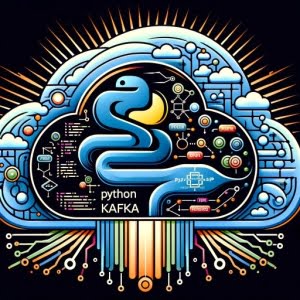
Are you finding it challenging to integrate Apache Kafka with Python? You’re not alone. Many developers grapple with this task, but there’s a tool that can make this process a breeze.
Like a skilled conductor, Apache Kafka can orchestrate your data streams with Python, ensuring a smooth flow of information. These streams can run on any system, even those without Python installed.
This guide will walk you through the steps to effectively use Apache Kafka with Python. We’ll explore Kafka’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Apache Kafka with Python!
TL;DR: How Do I Use Apache Kafka with Python?
To use Apache Kafka with Python, you need to install the
confluent-kafka
library likepip install confluent-kafka
. This library provides the necessary tools to produce and consume messages in Apache Kafka using Python.
Here’s a simple example:
from confluent_kafka import Producer, Consumer
p = Producer({'bootstrap.servers': 'localhost:9092'})
p.produce('mytopic', 'my message')
c = Consumer({'bootstrap.servers': 'localhost:9092', 'group.id': 'mygroup'})
c.subscribe(['mytopic'])
msg = c.poll(1.0)
print(msg.value())
# Output:
# 'my message'
In this example, we first import the necessary classes from the confluent-kafka
library. We then create a producer that connects to a Kafka server running on localhost and produce a message to a topic named ‘mytopic’.
Next, we create a consumer that also connects to the same Kafka server and subscribes to the ‘mytopic’ topic. The consumer then polls for new messages and prints the value of any message it receives.
This is just the beginning of what you can do with Apache Kafka and Python. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Getting Started with Apache Kafka and Python
- Handling Large Data Streams with Python Kafka
- Multiple Topics and Data Consistency
- Python Kafka: Exploring Alternative Approaches
- Troubleshooting Common Issues with Python Kafka
- Understanding Apache Kafka and Its Use Cases
- Why Use Apache Kafka with Python?
- Apache Kafka in Real-World Applications
- Wrapping Up: Mastering Apache Kafka with Python
Getting Started with Apache Kafka and Python
Before we can start using Apache Kafka with Python, we need to install the confluent-kafka
library. This library provides the necessary tools to produce and consume messages in Apache Kafka using Python.
You can install the confluent-kafka
library using pip:
pip install confluent-kafka
Now that we have the confluent-kafka
library installed, we can start producing and consuming messages with Apache Kafka.
Here’s a simple example:
from confluent_kafka import Producer, Consumer
p = Producer({'bootstrap.servers': 'localhost:9092'})
p.produce('mytopic', 'my message')
c = Consumer({'bootstrap.servers': 'localhost:9092', 'group.id': 'mygroup'})
c.subscribe(['mytopic'])
msg = c.poll(1.0)
print(msg.value())
# Output:
# 'my message'
In this example, we first import the necessary classes from the confluent-kafka
library. We then create a producer that connects to a Kafka server running on localhost and produce a message to a topic named ‘mytopic’. Next, we create a consumer that also connects to the same Kafka server and subscribes to the ‘mytopic’ topic. The consumer then polls for new messages and prints the value of any message it receives.
This example demonstrates the basic usage of Apache Kafka with Python. By using the confluent-kafka
library, we can easily produce and consume messages in Python. However, keep in mind that this is just a basic example and there are many more advanced features and techniques that we can use when working with Apache Kafka and Python.
Handling Large Data Streams with Python Kafka
Apache Kafka shines when it comes to handling large amounts of data. It’s designed to process streams of records in real-time, making it an excellent choice for big data applications. Let’s explore how to handle large amounts of data with Apache Kafka and Python.
from confluent_kafka import Producer, Consumer
import random
p = Producer({'bootstrap.servers': 'localhost:9092'})
for i in range(1000):
data = {'number': random.randint(1, 100)}
p.produce('mytopic', str(data))
p.flush()
c = Consumer({'bootstrap.servers': 'localhost:9092', 'group.id': 'mygroup'})
c.subscribe(['mytopic'])
while True:
msg = c.poll(1.0)
if msg is None:
continue
if msg.error():
print('Error: {}'.format(msg.error()))
continue
print('Received message: {}'.format(msg.value().decode('utf-8')))
# Output:
# 'Received message: {'number': 57}'
# 'Received message: {'number': 22}'
# 'Received message: {'number': 93}'
# ...
In this code, we’re producing a stream of 1000 messages, each containing a random number. We then consume these messages and print them out. This example demonstrates how Apache Kafka can handle large amounts of data with ease.
