Python Operators Guide | Usage, Syntax, Examples
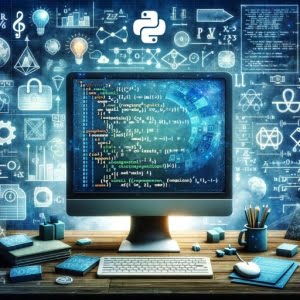
Are you finding Python operators puzzling? Don’t worry, you’re not alone. Python operators, like the symbols used by mathematicians for computations, might seem cryptic at first. But they are simply tools Python uses to perform operations
This comprehensive guide will take you from confusion to mastery of Python operators, covering everything from the basics to advanced usage. Whether you’re a beginner just starting out or an experienced programmer looking to brush up on your skills, this guide is for you.
TL;DR: What are Python Operators?
Operators in Python are special symbols that carry out arithmetic or logical computation. The value that the operator operates on is called the operand. Here’s a simple illustration:
x = 10
y = 5
print(x + y)
# Output:
# 15
In the example above, ‘+’ is the operator, while ‘x’ and ‘y’ are operands. The ‘+’ operator performs the addition operation on ‘x’ and ‘y’, resulting in the output ’15’.
This guide will delve deeper into the different types of Python operators and their uses, from the basic arithmetic operators to the more complex logical and bitwise operators. So, if you’re ready to master Python operators, read on!
Table of Contents
Python Arithmetic Operators: The Basics
Python arithmetic operators are the simplest type of operators in Python. They are used to perform basic mathematical operations such as addition (+), subtraction (-), multiplication (*), division (/), modulus (%), and exponentiation (**).
Let’s go through each of these operators with a simple code example:
# Addition
x = 5
y = 3
print(x + y) # Output: 8
# Subtraction
print(x - y) # Output: 2
# Multiplication
print(x * y) # Output: 15
# Division
print(x / y) # Output: 1.6666666666666667
# Modulus (remainder of the division)
print(x % y) # Output: 2
# Exponentiation (power)
print(x ** y) # Output: 125
The output of each operation is commented next to the print statements. These operators work just like they do in basic arithmetic. The modulus (%) operator might be new to you. It returns the remainder of the division of the two operands. For example, the remainder of 5 divided by 3 is 2. The exponentiation (**
) operator raises the first operand to the power of the second operand. For example, 5 raised to the power of 3 equals 125.
Exploring Python’s Comparison, Logical, and Bitwise Operators
As you progress in Python, you’ll encounter more complex operators. These include comparison operators, logical operators, and bitwise operators. These operators are crucial for decision making in Python scripts.
Python Comparison Operators
Comparison operators are used to compare two values. Here are the comparison operators in Python:
# Equal to
x = 5
y = 3
print(x == y) # Output: False
# Not equal to
print(x != y) # Output: True
# Greater than
print(x > y) # Output: True
# Less than
print(x < y) # Output: False
# Greater than or equal to
print(x >= y) # Output: True
# Less than or equal to
print(x <= y) # Output: False
These operators return either True or False depending on the validity of the comparison.
Python Logical Operators
Logical operators are used to combine conditional statements. Python has three logical operators: ‘and’, ‘or’, and ‘not’.
x = 5
# 'and' operator - Returns True if both statements are true
print(x > 3 and x < 10) # Output: True
# 'or' operator - Returns True if one of the statements is true
print(x > 3 or x < 4) # Output: True
# 'not' operator - Reverse the result, returns False if the result is true
print(not(x > 3 and x < 10)) # Output: False
Python Bitwise Operators
Bitwise operators act on operands as if they were strings of binary digits. They operate bit by bit.
x = 10 # binary: 1010
y = 4 # binary: 0100
# Bitwise AND
print(x & y) # Output: 0
# Bitwise OR
print(x | y) # Output: 14
# Bitwise NOT
print(~x) # Output: -11
# Bitwise XOR
print(x ^ y) # Output: 14
# Bitwise right shift
print(x >> 2) # Output: 2
# Bitwise left shift
print(x << 2) # Output: 40
Bitwise operators can be a bit tricky. They operate on the binary representations of values, and can be very useful in low-level programming.
Delving into Python’s Operator Module
Python’s operator module opens up a whole new world of functional programming. It provides a set of functions corresponding to the intrinsic operators of Python. For example, operator.add(x, y) is equivalent to the expression x + y.
Using the Operator Module
Here’s how you can use the operator module in Python:
import operator
x = 5
y = 3
# Addition
print(operator.add(x, y)) # Output: 8
# Subtraction
print(operator.sub(x, y)) # Output: 2
# Multiplication
print(operator.mul(x, y)) # Output: 15
# Division
print(operator.truediv(x, y)) # Output: 1.6666666666666667
As you can see, the operator module functions are equivalent to the Python arithmetic operators.
Benefits of the Operator Module
The operator module can make your code more readable and self-explanatory. This is particularly useful when you’re working with higher-order functions or when you’re manipulating data with tools like map() and filter().
Drawbacks and Considerations
The operator module can be a bit verbose compared to using the traditional operators. It’s also less commonly used, so it might make your code harder to understand for some Python developers.
When deciding whether to use the operator module or not, consider the complexity of your code, the performance implications, and the readability of your code. Always strive for writing code that is easy to read, understand, and maintain.
Python Operators: Common Pitfalls & Solutions
While Python operators are generally straightforward, you might encounter some common errors and obstacles. Let’s discuss these issues and how to resolve them.
