Python Pandas | Quick Start Guide (With Examples)
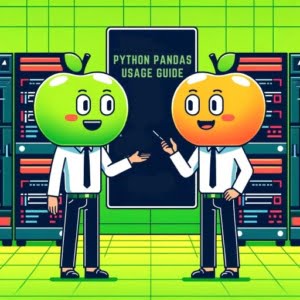
Harnessing the power of Python for data analysis is a core aspect of our operations at IOFLOOD, and Pandas is a pivotal tool in this endeavor. Today’s article has been designed to assist our customers utilize the capabilities and advantages of Pandas on their dedicated server hosting platforms.
In this comprehensive guide, we’ll start from the basics of Python Pandas, gradually moving to its advanced features. The goal is to help you become proficient in using this powerful library, enabling you to manipulate, analyze, and visualize data with ease.
Let’s dive into the world of Python Pandas and explore its capabilities!
TL;DR: What is Pandas in Python?
Pandas
is a powerful data manipulation library in Python. To use it only requires you to import it using the code,import pandas as pd
. It provides data structures and functions needed for manipulating structured data, making data analysis a more streamlined process.
Here’s a simple example of using pandas:
import pandas as pd
# Creating a dictionary with data
data = {'Name': ['John', 'Anna', 'Peter'], 'Age': [28, 24, 22]}
# Converting the dictionary into a DataFrame
df = pd.DataFrame(data)
# Printing the DataFrame
print(df)
# Output:
Name Age
0 John 28
1 Anna 24
2 Peter 22
In the above example, we first import the pandas library. Then, we create a dictionary with some data. This dictionary is converted into a pandas DataFrame using the pd.DataFrame()
function. Finally, we print the DataFrame, which neatly organizes our data into rows and columns.
Intrigued? Keep reading for a deeper understanding and more advanced usage of Python Pandas!
Table of Contents
The Basics of DataFrame Handling
Pandas revolves around two main data structures: Series and DataFrame. A Series is a one-dimensional array-like object, while a DataFrame is a two-dimensional table, similar to an Excel spreadsheet.
Creating a DataFrame
Creating a DataFrame in Pandas is straightforward. You can create one from a dictionary, a list, or even read data from a CSV file. Here’s an example of creating a DataFrame from a dictionary:
import pandas as pd
data = {'Name': ['John', 'Anna', 'Peter'], 'Age': [28, 24, 22]}
df = pd.DataFrame(data)
print(df)
# Output:
# Name Age
# 0 John 28
# 1 Anna 24
# 2 Peter 22
In the above code, we first import the pandas library as pd. Then, we create a dictionary ‘data’ with ‘Name’ and ‘Age’ as keys and lists of names and ages as values. We then pass this dictionary to the pd.DataFrame()
function to create a DataFrame. When we print this DataFrame, it displays our data in a neat tabular format.
Reading Data from Different Sources
Pandas also allows you to read data from different sources like CSV files, Excel files, SQL databases, and more. Here’s an example of reading data from a CSV file:
df = pd.read_csv('data.csv')
print(df.head())
# Output: Prints the first 5 rows of the DataFrame
In this example, we use the pd.read_csv()
function to read a CSV file named ‘data.csv’ and store it in a DataFrame. We then print the first 5 rows of the DataFrame using the df.head()
function.
Basic DataFrame Manipulation Techniques
Pandas provides several functions for basic data manipulation. You can add or drop columns, sort data, filter rows, and more. Here’s an example of adding a new column to our DataFrame:
df['Salary'] = [50000, 60000, 70000]
print(df)
# Output:
# Name Age Salary
# 0 John 28 50000
# 1 Anna 24 60000
# 2 Peter 22 70000
In this code, we add a new column ‘Salary’ to our DataFrame by assigning a list of salaries to df['Salary']
. When we print the DataFrame, it now includes the ‘Salary’ column.
These basic operations are just the tip of the iceberg. Python Pandas offers a plethora of functions to manipulate data in more advanced ways, which we’ll explore in the next section.
