Python ‘And’ Operator Usage Guide (With Examples)
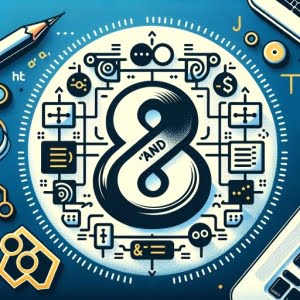
Are you finding it challenging to understand the ‘and’ operator in Python? You’re not alone. Many developers, especially beginners, often find themselves puzzled when it comes to using logical operators in Python.
Think of Python’s ‘and’ operator as a traffic cop at an intersection – it directs the flow of your code based on multiple conditions.
This guide will walk you through the process of using the ‘and’ operator in Python, from the basics to more advanced techniques. We’ll cover everything from how the ‘and’ operator works with boolean values, how it interacts with other logical operators like ‘or’ and ‘not’, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Use the ‘And’ Operator in Python?
The ‘and’ operator in Python is a logical operator that returns True if both the operands (values on the sides) are true. If not, it returns False.
Here’s a simple example:
x = 5
y = 10
print(x < 10 and y < 20)
# Output:
# True
In this example, we have two conditions: x < 10
and y < 20
. Both conditions are true (5 is less than 10
and 10 is less than 20
), so the ‘and’ operator returns True.
This is just the tip of the iceberg when it comes to using the ‘and’ operator in Python. Keep reading for more detailed usage and advanced scenarios.
Table of Contents
- Understanding the ‘And’ Operator in Python
- Combining ‘And’ with Other Logical Operators
- Exploring Alternative Methods to ‘And’ Operator
- Troubleshooting Common Issues with the ‘And’ Operator
- Python’s Logical Operators and Boolean Logic
- The ‘And’ Operator: Beyond the Basics
- Wrapping Up: Mastering the ‘And’ Operator in Python
Understanding the ‘And’ Operator in Python
In Python, the ‘and’ operator is a logical operator that takes two operands and returns True if both operands are true. If not, it returns False. This operator is often used in conditional statements to check multiple conditions.
Let’s break this down with a simple code example:
x = 5
y = 10
print(x < 10 and y < 20)
# Output:
# True
In this code snippet, we have two conditions: x < 10
and y < 20
. The ‘and’ operator checks both conditions. Since 5 is less than 10
and 10 is less than 20
, both conditions are true. Therefore, the ‘and’ operator returns True.
One of the advantages of the ‘and’ operator is its ability to simplify complex conditional statements. Instead of writing multiple if statements, you can combine conditions using the ‘and’ operator.
However, it’s important to remember that the ‘and’ operator in Python uses short-circuit evaluation. This means if the first operand is false, Python does not check the second operand because the overall result will be False regardless. This can lead to potential pitfalls if your code relies on checking both conditions.
For example:
x = 5
y = 10
print(x > 10 and y < 20)
# Output:
# False
In this example, the first condition x > 10
is false (5 is not greater than 10
), so Python does not check the second condition y < 20
. The ‘and’ operator returns False without evaluating the second condition. This is something to keep in mind when using the ‘and’ operator in your Python code.
Combining ‘And’ with Other Logical Operators
Python’s logical operators are not limited to ‘and’. You can also use ‘or’ and ‘not’ to create more complex conditions. Let’s explore how these operators work together.
Using ‘And’ and ‘Or’ Together
The ‘or’ operator in Python returns True if at least one of the operands is true. If both are false, it returns False. This operator can be combined with the ‘and’ operator to create more complex conditions.
Here’s an example:
x = 5
y = 10
z = 15
print((x < 10 and y < 20) or z < 10)
# Output:
# True
In this example, we have two conditions combined by the ‘and’ operator (x < 10 and y < 20)
, and one condition z < 10
. The ‘or’ operator checks if at least one of these conditions is true. Since the condition (x < 10 and y < 20)
is true, the ‘or’ operator returns True, even though z < 10
is false.
The ‘Not’ Operator
The ‘not’ operator in Python is used to reverse the logical state of its operand. If a condition is true, the ‘not’ operator will make it false, and vice versa.
Here’s an example of the ‘not’ operator in action:
x = 5
print(not x < 10)
# Output:
# False
In this example, x < 10
is true, but the ‘not’ operator reverses this, so the output is False.
