Python Click Library Guide: Easily Build Command Line Applications in Python
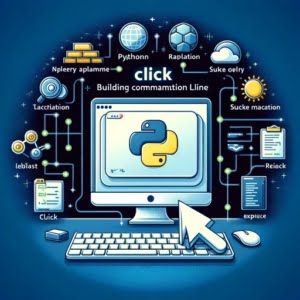
If you’ve been seeking a way to develop interactive and robust command line interfaces (CLI) in Python, you’re in the right place!
Python Click is a powerful library that simplifies CLI creation in Python, making your coding journey a breeze. Python Click is packed with features that facilitate CLI creation. It covers everything from passing context and handling nested commands to managing both mandatory and optional arguments.
Whether you’re a seasoned Python developer or a beginner, this article is designed to help you understand and harness the power of Python Click. So, buckle up and get ready to dive into the fascinating world of Python Click!
TL;DR: What is Python Click?
Python Click is a powerful library in Python that simplifies the creation of command line interfaces. It offers features such as passing context, handling nested commands, and managing arguments. For more advanced methods, background, tips and tricks, continue reading this article.
import click
@click.command()
def hello():
click.echo('Hello, Python Click!')
if __name__ == '__main__':
hello()
Table of Contents
- Python Click Fundamentals and Basic Usage
- Creating a Group with Python Click
- Understanding Context in Python Click
- Creating a Group Command that Interacts with a JSON Document
- Adding Commands to a Group
- Decorators in Python Click
- Secure Password Input with Python Click
- Advanced Features of Python Click
- Best Practices for Using Python Click
- Troubleshooting Tips
- Further Resources for Python Libraries
- Final Thoughts
Python Click Fundamentals and Basic Usage
Python Click is a third-party Python package designed to simplify the creation of interactive command line interfaces (CLIs). Think of it as a magic wand that transforms a few lines of code into a fully functional CLI.
Python Click stands out for its automatic help page generation. This feature is a significant time-saver, as it eliminates the need for manual documentation.
Python Click’s ease of use has made it a preferred choice among developers. Its simplicity and power make it a go-to tool for anyone looking to create command line interfaces in Python. So, if you’re ready to simplify your coding life and create more with less, Python Click is the way to go!
Creating a Group with Python Click
The first step in creating a command line interface with Python Click is creating a group. In Python Click, a group is akin to a command that houses other commands. You can think of it as the foundation of your CLI. Here’s how to create a group:
@click.command()
def greet():
click.echo('Hello, Python Click from greet command!')
@click.group()
def hello():
pass
hello.add_command(greet)
if __name__ == '__main__':
hello()
In the above code, the hello
group now has a sub-command greet
. When we run python filename.py greet
, it will print ‘Hello, Python Click from greet command!’.
Did you notice the @click.group()
decorator? That’s Python Click’s unique way of defining a group.
Understanding Context in Python Click
Next in line is understanding the concept of context in Python Click. Context is vital because it allows you to pass data between commands. This is implemented using the pass_context
decorator. Here’s an illustration of how to pass context:
import click
# Define the group and indicate that we're going to be using context
@click.group()
@click.pass_context
def hello(ctx):
# `ctx.obj` is a special object that's used for sharing data between commands
# You can store whatever you like in here
ctx.obj = 'Hello World!'
# Define a command that belongs to the group, and indicate that it accepts context
@hello.command()
@click.pass_context
def greet(ctx):
# When this command gets called, print the data stored in context
click.echo(ctx.obj)
if __name__ == '__main__':
hello()
In the above code, we’ve defined a command line interface with a group hello
and a command greet
. When the greet
command is invoked (e.g., by running python filename.py greet
), it accesses the context object that was defined in the hello
group, and prints out its value (Hello World!
). This demonstrates how data can be passed around between different commands.
