PySpark: Complete Guide to Big Data Processing
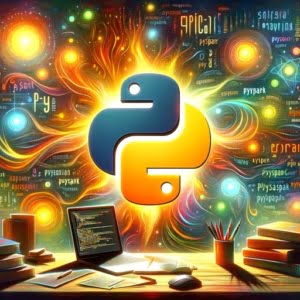
Are you on the lookout for a tool that can effectively harness the power of big data? PySpark could be the master key you’ve been searching for.
This powerful tool unlocks the potential of Apache Spark’s distributed computing capabilities, all from the comfort of your Python environment.
In this comprehensive guide, we’ll start with the basics of PySpark, gradually moving towards its advanced usage. By the end, you’ll have a solid understanding of how to use PySpark for big data processing, and you’ll be ready to tackle your own big data projects with confidence and ease.
TL;DR: How Do I Use PySpark for Big Data Processing?
PySpark can be used for big data processing by creating a SparkContext, loading data, and applying transformations and actions. Here’s a simple example:
from pyspark import SparkContext
sc = SparkContext('local', 'First App')
data = sc.parallelize([1,2,3,4,5])
data.count()
# Output:
# 5
In this example, we first import the SparkContext from PySpark. We then create a SparkContext, which is the entry point for any functionality in Spark. We’re naming our application ‘First App’ and running it locally. Next, we use the parallelize
method to distribute a list of numbers across multiple nodes in a cluster. Finally, we use the count
action to count the number of elements in our distributed data.
Stay tuned for more detailed explanations and advanced usage scenarios. This is just the tip of the iceberg when it comes to the power and versatility of PySpark for big data processing.
Table of Contents
- PySpark Basics: SparkContext and Data Loading
- Basic Transformations and Actions in PySpark
- Exploring PySpark’s Power: Spark SQL and DataFrames
- Dask and Hadoop: Alternatives to PySpark
- Troubleshooting PySpark: Common Issues and Solutions
- Understanding Apache Spark and Distributed Computing
- PySpark in Action: Real-world Big Data Projects
- Harnessing the Power of PySpark: A Recap
PySpark Basics: SparkContext and Data Loading
To start working with PySpark, we first need to establish a SparkContext. Think of the SparkContext as a communication channel between your Python program and the Spark cluster. It’s through this SparkContext that we can access all the functionalities of PySpark.
Here’s how to set up a SparkContext:
from pyspark import SparkContext
sc = SparkContext('local', 'First App')
In this code block, we first import the SparkContext from PySpark. We then instantiate a SparkContext object named ‘sc’. The parameters ‘local’ and ‘First App’ specify that we are running Spark on a single local machine and the name of our application is ‘First App’.
Now, let’s move on to data loading. In PySpark, we can load data into a distributed collection called an RDD (Resilient Distributed Dataset). Here’s an example of how to load data:
data = sc.parallelize([1,2,3,4,5])
In this line of code, we are using the parallelize
method of our SparkContext sc
to distribute a list of numbers across the nodes in the Spark cluster. This distributed collection of data is now stored in data
as an RDD.
Basic Transformations and Actions in PySpark
Once we have our data loaded into an RDD, we can perform transformations and actions on it. Let’s start with a simple transformation – filtering out even numbers from our data:
filtered_data = data.filter(lambda x: x % 2 == 0)
filtered_data.collect()
# Output:
# [2, 4]
In the first line, we use the filter
transformation to filter out even numbers. We pass a lambda function as an argument that returns True for even numbers. This transformation will create a new RDD, filtered_data
, which contains only the even numbers from our original data.
Next, we use the collect
action to retrieve the elements of the filtered_data
RDD from the Spark cluster to our local machine. The output, as expected, is a list of the even numbers [2, 4].
These are just the basics of PySpark. As we delve deeper into this guide, we’ll explore more complex operations and show how PySpark can be a powerful tool for big data processing.
