K-Means Clustering in Python | Detailed Tutorial
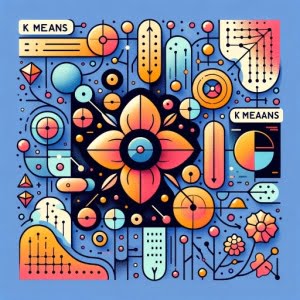
Are you grappling with the concept of k-means clustering? Think of it as a skilled cartographer for your data, helping you navigate and understand its complex terrain.
This algorithm, a key player in the machine learning arena, can be a little intimidating at first. But fear not!
This comprehensive guide is designed to walk you through the basics to the advanced techniques of k-means clustering. Whether you’re a beginner just dipping your toes into the world of machine learning, or an intermediate user looking to broaden your knowledge, this guide will serve as your map.
We’ll explore the concept of k-means clustering, its implementation, and how to tackle common issues that might arise. So, let’s embark on this journey to master k-means clustering together!
TL;DR: What is K-Means Clustering and How Do I Implement It?
K-means clustering is a type of unsupervised machine learning algorithm used to classify items into groups or clusters. It’s implemented by initializing ‘k’ centroids and iteratively assigning data points to the nearest centroid and recalculating the centroid until convergence.
Here’s a simple example:
from sklearn.cluster import KMeans
import numpy as np
# create a simple data set
X = np.array([[1, 2], [1, 4], [1, 0], [10, 2], [10, 4], [10, 0]])
# initialize k-means
kmeans = KMeans(n_clusters=2, random_state=0).fit(X)
# print cluster centers
print(kmeans.cluster_centers_)
# Output:
# [[10. 2.]
# [ 1. 2.]]
In this example, we import the necessary libraries and create a simple dataset. We then initialize k-means with two clusters and fit it to our data. Finally, we print the cluster centers, which are the centroids of our two clusters.
This is a basic way to implement k-means clustering in Python, but there’s much more to learn about handling different types of data, choosing the optimal number of clusters, and improving the performance. Continue reading for a more detailed understanding and practical examples.
Table of Contents
K-Means Clustering: A Beginner’s Guide
K-means clustering is an unsupervised machine learning algorithm that classifies data into a predetermined number of clusters. But how does it work? Let’s break it down.
The ‘K’ in K-means represents the number of clusters we want to classify our data into. The algorithm starts by randomly initializing these ‘K’ cluster centers or ‘centroids’. Each data point is then assigned to the nearest centroid, forming a cluster. The centroids are recalculated as the mean of all data points in the cluster. This process of assigning data points and recalculating centroids is repeated until the centroids no longer change significantly, indicating that the algorithm has converged.
Let’s look at a simple implementation of K-means clustering using the sklearn library in Python:
from sklearn.cluster import KMeans
import numpy as np
# create a simple data set
X = np.array([[1, 2], [1, 4], [1, 0], [10, 2], [10, 4], [10, 0]])
# initialize k-means
kmeans = KMeans(n_clusters=2, random_state=0).fit(X)
# print cluster centers
print(kmeans.cluster_centers_)
# Output:
# [[10. 2.]
# [ 1. 2.]]
In this example, we first import the necessary libraries and create a simple dataset. We then initialize K-means with two clusters and fit it to our data. The fit
method runs the algorithm on our data and calculates the centroids. Finally, we print the cluster centers, which are the centroids of our two clusters.
The output gives us two cluster centers at [10, 2] and [1, 2]. This means that our algorithm has successfully grouped our data into two clusters around these points.
This is a basic implementation of K-means clustering. However, as we’ll see in the next sections, there’s much more to consider, such as choosing the optimal number of clusters and handling different types of data.
Advanced Usage of K-Means Clustering
As we delve deeper into k-means clustering, we encounter more nuanced aspects such as selecting the optimal number of clusters, handling diverse datasets, and enhancing performance. Let’s explore these facets.
