Converting Array to List in Java: A Step-by-Step Guide
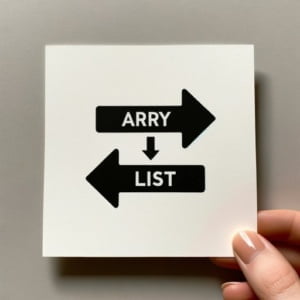
Ever found yourself wrestling with converting an array to a list in Java? You’re not alone. Many developers find this task a bit tricky, but Java provides several key methods to make this process smooth.
Think of Java’s array to list conversion as a magic trick – transforming an array into a list with just a few incantations of code. It’s a powerful tool that can greatly simplify your data manipulation tasks.
In this guide, we’ll walk you through the process of converting an array to a list in Java, from the basics to more advanced techniques. We’ll cover everything from using the Arrays.asList()
method, handling primitive and multi-dimensional arrays, to exploring alternative approaches using Java 8 Streams and the Collections framework.
Let’s dive in and start mastering array to list conversion in Java!
TL;DR: How Do I Convert an Array to a List in Java?
The simplest way to convert an array to a list in Java is by using the
Arrays.asList()
method. This function transforms your array into a list in a snap.
Here’s a quick example:
String[] array = {"A", "B", "C"};
List<String> list = Arrays.asList(array);
// Output:
// [A, B, C]
In this example, we have an array of strings array
. We use the Arrays.asList()
method to convert this array into a list list
. The output is a list containing the elements ‘A’, ‘B’, and ‘C’.
This is a basic way to convert an array to a list in Java, but there’s much more to learn about this process. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Converting Array to List with Arrays.asList()
- Handling Primitive and Multi-Dimensional Arrays
- Exploring Alternative Methods for Array to List Conversion
- Troubleshooting Common Issues in Array to List Conversion
- Understanding Java’s Array and List Data Types
- The Relevance of Array to List Conversion
- Further Resources for Mastering Array to List Conversion
- Wrapping Up: Java Array to List Conversion
Converting Array to List with Arrays.asList()
The Arrays.asList()
method is the most straightforward way to convert an array to a list in Java. This utility method returns a fixed-size list backed by the original array. Let’s dive into how it works and when to use it.
How Arrays.asList()
Works
Here’s a simple example:
String[] array = {"Apple", "Banana", "Cherry"};
List<String> list = Arrays.asList(array);
System.out.println(list);
// Output:
// [Apple, Banana, Cherry]
In this code snippet, we have an array of strings array
. We use the Arrays.asList()
method to convert this array into a list list
. The output is a list containing the elements ‘Apple’, ‘Banana’, and ‘Cherry’.
When to Use Arrays.asList()
The Arrays.asList()
method is best used when you have a non-primitive array that you want to convert to a list. It’s a quick and easy solution for this task. However, it’s important to note that the list returned by this method is backed by the original array, which means any changes to the original array will be reflected in the list and vice versa.
Advantages and Pitfalls of Arrays.asList()
The advantage of using Arrays.asList()
is its simplicity and efficiency. It’s a one-liner solution to convert an array to a list.
However, there are some pitfalls. The main one is that the returned list is fixed-size. That means you cannot add or remove elements from the list. If you try to do so, it will throw an UnsupportedOperationException
.
For example:
String[] array = {"Apple", "Banana", "Cherry"};
List<String> list = Arrays.asList(array);
list.add("Dragonfruit"); // This will throw an UnsupportedOperationException
// Output:
// Exception in thread "main" java.lang.UnsupportedOperationException
In this example, we tried to add a new element ‘Dragonfruit’ to the list. However, since the list is a fixed-size list, the add()
operation throws an UnsupportedOperationException
.
In the next section, we’ll discuss more advanced techniques for converting an array to a list in Java, including how to handle these potential pitfalls.
Handling Primitive and Multi-Dimensional Arrays
As you dive deeper into Java, you’ll encounter scenarios where you need to convert more complex arrays to lists. Two such scenarios include handling primitive arrays and multi-dimensional arrays.
Converting Primitive Arrays to List
The Arrays.asList()
method does not work directly with primitive arrays. If you use Arrays.asList()
with a primitive array, it will create a list with a single element, which is the array itself.
Let’s see this in action:
int[] array = {1, 2, 3};
List<int[]> list = Arrays.asList(array);
System.out.println(list);
// Output:
// [[I@4e25154f]
In this example, we tried to convert a primitive array of integers array
to a list list
using Arrays.asList()
. However, the output is not as expected. Instead of creating a list with the elements ‘1’, ‘2’, and ‘3’, it created a list with a single element, which is the array itself.
