Git Checkout Commit | How to retrieve a specific previous commit
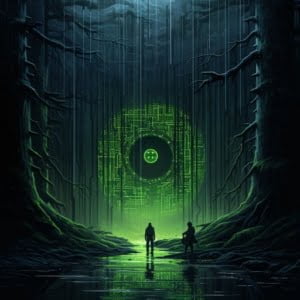
Navigating through the labyrinth of coding can sometimes lead you to a dead-end. At such moments, the ability to go back in time and revisit a previous version of your project can be a game-changer. This is where the knowledge of checking out a specific git commit ID comes in handy. In this guide, we’ll explore this process in detail, providing you with the skills to troubleshoot like a pro. So, let’s get started!
TL;DR: How do you checkout a specific git commit ID?
Clone the repository, fetch all changes, and get the commit ID. Then, checkout using the commit ID. If you need to make changes, create a new branch from the commit ID checkout. Always remember to backup your code before performing these steps.
Table of Contents
Preparing to Checkout a Git Commit
Before we dive into the intricacies of git checkout commit
, it’s crucial to take a moment for some digital housekeeping. When dealing with code, a good backup is your best friend. Just as you wouldn’t embark on a road trip without a spare tire, you should never perform git activities without a backup of your code.
But why is backing up your code so important, you ask?
Consider this: You’re working on a project and decide to checkout a previous git commit. But something goes wrong, and now your current project is in turmoil. If you had a backup, you could easily restore your project to its former state.
So, how do you back up your code? Regularly committing your work using git commit
is a good start. Additionally, consider using a cloud-based backup solution for an extra layer of protection.
Fetching all the latest changes and commits is also a key step. It’s akin to checking the weather before heading out – you want to ensure you’re up to date with any changes that might affect your journey.
Checking Out from a Specific Commit ID
Now, let’s talk about the git checkout command
. It’s a tool that lets you explore different versions of your project without disrupting the workflow. It’s your code’s time machine. With the git checkout command
, you can revisit an older version of your project, investigate, and then return to the present without messing up your current work.
Getting the Commit ID
With preparations done, let’s delve into the process of git checkout a specific commit
. The first step is to get the commit ID, also known as the SHA. This can be found in your local repository or on platforms like Github. It’s the address of the commit, guiding git to your desired destination.
To get your commit ID or SHA, open your Terminal, navigate to your local repository and type git log
. This will display a list of commits with their respective SHAs. The SHA of each commit is the long string of numbers and letters that appears next to “commit” in the log.
Alternatively, if you are using GitHub, you can find the commit ID by visiting the repository webpage, selecting “commit” from the repository menu. This will bring up the commit history. Each commit displays a unique, truncated version of the SHA. To view the entire SHA, simply click on the truncated SHA. The full SHA will then be displayed in the browser’s address bar.
In both methods, just copy and keep the SHA of the commit you are interested in. It will be used to checkout to that specific commit.
Using the Commit ID
The next step involves checking out using the commit ID. This is where you instruct git to take you to this specific commit. It’s like saying, “Git, transport me to this particular point in time in my project.”
To checkout to a specific commit using its commit ID, open your terminal and make sure you are in the root directory of your local repository.
Now, use the git checkout command with the commit’s SHA. The syntax of the command is git checkout [commit_id]
, where [commit_id]
is the SHA you copied earlier.
For example, if your commit ID is a9c1486e8b05cb833a52fcebc379edc9c54be67b
, you would use git checkout a9c1486e8b05cb833a52fcebc379edc9c54be67b
.
Hit enter after typing the command.
Detached Head
Git will then checkout to this specific commit, and you will see a note in the terminal indicating that you’re in ‘detached HEAD’ state. This means you are no longer on any branch, and any changes made won’t be tracked. If you want to make changes and save them, you should create a new branch while you are in this state by using git checkout -b [branch_name]
.
Shortcuts and Identifiers
But there’s more! Did you know you can use the short form of the SHA ID when checking out a commit? It’s a nifty shortcut that saves you some typing. Also, Git creates a unique identifier for each commit. This enables you to easily view or revert to a specific save point in your code, providing a robust mechanism for version control.
Git does a great job of letting you reference commits using a short version of the SHA ID, usually the first 7 characters are enough to uniquely identify a commit.
In the earlier example, for the SHA a9c1486e8b05cb833a52fcebc379edc9c54be67b
, you would use git checkout a9c1486
to checkout to that specific commit. Git will automatically recognize which commit you’re referencing.
Creating a Branch for Changes
After checking out a specific commit, you might want to make some modifications. But before you start experimenting, it’s crucial to create a branch from this commit. When you’re in ‘detached HEAD’ mode, any changes you make aren’t associated with a branch. So, if you checkout another commit or branch, your changes will vanish.
Creating a new branch from the checked-out commit provides a safe haven for your changes. This new branch retains everything from the commit, allowing you to make changes without fear of losing them. It’s like a secure playground where you can explore different ideas without impacting the rest of your project.