Choosing the Optimal Number of Clusters
The choice of ‘k’ or the number of clusters can significantly influence the outcome. A common method to determine ‘k’ is the Elbow Method. This involves running the k-means algorithm with varying ‘k’ values, calculating the within-cluster sum of squares (WCSS) for each, and plotting them. The ‘elbow’ point on the plot, where the WCSS starts to decrease slowly, is considered the optimal ‘k’.
Here’s how we can implement this in Python:
from sklearn.cluster import KMeans
import matplotlib.pyplot as plt
# create a simple data set
X = np.array([[1, 2], [1, 4], [1, 0], [10, 2], [10, 4], [10, 0]])
# calculate WCSS for different k values
wcss = []
for i in range(1, 6):
kmeans = KMeans(n_clusters=i, init='k-means++', max_iter=300, n_init=10, random_state=0)
kmeans.fit(X)
wcss.append(kmeans.inertia_)
# plot the elbow graph
plt.plot(range(1, 6), wcss)
plt.title('The Elbow Method')
plt.xlabel('Number of clusters')
plt.ylabel('WCSS')
plt.show()
# Output:
# An elbow plot where the x-axis represents the number of clusters and the y-axis represents the WCSS. The 'elbow' point on the plot is the optimal number of clusters.
In this code, we calculate the WCSS for ‘k’ values from 1 to 5 and plot them. The ‘elbow’ in the plot represents the optimal ‘k’. This method provides a more data-driven approach to choosing ‘k’.
Handling Different Types of Data
K-means clustering assumes that clusters are spherical and equally sized, which might not always be the case. For datasets with categorical variables or significant differences in cluster sizes, modifications to the algorithm or pre-processing steps might be required. For example, one might use K-Modes for categorical data or scale the variables to standardize cluster sizes.
Improving Performance
The performance of k-means clustering can be improved by using different initialization methods such as ‘k-means++’ which selects initial cluster centers in a way to speed up convergence. Also, reducing dimensionality of the data using techniques like PCA (Principal Component Analysis) can enhance the algorithm’s performance.
The advanced usage of k-means clustering involves a deeper understanding of the algorithm and its nuances. However, with practice and exploration, one can effectively use k-means clustering for complex and diverse datasets.
Exploring Alternative Clustering Techniques
While k-means clustering is a powerful tool, it’s not the only clustering technique available. Let’s look at some alternative approaches to clustering such as hierarchical clustering, DBSCAN, and spectral clustering. Each has its unique strengths and weaknesses, and your choice of algorithm should depend on the nature of your dataset and the specific problem you’re trying to solve.
Hierarchical Clustering
Hierarchical clustering is an algorithm that builds a hierarchy of clusters by merging or splitting existing clusters. This results in a tree-like diagram called a dendrogram, which provides a huge amount of information about the data. One of the main advantages of hierarchical clustering is that, unlike k-means, it doesn’t require us to specify the number of clusters beforehand.
Here’s a simple implementation of hierarchical clustering in Python using the scipy library:
from scipy.cluster.hierarchy import linkage, dendrogram
import matplotlib.pyplot as plt
# create a simple data set
X = np.array([[1, 2], [1, 4], [1, 0], [10, 2], [10, 4], [10, 0]])
# perform hierarchical clustering
Z = linkage(X, 'ward')
# plot dendrogram
dendrogram(Z)
plt.show()
# Output:
# A dendrogram representing the hierarchical clustering of the data points.
In this example, we use the ‘ward’ method for linkage, which minimizes the variance of the clusters being merged. The resulting dendrogram provides a visual representation of the hierarchical clustering.
DBSCAN
Density-Based Spatial Clustering of Applications with Noise (DBSCAN) is a density-based clustering algorithm, which can discover clusters of different shapes and sizes. It works by defining a cluster as a maximal set of density-connected points. One of the main advantages of DBSCAN over k-means is its capability to detect noise or outliers in the data.
Spectral Clustering
Spectral clustering uses the eigenvalues of a similarity matrix to reduce the dimensionality of the data before clustering in a lower-dimensional space. This can be very effective when the structure of the individual clusters is highly non-convex, or more generally when a measure of the center and spread of the cluster is not a suitable description of the complete cluster.