Advanced Uses of Pandas Library
Once you’ve grasped the basics of Python Pandas, you can start exploring more advanced data manipulation techniques. In this section, we’ll discuss merging, grouping, and reshaping data, all of which are fundamental to advanced data analysis.
Merging Data
Merging is a way of combining different DataFrames into one. Let’s say we have two DataFrames: df1
and df2
. We can merge them based on a common column using the merge()
function:
df1 = pd.DataFrame({'Name': ['John', 'Anna', 'Peter'], 'Age': [28, 24, 22]})
df2 = pd.DataFrame({'Name': ['John', 'Anna', 'Peter'], 'Salary': [50000, 60000, 70000]})
df = pd.merge(df1, df2, on='Name')
print(df)
# Output:
# Name Age Salary
# 0 John 28 50000
# 1 Anna 24 60000
# 2 Peter 22 70000
In this example, we merge df1
and df2
based on the ‘Name’ column. The resulting DataFrame df
contains the ‘Name’, ‘Age’, and ‘Salary’ columns.
Grouping Data
Grouping is another powerful feature of Pandas. It allows you to group your data based on certain criteria and then apply functions like sum, count, or mean to each group. Here’s an example:
df = pd.DataFrame({'Name': ['John', 'Anna', 'Peter', 'John', 'Anna'], 'Score': [85, 90, 95, 88, 92]})
grouped_df = df.groupby('Name').mean()
print(grouped_df)
# Output:
# Score
# Name
# Anna 91.0
# John 86.5
# Peter 95.0
In this code, we create a DataFrame with ‘Name’ and ‘Score’ columns. We then group this DataFrame by the ‘Name’ column and calculate the mean score for each name. The resulting DataFrame grouped_df
shows the average score for each name.
Reshaping Data
Reshaping data involves changing the structure of your data without altering its contents. One common way to reshape data in Pandas is by pivoting. Here’s an example:
df = pd.DataFrame({'Date': ['2020-01-01', '2020-01-01', '2020-01-02', '2020-01-02'], 'City': ['New York', 'Los Angeles', 'New York', 'Los Angeles'], 'Temperature': [32, 75, 30, 77]})
pivoted_df = df.pivot(index='Date', columns='City', values='Temperature')
print(pivoted_df)
# Output:
# City Los Angeles New York
# Date
# 2020-01-01 75 32
# 2020-01-02 77 30
In this example, we create a DataFrame with ‘Date’, ‘City’, and ‘Temperature’ columns. We then pivot this DataFrame to create a new DataFrame pivoted_df
where each row represents a date, each column represents a city, and cell values represent temperatures.
These advanced techniques allow you to manipulate your data in more sophisticated ways, enabling you to extract meaningful insights from complex datasets.
Alternative Data Tools to Explore
While Python Pandas is a powerful tool for data manipulation, it’s not the only one. Other libraries like NumPy and Dask also offer robust capabilities. Depending on your specific needs, these alternatives might sometimes be more suitable.
Diving into NumPy
NumPy, short for Numerical Python, is a library best known for its support for large multi-dimensional arrays and matrices. It also includes a collection of mathematical functions to operate on these arrays. Here’s a simple example of NumPy in action:
import numpy as np
# Creating a 2D NumPy array
array = np.array([[1, 2, 3], [4, 5, 6]])
print(array)
# Output:
# [[1 2 3]
# [4 5 6]]
In this example, we create a 2D array using NumPy. The np.array()
function creates an array from the list of lists we provided.
Discovering Dask
Dask is a flexible library for parallel computing in Python. It’s designed to integrate seamlessly with NumPy, Pandas, and Scikit-Learn. This makes it a powerful tool when working with larger-than-memory computations. Here’s an example of how you can use Dask to perform lazy computations:
import dask.array as da
# Creating a large array
x = da.ones((10000, 10000), chunks=(1000, 1000))
# Performing a lazy computation
y = x + x.T
# Computing the result
result = y.compute()
print(result)
# Output: A 10000x10000 array with 2's
In this code, we first create a large 10000×10000 Dask array filled with ones. The chunks
argument divides our array into smaller chunks, each of which fits into memory. We then perform a lazy computation, adding the array to its transpose. The computation isn’t performed immediately; instead, Dask builds up a task graph to execute when compute()
is called. Finally, we compute and print the result.
