Python Terminal Commands: Reference Guide
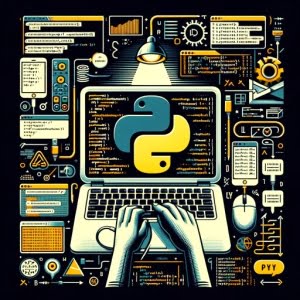
Ever felt like you’re lost in the wilderness when trying to navigate the world of Python through your terminal? You’re not alone. Many developers find the terminal intimidating, but it’s actually a powerful tool that can help you interact with Python in a more direct and efficient way.
Think of Python terminal commands as your compass and map in this wilderness.
In this guide, we’ll equip you with the knowledge to use Python terminal commands, from the basics to more advanced operations. They can help you traverse and manipulate your Python environment, allowing you to execute scripts, manage packages, and even debug your code directly from the terminal.
So, let’s dive in and start mastering Python terminal commands!
TL;DR: What are some basic Python terminal commands and how do I use them?
Python terminal commands are a set of instructions that allow you to interact with your Python environment directly from your terminal. They enable you to execute Python scripts, manage Python packages, and much more. Here’s a simple example of running a Python script:
python3 my_script.py
# Output:
# [Expected output from my_script.py]
In this example, we use the python3
command followed by the name of the script to execute it. This is one of the most basic Python terminal commands, but there’s a whole world of commands out there waiting to be discovered.
If you’re interested in learning more about Python terminal commands and how they can make your life as a developer easier, keep reading for more detailed information and advanced usage scenarios.
Table of Contents
- Python Terminal Commands for Beginners
- Delving Deeper: Intermediate Python Terminal Commands
- Alternative Interactions with Python
- Troubleshooting Python Terminal Commands
- Understanding the Terminal and Python’s Interaction
- Python Terminal Commands in Larger Projects
- Wrapping Up: Mastering Python Terminal Commands
Python Terminal Commands for Beginners
Executing Python Scripts
The most basic use of Python terminal commands is to execute a Python script. Here’s how you do it:
python3 my_script.py
# Output:
# [Expected output from my_script.py]
In this example, python3
is the command to run the Python interpreter, and my_script.py
is the name of the Python script you want to execute. The output will be whatever your script is programmed to print to the console.
Using Python’s Interactive Mode
Python’s interactive mode is a great way to experiment with Python code. You can enter Python commands one at a time and see the results immediately. Here’s how you enter Python’s interactive mode:
python3
This command opens the Python interpreter in your terminal. You can now enter Python commands directly into the terminal. For example:
print('Hello, world!')
# Output:
# Hello, world!
Managing Python Packages with pip
Python’s package manager, pip, is a powerful tool for managing Python packages. Here’s an example of how to install a package with pip:
pip install requests
This command installs the requests
package, which you can now import and use in your Python scripts. To uninstall a package, you can use the pip uninstall
command:
pip uninstall requests
This command removes the requests
package from your Python environment.
These are just the basics of Python terminal commands, but they can already significantly streamline your workflow. In the next section, we’ll look at some more advanced commands.
Delving Deeper: Intermediate Python Terminal Commands
As you become more comfortable with the basics, you can start exploring some of the more advanced Python terminal commands that can make your development process even more efficient.
Debugging with pdb
Python’s built-in debugger, pdb, is a powerful tool for finding and fixing bugs in your code. Here’s an example of how to use it:
import pdb
def buggy_function(x, y):
pdb.set_trace()
return x / y
buggy_function(5, 0)
When you run this script, it will pause at the line where pdb.set_trace()
is called. You can then inspect the values of variables, step through the code one line at a time, and do much more.
Profiling Python Scripts
Profiling is a technique used to measure the performance of your code. Python provides a built-in module, cProfile, for this purpose. Here’s an example of how to use it:
import cProfile
def my_function():
return sum([i * 2 for i in range(10000)])
cProfile.run('my_function()')
# Output:
# [Profiling results]
This script will output a table of profiling results, showing you how much time is spent on each function call.
Setting Environment Variables
Environment variables are a way to configure your Python environment. You can set them using the export
command in the terminal. For example:
export MY_VARIABLE='Hello, world!'
You can now access MY_VARIABLE
in your Python scripts like this:
import os
print(os.environ['MY_VARIABLE'])
# Output:
# Hello, world!
These advanced Python terminal commands can unlock new ways of interacting with Python and boost your productivity as a developer.
Alternative Interactions with Python
While mastering Python terminal commands is incredibly useful, it’s important to note that there are alternative ways to interact with Python, particularly through Integrated Development Environments (IDEs) and Jupyter notebooks.
Python IDEs: PyCharm
IDEs like PyCharm provide a comprehensive set of tools for Python development in a single application. They offer features such as syntax highlighting, code completion, and debugging tools. Here’s an example of running a Python script in PyCharm:
# This is a PyCharm example script
print('Hello, PyCharm!')
# Output:
# Hello, PyCharm!
You can simply click the ‘Run’ button in PyCharm to execute this script, and the output will be displayed in PyCharm’s built-in terminal. However, IDEs can be resource-intensive and might be overkill for simple scripts or tasks.
Jupyter Notebooks
Jupyter notebooks are an interactive computing environment that enables users to create and share documents that contain live code, equations, visualizations, and narrative text. Here’s an example of running a Python script in a Jupyter notebook:
# This is a Jupyter notebook example script
print('Hello, Jupyter!')
# Output:
# Hello, Jupyter!
You can simply click the ‘Run’ button in the Jupyter notebook to execute this cell, and the output will be displayed directly below the cell. Jupyter notebooks are particularly useful for data analysis and visualization, but they might not be suitable for developing larger applications.