Division by Zero
One of the most common errors in Python is division by zero. This happens when you try to divide any number by zero using the ‘/’ or ‘%’ operators. Python will raise a ZeroDivisionError
.
x = 10
y = 0
try:
print(x / y)
except ZeroDivisionError:
print('Error: Division by zero is not allowed')
# Output:
# Error: Division by zero is not allowed
In the above example, we’ve used a try/except block to catch the ZeroDivisionError
and print a friendly error message instead of crashing the program.
Operator Precedence
Another common issue is misunderstanding operator precedence. Python operators have a specific order of precedence, which determines the order in which operations are performed. For example, multiplication and division have higher precedence than addition and subtraction.
print(1 + 2 * 3) # Output: 7
In the above example, Python first performs the multiplication (2 * 3), then the addition (1 + 6), resulting in 7. To change the order of operations, you can use parentheses.
print((1 + 2) * 3) # Output: 9
In this case, Python first performs the operation inside the parentheses (1 + 2), then the multiplication (3 * 3), resulting in 9.
Bitwise Operators on Negative Numbers
Using bitwise operators on negative numbers can lead to unexpected results because Python uses a binary format called two’s complement for negative integers.
x = -10
print(~x) # Output: 9
In the above example, the bitwise NOT operator (~) returns 9 when applied to -10, which can be unexpected if you’re not familiar with two’s complement.
Remember, understanding Python operators and their peculiarities is key to writing efficient and bug-free code. Always test your code thoroughly and don’t hesitate to consult the Python documentation if you’re unsure about the behavior of an operator.
The Inner Workings of Python Operators
Understanding the fundamental concepts of operators is crucial in any programming language, and Python is no exception. Let’s delve deeper into how operators work in Python and in programming languages in general.
The Role of Operators in Programming
Operators in programming languages are the building blocks of most scripts. They allow us to perform operations on variables and values. The type of operation depends on the operator used. For example, the ‘+’ operator in Python performs addition, while the '=='
operator checks for equality.
x = 5
y = 3
# Addition
print(x + y) # Output: 8
# Equality check
print(x == y) # Output: False
In the example above, the ‘+’ operator adds the values of ‘x’ and ‘y’, while the ‘‘ operator checks if ‘x’ and ‘y’ are equal.
Operator Precedence and Associativity in Python
Operator precedence (also known as operator order) is a collection of rules that reflect conventions about which procedures to perform first in order to evaluate a given mathematical expression.
For example, in mathematics and most computer languages, multiplication is granted a higher precedence than addition. Therefore, in the expression 2 + 3 * 7, the multiplication is performed before the addition, resulting in an output of 23 rather than 35.
print(2 + 3 * 7) # Output: 23
Associativity is the rule that explains how an operator acts when it’s used with multiple operands. In Python, operators are usually left-associative, meaning they are grouped from the left. However, some operators like the exponentiation operator (**) are right-associative.
# Left-associative operators
print(100 - 10 - 5) # Output: 85
# Right-associative operators
print(2 ** 3 ** 2) # Output: 512 (same as 2 ** (3 ** 2))
In the first example, the subtraction is performed from left to right. In the second example, the exponentiation is performed from right to left.
Understanding the fundamentals of Python operators, including their roles, precedence, and associativity, can help you write more efficient and error-free code.
Python Operators in Real-World Scenarios
Python operators are not just theoretical concepts; they are practical tools used in a variety of real-world applications. Let’s explore how Python operators come into play in areas like data analysis and machine learning.
Data Analysis with Python Operators
In data analysis, Python operators are used to manipulate and analyze large datasets. For instance, arithmetic operators can be used to perform calculations on data, while comparison and logical operators can filter data based on specific conditions.
import pandas as pd
data = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
# Use arithmetic operators to perform calculations on data
print(data['A'] + data['B'])
# Output:
# 0 5
# 1 7
# 2 9
# dtype: int64
In the above example, we’ve used the ‘+’ operator to add the values of columns ‘A’ and ‘B’ in a pandas DataFrame.
Machine Learning with Python Operators
In machine learning, Python operators play a crucial role in creating and training models. For instance, arithmetic operators are used in the computation of loss functions and the optimization of model parameters.
Further Learning
Python operators are just the tip of the iceberg. To deepen your Python knowledge, consider exploring related topics such as Python data types, control structures (like loops and conditional statements), and Python’s extensive standard library. There are numerous online guides and resources that offer in-depth information on these topics.
Remember, mastering Python operators is a stepping stone to becoming proficient in Python. So, keep practicing and exploring, and soon you’ll find Python operators second nature.
Python Operators: A Quick Recap
We’ve covered a lot of ground in our exploration of Python operators. From basic arithmetic operators to more complex logical and bitwise operators, we’ve seen how these tools can manipulate data and control the flow of a Python program.
Here’s a quick summary of the different types of operators we discussed:
Operator Type | Description | Example |
---|---|---|
Arithmetic | Perform mathematical operations | x + y , x * y |
Comparison | Compare values and return True or False | x == y , x < y |
Logical | Combine conditional statements | x and y , x or y |
Bitwise | Operate on binary representations of integers | x & y , x >> 2 |
Remember, operators in Python are more than just symbols; they are powerful tools that can help you write efficient and effective code. Whether you’re performing simple calculations, making decisions based on conditions, or manipulating binary data, Python operators are up to the task.
Mastering Python operators is a crucial step in your journey to becoming a proficient Python programmer. Keep practicing, keep exploring, and don’t be afraid to dive deeper into the fascinating world of Python programming.