Exploring PySpark’s Power: Spark SQL and DataFrames
Now that we’ve covered the basics, let’s delve into some of the more advanced features of PySpark, like Spark SQL and DataFrames. These tools allow us to handle structured and semi-structured data in a more sophisticated way.
Spark SQL: Querying Data the SQL Way
Spark SQL allows us to execute SQL queries on our data, making it easier to filter, aggregate, and analyze our datasets. Here’s an example of how you can use Spark SQL with PySpark:
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName('sql_example').getOrCreate()
data_df = spark.createDataFrame([(1, 'John', 28), (2, 'Mike', 30), (3, 'Sara', 25)], ['ID', 'Name', 'Age'])
data_df.createOrReplaceTempView('people')
results = spark.sql('SELECT * FROM people WHERE Age > 27')
results.show()
# Output:
# +---+----+---+
# | ID|Name|Age|
# +---+----+---+
# | 1|John| 28|
# | 2|Mike| 30|
# +---+----+---+
In this example, we first create a SparkSession
, which is the entry point to any Spark functionality. We then use the createDataFrame
method to create a DataFrame from a list of tuples, where each tuple represents a row of data. We assign column names to our DataFrame using the second argument of the createDataFrame
method.
We then register our DataFrame as a SQL temporary view using the createOrReplaceTempView
method. This allows us to treat our DataFrame as a SQL table. Finally, we use the sql
method to execute our SQL query and the show
method to display the results.
PySpark DataFrames: Handling Data at Scale
DataFrames in PySpark are a distributed collection of data that is organized into named columns. They are designed to make large data sets processing even easier. Here’s how you can create and manipulate a DataFrame:
data_df = spark.createDataFrame([(1, 'John', 28), (2, 'Mike', 30), (3, 'Sara', 25)], ['ID', 'Name', 'Age'])
filtered_df = data_df.filter(data_df.Age > 27)
filtered_df.show()
# Output:
# +---+----+---+
# | ID|Name|Age|
# +---+----+---+
# | 1|John| 28|
# | 2|Mike| 30|
# +---+----+---+
In this example, we first create a DataFrame similar to the previous example. We then use the filter
method of DataFrame to filter out rows where the ‘Age’ column is greater than 27. The show
method is used to display the results.
These are just a few examples of the advanced operations you can perform with PySpark. As you become more comfortable with these concepts, you’ll find that PySpark offers a wide range of tools and functionalities for handling big data.
Dask and Hadoop: Alternatives to PySpark
While PySpark is an excellent tool for big data processing, there are other Python libraries that offer similar functionality. Two such libraries are Dask and Hadoop. Let’s delve into these alternatives and compare them with PySpark.
Dask: Parallel Computing Made Easy
Dask is a flexible library for parallel computing in Python. It allows you to work with larger-than-memory datasets and perform complex computations with a simple and familiar Pythonic interface. Here’s an example of how you can use Dask:
import dask.array as da
x = da.random.random((10000, 10000), chunks=(1000, 1000))
y = x + x.T
z = y[::2, 5000:].mean(axis=1)
z.compute()
# Output:
# array([1.00058856, 0.99915962, 1.00028558, ..., 0.9982826 , 0.99960784,
# 1.00028733])
In this example, we first create a large 2D array filled with random numbers. The chunks
argument allows us to specify how to break up the array into smaller chunks, which can be processed independently. We then perform some operations on our array, and use the compute
method to execute our computation.
Hadoop: Big Data Processing in Java
Hadoop is another popular tool for big data processing, although it’s not a Python library. Hadoop is written in Java and is designed to scale up from single servers to thousands of machines. While Hadoop can be a powerful tool for big data processing, it has a steeper learning curve compared to PySpark or Dask due to its Java-based nature.
PySpark vs Dask vs Hadoop: Which One to Choose?
