Char to String Conversion in Java: A How-To Guide
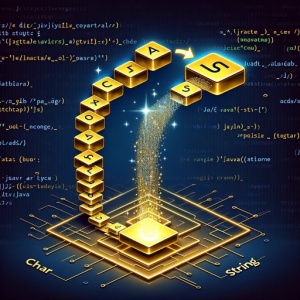
Are you finding it challenging to convert a char to a string in Java? You’re not alone. Many developers grapple with this task, but Java, like a skilled linguist, provides several ways to transform a single character into a string.
This guide will walk you through the process of converting char to string in Java. We’ll explore Java’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering char to string conversion in Java!
TL;DR: How Do I Convert a Char to a String in Java?
There are various methods to convert a char to a string in Java. The simplest method is by using the
.toString()
method, with the syntaxCharacter.toString(char)
.
Here’s a quick example:
char c = 'a';
String s = Character.toString(c);
// Output:
// 'a'
In this example, we have a character ‘a’ that we want to convert into a string. By using the Character.toString(char)
method, we can easily achieve this. The character ‘a’ is passed as an argument to the Character.toString(char)
method, which returns the string ‘a’.
But there’s more to char to string conversion in Java than just this. Continue reading for more detailed information and advanced techniques.
Table of Contents
- Basic Char to String Conversion in Java
- Advanced Char to String Conversion in Java
- Alternative Char to String Conversion Techniques in Java
- Troubleshooting Char to String Conversion in Java
- Understanding Char and String in Java
- Applying Char to String Conversion in Real-World Java Projects
- Wrapping Up: Char to String Conversion in Java
Basic Char to String Conversion in Java
At the beginner level, the most straightforward method to convert a char to a string in Java is by using the Character.toString(char)
method. This method is part of the Character class in Java and is designed to convert a char value into a String.
Let’s look at a simple example:
char myChar = 'b';
String myString = Character.toString(myChar);
System.out.println(myString);
// Output:
// 'b'
In this example, we have a character ‘b’ that we want to convert into a string. By using the Character.toString(char)
method, we can easily achieve this. The character ‘b’ is passed as an argument to the Character.toString(char)
method, which returns the string ‘b’.
Pros and Cons of Character.toString(char)
The primary advantage of this method is its simplicity and readability. Even someone with a basic understanding of Java can understand what’s going on. It’s also part of the Java standard library, which means you don’t need to import any external libraries.
However, this method can be inefficient if you’re dealing with multiple characters that you want to convert to strings. In such cases, other methods like StringBuilder
or StringBuffer
may be more efficient, which we will discuss in the next section.
Advanced Char to String Conversion in Java
As you become more familiar with Java, you might come across situations where you need to convert multiple characters into a string. In these cases, using StringBuilder
or StringBuffer
classes can be more efficient.
Using StringBuilder
StringBuilder
is a mutable sequence of characters. It provides an append method, which can be used to add a character to the existing sequence.
Let’s look at an example:
char myChar = 'c';
StringBuilder sb = new StringBuilder();
sb.append(myChar);
String myString = sb.toString();
System.out.println(myString);
// Output:
// 'c'
In this example, we create a StringBuilder
object and append the character ‘c’ to it. We then convert the StringBuilder
object into a string.
Using StringBuffer
StringBuffer
is similar to StringBuilder
, but it is thread-safe. This means it can be used in multithreaded environments.
Here’s how you can use StringBuffer
to convert a char to a string:
char myChar = 'd';
StringBuffer sb = new StringBuffer();
sb.append(myChar);
String myString = sb.toString();
System.out.println(myString);
// Output:
// 'd'
Just like the StringBuilder
example, we create a StringBuffer
object, append the character ‘d’ to it, and then convert the StringBuffer
object into a string.
Pros and Cons of StringBuilder
and StringBuffer
Both StringBuilder
and StringBuffer
are very efficient when dealing with multiple characters. They are more efficient than Character.toString(char)
because they don’t create a new string object for each character.
