Elif Statement in Python: A Complete Guide
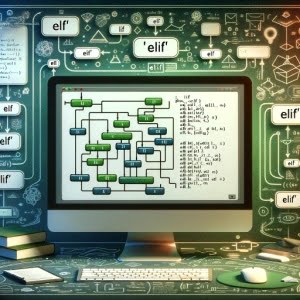
Are you finding yourself puzzled over the ‘elif’ statement in Python? Just like a traffic signal, ‘elif’ is a crucial component that helps control the flow of your Python code. It’s a bridge that connects different possibilities and outcomes within your code.
In this guide, we will dive deep into understanding and mastering the use of the ‘elif’ statement in Python. We will start from the basics, explaining the ‘elif’ statement, how it works, and then gradually move to more complex scenarios.
With practical examples, you will learn how to use ‘elif’ effectively to make your code more efficient and easier to understand. So, let’s get started!
TL;DR: What is the ‘elif’ statement in Python?
The ‘elif’ statement in Python is a part of the if-else structure, used for checking multiple conditions. It adds more flexibility to your code by allowing more than two possible outcomes.
Here’s a simple example:
x = 20
if x < 10:
print('Less than 10')
elif x < 20:
print('Between 10 and 20')
else:
print('20 or more')
# Output:
# '20 or more'
In this example, the ‘elif’ statement checks if ‘x’ is less than 20 after the ‘if’ condition has been evaluated as False. Since ‘x’ is 20, it doesn’t meet the ‘elif’ condition, so the program executes the code under the ‘else’ statement, printing ’20 or more’.
If you’re interested in a more detailed understanding of ‘elif’ in Python, including advanced usage scenarios, keep reading! We’re just getting started.
Table of Contents
Understanding the Elif Statement in Python
The ‘elif’ statement in Python is an essential part of the if-else structure, used for handling multiple conditions. It’s a combination of ‘else’ and ‘if’, which can be read as ‘else if’. It allows the program to check several conditions sequentially and execute a specific block of code as soon as a true condition is found.
Here’s a simple example:
x = 15
if x < 10:
print('x is less than 10')
elif x < 20:
print('x is between 10 and 20')
else:
print('x is 20 or more')
# Output:
# x is between 10 and 20
In this code, the ‘elif’ statement checks if ‘x’ is less than 20 after the ‘if’ condition has been evaluated as False. As ‘x’ is 15, it meets the ‘elif’ condition, so ‘x is between 10 and 20’ is printed.
Advantages of Using Elif
Elif statements provide greater flexibility to your code. They allow you to handle multiple conditions without having to nest multiple if-else structures, which can make your code complex and difficult to read.
Potential Pitfalls
One common mistake when using ‘elif’ is forgetting that the order of conditions matters. Python evaluates the conditions from top to bottom. Once it finds a condition that’s true, it executes the corresponding block of code and skips the rest. So, it’s important to ensure that your conditions are ordered correctly.
Another potential pitfall is not including an ‘else’ statement at the end. While it’s not always necessary, an ‘else’ statement can act as a catch-all for any conditions you haven’t explicitly handled.
Handling Complex Conditions with Elif
As you become more comfortable with Python, you’ll often encounter scenarios where you need to check more than just two or three conditions. That’s where multiple ‘elif’ statements come into play.
Multiple Elif Statements in Action
Let’s consider an example where we categorize a score into different grades:
score = 85
if score < 50:
grade = 'F'
elif score < 60:
grade = 'D'
elif score < 70:
grade = 'C'
elif score < 80:
grade = 'B'
else:
grade = 'A'
print('Grade:', grade)
# Output:
# Grade: B
In this example, we use multiple ‘elif’ statements to check the score against various ranges. The program evaluates each condition in order. Since the score is 85, it skips all the ‘elif’ conditions until it reaches the ‘else’ condition, and assigns ‘A’ to the grade.
