Bash and JSON: Your Guide to Parsing JSON in Bash
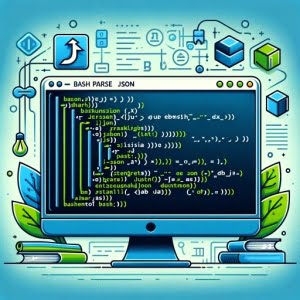
Are you finding it challenging to parse JSON data in Bash? You’re not alone. Many developers find themselves grappling with this task, but there’s a tool that can make this process a breeze.
Think of Bash as a skilled linguist that can interpret JSON data, making it easier to handle. These skills can help you manage and manipulate data more effectively, opening up a world of possibilities for your scripts.
This guide will walk you through the process of parsing JSON data in Bash, from basic to advanced techniques. We’ll cover everything from using tools like ‘jq’ and ‘json.tool’, to handling complex JSON structures, and even alternative approaches.
So, let’s dive in and start mastering JSON parsing in Bash!
TL;DR: How Do I Parse JSON in Bash?
Parsing JSON in Bash can be achieved using tools like
'json.tool'
, or'jq'
with the syntax,echo '{"key":"value"}' | jq '.key'
. These tools can interpret JSON data, making it easier to handle in your scripts.
Here’s a simple example using ‘jq’:
echo '{"key":"value"}' | jq '.key'
# Output:
# "value"
In this example, we’re using the ‘jq’ tool to parse a JSON string. The ‘jq’ command takes a JSON object (in this case, ‘{“key”:”value”}’) and a filter (‘.key’) as input. The filter ‘.key’ extracts the value associated with the ‘key’ in the JSON object, resulting in the output ‘value’.
This is just a basic way to parse JSON in Bash, but there’s much more to learn about handling JSON data effectively. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Getting Started with JSON Parsing in Bash
- Parsing Complex JSON Structures in Bash
- Expert-Level JSON Parsing in Bash: Exploring Alternatives
- Troubleshooting Common JSON Parsing Issues in Bash
- Understanding JSON Data and Bash Scripting
- The Power of JSON Parsing in Bash
- Wrapping Up: Mastering JSON Parsing in Bash
Getting Started with JSON Parsing in Bash
When starting with JSON parsing in Bash, two of the most commonly used tools are ‘jq’ and ‘json.tool’. These tools are designed to help you parse JSON data efficiently.
Parsing JSON with ‘jq’
‘jq’ is a lightweight and flexible command-line JSON processor. It’s like ‘sed’ for JSON data – you can use it to slice, filter, map, and transform structured data.
Consider the following example:
echo '{"name":"John", "age":30, "city":"New York"}' | jq '.name'
# Output:
# "John"
In this example, we’re using ‘jq’ to parse a JSON string. The ‘jq’ command takes a JSON object and a filter (‘.name’) as input. The filter ‘.name’ extracts the value associated with the ‘name’ in the JSON object, resulting in the output ‘John’.
Parsing JSON with ‘json.tool’
Python’s ‘json.tool’ module can also be used to parse JSON data in Bash. It’s a simple-to-use command-line tool for validating and pretty-printing JSON objects.
Here’s an example:
echo '{"name":"John", "age":30, "city":"New York"}' | python -m json.tool
# Output:
# {
# "name": "John",
# "age": 30,
# "city": "New York"
# }
In this case, we’re using Python’s ‘json.tool’ module to pretty-print a JSON string. The ‘json.tool’ module takes a JSON object as input and outputs a more readable version of it.
While both ‘jq’ and ‘json.tool’ are effective for parsing JSON data in Bash, they have their advantages and potential pitfalls. ‘jq’ is more powerful and flexible, allowing you to perform complex operations on JSON data. On the other hand, ‘json.tool’ is simpler to use and comes pre-installed with Python, but it doesn’t offer the same level of functionality as ‘jq’.
Parsing Complex JSON Structures in Bash
As you dive deeper into JSON parsing in Bash, you’ll come across more complex JSON structures, such as nested objects and arrays. Both ‘jq’ and ‘json.tool’ can handle these structures, but the approach might differ slightly.
Handling Nested Objects with ‘jq’
Let’s consider a JSON object with nested elements. ‘jq’ allows you to drill down into these nested structures using the dot notation.
echo '{"person":{"name":"John", "age":30, "city":"New York"}}' | jq '.person.name'
# Output:
# "John"
In this example, we’re extracting the ‘name’ from a nested ‘person’ object. The filter ‘.person.name’ navigates to the ‘person’ object and then extracts the ‘name’ value.
Parsing Arrays with ‘jq’
‘jq’ can also parse JSON arrays. Here’s an example:
echo '{"colors":["red", "green", "blue"]}' | jq '.colors[1]'
# Output:
# "green"
Here, we’re accessing the second element (index 1) of the ‘colors’ array. The filter ‘.colors[1]’ extracts the value at the specified index.
Dealing with Complex JSON using ‘json.tool’
‘json.tool’ can also handle complex JSON structures, but it primarily serves as a JSON pretty-printer. It’s not as versatile as ‘jq’ for extracting specific data from nested objects or arrays.
echo '{"person":{"name":"John", "age":30, "city":"New York"}}' | python -m json.tool
# Output:
# {
# "person": {
# "name": "John",
# "age": 30,
# "city": "New York"
# }
# }
In this example, ‘json.tool’ pretty-prints the nested JSON object, but it doesn’t provide a direct way to extract specific values like ‘jq’ does.