However, they are more complex to use and understand than Character.toString(char)
. Also, StringBuffer
is slower than StringBuilder
due to its thread safety feature, which is not always necessary.
Alternative Char to String Conversion Techniques in Java
While Java’s built-in methods like Character.toString(char)
, StringBuilder
, and StringBuffer
are efficient, there are other ways to convert a char to a string in Java, especially when working with third-party libraries.
Char to String Conversion Using Apache Commons
Apache Commons is a popular third-party library that provides utilities for the Java programming language. It includes the StringUtils
class, which has a method called valueOf(char)
. This method is similar to Character.toString(char)
, but it’s part of the Apache Commons library.
Here’s an example of how to use it:
import org.apache.commons.lang3.StringUtils;
char myChar = 'e';
String myString = StringUtils.valueOf(myChar);
System.out.println(myString);
// Output:
// 'e'
In this example, we import the StringUtils
class from the Apache Commons library, and then use its valueOf(char)
method to convert the character ‘e’ to a string.
Pros and Cons of Using Apache Commons
The main advantage of using Apache Commons is that it provides many other utilities that can make your Java code more efficient and easier to read. For example, it includes methods for string manipulation, number conversion, date/time manipulation, and much more.
However, the downside is that you need to add an external dependency to your project. This can make your project larger and potentially more difficult to manage. Also, if the Apache Commons library is not already part of your project, you would need to download and install it, which can be a hassle.
Char to String Conversion Using Guava
Guava is another popular third-party library for Java. It provides utilities for collections, caching, primitives support, concurrency, common annotations, string processing, and much more. It includes the Chars
class, which has a method called toString(char)
. This method is similar to Character.toString(char)
, but it’s part of the Guava library.
Here’s an example of how to use it:
import com.google.common.primitives.Chars;
char myChar = 'f';
String myString = Chars.toString(myChar);
System.out.println(myString);
// Output:
// 'f'
In this example, we import the Chars
class from the Guava library, and then use its toString(char)
method to convert the character ‘f’ to a string.
Pros and Cons of Using Guava
Guava provides a lot of powerful utilities that can make your Java code more efficient and easier to read. It’s a comprehensive library that can handle many common programming tasks.
However, like Apache Commons, you need to add an external dependency to your project. This can make your project larger and potentially more difficult to manage. Also, if the Guava library is not already part of your project, you would need to download and install it, which can be a hassle.
Troubleshooting Char to String Conversion in Java
While converting a char to a string in Java is generally straightforward, you might encounter some issues. Let’s discuss some common problems and their solutions.
Null Character
One common issue is trying to convert a null character into a string. In Java, a null character is represented as ‘\0’. If you attempt to convert this into a string using the Character.toString(char)
method, you might not get the result you expect.
char myChar = '\0';
String myString = Character.toString(myChar);
System.out.println(myString);
// Output:
// ''
In this example, we attempt to convert a null character into a string. The resulting string is empty, not ‘null’ or ‘\0’. This is because the null character is a non-printing character.
Special Characters
Another issue arises when dealing with special characters. In Java, special characters like newline (‘\n’), carriage return (‘\r’), and tab (‘\t’) can be represented as char values. If you attempt to convert these into strings, they will retain their special properties.
char myChar = '\n';
String myString = Character.toString(myChar);
System.out.println('Start' + myString + 'End');
// Output:
// Start
// End
In this example, we convert a newline character into a string. When we print the string, it creates a new line between ‘Start’ and ‘End’.
Unicode Characters
Java supports Unicode, which means you can represent a wide range of characters from different languages as char values. However, when converting these into strings, you need to be aware of the Unicode standard.
char myChar = '\u00E9'; // Unicode for é
String myString = Character.toString(myChar);
System.out.println(myString);
// Output:
// 'é'
In this example, we convert a Unicode character into a string. The resulting string is ‘é’, which is the character represented by the Unicode value ‘\u00E9’.