When it comes to choosing between PySpark, Dask, and Hadoop, it largely depends on your specific needs and the nature of your project. PySpark excels in processing large datasets and integrating with the larger Spark ecosystem. Dask is a great choice if you’re working with larger-than-memory computations and you prefer a Pythonic interface. Hadoop, on the other hand, is a good option if you’re dealing with extremely large datasets and you’re comfortable with Java.
All three tools have their strengths and weaknesses, and understanding these can help you choose the right tool for your big data processing needs.
Troubleshooting PySpark: Common Issues and Solutions
As with any tool, you might encounter some issues when working with PySpark. Let’s discuss some common problems and their solutions to help you navigate through these hurdles.
SparkContext Issues
One common issue is related to the initialization of the SparkContext. If you try to create more than one SparkContext in a single application, you’ll encounter an error. PySpark allows only one SparkContext per JVM.
from pyspark import SparkContext
sc = SparkContext('local', 'First App')
sc1 = SparkContext('local', 'Second App')
# Output:
# ValueError: Cannot run multiple SparkContexts at once
The solution is simple: you can stop the current SparkContext using the stop
method before creating a new one.
sc.stop()
sc1 = SparkContext('local', 'Second App')
Data Loading Problems
Another common issue is related to data loading. You might encounter an error if the file you’re trying to load doesn’t exist or the path is incorrect.
data = sc.textFile('nonexistent_file.txt')
data.count()
# Output:
# Py4JJavaError: An error occurred while calling o27.count.
To resolve this issue, ensure the file exists and the path specified is correct. If you’re loading a local file, it should be available on all worker nodes.
These are just a couple of the common issues you might encounter when working with PySpark. Remember, the key to effective troubleshooting is understanding the error messages and knowing where to look for solutions. With practice, you’ll become more proficient in navigating through these challenges and harnessing the full power of PySpark.
Understanding Apache Spark and Distributed Computing
To fully grasp the power of PySpark, we first need to understand the fundamentals of Apache Spark and distributed computing.
Apache Spark: A Powerful Data Processing Engine
Apache Spark is an open-source, distributed computing system used for big data processing and analytics. It provides an interface for programming entire clusters with implicit data parallelism and fault tolerance.
from pyspark import SparkContext
sc = SparkContext('local', 'First App')
data = sc.parallelize([1,2,3,4,5])
data.count()
# Output:
# 5
In this example, we’re using Apache Spark to distribute a list of numbers across a cluster and count the number of elements. This simple operation demonstrates the power of Spark’s distributed computing capabilities.
Distributed Computing: Breaking Down Big Data
Distributed computing is a model in which components of a software system are shared among multiple computers to improve efficiency and performance. In the context of big data, distributed computing allows us to process large datasets faster by dividing the data into smaller chunks, processing these chunks simultaneously across multiple nodes in a cluster.
sc = SparkContext('local', 'First App')
data = sc.parallelize([1,2,3,4,5,6,7,8,9,10], 2)
data.glom().collect()
# Output:
# [[1, 2, 3, 4, 5], [6, 7, 8, 9, 10]]
In this example, we’re dividing a list of numbers into two chunks using the parallelize
method’s second argument. The glom
method is then used to display the data as it is partitioned across the nodes in the cluster. This demonstrates how distributed computing breaks down data for faster processing.
PySpark: Bridging Python and Apache Spark
PySpark is the Python library for Apache Spark that allows you to harness the power of Spark’s distributed computing capabilities using Python. PySpark provides all the functionality of Spark’s built-in Scala library but with the familiar interface of Python, making it a popular choice for data scientists and developers alike.
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName('pyspark_example').getOrCreate()
data_df = spark.createDataFrame([(1, 'John', 28), (2, 'Mike', 30), (3, 'Sara', 25)], ['ID', 'Name', 'Age'])
data_df.show()
# Output:
# +---+----+---+
# | ID|Name|Age|
# +---+----+---+
# | 1|John| 28|
# | 2|Mike| 30|
# | 3|Sara| 25|
# +---+----+---+
In this example, we’re using PySpark to create a DataFrame, a distributed collection of data organized into named columns. This showcases PySpark’s ability to handle structured data at scale.
