How To Create an Empty Dataframe: Python Pandas Guide
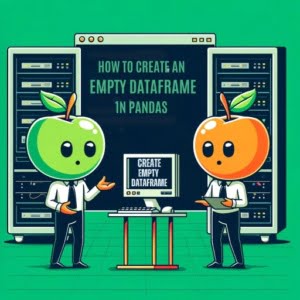
Initializing and working with empty data structures is a common task in data analysis workflows on our servers at IOFLOOD. In Python, creating an empty DataFrame using pandas is straightforward and useful for various data manipulation tasks. Join us as we explore how to create an empty DataFrame in Python, providing step-by-step guidance and practical examples for our dedicated server customers and other developers.
This guide will walk you through the process, from the basics to more advanced techniques, ensuring you have a solid foundation in this essential skill.
So, let’s dive right in and start setting up your data canvas!
TL;DR: How Do I Create an Empty DataFrame in Python?
The quickest way to create an empty DataFrame in Python is by using the
pandas library
and the syntax,dataframe = pd.DataFrame()
.
Here’s a quick example:
import pandas as pd
df = pd.DataFrame()
print(df)
# Output:
# Empty DataFrame
# Columns: []
# Index: []
In the above example, we first import the pandas library, which provides us with the DataFrame() function. We then call this function and assign it to the variable ‘df’. When we print ‘df’, we see that it is indeed an empty DataFrame with no columns or indices.
If you’re interested in learning more about creating empty DataFrames, including more advanced techniques, keep reading! We’ll be diving into all the nuances to give you a comprehensive understanding.
Table of Contents
Basics of DataFrame Creation
In Python, pandas is a powerful library that provides high-performance, easy-to-use data structures and data analysis tools. One such data structure is the DataFrame.
A DataFrame is a two-dimensional labeled data structure with columns potentially of different types. You can think of it like a spreadsheet or SQL table, or a dictionary of Series objects.
Creating an empty DataFrame can be essential for many tasks, such as setting up a structure to hold data that will be loaded or calculated later.
Let’s take a look at how to create an empty DataFrame using pandas:
import pandas as pd
df = pd.DataFrame()
print(df)
# Output:
# Empty DataFrame
# Columns: []
# Index: []
In this example, we first import the pandas library. We then create an empty DataFrame by calling the DataFrame() function from the pandas library and assigning it to the variable ‘df’. When we print ‘df’, we see an empty DataFrame with no columns or indices.
Advantages and Potential Pitfalls
The main advantage of this method is its simplicity. It’s a straightforward way to create a DataFrame when you don’t need to initialize any data.
However, there are potential pitfalls. One is that you might forget to populate the DataFrame with data later on, leading to errors. Another is that you might attempt to perform operations on the empty DataFrame that require data, which will also lead to errors.
It’s crucial to understand when and why you’re creating an empty DataFrame to avoid these issues.
Predefining DataFrame Structure
As you progress in your Python journey, you’ll find that sometimes, you need more control over your DataFrame structure.
This is where predefined columns or indices come into play. Even when creating an empty DataFrame, you can define its structure by specifying the columns or index.
Here’s an example of how to create an empty DataFrame with predefined columns:
import pandas as pd
columns = ['Name', 'Age', 'Gender']
df = pd.DataFrame(columns=columns)
print(df)
# Output:
# Empty DataFrame
# Columns: [Name, Age, Gender]
# Index: []
In the above code, we first define a list of column names. We then create an empty DataFrame, but this time, we pass our list to the ‘columns’ parameter of the DataFrame() function. The resulting DataFrame has the columns ‘Name’, ‘Age’, and ‘Gender’, but no data.
Advantages and Potential Pitfalls
The advantage of this method is that it gives you more control over the structure of your DataFrame from the outset. This can be useful when you know the structure of the data you’ll be working with, even if you don’t have the data yet.
The potential pitfall is that you might define columns or an index that you end up not using, which can lead to unnecessary memory usage. Moreover, if you define columns and then try to add data that doesn’t match these columns, you’ll run into errors.
As with the basic use, it’s important to understand your data and your needs to make the most of this method.
Other Ways to Make a DataFrame
Beyond the basic and intermediate techniques, there are alternative methods to create an empty DataFrame in Python.
One such approach is using the numpy library. Numpy is a powerful library for numerical operations, and it can be used in conjunction with pandas to create DataFrames.
Let’s take a look at an example:
import pandas as pd
import numpy as np
df = pd.DataFrame(np.empty(0, dtype=[('Name', 'str'), ('Age', 'int'), ('Gender', 'str')]))
print(df)
# Output:
# Empty DataFrame
# Columns: [Name, Age, Gender]
# Index: []
In the above code, we first import the pandas and numpy libraries. We then create an empty DataFrame using the numpy function ’empty’.
This function returns a new array of given shape and type, without initializing entries. We define the structure of the array with a dtype parameter, which is a list of tuples. Each tuple represents a column and its type.
The resulting DataFrame has the columns ‘Name’, ‘Age’, and ‘Gender’, but no data.
Advantages and Disadvantages
The advantage of this method is that it allows for more complex DataFrame structures. This can be useful when working with more complex data or when you need to create a DataFrame with a specific structure.
The disadvantage is that it requires a deeper understanding of numpy and pandas, and it can be more complex to debug if something goes wrong. Moreover, as with the previous methods, it’s essential to understand your data and your needs to make the most of this method.
Solving Issues in DataFrame Creation
Creating an empty DataFrame in Python is generally straightforward, but like any coding task, it can sometimes lead to unexpected issues. Let’s discuss some of the common problems you might encounter and how to solve them.
