Bash Loops | ‘For’, ‘While’, and ‘Until’ How-to Guide
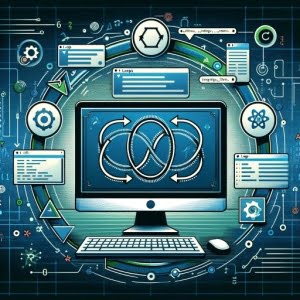
Are you feeling a bit lost when it comes to loops in Bash? You’re not alone. Many developers find themselves in a maze when it comes to using loops in Bash, but we’re here to guide you through it.
Think of Bash loops as a well-oiled machine – they can automate repetitive tasks, making your scripts more efficient and easier to maintain. They’re like the gears that keep the engine running smoothly.
In this guide, we’ll walk you through the process of using loops in Bash, from the basics to more advanced techniques. We’ll cover everything from creating simple loops to handling more complex looping scenarios, as well as troubleshooting common issues.
So, let’s dive in and start mastering Bash loops!
TL;DR: How Do I Create a Loop in Bash?
In Bash, you can create a loop using the
'for'
,'while'
, or'until'
keywords. Afor
loop follows the syntax,for x in {y}
. Both theuntil
andwhile
loops follow the same syntax,until/while [condtion]
. These loops are powerful tools that can automate repetitive tasks in your scripts.
Here’s a simple example of a ‘for’ loop:
for i in {1..5}
do
echo $i
done
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, we create a ‘for’ loop that iterates over the numbers 1 through 5. The echo $i
command is executed for each iteration, printing the current value of i
.
This is just a basic way to use loops in Bash, but there’s much more to learn about creating and controlling loops for various tasks. Continue reading for more detailed examples and advanced usage scenarios.
Table of Contents
- Bash Loop Basics: For, While, and Until
- Advanced Bash Loop Techniques
- Exploring Alternative Approaches in Bash Looping
- Troubleshooting Bash Loops: Common Issues and Solutions
- Understanding the Concept of Loops in Bash
- Applying Bash Loops in Larger Projects
- Further Resources for Mastering Bash Loops
- Wrapping Up: Mastering Bash Loops
Bash Loop Basics: For, While, and Until
The ‘For’ Loop in Bash
The ‘for’ loop in Bash is one of the easiest ways to start automating repetitive tasks. It allows you to execute a command or series of commands for each item in a list.
Here’s a simple example:
for name in Alice Bob Charlie
do
echo Hello, $name!
done
# Output:
# Hello, Alice!
# Hello, Bob!
# Hello, Charlie!
In this example, we’re using a ‘for’ loop to say hello to Alice, Bob, and Charlie. The loop iterates over each name in the list, and for each iteration, it executes the echo
command.
The ‘While’ Loop
The ‘while’ loop in Bash is used to perform a task while a certain condition is true. Here’s an example:
counter=1
while [ $counter -le 5 ]
do
echo Counter: $counter
((counter++))
done
# Output:
# Counter: 1
# Counter: 2
# Counter: 3
# Counter: 4
# Counter: 5
In this example, we’re using a ‘while’ loop to count from 1 to 5. The loop continues as long as the counter is less than or equal to 5. Each time the loop runs, it increments the counter by 1.
The ‘Until’ Loop
The ‘until’ loop in Bash is similar to the ‘while’ loop, but it runs until the condition is true. Here’s an example:
counter=1
until [ $counter -gt 5 ]
do
echo Counter: $counter
((counter++))
done
# Output:
# Counter: 1
# Counter: 2
# Counter: 3
# Counter: 4
# Counter: 5
In this example, the ‘until’ loop also counts from 1 to 5. However, it continues until the counter is greater than 5. Each time the loop runs, it increments the counter by 1.
These are the basic uses of ‘for’, ‘while’, and ‘until’ loops in Bash. They’re simple yet powerful tools for automating repetitive tasks in your scripts. However, like any tool, they must be used wisely to avoid potential pitfalls, such as infinite loops.
Advanced Bash Loop Techniques
Nested Loops in Bash
Nested loops are loops within loops, and they can be incredibly useful for handling more complex tasks. Here’s an example of a nested ‘for’ loop in Bash:
for i in {1..3}
do
for j in {1..3}
do
echo "$i - $j"
done
done
# Output:
# 1 - 1
# 1 - 2
# 1 - 3
# 2 - 1
# 2 - 2
# 2 - 3
# 3 - 1
# 3 - 2
# 3 - 3
In this example, we have an outer loop that iterates over the numbers 1 to 3, and an inner loop that also iterates over the numbers 1 to 3. For each iteration of the outer loop, the inner loop runs completely, resulting in a total of 9 iterations.