To create a new branch from the checked-out commit, follow these steps:
- Assuming you’re already checked out to your desired commit, we will create a new branch from here. In your terminal, type
git checkout -b [branch_name]
. Replace[branch_name]
with your intended new branch’s name. For instance,git checkout -b experiment_branch
. When you hit enter, git will create a new branch named ‘experiment_branch’ (or whatever name you chose) starting at the current commit and switch to it immediately.
Now, you can start making changes to your code, and these changes will only affect the new branch, leaving your other branches unchanged. You can add changes to the staging area using
git add .
orgit add [file_name]
, and then commit them usinggit commit -m "[Your_Message]"
.Once you are satisfied with your changes, you can merge this branch back into your main branch (usually master or main). Make sure you have checked out to the branch you want to merge into then run
git merge experiment_branch
. This will bring in all the new changes you made on your experimental branch into your main branch.
Always remember, working with branches allows for safe experimentation and less risk of conflicts or accidental overwrites.
Cloning from a Specific Commit ID
Occasionally, you might want to clone a repository from a specific commit. However, Git doesn’t provide a direct way to do this. But don’t worry! There’s a workaround. After cloning the repository, you can perform a hard reset with the commit SHA ID. This effectively makes the current HEAD point to the specific commit ID, giving you a clone from that commit.
If you want to clone a repository from a specific commit, follow these steps:
- First, you need to clone the repository. Open your terminal and use the
git clone
command followed by the URL of the repository. For example:git clone https://github.com/username/repo.git
. Change your directory to the cloned repository using the
cd
command. For example:cd repo
.Now, find the commit SHA ID you wish to clone from. Use the
git log
command to find this. It will display a reverse chronological list of commits with their respective SHA IDs.Copy the SHA ID of the commit you wish to clone from. Then, use the
git reset --hard [commit_id]
command to perform a hard reset of the repository to this commit. Replace[commit_id]
with the SHA ID you copied.
When you execute the git reset --hard [commit_id]
command, it changes the HEAD, index and working directory to match the files of the specified commit. Any changes made to tracked files in the working tree since that commit are discarded.
Now, your repository clone is in the state of the specific commit you wanted to clone from.
This workaround may seem a bit indirect, but it’s actually quite efficient. By first cloning the repository and then performing a hard reset, you achieve the same result as if you had cloned from a specific commit. It’s like taking a detour on your journey, but still ending up at your desired destination.
Resetting Git HEAD to a Specific Commit ID
Having checked out and cloned from a specific commit, let’s discuss resetting the Git HEAD to a specific commit. This can be done with a hard reset after pulling changes. It’s like retracing your steps and starting again from a specific point.
But why would you want to reset the Git HEAD? Suppose you’ve made some changes that didn’t work out, and you want to revert to a previous version of your project. By resetting the Git HEAD to a specific commit, you can easily go back to that version.
To reset the Git HEAD to a specific commit, follow these steps:
- First, you would need to pull the latest changes from your repository, you can do this by using
git pull
orgit pull origin [branch_name]
. In your terminal, run the
git log
command, which will show you a history of your commits.Find the commit hash (commit ID) that you want to revert back to. This hash is a long string of numbers and letters linked to each specific commit.
Once you have the desired commit hash, type
git reset --hard [commit_hash]
. Replace[commit_hash]
with the hash that you copied. For example, if your commit hash isd4m8s3h5
, you would usegit reset --hard d4m8s3h5
.Hit enter. Git will then reset the HEAD, index, and working directory to the state of the specified commit.
Please note that git reset --hard [commit_hash]
will permanently undo all commits and changes that came after the specified commit. This is a destructive action and should be used with caution. Make sure to backup any changes that you don’t want to lose or consider using git reset --soft [commit_hash]
that undoes commits but keeps the changes in your working directory.
Summarizing the Process
We’ve covered a lot of ground! From preparing for a git checkout commit
, to checking out from a specific commit ID, creating a branch for making changes, cloning from a specific commit, and resetting the Git HEAD, we’ve delved into the various aspects of working with specific git commit IDs.
The ability to checkout a specific git commit ID is a priceless tool in your developer’s toolkit. It enables you to seamlessly navigate through different versions of your project, troubleshoot issues, and explore new ideas without jeopardizing your current progress. It’s akin to having a time machine for your code – allowing you to revisit the past, make changes, and then return to the present.
In conclusion, mastering the git checkout commit
process equips you with the ability to navigate through your project’s history, troubleshoot issues, and manage different project versions. It’s like having a roadmap to your project’s past, enabling you to revisit, explore, and learn from it. So, go ahead and checkout that specific git commit ID. Happy coding!
This comprehensive guide, should equip you with a detailed understanding of how to checkout a specific git commit ID. By adhering to the guidelines outlined here, you should be able to navigate your way through your project’s history, manage different versions, and troubleshoot issues with ease. Remember, the journey of coding is a continuous learning process, so keep exploring, keep learning, and keep coding!