To convert a primitive array to a list, you can use Java 8’s Arrays.stream()
method combined with boxed()
and collect()
methods:
int[] array = {1, 2, 3};
List<Integer> list = Arrays.stream(array).boxed().collect(Collectors.toList());
System.out.println(list);
// Output:
// [1, 2, 3]
In this example, we used Arrays.stream()
to create a stream from the array. Then, we used boxed()
to convert the stream of primitives to a stream of wrapper objects, and collect()
to convert the stream into a list. The output is a list with the elements ‘1’, ‘2’, and ‘3’.
Converting Multi-Dimensional Arrays to List
Converting a multi-dimensional array to a list is a bit trickier. Let’s see an example:
String[][] array = { {"Apple", "Banana"}, {"Cherry", "Dragonfruit"} };
List<List<String>> list = Arrays.stream(array).map(Arrays::asList).collect(Collectors.toList());
System.out.println(list);
// Output:
// [[Apple, Banana], [Cherry, Dragonfruit]]
In this example, we have a two-dimensional array of strings array
. We used Arrays.stream()
to create a stream from the array. Then, we used map()
with Arrays.asList()
to convert each sub-array into a list, and collect()
to convert the stream into a list. The output is a list of lists, with each sub-list containing the elements of the corresponding sub-array.
In the next section, we’ll explore alternative approaches for converting an array to a list in Java, including using Java 8 Streams and the Collections framework.
Exploring Alternative Methods for Array to List Conversion
While Arrays.asList()
is a handy tool for array to list conversion in Java, there are other methods that offer more flexibility and control. Two such methods are using Java 8 Streams and the Collections framework.
Using Java 8 Streams
Java 8 introduced the concept of Streams, which can be used to perform complex data manipulation tasks. With Streams, you can convert an array to a list in a more flexible way compared to Arrays.asList()
.
Here is an example of converting an array to a list using Streams:
String[] array = {"Apple", "Banana", "Cherry"};
List<String> list = Arrays.stream(array).collect(Collectors.toList());
System.out.println(list);
// Output:
// [Apple, Banana, Cherry]
In this example, we used Arrays.stream()
to create a stream from the array, and collect()
with Collectors.toList()
to convert the stream into a list. The output is a list with the elements ‘Apple’, ‘Banana’, and ‘Cherry’.
The advantage of using Streams is that you can perform additional operations on the stream, such as filtering or mapping, before converting it into a list.
Using the Collections Framework
The Collections framework provides the Collections.addAll()
method, which can be used to convert an array to a list. This method is more flexible than Arrays.asList()
because the resulting list is modifiable.
Here is an example of converting an array to a list using Collections.addAll()
:
String[] array = {"Apple", "Banana", "Cherry"};
List<String> list = new ArrayList<>();
Collections.addAll(list, array);
System.out.println(list);
// Output:
// [Apple, Banana, Cherry]
In this example, we created an empty list list
and used Collections.addAll()
to add all elements from the array to the list. The output is a list with the elements ‘Apple’, ‘Banana’, and ‘Cherry’.
The advantage of using Collections.addAll()
is that the resulting list is modifiable, meaning you can add or remove elements from the list. However, it requires an extra step of creating an empty list before adding the elements.
In the next section, we’ll discuss common issues you might encounter during array to list conversion in Java, and how to solve them.
Troubleshooting Common Issues in Array to List Conversion
While converting an array to a list in Java, you might encounter some common issues. Understanding these issues and knowing how to solve them can make your coding journey smoother.
Dealing with ‘UnsupportedOperationException’
One common issue is the UnsupportedOperationException
that occurs when trying to modify the list returned by Arrays.asList()
. This happens because Arrays.asList()
returns a fixed-size list backed by the original array.
Let’s see an example:
String[] array = {"Apple", "Banana", "Cherry"};
List<String> list = Arrays.asList(array);
list.add("Dragonfruit"); // This will throw an UnsupportedOperationException
// Output:
// Exception in thread "main" java.lang.UnsupportedOperationException
In this example, we tried to add a new element ‘Dragonfruit’ to the list. However, since the list is a fixed-size list, the add()
operation throws an UnsupportedOperationException
.
To avoid this issue, you can create a new list that is not backed by the original array. One way to do this is by using the ArrayList
constructor:
String[] array = {"Apple", "Banana", "Cherry"};
List<String> list = new ArrayList<>(Arrays.asList(array));
list.add("Dragonfruit"); // This will not throw an exception
System.out.println(list);
// Output:
// [Apple, Banana, Cherry, Dragonfruit]
In this example, we created a new ArrayList
from the list returned by Arrays.asList()
. This new list is not backed by the original array, so you can add or remove elements without throwing an UnsupportedOperationException
.