In Python, you can combine ‘and’, ‘or’, and ‘not’ to create complex conditions that fit your needs. However, be mindful of the order of operations: ‘not’ is evaluated first, then ‘and’, and finally ‘or’. To override this order, you can use parentheses, as shown in the first example.
Exploring Alternative Methods to ‘And’ Operator
While the ‘and’ operator is efficient for evaluating multiple conditions, Python offers alternative methods that can achieve the same results. Two of these alternatives are nested if statements and the all() function.
Using Nested If Statements
Nested if statements are a method of checking multiple conditions sequentially. Here’s an example of how you can use nested if statements instead of the ‘and’ operator:
x = 5
y = 10
if x < 10:
if y < 20:
print(True)
else:
print(False)
else:
print(False)
# Output:
# True
In this example, the first if statement checks if x < 10
. If this condition is true, the nested if statement checks if y < 20
. If both conditions are true, the program prints True.
While nested if statements can replace the ‘and’ operator, they can make your code longer and harder to read, especially when dealing with numerous conditions.
The All() Function
The all() function is a built-in Python function that returns True if all items in an iterable are true. Otherwise, it returns False. Here’s how you can use the all() function instead of the ‘and’ operator:
x = 5
y = 10
print(all([x < 10, y < 20]))
# Output:
# True
In this example, the all() function checks if all conditions in the list [x < 10, y < 20]
are true. If they are, it returns True.
The all() function can be a powerful tool when dealing with a large number of conditions. However, it can be less readable than the ‘and’ operator, especially for beginners.
In conclusion, while the ‘and’ operator is a useful tool for checking multiple conditions, Python offers alternative methods, such as nested if statements and the all() function. Depending on your needs and the complexity of your conditions, these alternatives can sometimes be more suitable. However, the ‘and’ operator is often the most readable and efficient choice, especially for simple conditions.
Troubleshooting Common Issues with the ‘And’ Operator
While the ‘and’ operator is a fundamental part of Python, it can sometimes lead to unexpected results or confusion. Let’s discuss common issues you might encounter and how to resolve them.
Logical ‘And’ vs Bitwise ‘And’
In Python, there are two ‘and’ operators: the logical ‘and’ and the bitwise ‘and’. The logical ‘and’ is what we’ve been discussing so far. It checks if both conditions are true. On the other hand, the bitwise ‘and’ operates on bits and performs bit by bit operation.
Here’s an example of the bitwise ‘and’ operator:
x = 5 # binary: 101
y = 3 # binary: 011
print(x & y)
# Output:
# 1
In this example, the bitwise ‘and’ operator performs a binary AND operation on the binary representations of x
and y
. The result is 001
in binary, which is 1
in decimal.
It’s important to use the correct ‘and’ operator for your needs. If you’re dealing with boolean logic, use the logical ‘and’. If you’re working with binary data, use the bitwise ‘and’.
Short-Circuit Evaluation Pitfalls
As mentioned earlier, Python’s ‘and’ operator uses short-circuit evaluation. This means if the first operand is false, Python does not evaluate the second operand. While this can speed up your code, it can also lead to unexpected results if your second operand has side effects.
For example:
x = 5
y = print('Hello, World!')
print(x > 10 and y)
# Output:
# Nothing is printed
In this example, we expect ‘Hello, World!’ to be printed when we evaluate y
. However, since x > 10
is false, Python does not evaluate y
, so nothing is printed.
To avoid this pitfall, make sure any code with side effects is evaluated outside of your logical expressions.
While the ‘and’ operator in Python is straightforward, understanding its intricacies can help you avoid common pitfalls and write more efficient code.
Python’s Logical Operators and Boolean Logic
To fully grasp the ‘and’ operator, it’s crucial to understand Python’s logical operators and the concept of Boolean logic that underlies them.
Understanding Logical Operators
Python has three logical operators: ‘and’, ‘or’, and ‘not’.
- The ‘and’ operator returns True if both operands are true. Otherwise, it returns False.
- The ‘or’ operator returns True if at least one of the operands is true. If both are false, it returns False.
- The ‘not’ operator reverses the logical state of its operand. If a condition is true, the ‘not’ operator will make it false, and vice versa.
These operators are often used in conditional statements to check multiple conditions at once. They follow the order of operations: ‘not’ is evaluated first, then ‘and’, and finally ‘or’.
Boolean Logic: The Foundation of Logical Operators
Boolean logic, named after mathematician George Boole, is a subset of algebra used for creating true/false statements. Boolean logic forms the foundation of logical operators in Python.