Handling Import Errors
One of the most common issues you might face is an import error. This typically occurs when the pandas library is not installed or not correctly installed in your Python environment. Here’s what the error might look like:
import pandas as pd
# Output:
# ImportError: No module named pandas
If you encounter this error, the solution is to install pandas. You can do this using the pip Python package installer. Open your terminal and type the following command:
pip install pandas
Dealing with Pandas Version Issues
Another common issue is related to the version of pandas you’re using. Some functions might not work as expected or at all if you’re using an older version of pandas. To check your pandas version, you can use the following command:
import pandas as pd
print(pd.__version__)
# Output:
# '1.3.3' # for example
If you’re using an older version of pandas and experiencing issues, you might need to update it. You can do this using pip:
pip install --upgrade pandas
Remember, troubleshooting is a normal part of the coding process. Don’t be discouraged if you encounter issues. With a bit of patience and persistence, you’ll be able to create empty DataFrames with ease.
DataFrames and Their Importance
Before we delve further into creating empty DataFrames, it’s crucial to understand what a DataFrame is and why you might want to create one.
In Python, a DataFrame is a data structure provided by the pandas library. It’s a two-dimensional labeled data structure, much like a table in a relational database, or an Excel spreadsheet with rows and columns.
Columns
Each column in a DataFrame can hold items of the same data type, but different columns can hold different types, such as integers, strings, or floats.
import pandas as pd
data = {'Name': ['John', 'Anna', 'Peter'], 'Age': [28, 24, 35]}
df = pd.DataFrame(data)
print(df)
# Output:
# Name Age
# 0 John 28
# 1 Anna 24
# 2 Peter 35
In the above example, we create a DataFrame from a dictionary. The keys of the dictionary (‘Name’ and ‘Age’) become the column names, and the values become the data in these columns.
Why would you want to create an empty DataFrame?
There are several reasons. One of the most common is when you’re preparing to load data into it. For instance, you might be writing a program that fetches data from an API or a database. You can create an empty DataFrame first, and then fill it with the fetched data.
Series
Another related concept is the Series, another core data structure in pandas.
A Series is like a single column in a DataFrame. It’s a one-dimensional array-like object that can hold any data type. A DataFrame is essentially a collection of Series that share a common index.
import pandas as pd
s = pd.Series(['John', 'Anna', 'Peter'], name='Name')
print(s)
# Output:
# 0 John
# 1 Anna
# 2 Peter
# Name: Name, dtype: object
In the above example, we create a Series with the data ['John', 'Anna', 'Peter']
and the name 'Name'
. When we print the Series, we see that it has an index on the left and the data on the right.
Understanding these fundamentals is key to working effectively with pandas and Python for data analysis.
Wider Applications of DataFrames
Creating an empty DataFrame is not just a coding exercise, it has real-world implications in the fields of data analysis, machine learning, and more. In data analysis, empty DataFrames are often used as a starting point for data loading and manipulation. In machine learning applications, they can be used to structure the results of model predictions, among other uses.
# An example of using empty DataFrame in machine learning
import pandas as pd
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
# Load iris dataset
iris = load_iris()
X = iris.data
y = iris.target
# Split the dataset
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create a random forest classifier
clf = RandomForestClassifier(n_estimators=100, random_state=42)
clf.fit(X_train, y_train)
# Make predictions
predictions = clf.predict(X_test)
# Create an empty DataFrame to store the predictions
df_predictions = pd.DataFrame()
df_predictions['Predictions'] = predictions
print(df_predictions.head())
# Output:
# Predictions
# 0 1
# 1 0
# 2 2
# 3 1
# 4 1
In the above example, we first load the iris dataset and split it into training and testing sets. We then create a random forest classifier and use it to make predictions on the testing set. We create an empty DataFrame and add our predictions to it, providing a structured way to view and analyze our model’s predictions.
Further Resources for Pandas Library
Beyond creating empty DataFrames, there’s a wealth of related concepts and techniques in pandas you might find useful, such as data manipulation functions like merge
, concat
, pivot
, and many more.
To continue your journey in mastering pandas and Python, consider exploring these concepts and seeking out resources for deeper understanding. Python’s official documentation, pandas’ user guide, and various online coding platforms offer in-depth tutorials and exercises that can help you expand your skills.
Here are a few resources from our blog that you might find helpful:
- Data Analysis with Pandas: A guide tailored for beginners learning data analysis with Pandas.
Introduction to Pandas DataFrame provides an overview of how to create and manipulate data in a Pandas DataFrame.
Merging DataFrames in Pandas: This tutorial explores how to use the merge() function in Pandas.
pandas.DataFrame.empty – pandas API Reference: The official pandas documentation explaining how to check if a DataFrame is empty.
How to Create an Empty DataFrame demonstrates different techniques to create an empty DataFrame.
Pandas Empty DataFrame: Creation and Handling: A blog post that covers the creation and handling of empty DataFrames in Pandas, providing examples and explanations.
Final Thoughts: Empty DataFrames
In this comprehensive guide, we’ve journeyed through the process of creating an empty DataFrame in Python, a fundamental task in data analysis and machine learning.
We started with the basics, using the pandas library’s DataFrame() function. We then explored more advanced techniques, such as predefining the structure of our DataFrame with columns or indices. We also discussed alternative approaches, like using the numpy library to create more complex DataFrame structures.
Throughout this journey, we’ve encountered some common issues, such as import errors and version-related problems. We’ve learned how to troubleshoot these issues, ensuring a smooth DataFrame creation process.
As you continue your journey, remember to explore beyond the creation of empty DataFrames. The pandas library offers a wealth of functions and techniques for data manipulation, and mastering these will equip you with the tools to tackle real-world data analysis tasks.