Best Practices with Multiple Elif Statements
When using multiple ‘elif’ statements, remember to order your conditions from the narrowest to the broadest or from the lowest to the highest. Python will execute the first true condition it encounters, so the order is crucial to get the expected result.
Also, using an ‘else’ statement at the end is a good practice. It acts as a safety net, catching any conditions that aren’t explicitly handled by your ‘if’ and ‘elif’ statements.
Exploring Alternative Approaches to Elif
While the ‘elif’ statement is a powerful tool for handling multiple conditions, Python offers other approaches that can be more suitable in certain scenarios. Let’s explore some of these alternatives.
Nested If-Else Structures
One alternative is using nested if-else structures. This approach can be useful when you have conditions that depend on other conditions.
Here’s an example:
x = 5
y = 10
if x < 10:
if y < 10:
print('Both x and y are less than 10')
else:
print('x is less than 10, but y is not')
else:
print('x is not less than 10')
# Output:
# x is less than 10, but y is not
In this code, the inner if-else structure is only evaluated if ‘x’ is less than 10. While nested if-else structures can handle complex conditions, they can become difficult to read and maintain if overused.
Switch-Case Structures Using Dictionaries
Python doesn’t have a built-in switch-case structure like some other languages, but you can achieve similar functionality using dictionaries. This approach can be useful when you have a single condition that can have many possible values.
Here’s an example:
def switch_case(value):
return {
'a': 1,
'b': 2,
'c': 3,
}.get(value, 'Invalid value')
print(switch_case('a'))
print(switch_case('d'))
# Output:
# 1
# Invalid value
In this code, the dictionary keys act as the case conditions, and the values are the results. If the input doesn’t match any key, the ‘get’ method returns ‘Invalid value’. This approach is clean and efficient, but it’s limited to conditions that can be used as dictionary keys.
In conclusion, while ‘elif’ is a versatile tool for handling multiple conditions, it’s always good to know the alternatives. Depending on the scenario, a nested if-else structure or a dictionary-based switch-case might be a better choice. Always consider the nature of your conditions and the readability of your code when choosing the right approach.
Common Issues and Solutions with Elif
As you start using the ‘elif’ statement more frequently in Python, you might encounter some common issues. Let’s discuss these problems and their solutions to make your coding journey smoother.
Indentation Errors
Python uses indentation to define blocks of code. If your ‘elif’ statement or the code inside it is not indented correctly, Python will raise an IndentationError.
x = 5
if x < 3:
print('x is less than 3')
# Output:
# IndentationError: expected an indented block
In this example, the print statement is not indented, so Python raises an IndentationError. To fix this, make sure each statement inside your ‘if’, ‘elif’, and ‘else’ blocks is indented.
Logical Errors
Logical errors occur when your conditions don’t produce the expected result. This can often happen if your conditions in the ‘elif’ statements are not ordered correctly.
x = 5
if x < 10:
print('x is less than 10')
elif x < 3:
print('x is less than 3')
# Output:
# x is less than 10
In this example, even though ‘x’ is less than 3, the program prints ‘x is less than 10’. This is because the ‘elif’ condition is never checked once a true condition is found. To fix this, reorder your conditions from the most specific to the most general.
Remember, troubleshooting is a key part of programming. The more you understand the common issues and their solutions, the more efficient you’ll become at writing and debugging your code.
Python’s Control Flow: A Primer
To truly master the ‘elif’ statement in Python, it’s important to understand the fundamental concepts that underpin it. These include Python’s control flow statements and logical operators.
Control Flow Statements in Python
Control flow statements in Python dictate the order in which your code is executed. They include ‘if’, ‘elif’, and ‘else’ statements, which allow your program to make decisions based on certain conditions.
In an if-else structure, the ‘if’ statement checks a condition: if it’s true, the code inside the ‘if’ block is executed. If it’s false, the code inside the ‘else’ block is executed instead.
x = 5
if x < 3:
print('x is less than 3')
else:
print('x is not less than 3')
# Output:
# x is not less than 3
In this example, the ‘if’ condition is false, so the program skips the ‘if’ block and executes the ‘else’ block.