Looping Over Arrays
Bash loops can also iterate over arrays. Here’s an example:
fruits=('Apple' 'Banana' 'Cherry')
for fruit in "${fruits[@]}"
do
echo "I like $fruit"
done
# Output:
# I like Apple
# I like Banana
# I like Cherry
In this example, we’re using a ‘for’ loop to iterate over an array of fruits. The loop executes the echo
command for each fruit in the array.
Loop Control with ‘Break’ and ‘Continue’
The ‘break’ and ‘continue’ commands can be used to control the flow of a loop. Here’s an example:
for number in {1..10}
do
if [ $number -eq 5 ]
then
break
fi
echo $number
done
# Output:
# 1
# 2
# 3
# 4
In this example, we’re using a ‘for’ loop to count from 1 to 10. However, we’ve added a condition inside the loop that breaks the loop when the number equals 5. As a result, the loop only counts from 1 to 4.
These are just a few examples of the more complex uses of loops in Bash. As you can see, Bash loops are not just about automating repetitive tasks – they’re also about controlling the flow of your scripts to achieve your desired outcomes.
Exploring Alternative Approaches in Bash Looping
Using ‘seq’ with a ‘for’ Loop
The ‘seq’ command in Bash generates a sequence of numbers, which can be used with a ‘for’ loop for more flexible control over the iteration. Here’s an example:
for i in $(seq 1 2 10)
do
echo $i
done
# Output:
# 1
# 3
# 5
# 7
# 9
In this example, we’re using ‘seq’ to generate a sequence of odd numbers from 1 to 10. The ‘for’ loop then iterates over this sequence. This approach gives you more control over the iteration, allowing you to specify the start, step, and end values.
Using the ‘select’ Construct
The ‘select’ construct in Bash is a less-known feature that can be used to create menu-driven scripts. Here’s an example:
options=('Apple' 'Banana' 'Cherry' 'Quit')
select opt in "${options[@]}"
do
case $opt in
'Apple')
echo 'You chose Apple.'
;;
'Banana')
echo 'You chose Banana.'
;;
'Cherry')
echo 'You chose Cherry.'
;;
'Quit')
break
;;
*)
echo 'Invalid option.'
;;
esac
done
In this example, we’re using ‘select’ to present a menu of fruits. The user can enter a number to choose a fruit, or choose to quit the menu. This approach can be very useful for creating interactive scripts.
These alternative approaches to Bash looping can provide more flexibility and control over your scripts. However, they also come with their own set of considerations. For example, using ‘seq’ with a ‘for’ loop can make your scripts more complex, and using the ‘select’ construct can make them more interactive but also more prone to user error. As always, the best approach depends on your specific needs and circumstances.
Troubleshooting Bash Loops: Common Issues and Solutions
Avoiding Infinite Loops
One of the most common issues when working with loops in Bash is creating an infinite loop. This happens when the loop’s condition always evaluates to true, causing the loop to run indefinitely. Here’s an example of an infinite ‘while’ loop:
# WARNING: This is an infinite loop. Do not run this code.
while true
do
echo 'Looping...'
done
In this example, because the condition true
is always true, the loop will run forever, printing ‘Looping…’ until you manually stop it. To avoid this, always ensure that your loop’s condition will eventually evaluate to false.
Handling Syntax Errors
Another common issue is syntax errors, which can occur if you forget to include necessary keywords or use incorrect syntax. For instance, forgetting to include the do
keyword in a ‘for’ loop will result in a syntax error:
# This code will result in a syntax error.
for i in {1..5}
echo $i
done
To fix this, simply include the do
keyword:
for i in {1..5}
do
echo $i
done
# Output:
# 1
# 2
# 3
# 4
# 5
Optimizing Your Loops
Finally, while Bash loops are powerful, they can also be slow, especially when working with large amounts of data. To optimize your loops, consider using built-in Bash commands or utilities that can perform the task more efficiently. For instance, instead of using a ‘for’ loop to process a file line by line, you could use the awk
or sed
command.
These are just a few of the common issues you might encounter when working with loops in Bash, as well as some tips for best practices and optimization. Remember, the key to effective Bash scripting is not just knowing how to write loops, but also understanding how to troubleshoot and optimize them.
Understanding the Concept of Loops in Bash
The Power of Looping
In Bash scripting, a loop is a block of code that is executed repeatedly based on a condition. It’s a fundamental concept in programming, allowing us to automate repetitive tasks and make our scripts more efficient.
Consider a scenario where you need to print the numbers 1 to 5. Without loops, you’d have to write five separate echo
commands. But with a simple ‘for’ loop, you can accomplish the task with just a few lines of code:
for i in {1..5}
do
echo $i
done
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, the ‘for’ loop iterates over the numbers 1 to 5, executing the echo
command for each iteration. This demonstrates the power of looping – it simplifies our script and reduces the amount of code we have to write.