In conclusion, while Python terminal commands offer a direct and powerful way to interact with Python, IDEs and Jupyter notebooks provide alternative approaches that might be more suitable depending on your specific needs and circumstances.
Troubleshooting Python Terminal Commands
Even with a good understanding of Python terminal commands, you might occasionally run into issues. Let’s discuss some common problems and their solutions.
Syntax Errors
Syntax errors occur when Python can’t understand your code due to incorrect syntax. For example, forgetting to close a string with a quotation mark will result in a syntax error:
print('Hello, world)
# Output:
# SyntaxError: EOL while scanning string literal
To fix this, make sure all your strings are properly closed:
print('Hello, world!')
# Output:
# Hello, world!
Module Not Found Errors
If Python can’t find a module you’re trying to import, it will raise a ModuleNotFoundError
:
import non_existent_module
# Output:
# ModuleNotFoundError: No module named 'non_existent_module'
To fix this, make sure the module you’re trying to import is installed in your Python environment. If it’s a custom module, make sure it’s in the same directory as your script or included in your PYTHONPATH
.
Python Version Issues
Different versions of Python can behave slightly differently, and code that works in one version might not work in another. For example, the print
statement from Python 2 doesn’t work in Python 3:
print 'Hello, world!'
# Output:
# SyntaxError: Missing parentheses in call to 'print'. Did you mean print('Hello, world!')?
To fix this, make sure you’re using the correct version of Python for your code. You can check your Python version with the following command:
python --version
# Output:
# Python 3.8.5
By understanding these common issues and their solutions, you can troubleshoot problems more effectively when using Python terminal commands.
Understanding the Terminal and Python’s Interaction
Before we delve deeper into Python terminal commands, it’s crucial to understand the terminal or command line interface and how Python interacts with it.
The Terminal: Your Direct Line to the Operating System
The terminal, also known as the command line interface, is a text-based interface to the operating system. It’s a direct line of communication between you and your computer. You enter commands, and the computer executes them.
In the context of Python, the terminal allows you to execute Python scripts, interact with the Python interpreter, manage Python packages, and much more. Here’s an example of running a Python script from the terminal:
python3 my_script.py
# Output:
# [Expected output from my_script.py]
The Concept of PATH
When you enter a command in the terminal, the operating system needs to know where to find the program that can execute this command. This is where the PATH environment variable comes in. It’s a list of directories where the operating system looks for programs.
For example, when you enter the python3
command, the operating system will look in the directories listed in the PATH to find the python3
program. If it can’t find it, you’ll get a command not found
error.
You can view your PATH with the following command:
echo $PATH
# Output:
# /usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin
This command will print the directories in your PATH, separated by colons.
How Python Finds Its Modules
When you import a module in Python, Python needs to know where to find this module. It does this by looking in several places, including the directory where the script is located and the directories listed in the PYTHONPATH environment variable.
If Python can’t find the module, it will raise a ModuleNotFoundError
. To avoid this, make sure your modules are in the correct location and your PYTHONPATH is set up correctly.
Understanding these fundamentals will give you a better grasp of Python terminal commands and how they interact with your Python environment.
Python Terminal Commands in Larger Projects
Python terminal commands aren’t just for small scripts or quick tasks. They can also play a crucial role in larger projects, helping you manage your codebase and improve your productivity.
Shell Scripting with Python
One powerful application of Python terminal commands is shell scripting. A shell script is a file containing a series of commands that the shell can execute. You can use Python in your shell scripts to automate tasks, manipulate files, and much more. Here’s an example of a simple shell script that uses Python:
#!/bin/bash
python3 my_script.py
This script runs my_script.py
when executed. You could expand this script to run multiple Python scripts, manage Python packages, and much more.
Automation with Python
Python is an excellent language for automation, and Python terminal commands are a crucial part of this. With Python and the terminal, you can automate almost any task on your computer. For example, you could write a Python script that automatically downloads and processes data, then use a terminal command to run this script every day at a specific time.
Python Terminal Commands and Efficiency
Mastering Python terminal commands can significantly improve your efficiency as a developer. By automating tasks and managing your Python environment from the terminal, you can spend less time on tedious tasks and more time on writing and improving your code.
Further Resources for Mastering Python Terminal Commands
- Python’s Official Documentation: A comprehensive guide to using Python from the command line, straight from the creators of Python.
Real Python: Real Python offers a variety of tutorials and articles on Python programming, including Python terminal commands.
Pythonspot: Pythonspot provides tutorials on a wide range of Python topics, including Python and the command line.
Wrapping Up: Mastering Python Terminal Commands
In this comprehensive guide, we’ve navigated the world of Python terminal commands, exploring everything from basic execution of scripts to advanced debugging and profiling techniques.
We began with the basics, learning how to execute Python scripts, use Python’s interactive mode, and manage Python packages using pip from the terminal. We then ventured into more complex terrain, tackling Python’s built-in debugger (pdb), profiling Python scripts, and setting environment variables. Along the way, we addressed common issues that might arise when using Python terminal commands, such as syntax errors, module not found errors, and issues with Python versions, providing you with solutions and workarounds for each issue.
We also explored alternative ways to interact with Python, such as using IDEs like PyCharm or Jupyter notebooks, giving you a sense of the broader landscape of tools for interacting with Python. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Python Terminal Commands | Powerful, Direct Interaction | Requires Familiarity with Terminal |
PyCharm | Comprehensive Tools, GUI | Resource-Intensive |
Jupyter Notebooks | Interactive, Great for Data Analysis | Not Suitable for Larger Applications |
Whether you’re a beginner just starting out with Python terminal commands or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of Python terminal commands and their power. Happy coding!