Python ‘Not Equal’ | Operator Usage Guide
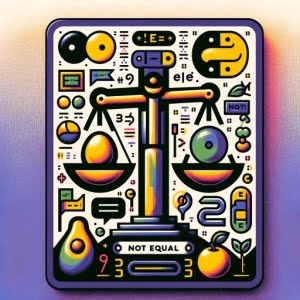
Whether you’re creating conditional statements or filtering data, understanding how to use the ‘not equal’ operator in Python can significantly streamline your coding process.
In this guide, we’ll walk you through the process of using the ‘not equal’ operator in Python, from the basics to more advanced techniques.
Let’s get started!
TL;DR: How Do I Use the ‘Not Equal’ Operator in Python?
In Python, the ‘not equal’ operator is ‘!=’. It compares two values, returning True if they are not equal and False if they are equal.
Here’s a simple example:
if 5 != 3:
print('5 is not equal to 3')
# Output:
# '5 is not equal to 3'
In this example, we’re using the ‘not equal’ operator to compare 5 and 3. Since these two numbers are not equal, the condition in the ‘if’ statement is True, and the print statement is executed, outputting ‘5 is not equal to 3’.
This is just the tip of the iceberg when it comes to using the ‘not equal’ operator in Python. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
‘Not Equal’ Operator: Basic Use
In Python, the ‘not equal’ operator is represented by ‘!=’. It’s a comparison operator used to compare two values. The operator returns True if the values are not equal and False if they are equal. This operator can be used with various data types in Python, including integers, floats, strings, and more.
Let’s take a look at a simple example:
if 'apple' != 'orange':
print('apple is not orange')
# Output:
# 'apple is not orange'
In this code snippet, we’re comparing two strings, ‘apple’ and ‘orange’. Since these two strings are not equal, the condition in the ‘if’ statement is True, and the print statement is executed.
The ‘not equal’ operator is a fundamental part of Python programming, and understanding how to use it effectively can help you write more efficient and readable code.
It’s important to understand that the ‘not equal’ operator can sometimes lead to unexpected results when used with different data types. For example, comparing a string and an integer will always return True, even if they represent the same value.
Here’s an example:
if '5' != 5:
print(''5' is not 5')
# Output:
# ''5' is not 5'
In this case, even though ‘5’ and 5 might seem equal, one is a string and the other is an integer. Python treats these as different types, so the ‘not equal’ operator returns True. This is an important pitfall to be aware of when working with the ‘not equal’ operator in Python.
Advanced Use
As you become more comfortable with the ‘not equal’ operator, you can start using it in more complex scenarios. One such example is within loops or conditional statements. Let’s take a look at how this works.
Not Equal in Loops
Suppose you have a list of fruits and you want to print out all the fruits that are not ‘banana’. You can use the ‘not equal’ operator in a loop to achieve this.
fruits = ['apple', 'banana', 'cherry', 'date', 'elderberry']
for fruit in fruits:
if fruit != 'banana':
print(fruit)
# Output:
# 'apple'
# 'cherry'
# 'date'
# 'elderberry'
In this example, the loop iterates over each fruit in the list. If the fruit is not ‘banana’, it gets printed. As a result, all fruits except ‘banana’ are printed.
Conditional Statements
You can also use the ‘not equal’ operator in conditional statements to execute specific blocks of code based on whether two values are not equal. Here’s an example:
age = 20
if age != 18:
print('You are not 18 years old.')
else:
print('You are 18 years old.')
# Output:
# 'You are not 18 years old.'
In this scenario, the ‘not equal’ operator checks if the variable age is not equal to 18. If it’s not equal (which is the case here), the first print statement is executed. If it were equal, the second print statement would be executed.
Alternatives
While the ‘not equal’ operator is a powerful tool for checking inequality in Python, it’s not the only method available. Python provides other ways to check for inequality, such as using the ‘is not’ operator or comparing the results of functions. Let’s explore these alternatives.
The ‘is not’ Operator
The ‘is not’ operator is used to compare the identity of two objects. It returns True if the two objects are not the same and False if they are. Here’s an example:
x = ['apple']
y = ['apple']
if x is not y:
print('x is not y')
# Output:
# 'x is not y'
In this case, even though x and y contain the same value, they are not the same object. Hence, the ‘is not’ operator returns True.
Comparing Function Results
You can also check for inequality by comparing the results of functions. Here’s an example:
def square(n):
return n ** 2
if square(3) != square(4):
print('The squares of 3 and 4 are not equal')
# Output:
# 'The squares of 3 and 4 are not equal'
In this scenario, we’re comparing the results of the square function with different arguments. Since the squares of 3 and 4 are not equal, the print statement is executed.
These alternatives have their own trade-offs. The ‘is not’ operator, for instance, checks for object identity rather than value equality, which might not be what you want in some cases.
Troubleshooting ‘Not Equal’
While the ‘not equal’ operator is a powerful tool in Python, it’s not without its quirks. There are common issues you may encounter when using it, such as type errors or logical errors. Let’s discuss some of these problems and how to resolve them.