Variables and Command Substitution
Variables and command substitution are two related concepts that often come into play when working with loops in Bash.
A variable in Bash is a name assigned to a piece of data. In the context of loops, variables are often used to control the loop’s behavior. For instance, in a ‘while’ loop, a variable might be used as a counter:
counter=1
while [ $counter -le 5 ]
do
echo $counter
((counter++))
done
# Output:
# 1
# 2
# 3
# 4
# 5
Command substitution is a feature in Bash that allows you to use the output of a command as an input to another command. It’s often used in loops to generate the list of items to iterate over. For example, you can use the ls
command to generate a list of files, and then use a ‘for’ loop to iterate over the files:
for file in $(ls)
do
echo $file
done
In this example, the $(ls)
command substitution generates a list of files, and the ‘for’ loop iterates over this list, printing the name of each file.
Understanding Exit Status
The exit status of a command is a value returned by the command to indicate whether it succeeded or failed. In Bash, an exit status of 0 indicates success, while any other value indicates failure. The exit status is often used in ‘while’ and ‘until’ loops to control the loop’s execution. For instance, you might use a ‘while’ loop to keep asking for user input until a valid input is entered:
while true
do
read -p "Enter a number: " number
if [[ $number =~ ^[0-9]+$ ]]
then
break
fi
done
In this example, the ‘while’ loop keeps asking for user input until a valid number is entered. The [[ $number =~ ^[0-9]+$ ]]
condition checks if the input is a number, and if it is, the loop breaks.
Understanding these fundamental concepts – loops, variables, command substitution, and exit status – is crucial to mastering Bash scripting and creating efficient, powerful scripts.
Applying Bash Loops in Larger Projects
Bash Loops and Conditional Statements
In the world of Bash scripting, loops often go hand in hand with conditional statements. Conditional statements allow your scripts to make decisions based on certain conditions, leading to more complex and dynamic scripts.
For instance, you might use a ‘for’ loop to iterate over a series of files, and a conditional ‘if’ statement to perform different actions depending on the file type:
for file in *.*
do
if [[ $file == *.txt ]]
then
echo "$file is a text file."
else
echo "$file is not a text file."
fi
done
# Output:
# file1.txt is a text file.
# file2.jpg is not a text file.
# file3.txt is a text file.
In this example, the ‘for’ loop iterates over all files in the current directory, and the ‘if’ statement checks if the file extension is ‘.txt’. Depending on the result, it prints a different message.
Bash Loops and Functions
Functions are another concept that often accompanies loops in Bash scripts. Functions allow you to group commands into reusable blocks, making your scripts more modular and easier to maintain.
For example, you might create a function to perform a task, and then use a ‘for’ loop to call that function for each item in a list:
function greet() {
echo "Hello, $1!"
}
for name in Alice Bob Charlie
do
greet $name
done
# Output:
# Hello, Alice!
# Hello, Bob!
# Hello, Charlie!
In this example, we define a function greet
that takes one argument and prints a greeting message. The ‘for’ loop then calls this function for each name in the list.
Further Resources for Mastering Bash Loops
If you’re eager to learn more and take your Bash scripting skills to the next level, here are some resources that offer more in-depth information about Bash loops and related topics:
- GNU Bash Manual: The official manual for Bash, offering comprehensive information about all features of the shell, including loops.
Bash Scripting Guide: An in-depth guide to Bash scripting, covering everything from basic to advanced topics.
Learn Shell: An interactive platform that offers tutorials on various aspects of shell scripting, including loops, conditional statements, and functions.
Wrapping Up: Mastering Bash Loops
In this comprehensive guide, we’ve traversed the landscape of Bash loops, a fundamental concept in Bash scripting that can automate repetitive tasks and make your scripts more efficient and maintainable.
We began with the basics, exploring how to create ‘for’, ‘while’, and ‘until’ loops in Bash. We then delved into more advanced topics, such as nested loops, looping over arrays, and controlling loops with ‘break’ and ‘continue’. We also tackled common issues you might encounter when working with loops in Bash, such as infinite loops and syntax errors, and provided solutions to these challenges.
We didn’t stop at the traditional uses of loops, but also explored alternative approaches, such as using ‘seq’ with a ‘for’ loop and using the ‘select’ construct for menu-driven scripts. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Traditional Looping | Simple, direct | Can be slow for large data |
‘seq’ with ‘for’ Loop | Flexible control over iteration | Can make scripts more complex |
‘select’ Construct | Creates interactive scripts | More prone to user error |
Whether you’re just starting out with Bash loops or you’re looking to level up your Bash scripting skills, we hope this guide has given you a deeper understanding of Bash loops and their capabilities.
The ability to use loops effectively is a powerful tool in Bash scripting. Now, you’re well equipped to harness the power of Bash loops in your scripts. Happy scripting!