Logical Operators in Python
Logical operators in Python include ‘and’, ‘or’, and ‘not’. They allow you to combine or modify conditions.
- ‘and’: If both conditions are true, the ‘and’ operator returns True.
- ‘or’: If at least one condition is true, the ‘or’ operator returns True.
- ‘not’: The ‘not’ operator returns the opposite of the condition.
x = 5
if x > 3 and x < 10:
print('x is between 3 and 10')
# Output:
# x is between 3 and 10
In this example, both conditions are true, so the ‘and’ operator returns True, and the program prints ‘x is between 3 and 10’.
Understanding these fundamental concepts will help you write more complex and efficient code using the ‘elif’ statement in Python.
The Relevance of Elif in Larger Projects
The ‘elif’ statement, while simple, plays an instrumental role in larger scripts or projects in Python. Its ability to handle multiple conditions in a clean and efficient manner makes it a valuable tool for any Python developer.
Consider a large script where you need to process different types of data differently. Instead of writing multiple nested if-else structures, you can use ‘elif’ to check each data type and process it accordingly.
Exploring Related Concepts
While ‘elif’ is a powerful tool, Python offers many other control flow tools that are worth exploring. These include loops (like ‘for’ and ‘while’ loops), functions, and more complex control flow statements like ‘try’ and ‘except’.
For instance, combining ‘elif’ with a ‘for’ loop can allow you to process a list of items, each in a different way based on certain conditions.
values = [5, 20, 'hello', 30]
for value in values:
if isinstance(value, str):
print(f'{value} is a string')
elif value < 10:
print(f'{value} is less than 10')
else:
print(f'{value} is 10 or more')
# Output:
# 5 is less than 10
# 20 is 10 or more
# hello is a string
# 30 is 10 or more
In this example, the ‘elif’ statement combined with the ‘for’ loop allows us to process different types of values in the list.
To deepen your understanding of Python’s control flow, we recommend exploring these related concepts and practicing with real-world examples. Remember, practice is the key to mastering Python!
Further Resources for Python Conditionals
For those looking to strengthen their proficiency in Python conditional statements, we’ve curated a selection of resources packed with insights:
- Mastering Python If Statements – A Step-by-Step tutorial of Python’s if statements and control program flow.
Python’s “!=” Operator – Dive into the world of inequality testing and conditional logic.
Mastering “else if” in Python – Learn to create dynamic decision trees with “else if” in Python.
Python If-Else – JavaTpoint provides a detailed tutorial on using if-else conditionals in Python.
Python ‘if’ Keyword – W3Schools offers a reference guide explaining the use of the ‘if’ keyword in Python.
Python If-Else Statement – A FreeCodeCamp guide providing detailed explanations on the use of conditional “if-else” statements in Python.
By delving into these resources and sharpening your expertise in Python conditional statements, you can elevate your potential as a Python developer.
Wrapping Up: The Power of Elif in Python
In this comprehensive guide, we’ve explored the ‘elif’ statement in Python, a powerful tool that allows for cleaner, more efficient handling of multiple conditions in your code. We’ve seen its usage from basic to advanced levels, with practical code examples to illustrate each concept.
x = 15
if x < 10:
print('x is less than 10')
elif x < 20:
print('x is between 10 and 20')
else:
print('x is 20 or more')
# Output:
# x is between 10 and 20
Remember, the ‘elif’ statement is part of Python’s control flow tools, allowing your program to make decisions based on different conditions. However, it’s crucial to be aware of common issues, such as indentation and logical errors, to ensure your code runs as expected.
We also discussed alternative approaches to handle multiple conditions, like nested if-else structures and dictionary-based switch-case structures. Each method has its advantages and is suitable for different scenarios, so choose the one that best fits your needs.
In conclusion, mastering the ‘elif’ statement and its alternatives will enable you to write more complex and efficient Python code. Keep practicing, explore related concepts, and continue your journey in mastering Python!