Choosing the Right Tool
While Pandas, NumPy, and Dask all offer powerful features, the right tool depends on your specific needs. If you’re working with structured data and need high-level data manipulation capabilities, Pandas is the way to go. For numerical operations on large multi-dimensional arrays, NumPy is your best bet. And if you’re dealing with computations that don’t fit into memory, Dask is an excellent choice.
Troubleshooting Errors in Pandas
Like any powerful tool, Python Pandas can sometimes be complex and tricky to handle. It’s not uncommon to encounter issues, especially when dealing with large and messy real-world data. Here, we’ll discuss some common problems and their solutions.
Handling Missing Data
Missing data is a common issue in data analysis. Thankfully, Pandas provides several ways to handle it. You can choose to either drop the missing data or fill it with some value.
Here’s an example of dropping missing data:
import numpy as np
import pandas as pd
df = pd.DataFrame({'A': [1, 2, np.nan], 'B': [5, np.nan, np.nan], 'C': [1, 2, 3]})
df = df.dropna()
print(df)
# Output:
# A B C
# 0 1.0 5.0 1.0
In this example, we create a DataFrame with some missing values (np.nan). We then use the dropna()
function to remove any rows with missing values.
Here’s an example of filling missing data with a value (e.g., 0):
import numpy as np
import pandas as pd
df = pd.DataFrame({'A': [1, 2, np.nan], 'B': [5, np.nan, np.nan], 'C': [1, 2, 3]})
df = df.fillna(value=0)
print(df)
# Output:
# A B C
# 0 1.0 5.0 1
# 1 2.0 0.0 2
# 2 0.0 0.0 3
In this code, we use the fillna()
function to replace all missing values with 0.
Dealing with Type Errors
Another common issue is type errors. These occur when an operation is performed on a data type that doesn’t support it. To avoid type errors, it’s important to ensure that your data types are correct.
Here’s an example of checking the data types of a DataFrame:
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4.0, 5.0, 6.0], 'C': ['7', '8', '9']})
print(df.dtypes)
# Output:
# A int64
# B float64
# C object
# dtype: object
In this example, we create a DataFrame with integer, float, and string columns. We then use the dtypes
attribute to print the data types of the columns.
By understanding these common issues and how to resolve them, you can avoid many pitfalls and work more efficiently with Python Pandas.
Overview of Pandas Data Structures
The heart of Python Pandas lies in its fundamental data structures: the Series and DataFrame. Understanding these structures is key to mastering data manipulation with Pandas.
Diving into Series
A Series in Pandas is a one-dimensional array-like object that can hold any data type. It’s similar to a column in an Excel spreadsheet. Here’s an example of creating a Series:
import pandas as pd
s = pd.Series([1, 3, 5, np.nan, 6, 8])
print(s)
# Output:
# 0 1.0
# 1 3.0
# 2 5.0
# 3 NaN
# 4 6.0
# 5 8.0
# dtype: float64
In this example, we create a Series s
by passing a list of values to the pd.Series()
function. The resulting Series consists of an index on the left and values on the right.
Exploring DataFrame
A DataFrame, on the other hand, is a two-dimensional table of data with rows and columns. It’s similar to a spreadsheet or SQL table, or a dictionary of Series objects. Here’s an example of creating a DataFrame:
import pandas as pd
df = pd.DataFrame({'A': pd.Timestamp('20200101'),
'B': pd.Series(1, index=list(range(4)), dtype='float32'),
'C': np.array([3] * 4, dtype='int32'),
'D': pd.Categorical(['test', 'train', 'test', 'train']),
'E': 'foo'})
print(df)
# Output:
# A B C D E
# 0 2020-01-01 1.0 3 test foo
# 1 2020-01-01 1.0 3 train foo
# 2 2020-01-01 1.0 3 test foo
# 3 2020-01-01 1.0 3 train foo
In this code, we create a DataFrame df
by passing a dictionary of objects to the pd.DataFrame()
function. Each key-value pair in the dictionary corresponds to a column in the DataFrame. The resulting DataFrame displays a neat tabular representation of our data.
