Python rstrip() Function Guide (With Examples)
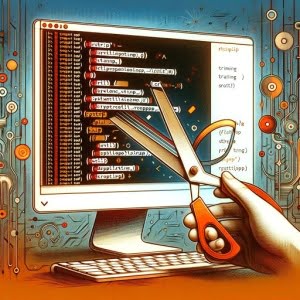
Are you grappling with unwanted characters at the end of your Python string? Imagine the rstrip() function as a skilled surgeon, adept at eliminating any pesky trailing characters.
This guide will help you navigate the usage of Python’s rstrip() function, starting from the basic usage to even more nuanced techniques. We’ll also discuss some troubleshooting scenarios and alternatives to rstrip() for more complex string manipulation tasks.
TL;DR: How Do I Use rstrip() in Python?
The rstrip() function in Python is used to remove trailing characters (spaces by default) from a string. Here’s a simple example:
text = 'Hello World '
text = text.rstrip()
print(text)
# Output:
# 'Hello World'
In this example, we’re using rstrip() to remove the trailing spaces from the string 'Hello World '
. The function is called on the string and the result is 'Hello World'
, with no trailing spaces.
This example shows the basic use of rstrip(), but there’s a lot more to it. Continue reading for a more detailed explanation and advanced uses of rstrip().
Table of Contents
Python’s rstrip(): The Basics
Python’s rstrip() is a built-in string method used for removing trailing characters from a string. The term ‘trailing’ refers to the characters at the end of a string. By default, rstrip() removes any trailing spaces if no specific characters are defined.
Let’s take a look at a simple example:
text = 'Hello World '
text = text.rstrip()
print(text)
# Output:
# 'Hello World'
In this example, the rstrip() function is called on the string 'Hello World '
, which has trailing spaces. The function removes these spaces, resulting in ‘Hello World’ without any trailing spaces.
The rstrip() function is particularly useful when you’re dealing with user input or data cleaning where trailing spaces or specific characters need to be removed for consistent data processing.
Note that rstrip() only removes characters from the end of a string. If you need to remove characters from the beginning or both ends of a string, you’ll need to use different Python string methods, such as lstrip() or strip().
Customizing rstrip(): Removing Specific Characters
Python’s rstrip() function is not limited to removing just trailing spaces. It can also remove specific characters provided as arguments. This functionality is particularly useful when dealing with trailing characters that aren’t spaces.
Take a look at the following example:
text = 'Hello World!!!'
text = text.rstrip('!')
print(text)
# Output:
# 'Hello World'
In this example, we’re calling rstrip() on the string ‘Hello World!!!’, which has trailing exclamation marks. We pass ‘!’ as an argument to rstrip(), instructing it to remove all trailing exclamation marks. The result is ‘Hello World’ without any trailing exclamation marks.
It’s important to note that rstrip() removes all instances of each character provided, not the entire sequence.
For example, if you call rstrip(‘ab’) on the string ‘Helloabba’, the result will be ‘Hello’, not ‘Helloab’. This is because rstrip(‘ab’) removes all trailing ‘a’s and ‘b’s, not the specific sequence ‘ab’.
text = 'Helloabba'
text = text.rstrip('ab')
print(text)
# Output:
# 'Hello'
In this case, rstrip(‘ab’) removed all trailing ‘a’s and ‘b’s, resulting in ‘Hello’. Remember, the order of the characters in the argument does not matter. rstrip(‘ab’) and rstrip(‘ba’) will give the same result.
This advanced use of rstrip() allows you to handle more complex string manipulation scenarios, making it a powerful tool in your Python toolkit.
Alternatives to rstrip()
While Python’s rstrip() is an effective tool for removing trailing characters, it’s not the only method available. There are other techniques such as string slicing and regular expressions that can also be used to achieve the same result. Let’s explore these alternatives.
Using String Slicing
Python’s string slicing can be used to remove specific trailing characters. Here’s an example:
text = 'Hello World!!!'
while text[-1] == '!':
text = text[:-1]
print(text)
# Output:
# 'Hello World'
In this example, we’re using a while loop to remove all trailing exclamation marks from the string ‘Hello World!!!’. The loop continues until the last character of the string is not an exclamation mark. This method provides more control but can be more complex and less readable than using rstrip().
Using Regular Expressions
Regular expressions (regex) can also be used to remove trailing characters, especially when dealing with more complex patterns. Python’s re module provides the tools to use regex. Here’s an example:
import re
text = 'Hello World!!!'
text = re.sub('!+$', '', text)
print(text)
# Output:
# 'Hello World'
Here, we’re using the re.sub function to replace all trailing exclamation marks with nothing. The pattern ‘!+$’ matches one or more exclamation marks at the end of the string. This method is powerful and flexible, but can be overkill for simple cases and harder to read for those not familiar with regex.