These are just a few of the many clustering algorithms available. Depending on the nature of your data and the specific problem you’re trying to solve, one of these methods might be more suitable than k-means clustering. It’s always a good idea to explore multiple approaches and choose the one that best meets your needs.
Troubleshooting Common Issues in K-Means Clustering
While k-means clustering is a powerful tool, like any algorithm, it has its quirks. Let’s explore some common issues you may encounter during k-means clustering and discuss potential solutions and workarounds.
Dealing with the ‘Curse of Dimensionality’
The ‘curse of dimensionality’ refers to the challenges and complications that arise when dealing with high-dimensional data. As the number of features increases, the feature space becomes increasingly sparse, making clusters harder to define. One potential solution is to reduce the dimensionality of the data using techniques such as Principal Component Analysis (PCA) or t-Distributed Stochastic Neighbor Embedding (t-SNE).
Sensitivity to Initial Centroids
The k-means algorithm is sensitive to the initial placement of centroids. Different initial placements can lead to different final clusters. A common workaround is to use the ‘k-means++’ initialization method, which chooses initial cluster centers in a way that speeds up convergence.
Here’s how you can implement k-means clustering with ‘k-means++’ initialization in Python:
from sklearn.cluster import KMeans
import numpy as np
# create a simple data set
X = np.array([[1, 2], [1, 4], [1, 0], [10, 2], [10, 4], [10, 0]])
# initialize k-means with k-means++ initialization
kmeans = KMeans(n_clusters=2, init='k-means++', random_state=0).fit(X)
# print cluster centers
print(kmeans.cluster_centers_)
# Output:
# [[10. 2.]
# [ 1. 2.]]
In this example, we use the ‘k-means++’ initialization method, which chooses initial cluster centers in a way that speeds up convergence. This can help mitigate the issue of sensitivity to initial centroids.
Handling Different Types of Data
As mentioned earlier, k-means clustering assumes that clusters are spherical and equally sized, which might not always be the case. For datasets with categorical variables or significant differences in cluster sizes, modifications to the algorithm or pre-processing steps might be required.
These are just a few of the issues you might encounter when working with k-means clustering. By understanding these potential pitfalls and how to address them, you can ensure that you’re using the k-means algorithm effectively.
Unraveling the Fundamentals of K-Means Clustering
To truly master k-means clustering, it’s important to understand the underlying theory and fundamental concepts that it’s based on. Let’s delve into the theory of clustering, the concept of distance metrics, and the role of centroids.
The Theory of Clustering
Clustering is a technique used in unsupervised machine learning to group similar data points together. The goal is to partition the data set into clusters so that data points within the same cluster are more similar to each other than to those in other clusters. This similarity is often based on a certain distance measure, which brings us to our next fundamental concept.
Understanding Distance Metrics
Distance metrics, such as Euclidean or Manhattan distance, quantify the similarity between data points. In the context of k-means clustering, we often use the Euclidean distance. The Euclidean distance between two points in a plane is the length of a straight line between them. In a high-dimensional space (like our data set), this concept extends naturally, but the distance is calculated in that high-dimensional space.
Here’s how you can calculate Euclidean distance in Python:
import numpy as np
# define two data points
point1 = np.array([1, 2, 3])
point2 = np.array([4, 5, 6])
# calculate Euclidean distance
distance = np.linalg.norm(point1 - point2)
print(distance)
# Output:
# 5.196152422706632
In this example, we calculate the Euclidean distance between two points in a 3-dimensional space. The result is approximately 5.2, which represents the ‘straight-line’ distance between the two points.
The Role of Centroids
Centroids play a crucial role in k-means clustering. A centroid is the center of a cluster, calculated as the mean of all the data points in the cluster. In k-means clustering, data points are assigned to the cluster whose centroid is nearest. The centroids are then recalculated, and this process repeats until the algorithm converges.