Understanding Char and String in Java
Before we delve deeper into converting char to string in Java, it’s essential to understand what char and string are in the context of Java.
What is Char in Java?
In Java, the char keyword is a primitive data type that is used to store a single character. A char value can store any character from the Unicode character set, which includes letters (both uppercase and lowercase), digits, punctuation marks, special characters, and even characters from non-English languages.
Here’s a simple example of declaring a char variable in Java:
char myChar = 'g';
System.out.println(myChar);
// Output:
// 'g'
In this example, we declare a char variable named myChar
and assign it the character ‘g’. When we print myChar
, it outputs ‘g’.
What is String in Java?
A String in Java is not a primitive data type like char. Instead, it’s a class that represents a sequence of characters. In other words, a String can contain multiple characters, including zero characters (an empty string).
Here’s a simple example of declaring a String variable in Java:
String myString = "hello";
System.out.println(myString);
// Output:
// 'hello'
In this example, we declare a String variable named myString
and assign it the string “hello”. When we print myString
, it outputs ‘hello’.
Char to String Conversion: Why is it Important?
In Java, char and String are different types, and they can’t be used interchangeably. For example, you can’t pass a char value to a method that expects a String. This is where char to string conversion comes in handy.
By understanding the fundamentals of char and String in Java, you can better grasp the methods and techniques we’ve discussed for converting char to string. It also helps you understand why certain methods are more efficient than others, depending on the situation.
Applying Char to String Conversion in Real-World Java Projects
The techniques of converting char to string in Java we’ve discussed are not just theoretical. They have practical applications in real-world Java projects. For instance, you might need to convert user input into a format that your program can process. Or, you might need to manipulate text data in a file or database.
In all these scenarios, understanding how to convert char to string in Java becomes crucial. Whether you’re using the basic Character.toString(char)
method, the advanced StringBuilder
or StringBuffer
classes, or even third-party libraries like Apache Commons or Guava, you’re equipped to handle a wide range of situations.
Exploring Related Topics
Once you’ve mastered char to string conversion in Java, you might want to explore related topics. For example, you could learn about string manipulation in Java, which includes tasks like comparing strings, concatenating strings, and searching for substrings. Or, you could delve into Java’s support for Unicode and learn how to handle characters from non-English languages.
Further Resources for Mastering Char to String Conversion in Java
To help you further your understanding, here are some external resources that provide more in-depth information on char to string conversion in Java and related topics:
- Beginner’s Guide to Java Casting – Discover how casting allows you to convert values between different data types in Java.
ToString Method in Java – Explore the toString() method in Java for converting objects to string representations.
Converting Array to List – Explore methods to convert array data structures into list collections.
Java String Documentation – Official Java documentation for the String class.
Java Character Documentation – Official Java documentation for the Character class.
Java Practices – A collection of best practices for Java development.
Wrapping Up: Char to String Conversion in Java
In this comprehensive guide, we’ve explored the various ways to convert a char to a string in Java, from the simplest methods to more advanced techniques.
We began with the basic Character.toString(char)
method, a straightforward and easy-to-understand approach. We then delved into more advanced techniques using the StringBuilder
and StringBuffer
classes, which provide more efficiency when dealing with multiple characters. Finally, we explored alternative approaches using third-party libraries like Apache Commons and Guava, offering more utilities and functionalities.
Along the way, we tackled common issues you might encounter when converting a char to a string in Java, such as null characters, special characters, and Unicode characters, providing you with solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Character.toString(char) | Simple and easy to understand | Less efficient for multiple characters |
StringBuilder / StringBuffer | Efficient for multiple characters | More complex to use |
Apache Commons / Guava | Provides many other utilities | Requires external dependency |
Whether you’re just starting out with Java or you’re looking to level up your char to string conversion skills, we hope this guide has given you a deeper understanding of the different methods and their pros and cons.
With the knowledge you’ve gained from this guide, you’re now well-equipped to handle any char to string conversion task in Java. Happy coding!