else if Python | How to use Python Conditional Statements
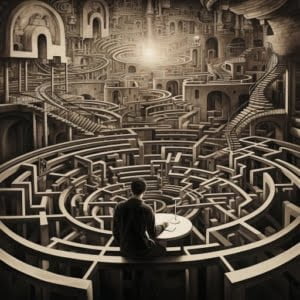
Ever wondered how Python programs make decisions, choosing between different paths of execution like a GPS navigating through various routes? The secret lies in Python’s decision-making statements. These statements are like the brain of your Python code, making decisions based on different conditions, similar to how we decide what to wear based on the weather.
In this blog post, we’ll delve deeper into these decision-making statements. We’ll explore how to use them effectively to control the flow of your Python programs, enabling them to make intelligent decisions. If you’ve ever found yourself puzzled by ‘if else Python’, ‘python if else’, or ‘python if statement’, you’re in the right place. Let’s dive in!
TL;DR: What is else if in Python?
Python’s decision-making statements include the
if
statement,if-else
statement, nestedif
statement, and theif-elif-else
ladder. Each of these statements helps control the flow of program execution based on certain conditions.
Table of Contents
- Understanding Control Flow in Python
- Exploring the Python If Statement
- Harnessing the Power of Shorthand If-Else and Ternary Operators in Python
- Understanding Nested If Statements in Python
- Navigating the If-Elif-Else Ladder in Python
- Further Resources for Python Conditionals
- Wrapping Up: The Power of Decision-Making in Python
Understanding Control Flow in Python
Just like a traffic signal organizes the flow of vehicles on the road, control flow in Python programming governs the sequence in which your code is executed. It’s a fundamental concept that makes your program dynamic, responsive, and intelligent, allowing it to make decisions and repeat actions based on specific conditions.
In this section, we’ll be exploring the four primary types of control flow statements in Python:
- If statement: This is the most basic form of control flow statement in Python. It allows your program to execute a block of code if a certain condition is true, much like a digital version of a ‘yes’ or ‘no’ question.
If-else statement: This extends the
if
statement by offering an alternative path of execution when the condition in theif
statement isn’t met. It’s like a fork in the road, giving your program a choice between two paths.Nested-if statement: This occurs when an
if
orif-else
statement is nestled within anotherif
orif-else
statement. It allows for more complex decision-making by evaluating multiple conditions, similar to a multi-layered decision tree.If-elif-else ladder: This is used when several conditions need to be checked. The program executes different blocks of code depending on which condition is met first, much like a multi-step decision-making process.
Control Statement | Description | Use Case |
---|---|---|
If Statement | Executes a block of code if a certain condition is true | Single condition decision making |
If-Else Statement | Offers an alternative path of execution when the condition in the if statement isn’t met | Two possible paths of execution |
Nested-If Statement | Allows for more complex decision-making by evaluating multiple conditions | Multi-layered decision tree |
If-Elif-Else Ladder | Executes different blocks of code depending on which condition is met first | Multi-step decision-making process |
Mastering these control flow statements is essential to structuring your Python code effectively. They empower your program to make smart decisions and execute different code blocks based on varying conditions. So, whether you’re grappling with ‘if else python’, ‘python else if’, or ‘if statements python’, getting a grip on these control flow statements is your first step.
Exploring the Python If Statement
Our first pit stop in the journey of Python’s decision-making statements is the if
statement. This statement is the simplest form of decision-making in Python, acting as a basic ‘yes’ or ‘no’ question to guide your program’s execution flow.
The if
statement in Python tests a specific condition and executes a block of code only if that condition proves to be true. Think of it as a road sign, directing your program down a particular path if the ‘yes’ condition is met.
The syntax of the if
statement in Python is simple and intuitive. It begins with the keyword if
, followed by the condition you want to test, and then a colon. The block of code to be executed if the condition is true is then written on the next line, indented under the if
statement.
Here’s a simple example to illustrate this:
x = 10
if x > 5:
print('x is greater than 5')
In this example, the if
statement checks if the condition x > 5
is true. Since it is, the print statement under the if
statement is executed, and ‘x is greater than 5’ is printed on the console.
Python’s unique use of indentation to define blocks of code is evident here. The print statement is indented under the if
statement to indicate that it’s part of the if
statement’s block of code. This use of indentation enhances the readability of Python code.
In essence, the if
statement is the foundation of decision-making in Python. If the condition is true, your program takes one path. If it’s false, it either does nothing or takes a different path, as we’ll see in the following sections.
Harnessing the Power of Shorthand If-Else and Ternary Operators in Python
Python provides a succinct version of the if-else
statement for more streamlined and space-efficient coding. This shorthand version, known as the Ternary Operator or Conditional Expression, enables you to encapsulate an entire if-else
statement in a single line of code.
The syntax for Python’s shorthand if-else
is straightforward:
[on_true] if [expression] else [on_false]
Here’s a practical illustration of the shorthand if-else
statement in Python:
x = 10
print('x is greater than 5') if x > 5 else print('x is not greater than 5')
In this example, the condition x > 5
holds true, so ‘x is greater than 5’ is printed on the console. If x
were less than or equal to 5, ‘x is not greater than 5’ would be printed instead.