K-means clustering is a powerful tool in the machine learning toolkit, but it doesn’t exist in isolation. It’s part of the broader field of machine learning, which encompasses a range of techniques used to extract patterns from data. By understanding the fundamentals of k-means clustering, you can gain a deeper understanding of machine learning as a whole.
K-Means Clustering: Beyond the Basics
Having explored the fundamentals and advanced aspects of k-means clustering, it’s time to look at its real-world applications and related concepts. K-means clustering is not just a theoretical concept – it’s a practical tool used in a variety of fields and applications.
Real-World Applications of K-Means Clustering
One common application of k-means clustering is customer segmentation. Businesses with a large customer base often use k-means clustering to segment their customers into distinct groups based on purchasing behavior, demographics, or other characteristics. This allows them to target marketing efforts more effectively and improve customer service.
Another application of k-means clustering is image compression. By clustering the colors used in an image, k-means can reduce the number of unique colors to a set number of clusters. The colors in each pixel are then replaced with the centroid color of the cluster they belong to, significantly reducing the size of the image file.
Exploring Related Concepts
While k-means clustering is a powerful tool in itself, it’s just one piece of the machine learning puzzle. To deepen your understanding, consider exploring related concepts such as dimensionality reduction and feature selection.
Dimensionality reduction techniques, like Principal Component Analysis (PCA), can help simplify your data without losing important information. This can make k-means clustering more effective, particularly with high-dimensional data.
Feature selection, on the other hand, involves selecting the most relevant features for your model. This can improve the performance of your model and provide insight into the relationships between features.
Further Resources for Mastering K-Means Clustering
To further your understanding of k-means clustering, here are some resources that provide more in-depth information:
- Python Libraries Basics: Quick Insights – Dive deep into libraries for working with audio and video files.
Getting Started with PySpark – Explore PySpark, the Python library for big data processing with Apache Spark.
String Manipulation with Python Regex – Learn how to use regex for text validation, searching, and manipulation in Python.
Python Data Science Handbook by Jake VanderPlas contains a detailed section on k-means clustering with examples.
Scikit-Learn User Guide – The official Scikit-Learn user guide provides comprehensive information about k-means clustering.
Machine Learning Mastery – This website offers a wealth of articles and tutorials on various machine learning algorithms.
By exploring these resources and practicing your skills, you can continue your journey towards mastering k-means clustering and machine learning as a whole.
Wrapping Up K-Means Clustering
In this comprehensive guide, we’ve journeyed through the terrain of k-means clustering
, from its basic concepts to advanced techniques.
We began with an overview of k-means clustering, understanding it as a cartographer for data, mapping and grouping similar data points into clusters. We saw how it’s implemented in Python using the sklearn
library, initializing k
centroids and iterating through data points to assign them to the nearest centroid.
We explored common issues that might arise during k-means clustering, such as the ‘curse of dimensionality’ and sensitivity to initial centroids, and discussed potential solutions and workarounds. We also learned about the choice of ‘k’ or the number of clusters, and how it significantly influences the outcome. We introduced the Elbow Method as a data-driven approach to choose ‘k’, calculating the within-cluster sum of squares (WCSS) for varying ‘k’ values.
Beyond k-means clustering, we also looked at alternative clustering techniques like hierarchical clustering, DBSCAN, and spectral clustering. Each of these methods has its unique strengths and weaknesses, and the choice of method should depend on the nature of your dataset and the specific problem you’re trying to solve.
Finally, we discussed real-world applications of k-means clustering, such as customer segmentation and image compression, and suggested further resources for mastering this powerful machine learning tool.
Method | Strengths | Weaknesses |
---|---|---|
K-Means | Simple, efficient for large datasets | Assumes spherical clusters, sensitive to initial centroids |
Hierarchical | No need to specify number of clusters, provides a lot of information | More complex, slower for large datasets |
DBSCAN | Can find arbitrarily shaped clusters, good with noise | Not good with clusters of different densities |
Spectral | Good with non-convex clusters | Can be computationally expensive |
Remember, the journey to mastering machine learning is a marathon, not a sprint. Keep exploring, keep learning, and most importantly, keep practicing!