Creating a Group Command that Interacts with a JSON Document
Python Click also enables you to create a group command that interacts with a JSON document. This comes in handy when you need to parse JSON data from the command line. Here’s an example:$2
Here’s an example of interacting with a JSON document:
import json
@click.command()
@click.option('--json', type=click.File('r'))
def hello(json_file):
data = json.load(json_file)
click.echo(f'Hello {data['name']}!')
In this example, we’re creating a command hello
that takes a JSON file as an option. The JSON file is read and parsed, and the name
value from the JSON data is used in the greeting.
Example json file:
// file: user.json
{
"name": "Alice"
}
Now to run this Python Click script, you would navigate to the directory containing the script and your JSON file in the terminal and issue the following command:
python filename.py --json user.json
Hello Alice!
This assumes that filename.py
is the name of the Python file containing the Click script. The script has read the JSON file, extracted the “name” field, and printed a greeting message using this name.
Adding Commands to a Group
Now that we’ve successfully created a group and understood the concept of context, let’s move forward and add some commands to our group.
The process of adding the first command to a Python Click group is quite straightforward. Let’s demonstrate this by adding a command greet
to our hello
group:
@click.group()
def hello():
pass
@click.command()
def greet():
click.echo('Hello, Python Click!')
hello.add_command(greet)
In the above example, we’re creating a command greet
and adding it to the hello
group using the add_command
function.
If the above python script is saved as hello.py
, here’s how you could run it in a bash shell using the greet
command:
python hello.py greet
Hello, Python Click!
Decorators in Python Click
Decorators play a pivotal role in Python Click. They provide a way to add functionality to an existing object without altering its structure. This is a powerful feature that contributes to Python Click’s versatility in CLI creation.
Python Click allows you to create a command that checks the context. This can be useful when you want to ensure that certain conditions are met before executing a command. Here’s an example:
@click.command()
@click.pass_context
def greet(ctx):
if ctx.obj == 'Hello World!':
click.echo('Hello, Python Click!')
else:
click.echo('Goodbye, Python Click!')
hello.add_command(greet)
In the above example, the greet
command checks the context before executing. If the context is ‘Hello World!’, it outputs ‘Hello, Python Click!’. Otherwise, it outputs ‘Goodbye, Python Click!’.
Secure Password Input with Python Click
Python Click also offers a unique feature, the password_option()
decorator, that allows secure password input through a hidden prompt. This is a handy feature when you need to take password input from the user. Here’s an example:
@click.command()
@click.password_option()
def login(password):
click.echo(f'Logging in with password: {password}')
In the above example, the password_option()
decorator creates a hidden prompt for password input. The entered password is then passed to the login
command.
Advanced Features of Python Click
As we delve deeper into Python Click, you’ll discover that it has a lot more to offer. One of these is the ability to make a pass
decorator. This feature is particularly useful when you want to pass some information from the group to the command. Here’s how you can do it:
@click.group()
@click.pass_context
def cli(ctx):
ctx.obj = {'key': 'value'}
@click.command()
@click.pass_context
def command(ctx):
click.echo(ctx.obj['key'])
cli.add_command(command)
In the above example, we’re passing a dictionary {'key': 'value'}
from the cli
group to the command
command using the pass_context
decorator.
The Power of the ‘pass_obj’ Decorator
Another powerful tool in Python Click is the pass_obj
decorator. This decorator allows you to pass an object from the group to the command. Here’s an example:
@click.group()
@click.pass_context
def cli(ctx):
ctx.obj = 'Hello, Python Click!'
@click.command()
@click.pass_obj
def command(obj):
click.echo(obj)
cli.add_command(command)
In the above example, we’re passing a string ‘Hello, Python Click!’ from the cli
group to the command
command using the pass_obj
decorator.
Creating Nested Commands with Python Click
Python Click also supports the creation of nested commands. This provides more flexibility and control to developers. Nested commands allow you to create a command hierarchy, making your CLI more organized and intuitive. Here’s how you can create nested commands:
@click.group()
def cli():
pass
@click.group()
def group():
pass
@click.command()
def command():
click.echo('Hello, Python Click!')
group.add_command(command)
cli.add_command(group)
In the above example, we’re creating a nested command command
inside the group
group, which is part of the cli
group.