In the next section, we’ll delve into the fundamentals of Java’s array and list data types, and the underlying concepts of array to list conversion.
Understanding Java’s Array and List Data Types
Before we delve deeper into the process of converting arrays to lists in Java, it’s important to understand the fundamental concepts underlying these two data types.
What is an Array in Java?
An array in Java is a static data structure that can store a fixed number of elements of the same type. The elements of an array are stored in contiguous memory locations, and each element can be accessed directly using its index.
Here’s an example of declaring and initializing an array in Java:
int[] array = {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(array));
// Output:
// [1, 2, 3, 4, 5]
In this example, we declared an array array
of integers and initialized it with five elements. The Arrays.toString()
method is used to print the elements of the array.
What is a List in Java?
A list in Java, on the other hand, is a dynamic data structure that can grow or shrink in size as needed. It is part of the Java Collections Framework and implements the List
interface. In a list, elements can be added or removed, and it can store elements of different types.
Here’s an example of declaring and initializing a list in Java:
List<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Cherry");
System.out.println(list);
// Output:
// [Apple, Banana, Cherry]
In this example, we declared a list list
of strings and added three elements to it. The add()
method is used to add elements to the list.
Differences Between Arrays and Lists in Java
The main difference between arrays and lists in Java is their flexibility. While arrays are static and have a fixed size, lists are dynamic and can grow or shrink as needed. This makes lists a more flexible option for storing data that may change in size over time.
However, arrays have the advantage of being simpler and more efficient in terms of memory usage and performance. Arrays are also more suitable for storing data of a known, fixed size.
In the next section, we’ll discuss the relevance of array to list conversion in different scenarios, such as data processing or working with APIs.
The Relevance of Array to List Conversion
Converting arrays to lists in Java is not just a programming exercise. It has real-world applications that can make your code more efficient and adaptable. Let’s explore some of these scenarios.
Data Processing with Java
In data processing tasks, you often need to manipulate and transform data. Arrays and lists are two of the most common data structures used for storing data. Converting between these two data structures can give you the flexibility to use the most appropriate data structure for each task.
Working with APIs in Java
When working with APIs in Java, you often need to convert data between different formats. For example, an API might return data as an array, but your code might be easier to write and read if you convert that array to a list.
Exploring Java Collections Framework
The Java Collections Framework is a set of classes and interfaces that implement common data structures and algorithms. By understanding how to convert arrays to lists, you can unlock the full potential of this powerful framework.
Diving into Java 8 Streams
Java 8 introduced the concept of Streams, which provide a new abstraction for performing complex data manipulation tasks. Converting arrays to lists is a fundamental operation that you’ll use frequently when working with Streams.
Further Resources for Mastering Array to List Conversion
To deepen your understanding of converting arrays to lists in Java, here are some additional resources you might find helpful:
- Tips and Techniques for Java Casting – Explore the use of generics and casting in Java collections for type safety.
Char to String in Java – Convert individual characters to strings in Java effortlessly.
Converting List to Array – Learn techniques to convert list collections to array data structures.
Official Java Arrays and Lists Tutorial – An official Oracle guide on arrays and lists in Java programming.
Java 8 Streams Tutorial – Detailed Baeldung tutorial on the effective use of streams in Java 8.
Java Collections Framework – Comprehensive coverage of Java collections framework by Java Guides.
Wrapping Up: Java Array to List Conversion
In this comprehensive guide, we’ve explored the ins and outs of converting arrays to lists in Java, a fundamental operation in data manipulation.
We began with the basics, learning how to convert an array to a list using the Arrays.asList()
method. We then delved into more advanced techniques, such as handling primitive and multi-dimensional arrays. Along the way, we addressed common issues you might encounter during this conversion process, such as the UnsupportedOperationException
when trying to modify the fixed-size list returned by Arrays.asList()
.
We also ventured into alternative methods for this conversion, exploring the power of Java 8 Streams and the flexibility of the Collections framework. These alternatives offer more control and versatility compared to Arrays.asList()
, equipping you with a wider range of tools for your programming tasks.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity |
---|---|---|
Arrays.asList() | Low | Low |
Java 8 Streams | High | Moderate |
Collections Framework | High | Moderate |
Whether you’re just starting out with Java or looking to deepen your understanding of arrays and lists, we hope this guide has been a valuable resource. With these techniques in your toolkit, you’re well-equipped to handle array to list conversion in Java with skill and confidence.
Converting arrays to lists is a key operation in Java, enabling more dynamic and adaptable data manipulation. Now, you’re ready to harness this power in your own code. Happy coding!