The shorthand if-else
statement in Python is a powerful tool when you aim to write cleaner, more efficient code.
Here’s an example of a complex shorthand if-else
statement:
x = 10
y = 5
print('x and y are equal') if x == y else print('x is greater than y') if x > y else print('y is greater than x')
In this example, the program checks if x
and y
are equal. If they are not equal, it checks if x
is greater than y
. If x
is not greater than y
, it concludes that y
is greater than x
. This statement prints ‘x is greater than y’ because x
is indeed greater than y
.
It condenses a multi-line if-else
statement into a single line, enhancing the readability and conciseness of your code.
However, it’s worth noting that while this shorthand is a potent tool, it is best utilized in scenarios where the decision-making logic is simple and can be easily comprehended in one line. For more complex decision-making, the traditional if-else
statement or other control flow statements are more fitting.
Understanding Nested If Statements in Python
As we delve deeper into Python’s decision-making statements, we encounter a more complex concept – nested if
statements. A nested if
statement is essentially an if
or if-else
statement housed within another if
or if-else
statement. This allows for more intricate decision-making by facilitating layered condition checking, much like a decision within a decision.
In essence, nested if
statements enable you to evaluate another condition after the first condition is met. It’s akin to a two-step verification process, where the second step is only accessible after the first step is verified.
Consider the following example of a nested if
statement in Python:
x = 10
if x > 0:
print('x is positive')
if x > 5:
print('x is greater than 5')
In this example, the program initially checks if x
is greater than 0. If this condition is true, it prints ‘x is positive’ and then proceeds to check if x
is greater than 5. If this second condition is also true, it prints ‘x is greater than 5’. Thus, nested if
statements allow the program to make a subsequent decision based on the outcome of the initial decision.
Nested if
statements prove particularly useful when you need to evaluate multiple conditions that are interconnected. However, they should be used judiciously, as excessive nesting can make your code harder to read and understand. Also, remember that Python’s indentation rules apply to nested if
statements, so it’s crucial to indent your code correctly to define the different blocks of code.
In summary, nested if
statements in Python provide a means to make intricate decisions based on multiple layers of conditions, allowing for more complex and specific code execution.
The final destination in our exploration of Python’s decision-making statements is the if-elif-else
ladder. This control flow statement is akin to a complex decision tree, steering the flow of execution based on a sequence of conditions. It comes into play when there are multiple conditions to be evaluated, with different blocks of code to be executed for each condition.
The if-elif-else
ladder in Python commences with an if
statement, succeeded by one or more elif
(an abbreviation for ‘else if’) statements, and concludes with an optional else
statement. The program evaluates each condition in sequence, from top to bottom, and executes the code block corresponding to the first true condition. If all conditions prove false, it will execute the code block under the else
statement, if present.
Consider this example of an if-elif-else
ladder in Python:
x = 10
if x < 0:
print('x is negative')
elif x > 0:
print('x is positive')
else:
print('x is zero')
In this example, the program initially checks if x
is less than 0. Upon finding this condition false, it proceeds to the next condition (x > 0
). As this condition holds true, it prints ‘x is positive’ and bypasses the rest of the ladder. If x
were 0, none of the if
or elif
conditions would be true, leading it to print ‘x is zero’.
The if-elif-else
ladder is a potent tool for managing multiple conditions in Python. It empowers your program to make intricate decisions based on a series of conditions, each leading to a distinct path of execution. However, like any powerful tool, it should be employed with discretion, as an excessive number of conditions can render your code more challenging to read and understand.
Further Resources for Python Conditionals
- Python If Statement Essentials – Discover the power of Python’s if-else statements for handling multiple scenarios.
Python’s elif Conditional – Dive into the details of using “elif” for handling conditions in Python.
Logical OR in Python – Discover how to combine conditions with “or” in Python for logical disjunctions.
Python’s official documentation on assignment expressions provides a detailed explanation of all conditionals.
Guide to Python Booleans – An exhaustive guide by W3Schools that focuses on understanding and working with booleans in Python.
Conditional Statements in Python on Medium – A Medium article, discussing the use of conditional statements in Python.
Wrapping Up: The Power of Decision-Making in Python
Through this blog post, we’ve journeyed through the landscape of Python’s decision-making statements, exploring their power and versatility in shaping the flow of your Python programs. We started with the basic if
statement, the cornerstone of decision-making that enables your program to make simple, binary decisions.
We then navigated through the if-else
statement and its shorthand version, offering an alternative route of execution when the if
condition isn’t satisfied. We delved deeper into the realm of complex decision-making with nested if
statements, enabling layered condition checking for intricate logical structures.
Finally, we climbed the if-elif-else
ladder, a potent tool for handling multiple conditions and directing the flow of execution based on a series of conditions. Each of these decision-making statements is like a control dial, allowing your Python programs to make intelligent decisions based on varying conditions.
Python’s vast syntax can be overwhelming. Navigate it effortlessly with our comprehensive guide.
Whether you’re trying to figure out ‘if else python’, ‘python else if’, or ‘if statements python’, understanding these control flow statements is your first step. But remember, the key to truly mastering Python’s decision-making statements lies in practice. So, don’t hesitate to roll up your sleeves and start writing some Python code. The best way to learn is by doing. Happy coding!