Enhancing User Interaction with Python Click
But that’s not all. Python Click also enhances user interaction by prompting for value and providing colored output and text styling. For instance, you can use the prompt
option to ask for user input, and the style
function to colorize your output. Here’s an example:
@click.command()
@click.option('--name', prompt='Your name')
def hello(name):
click.secho(f'Hello, {name}!', fg='green')
In the above example, we’re prompting the user for their name and outputting a greeting in green color.
Best Practices for Using Python Click
Python Click is indeed a powerful tool, and like any tool, its effectiveness is dependent on how well you use it. Adhering to best practices can assure efficient and effective CLI creation. Here are some tips and best practices to maximize the benefits of Python Click.
Start With Defining a Group
Firstly, always commence by defining a group. A group acts as a foundation for your CLI and allows you to add commands and subcommands. This approach makes your CLI organized and intuitive. Here’s an example of defining a group:
@click.group()
def cli():
pass
Make Good Use of Decorators
Secondly, make optimal use of decorators. Decorators in Python Click allow you to add functionality to your commands without altering their structure. This approach makes your code cleaner and easier to maintain.
Always Remember to Pass Context
Next, always remember to pass context. Context allows you to share data between commands, making your CLI more flexible and powerful. Here’s how you can pass context:
@click.group()
@click.pass_context
def cli(ctx):
ctx.obj = 'Hello, Python Click!'
Troubleshooting Tips
Troubleshooting common issues in Python Click can be made easier with a few handy tips. For instance, if you’re having trouble with the pass_context
decorator, ensure you’re using it correctly. The pass_context
decorator should be used with a group or command, and the function should take a single parameter, usually named ctx
.
Optimizing the Use of Python Click
Optimizing the use of Python Click can lead to more streamlined and effective CLI creation. For instance, you can use the echo
function for output instead of the traditional print
function. The echo
function supports both bytes and Unicode and is more flexible, making it more suitable for CLI applications.
Here’s an example of using the echo
function:
@click.command()
def hello():
click.echo('Hello, Python Click!')
Maximizing the potential of Python Click can significantly enhance CLI creation. By following best practices, using decorators, passing context, and optimizing output, you can create powerful and user-friendly CLI applications with Python Click.
Further Resources for Python Libraries
For more insights on Python libraries and database interaction and management, Click Here! And if you are interested in learning more on specific useful Python libraries, consider the following resources:
- Kafka Integration with Python – Learn how to leverage Kafka in Python for scalable and distributed systems.
Big Data Processing with PySpark – A quick overview on distributed data processing, machine learning, and analytics with PySpark.
Python Modules – Get a basic understanding of Python modules with W3Schools.
Libraries in Python – Learn about various Python libraries with GeeksForGeeks.
Python Package Index (PyPI) – Explore and download various Python packages with the official Python Package Index.
The use of various Python Libraries can streamline your code, improve efficiency, and create more possibilities for innovation. As you journey through these libraries, you will unlock the true potential of Python.
Final Thoughts
And there you have it! We’ve embarked on an in-depth exploration into the world of Python Click, unveiling its power and versatility in creating command line interfaces. We’ve journeyed from understanding the basics of Python Click, to creating a group, adding commands, and delving into its advanced features, covering the entire spectrum.
We’ve also underscored the importance of context in Python Click and the power of decorators. We’ve seen how the pass_context
and pass_obj
decorators offer flexibility and ease in creating complex and robust CLI applications. We’ve also noted how Python Click enhances user interaction with features like prompting for value and providing colored output.
Ready to elevate your Python game? This is a must-read.
So, the next time you find yourself needing to create a command line interface in Python, remember Python Click. It’s not just a library, it’s your Swiss Army Knife for creating command line interfaces. It’s a powerful tool that can transform your coding experience. Happy coding!