Pandas in the Python Data Analysis Ecosystem
Python Pandas fits into the data analysis ecosystem as a versatile tool for data manipulation. It works seamlessly with other libraries like NumPy for numerical operations, Matplotlib for plotting, and Scikit-learn for machine learning, making it a central part of any data analysis workflow in Python.
Practical Data Analysis using Pandas
Python Pandas is not just a tool for manipulating data; it’s a powerful library that plays a crucial role in real-world data analysis projects. Its functionalities extend beyond the basics, making it an invaluable asset in various stages of data analysis.
Pandas and Data Cleaning
Data cleaning is a critical step in any data analysis project. It involves handling missing data, dealing with outliers, and correcting inconsistent data types. With its robust data manipulation capabilities, Pandas simplifies these tasks, making data cleaning a more manageable process.
Exploratory Data Analysis with Pandas
Exploratory data analysis (EDA) is another area where Pandas shines. EDA involves understanding the patterns and relationships in data, and Pandas provides several functions for this purpose. For example, the describe()
function gives a quick statistical summary of your data, while the corr()
function calculates the pairwise correlation of columns.
Related Topics: Data Visualization and Machine Learning
Beyond data cleaning and EDA, Python Pandas also integrates well with other libraries in the Python ecosystem. For instance, you can use Pandas with Matplotlib for data visualization, creating plots and graphs from your DataFrames. Here’s a simple example:
import matplotlib.pyplot as plt
df = pd.DataFrame({'A': [1, 2, 3, 4, 5], 'B': [2, 4, 6, 8, 10]})
df.plot(kind='line')
plt.show()
# Output: A line plot of the data in df
In this code, we create a DataFrame and then plot it using the plot()
function. We then display the plot using plt.show()
. The result is a line plot of our data.
For machine learning tasks, you can use Pandas with Scikit-learn. You can easily convert your DataFrames into arrays that can be fed into Scikit-learn models.
Further Resources for Pandas Library
If you’re interested in learning more ways to utilize the Pandas library, here are a few resources that you might find helpful:
- This article by IOFlood on explains how to use the Pandas Merge() function to combine two dataframes based on a common key.
Reset Index | Step-by-Step Guide: This will guide will walk you through the process of resetting indexes in pandas with the reset_index() function.
Pandas documentation: For further reading, the official Pandas documentation is a great resource.
Pandas Tutorial – Learn Pandas by Examples: A tutorial on Pandas by DataCamp, with numerous examples to enhance learning.
Python Pandas Tutorial: A comprehensive tutorial on Python Pandas from W3Schools.
Wrapping Up Data Analysis in Pandas
Throughout this guide, we’ve explored the various facets of Python Pandas, from its basic usage to advanced techniques. We’ve seen how it simplifies data manipulation, making it an essential tool in any data scientist’s arsenal.
Navigating Through Python Pandas
We started with the basics, creating DataFrames and performing simple data manipulations. We then moved on to more advanced operations like merging, grouping, and reshaping data. Along the way, we tackled common issues like handling missing data and type errors, providing practical solutions to these problems.
Exploring Alternatives: NumPy and Dask
While Pandas is a powerful library, it’s not the only one. We also discussed alternatives like NumPy and Dask, each with its own strengths. NumPy shines with numerical operations on large multi-dimensional arrays, while Dask excels at computations that don’t fit into memory.
The Role of Pandas in Data Analysis
Beyond its data manipulation capabilities, Python Pandas plays a crucial role in real-world data analysis. It’s instrumental in data cleaning and exploratory data analysis, and integrates well with other libraries for data visualization and machine learning.
In conclusion, Python Pandas is a versatile and powerful library that simplifies data analysis in Python. Whether you’re a beginner just starting out or an experienced data scientist, mastering Pandas is a valuable skill that will undoubtedly enhance your data analysis workflow. Happy data analyzing!