Comparison of Methods
Method | Advantages | Disadvantages |
---|---|---|
rstrip() | Simple, easy to read | Limited to trailing characters, removes all instances of each character |
Slicing | More control, can handle complex cases | More complex, less readable |
Regex | Very powerful, can handle complex patterns | Overkill for simple cases, less readable |
In conclusion, while rstrip() is a simple and effective method for removing trailing characters, alternatives like slicing and regex offer more control and flexibility, especially in more complex cases.
Troubleshooting rstrip(): Common Issues and Solutions
While Python’s rstrip() is a powerful tool for removing trailing characters, you might encounter some issues when using it. Let’s discuss some common challenges and their solutions.
Issue: rstrip() Not Removing Expected Characters
One common issue is that rstrip() might not remove the characters you expect. This usually happens when you’re trying to remove a sequence of characters, but rstrip() treats the argument as a set of characters to remove, not a sequence.
text = 'Helloabba'
text = text.rstrip('ab')
print(text)
# Output:
# 'Hello'
In this example, we’re trying to remove the trailing 'ab'
from 'Helloabba'
. But rstrip(‘ab’) removes all trailing 'a'
s and 'b'
s, not the specific sequence 'ab'
. The result is 'Hello'
, not 'Helloab'
as you might expect.
Solution: Using String Slicing or Regex
If you need to remove a specific sequence of characters, you can use string slicing or regex, as discussed in the previous section. For example, using regex:
import re
text = 'Helloabba'
text = re.sub('ab$', '', text)
print(text)
# Output:
# 'Helloab'
In this example, we’re using the re.sub function with the pattern 'ab$'
to match the specific sequence 'ab'
at the end of the string. The function replaces this sequence with nothing, resulting in 'Helloab'
.
Remember, understanding the behavior of rstrip() and how it treats its arguments is key to using it effectively. When in doubt, refer back to the basics and work your way up.
Understanding Python Strings and Manipulation
Before delving deeper into Python’s rstrip() function, it’s crucial to grasp the fundamentals of Python strings and how string manipulation works.
Python Strings: A Brief Overview
In Python, a string is a sequence of characters enclosed in single quotes, double quotes, or triple quotes. It’s one of Python’s built-in data types. For example:
text = 'Hello World'
print(text)
# Output:
# 'Hello World'
In this example, ‘Hello World’ is a string. Python treats each character in the string as a separate item that can be accessed via its index.
String Manipulation in Python
Python provides a variety of built-in methods for string manipulation, allowing you to perform operations like converting to uppercase or lowercase, replacing substrings, splitting strings, and more. One such method is rstrip().
text = 'Hello World '
text = text.rstrip()
print(text)
# Output:
# 'Hello World'
In this example, the rstrip() function is used to remove the trailing spaces from the string ‘Hello World ‘, resulting in ‘Hello World’.
Understanding Python’s string data type and the basics of string manipulation is key to mastering the use of rstrip() and other string methods. With this foundation, you can effectively manipulate strings in Python to suit your needs.
Further Resources for Python Strings
While rstrip() is a useful function, Python offers a wealth of other string methods and techniques. If you’re interested in diving deeper, you might want to explore related concepts like regular expressions for pattern matching and string formatting for creating formatted output. Here are a few resources to get you started:
- Practical Guide to Python Strings for Everyday Use: A practical guide that focuses on everyday use of Python strings, making them approachable and useful for routine programming tasks.
Using Python to Replace Characters in a String: Learn how to use Python’s built-in functions and string methods to replace characters in a string, with practical examples and code snippets.
Python String Contains: Checking if a String Contains a Substring: This article explains different methods to check if a string contains a substring in Python, including the use of in operator and the find() method.
Python rstrip() Method: A Comprehensive Guide: A comprehensive guide on w3schools.com that explains the rstrip() method in Python, used to remove specific characters from the end of a string.
Python String rstrip() Method: GeeksforGeeks provides information on the rstrip() method, which helps remove specified characters or whitespace from the right end of a string in Python.
Python String rstrip() Method: Tutorial and Examples: Programiz offers a tutorial with examples on how to use the rstrip() method in Python to remove trailing characters from a string.
Wrapping Up: Python’s rstrip() Function
In this guide, we’ve explored the Python rstrip() function in depth. This built-in function is a powerful tool for removing trailing characters from a string, whether they’re spaces or specific characters. We’ve seen how to use rstrip() in its simplest form, as well as how to customize it to remove specific trailing characters.
We’ve also addressed common issues you might encounter when using rstrip(), such as not removing the expected characters, and provided solutions and workarounds for these problems.
Beyond rstrip(), we’ve looked at alternative methods for removing trailing characters, including string slicing and regular expressions. These alternatives offer more control and flexibility, especially in more complex cases.
Comparison of Methods
Method | Advantages | Disadvantages |
---|---|---|
rstrip() | Simple, easy to read | Limited to trailing characters, removes all instances of each character |
Slicing | More control, can handle complex cases | More complex, less readable |
Regex | Very powerful, can handle complex patterns | Overkill for simple cases, less readable |
Whether you’re a beginner just starting out with Python or an intermediate developer looking to deepen your understanding, mastering rstrip() and other string methods is a valuable investment. Happy coding!