Multiple Topics and Data Consistency
Apache Kafka allows you to work with multiple topics. This can be useful when you want to segment your data stream for different types of data or different consumers. Let’s see how we can work with multiple topics in Apache Kafka.
from confluent_kafka import Producer, Consumer
p = Producer({'bootstrap.servers': 'localhost:9092'})
p.produce('topic1', 'message for topic1')
p.produce('topic2', 'message for topic2')
p.flush()
c = Consumer({'bootstrap.servers': 'localhost:9092', 'group.id': 'mygroup'})
c.subscribe(['topic1', 'topic2'])
while True:
msg = c.poll(1.0)
if msg is None:
continue
if msg.error():
print('Error: {}'.format(msg.error()))
continue
print('Received message on {}: {}'.format(msg.topic(), msg.value().decode('utf-8')))
# Output:
# 'Received message on topic1: message for topic1'
# 'Received message on topic2: message for topic2'
In this example, we’re producing messages to two different topics (‘topic1’ and ‘topic2’). We then consume messages from both topics and print out the topic and message. This demonstrates how you can work with multiple topics in Apache Kafka.
Data consistency is a crucial aspect of any data streaming application. Apache Kafka ensures data consistency by using replication and partitioning. This means that even if a part of your Kafka cluster fails, your data will still be safe and consistent.
As you can see, Apache Kafka offers a wide range of features for handling large amounts of data, working with multiple topics, and ensuring data consistency. With these tools at your disposal, you can build robust and scalable data streaming applications with Python and Apache Kafka.
Python Kafka: Exploring Alternative Approaches
While the confluent-kafka
library is a popular choice for integrating Apache Kafka with Python, there are other libraries and frameworks that you might consider depending on your specific needs. Let’s explore some of these alternatives.
PyKafka: A Pythonic Kafka API
PyKafka is a Python library that provides a high-level, Pythonic API for Apache Kafka. It supports Kafka’s low-level and high-level consumer APIs, as well as its producer API.
Here’s an example of how you can use PyKafka to produce and consume messages:
from pykafka import KafkaClient
client = KafkaClient(hosts='localhost:9092')
producer = client.topics['mytopic'].get_producer()
producer.produce('my message')
consumer = client.topics['mytopic'].get_balanced_consumer(consumer_group='mygroup')
message = consumer.consume()
print(message.value)
# Output:
# 'my message'
Faust: Stream Processing with Python
Faust is a Python library for stream processing and event-driven programming that is built on top of Kafka. With Faust, you can write Kafka applications in pure Python.
Here’s an example of how you can use Faust to process a stream of messages:
import faust
app = faust.App('myapp', broker='kafka://localhost:9092')
topic = app.topic('mytopic')
@app.agent(topic)
async def process(stream):
async for message in stream:
print(message)
Decision-Making Considerations
When choosing a library or framework for integrating Apache Kafka with Python, consider the following factors:
- Ease of use: How easy is it to produce and consume messages with the library or framework?
- Performance: How well does the library or framework handle large amounts of data?
- Features: Does the library or framework support the features you need, such as multiple topics, partitioning, and replication?
- Community and support: How active is the library or framework’s community? Is there good documentation and support available?
By considering these factors, you can choose the library or framework that best fits your needs for integrating Apache Kafka with Python.
Troubleshooting Common Issues with Python Kafka
As you work with Apache Kafka and Python, you might encounter a few common issues. Let’s discuss these potential roadblocks and how to overcome them.
Connection Issues
One common issue when working with Apache Kafka is connection problems. This can be due to a variety of reasons, such as the Kafka server not running, the server being overloaded, or network issues.
To troubleshoot this, you can check the status of the Kafka server and ensure it’s running correctly. If the server is overloaded, you might need to scale up your Kafka cluster or optimize your data processing.
Data Loss
Data loss can occur if messages are not properly acknowledged. This can happen if the consumer crashes before it can send an acknowledgment to the producer.
To prevent data loss, you can use Kafka’s built-in acknowledgment feature. This ensures that the producer receives an acknowledgment from the consumer before it sends the next message.
Here’s an example of how you can configure the producer to wait for acknowledgments:
from confluent_kafka import Producer
p = Producer({'bootstrap.servers': 'localhost:9092', 'acks': 'all'})
p.produce('mytopic', 'my message')
p.flush()
In this code, we’re setting the ‘acks’ option to ‘all’, which means the producer will wait for acknowledgments from all in-sync replicas before it sends the next message. This can help prevent data loss, but it might also increase latency.
Performance Problems
Performance problems can occur if you’re processing large amounts of data or if your Kafka cluster is not properly optimized.
To troubleshoot performance problems, you can use Kafka’s built-in monitoring tools to check the performance of your Kafka cluster. You might also need to optimize your data processing or scale up your Kafka cluster.
Remember, Apache Kafka is a powerful tool for handling real-time data streams, but it also comes with its own set of challenges. By understanding these common issues and their solutions, you can use Apache Kafka more effectively in your Python applications.
Understanding Apache Kafka and Its Use Cases
Apache Kafka is a distributed streaming platform designed to handle real-time data feeds. It’s a powerful tool used by many large-scale, data-intensive applications for its versatility and robustness.
Kafka works by storing, categorizing, and processing streams of records, called messages, in real-time. These messages are categorized into topics, which can be thought of as channels or streams of data.
# Representation of a Kafka message
{ 'topic': 'mytopic', 'message': 'my message' }
In this example, each message is associated with a topic (‘mytopic’) and contains some data (‘my message’).