In Boolean logic, an expression is either true or false. For example, the statement 5 is less than 10
is a Boolean expression that is True.
Boolean logic is essential for controlling the flow of programs. In Python, Boolean logic is used in conditional statements, loops, and functions.
Let’s see a simple example of Boolean logic with Python’s ‘and’ operator:
x = 5
y = 10
print(x < 10 and y < 20)
# Output:
# True
In this example, we have two Boolean expressions: x < 10
and y < 20
. The ‘and’ operator checks if both expressions are true. Since 5 is less than 10
and 10 is less than 20
, the ‘and’ operator returns True.
Understanding Python’s logical operators and Boolean logic is key to mastering the ‘and’ operator. With these concepts, you can create complex conditions, control the flow of your programs, and write more efficient Python code.
The ‘And’ Operator: Beyond the Basics
Once you’ve mastered the ‘and’ operator, you can leverage its power in various areas of Python programming. The ‘and’ operator plays a significant role in control flow and decision making in scripts, making it an indispensable tool for any Python programmer.
Control Flow with the ‘And’ Operator
In Python, control flow is the order in which the program’s code executes. The ‘and’ operator can influence this order by creating conditions that need to be met for certain code blocks to execute. For example, in an if statement, the code block will only run if the condition is true.
x = 5
y = 10
if x < 10 and y < 20:
print('Both conditions are true!')
# Output:
# 'Both conditions are true!'
In this example, the print statement only runs if both x < 10
and y < 20
are true. This is a simple demonstration of how the ‘and’ operator can control the flow of a Python program.
Decision Making in Scripts
Python scripts often need to make decisions based on certain conditions. The ‘and’ operator is an excellent tool for this, as it allows you to check multiple conditions at once.
x = 5
y = 10
if x < 10 and y < 20:
decision = 'Execute Plan A'
else:
decision = 'Execute Plan B'
print(decision)
# Output:
# 'Execute Plan A'
In this script, the decision to ‘Execute Plan A’ or ‘Execute Plan B’ depends on whether both conditions x < 10
and y < 20
are true. This is a simple but powerful way to make decisions in your Python scripts.
Exploring Related Concepts
Once you’re comfortable with the ‘and’ operator, you might want to explore related concepts. Other logical operators like ‘or’ and ‘not’, as well as conditional statements like if-else and switch, can further enhance your ability to write complex and efficient Python code.
Further Resources for Mastering Python Logical Operators
To deepen your understanding of Python’s logical operators, including the ‘and’ operator, here are some resources you might find helpful:
- IOFlood’s article, Navigating Python If Statements can help you create user-friendly Python programs with well-structured if-else blocks.
Python Boolean Variables – Discover conditional logic with boolean values in Python and understand their nature.
A Guide to the “not equal” Operator – Learn how to use the “not equal” operator (!=) in Python for comparing values.
Real Python’s Tutorial on Conditional Statements focuses on the use of conditional (if, elif, else) statements in Python.
GeeksforGeeks Guide on Python Operators covers different types of operators used in Python programming.
Python’s Official Documentation on Logical Operators provides information on the usage of boolean and logical operators.
These resources offer in-depth explanations and examples that can help you become more proficient in using Python’s logical operators.
Wrapping Up: Mastering the ‘And’ Operator in Python
In this comprehensive guide, we’ve delved into the ‘and’ operator in Python, a fundamental tool in crafting logical expressions and controlling the flow of your code.
We began with the basics, understanding how the ‘and’ operator works with boolean values and how to use it in simple conditions. We then explored more advanced uses, such as combining the ‘and’ operator with other logical operators like ‘or’ and ‘not’.
Along the way, we tackled common issues that you might encounter when using the ‘and’ operator, such as the difference between logical ‘and’ and bitwise ‘and’, and the pitfalls of short-circuit evaluation. We provided solutions and workarounds for each issue to help you write more efficient and error-free Python code.
We also discussed alternative approaches to the ‘and’ operator, such as nested if statements and the all() function. These alternatives can be useful when dealing with a large number of conditions or when you want to add complexity to your code.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
‘And’ Operator | Simple, Efficient | Pitfalls with Short-Circuit Evaluation |
Nested If Statements | Flexible, Can Handle Complex Conditions | Can Make Code Longer and Harder to Read |
All() Function | Can Handle Large Number of Conditions | Less Readable for Beginners |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of the ‘and’ operator and its usage in Python. Happy coding!