Understanding these fundamentals of Apache Spark, distributed computing, and PySpark will provide a strong foundation as we delve deeper into the world of big data processing with PySpark.
PySpark in Action: Real-world Big Data Projects
In the real world, PySpark is used extensively in big data projects across various industries. From healthcare to finance, PySpark’s distributed computing capabilities make it a powerful tool for processing large datasets and extracting valuable insights.
For instance, in the healthcare industry, PySpark can be used to analyze patient data and identify trends or patterns that can aid in disease prediction and prevention. In finance, PySpark can process transaction data to detect fraudulent activities or to make investment decisions.
Machine Learning with Spark MLlib
In addition to big data processing, PySpark also opens the door to machine learning with Spark MLlib. MLlib is Spark’s built-in library for machine learning, providing several algorithms for classification, regression, clustering, and collaborative filtering, as well as tools for model selection and evaluation.
Here’s a simple example of how to use Spark MLlib for linear regression:
from pyspark.ml.regression import LinearRegression
from pyspark.ml.linalg import Vectors
# Creating DataFrame
data = [(Vectors.dense([0.0]), 1.0), (Vectors.dense([1.0]), 2.0), (Vectors.dense([2.0]), 3.0)]
df = spark.createDataFrame(data, ['features', 'label'])
# Training a linear regression model
lr = LinearRegression()
model = lr.fit(df)
# Output:
# LinearRegressionModel: uid=LinearRegression_40b49c149b2f, numFeatures=1
In this example, we first create a DataFrame with two columns: ‘features’ and ‘label’. We then train a linear regression model using the fit
method of the LinearRegression
class. The output is a trained LinearRegressionModel
.
Further Resources for Mastering PySpark
Ready to dive deeper into PySpark? Here are some resources that can help you continue your learning journey:
- Python Libraries Tips for Productivity – Master the art of creating and parsing XML and JSON data with libraries.
CLI App Development with Python’s Click Library – Learn how to build robust command-line interfaces in Python with Click.
Python Clustering with K-Means – Practical examples of cluster analysis and data segmentation using K-means.
Apache Spark Documentation – The official Apache Spark documentation covers all aspects of Spark, including PySpark.
The Databricks Blog features several articles on PySpark, written by the creators of Apache Spark.
PySpark in Action by Manning Publications provides a hands-on introduction to PySpark, with plenty of examples and exercises.
With these resources and the knowledge you’ve gained from this guide, you’re well on your way to becoming a PySpark expert. Happy coding!
Harnessing the Power of PySpark: A Recap
Throughout this comprehensive guide, we’ve journeyed from the basics of PySpark to its advanced usage, exploring how this powerful tool can be harnessed for big data processing.
We’ve seen how to set up a SparkContext
, load data into an RDD, and apply transformations and actions. We’ve also delved into more complex operations like using Spark SQL and DataFrames, and even compared PySpark with alternative big data processing tools in Python such as Dask and Hadoop.
We’ve also discussed common issues you might encounter when using PySpark, such as problems with SparkContext
initialization and data loading, and provided solutions to navigate these hurdles. Remember, effective troubleshooting is all about understanding the error messages and knowing where to look for solutions.
PySpark | Dask | Hadoop |
---|---|---|
Powerful distributed computing capabilities | Pythonic interface for larger-than-memory computations | Handles extremely large datasets, Java-based |
In conclusion, PySpark stands out as a powerful tool for big data processing, combining the power of distributed computing with the simplicity and versatility of Python. Whether you’re a beginner just starting out or an expert looking to enhance your skills, PySpark offers a wide range of functionalities to help you handle big data with ease.
Keep exploring, keep learning, and harness the full power of PySpark in your data processing projects.