Kafka’s architecture allows it to handle massive amounts of data in real-time, making it a popular choice for big data applications. Some common use cases for Kafka include:
- Real-time analytics: Kafka can process and analyze data in real-time, making it a good choice for live dashboards and monitoring systems.
- Event sourcing: Kafka can store a sequence of events in order, which can be useful for reconstructing the state of a system.
- Log aggregation: Kafka can collect log data from multiple services, making it easier to monitor and debug distributed systems.
Why Use Apache Kafka with Python?
Python is a popular, high-level programming language known for its readability and simplicity. It has a rich ecosystem of libraries and frameworks, making it a versatile tool for many types of projects.
Using Apache Kafka with Python allows you to leverage Python’s simplicity and Kafka’s power to build robust, scalable data streaming applications. Python’s confluent-kafka
library provides a high-level API for Kafka, making it easy to produce and consume messages in Python.
In addition, Python’s strong support for data processing and analysis makes it a good match for Kafka’s real-time data handling capabilities. By using Apache Kafka with Python, you can build powerful data pipelines that can process, analyze, and react to data in real-time.
Apache Kafka in Real-World Applications
Apache Kafka’s ability to handle real-time data streams makes it a valuable tool in many real-world applications. Let’s explore a few of these applications in more detail.
Real-Time Analytics with Kafka and Python
By integrating Apache Kafka with Python’s data analysis libraries, you can build powerful real-time analytics systems. These systems can process and analyze data as it comes in, providing insights and visualizations in real time.
from confluent_kafka import Consumer
import pandas as pd
c = Consumer({'bootstrap.servers': 'localhost:9092', 'group.id': 'mygroup'})
c.subscribe(['mytopic'])
data = []
for i in range(1000):
msg = c.poll(1.0)
if msg is not None:
data.append(msg.value())
df = pd.DataFrame(data)
print(df.describe())
# Output:
# 0
# count 1000.000000
# mean 49.530000
# std 28.867707
# min 1.000000
# 25% 25.000000
# 50% 50.000000
# 75% 74.000000
# max 100.000000
In this example, we’re consuming messages from a Kafka topic and storing them in a list. We then convert this list into a Pandas DataFrame and use the describe
method to provide a statistical summary of the data.
Event Sourcing and Log Aggregation with Kafka
Kafka’s ability to store a sequence of events in order makes it a good choice for event sourcing. In an event-sourced system, state changes are logged as a sequence of events, which can be replayed to reconstruct the system’s state at any point in time.
Similarly, Kafka’s ability to collect and categorize data from multiple sources makes it a powerful tool for log aggregation. By collecting logs from multiple services into a single Kafka topic, you can monitor and debug your distributed systems more easily.
Exploring Data Streaming and Distributed Systems
If you’re interested in real-time data processing, you might want to explore related concepts like data streaming and distributed systems. Data streaming involves processing data in real time as it comes in, while distributed systems involve splitting a single system across multiple machines or servers.
Further Resources for Mastering Kafka with Python
Here are some resources to help you learn more about Apache Kafka and its integration with Python:
- Python Libraries Selection: Quick Guide – Discover libraries for cybersecurity and encryption in Python.
Hypothesis Testing with t-Tests in Python – Learn how to perform t-tests in Python for data-driven decision-making.
Command-Line Applications in Python with Click – Dive into Click’s intuitive syntax and command definition features.
Apache Kafka – Official documentation of Apache Kafka and an overview of its features, and use cases.
Confluent’s Apache Kafka Python client – Detailed reference documentation of the
confluent-kafka
library, including installation instructions and examples.
Wrapping Up: Mastering Apache Kafka with Python
In this comprehensive guide, we’ve delved into the world of Apache Kafka and its integration with Python. We’ve explored how Apache Kafka can be a powerful tool for handling real-time data streams, and how Python’s simplicity and robustness make it an ideal language for building Kafka applications.
We started off with the basics, showing how to use the confluent-kafka
library to produce and consume messages in Apache Kafka. Then, we ventured into more advanced territory, demonstrating how to handle large amounts of data, work with multiple topics, and ensure data consistency.
We also tackled common issues you might face when using Apache Kafka with Python, such as connection problems, data loss, and performance issues, providing you with solutions and workarounds for each issue.
We didn’t stop there. We also explored alternative approaches to using Apache Kafka with Python, looking at other libraries and frameworks like PyKafka and Faust. We discussed the pros and cons of these alternatives and provided code examples to help you understand how they work.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Confluent-Kafka | Robust, supports many Kafka features | May require troubleshooting for some programs |
PyKafka | Simple and Pythonic API | Less robust than Confluent-Kafka |
Faust | High-level stream processing | Requires understanding of Kafka’s lower-level details |
Whether you’re just starting out with Apache Kafka and Python or you’re looking to level up your data streaming skills, we hope this guide has given you a deeper understanding of Apache Kafka and its capabilities.
With its balance of robustness, flexibility, and ease of use, Apache Kafka is a powerful tool for handling real-time data streams in Python. Now, you’re well equipped to enjoy those benefits. Happy coding!