Type Errors
One common issue is a TypeError, which occurs when you try to compare incompatible data types. For example, you might run into a problem when comparing a string and a list.
if 'apple' != ['apple']:
print('Mismatch')
# Output:
# 'Mismatch'
At first glance, you might expect a TypeError since we’re comparing a string to a list. However, Python allows this comparison and treats the string and list as not equal, thus printing ‘Mismatch’.
Logical Errors
Logical errors are harder to spot because they don’t cause your program to crash. Instead, they cause your program to behave incorrectly. For example, remember that the ‘not equal’ operator treats different data types as not equal, even if they represent the same value.
if '5' != 5:
print(''5' is not 5')
# Output:
# ''5' is not 5'
In this case, ‘5’ and 5 represent the same value but are different types (string and integer, respectively). This can lead to unexpected results if you’re not careful.
To avoid these issues, always ensure that the values you’re comparing are of the same data type. If necessary, use type conversion functions like int(), str(), or list() to convert values to the appropriate type before comparing them.
By understanding these common issues and how to resolve them, you can use the ‘not equal’ operator more effectively and write more robust Python code.
Equality and Inequality in Python: The Fundamentals
Before we delve deeper into the ‘not equal’ operator, let’s take a step back and understand the fundamental concepts of equality and inequality in Python.
Python provides several operators to compare values. These include the equality operator ‘‘ and the inequality operators ‘!=’ and ‘<>’, among others. These operators allow you to compare two values and make decisions based on their relationship.
Understanding Equality in Python
The equality operator ‘‘ compares the values on either side and returns True if they are equal and False otherwise. Here’s a simple example:
if 5 == 5:
print('5 is equal to 5')
# Output:
# '5 is equal to 5'
In this case, since 5 is equal to 5, the print statement is executed.
Understanding Inequality in Python
The inequality operator ‘!=’ works the opposite way. It compares the values on either side and returns True if they are not equal and False otherwise. Here’s an example:
if 5 != 3:
print('5 is not equal to 3')
# Output:
# '5 is not equal to 3'
In this scenario, since 5 is not equal to 3, the print statement is executed.
How Python Compares Different Data Types
When comparing different data types, Python follows certain rules. For instance, when comparing a string and a number, Python considers them not equal, even if they represent the same value. Here’s an example:
if '5' != 5:
print(''5' is not 5')
# Output:
# ''5' is not 5'
In this case, even though ‘5’ and 5 represent the same value, they are different types (string and integer, respectively), so Python treats them as not equal.
Understanding these fundamentals of equality and inequality in Python is crucial for using the ‘not equal’ operator effectively. It helps you write accurate conditional statements and avoid common pitfalls.
‘Not Equal’ Operator: Beyond Basic Python
The ‘not equal’ operator in Python is not just a tool for simple comparisons. Its relevance extends to larger scripts or projects, where it plays a crucial role in controlling the flow of execution and making decisions.
Logical Operators and Conditional Statements
The ‘not equal’ operator is often used in conjunction with other logical operators, such as ‘and’ and ‘or’, to create more complex conditions. For instance, you might want to check if a variable is not equal to two different values, which you can do with the ‘and’ operator.
x = 5
if x != 3 and x != 7:
print('x is not 3 and 7')
# Output:
# 'x is not 3 and 7'
In this case, since x is not equal to 3 and x is also not equal to 7, the print statement is executed.
Conditional statements like ‘if-else’ and ‘if-elif-else’ often make use of the ‘not equal’ operator to perform different actions based on various conditions.
Further Resources for Python Mastery
To deepen your understanding of the ‘not equal’ operator and related concepts, consider exploring the following resources:
- A Beginner’s Guide to Python If Statements – Dive deep into the world of Python’s if-elif-else statements for complex decision trees.
Using “and” in Python – Master the art of creating complex conditionals using “and” in Python’s control flow.
The elif Statement in Python – A detailed explanation on structuring Python code with “elif” for complex branching logic.
The official Python Documentation is a resource that covers all aspects of Python, including operators and control flow.
Real Python offers in-depth tutorials and articles on various Python topics, including operators and conditional statements.
Python for Beginners – This website provides easy-to-understand tutorials for beginners, covering basic and advanced concepts in Python.
Remember, mastering Python involves continuous learning and practice. Don’t be afraid to explore new concepts and push your boundaries.
Wrapping Up: Mastering the ‘Not Equal’ Operator in Python
In this comprehensive guide, we’ve delved into the depths of the ‘not equal’ operator in Python, exploring its uses, quirks, and alternatives.
We began with the basics, learning how to use the ‘not equal’ operator in simple comparisons. We then progressed to more advanced usage scenarios, such as using the operator in loops and conditional statements. Along the way, we tackled common challenges you might encounter when using the ‘not equal’ operator, such as type errors and logical errors, providing you with solutions and workarounds for each issue.
We also explored alternative approaches to checking for inequality in Python, including the ‘is not’ operator and comparing function results. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
‘Not Equal’ | Simple, works with different data types | Can lead to unexpected results with different data types |
‘Is Not’ | Checks for object identity | Might not be suitable for value comparisons |
Comparing Function Results | Flexible, can compare complex conditions | Can lead to code that’s harder to read if overused |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of the ‘not equal’ operator in Python and how to use it effectively. Happy coding!