As you can see, ‘jq’ offers more advanced functionality for dealing with complex JSON structures in Bash. However, ‘json.tool’ can still be useful for validating and pretty-printing JSON data. As always, choose the tool that best fits your needs.
Expert-Level JSON Parsing in Bash: Exploring Alternatives
While ‘jq’ and ‘json.tool’ are popular choices for parsing JSON in Bash, there are alternative approaches that can offer different advantages. One such method is using Python’s ‘json’ module in a Bash script.
Leveraging Python’s ‘json’ Module
Python’s ‘json’ module provides methods to encode and decode JSON data. It can be used in a Bash script to parse JSON data in a more Pythonic way. Here’s an example:
echo '{"name":"John", "age":30, "city":"New York"}' | python -c "import sys, json; print(json.load(sys.stdin)['name'])"
# Output:
# John
In this example, we’re using Python’s ‘json’ module to parse a JSON string within a Bash script. The Python command takes a JSON object from stdin and uses ‘json.load’ to load the JSON data. It then extracts the ‘name’ value, resulting in the output ‘John’.
This approach can be especially beneficial if you’re already comfortable with Python and prefer its syntax over Bash. However, it does require Python to be installed and available on your system.
Weighing Your Options
Each method of parsing JSON in Bash has its strengths and weaknesses. ‘jq’ is powerful and flexible, making it ideal for complex JSON data. ‘json.tool’ is simpler and comes pre-installed with Python, making it a good choice for basic JSON parsing and pretty-printing. Python’s ‘json’ module offers a Pythonic approach to JSON parsing within Bash scripts, but it requires Python to be installed.
Ultimately, the best method depends on your specific needs, the complexity of your JSON data, and your comfort level with each tool. Experiment with each approach to find the one that works best for you.
Troubleshooting Common JSON Parsing Issues in Bash
When parsing JSON data in Bash, you might encounter a few common issues. Let’s discuss these potential problems and how to resolve them.
Handling Syntax Errors
JSON data must be properly formatted to be parsed correctly. A missing comma, an extra bracket, or a misplaced quote can lead to a syntax error.
Consider the following incorrect JSON data:
echo '{"name":"John" "age":30}' | jq '.name'
# Output:
# parse error: Expected separator between values at line 1, column 18
In this example, a comma is missing between the ‘name’ and ‘age’ properties, causing a syntax error. To resolve this, ensure your JSON data is correctly formatted.
Dealing with Missing Tools
If ‘jq’ or ‘json.tool’ is not installed on your system, you’ll encounter an error when trying to parse JSON data. Here’s what an error might look like if ‘jq’ is missing:
echo '{"name":"John", "age":30}' | jq '.name'
# Output:
# bash: jq: command not found
In this case, you’ll need to install the missing tool. For ‘jq’, you can do this with the following command:
sudo apt-get install jq
For ‘json.tool’, ensure that Python is installed on your system as it comes pre-packaged with Python.
Tips for Successful JSON Parsing in Bash
- Always validate your JSON data to ensure it’s correctly formatted.
- Make sure necessary tools like ‘jq’ or ‘json.tool’ are installed on your system.
- Use ‘jq’ for more complex operations and ‘json.tool’ for simpler JSON parsing and pretty-printing.
- Consider alternative methods like using Python’s ‘json’ module if they better suit your needs.
Understanding JSON Data and Bash Scripting
Before diving deeper into JSON parsing in Bash, let’s establish a solid understanding of the fundamental concepts: JSON data, Bash scripting, and the tools ‘jq’ and ‘json.tool’.
What is JSON?
JSON (JavaScript Object Notation) is a lightweight data-interchange format that is easy for humans to read and write, and easy for machines to parse and generate. It’s a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others.
A basic JSON structure looks something like this:
{
"name": "John",
"age": 30,
"city": "New York"
}
In this example, ‘name’, ‘age’, and ‘city’ are keys, and ‘John’, 30, and ‘New York’ are their respective values.
Bash Scripting Basics
Bash (Bourne Again Shell) is a shell and command language. It’s widely used for its ability to control job control, shell functions and scripts, command-line editing, and more. Bash scripts allow you to automate tasks on your Unix or Linux system.
A simple Bash script might look like this:
echo "Hello, world!"
# Output:
# Hello, world!
In this example, the ‘echo’ command is used to print the string ‘Hello, world!’ to the terminal.
Diving into ‘jq’ and ‘json.tool’
‘jq’ is a powerful command-line JSON processor. It can parse JSON data, allowing you to filter, map, and transform JSON structures. ‘json.tool’, on the other hand, is a module in Python that provides a simple way to pretty-print JSON data.
By understanding these fundamental concepts, you’ll be better equipped to parse JSON data in Bash effectively.
The Power of JSON Parsing in Bash
Parsing JSON in Bash isn’t just a theoretical exercise. It has practical applications in the real world, particularly when it comes to automation scripts and data processing.
JSON Parsing in Automation Scripts
Automation scripts are an integral part of modern system administration and DevOps. When these scripts need to interact with web APIs, they often have to deal with JSON data. Being able to parse this data in Bash can make your scripts more powerful and flexible.
# An example of an automation script interacting with a web API
response=$(curl -s 'https://api.example.com/data')
name=$(echo "$response" | jq '.name')
echo "The name is $name"
# Output:
# The name is John
In this example, the script makes a request to a web API and stores the response in a variable. It then uses ‘jq’ to parse the JSON data and extract the ‘name’ value.
Data Processing with JSON Parsing
Data comes in many forms, and JSON is one of the most common. Whether you’re processing log files, analyzing user behavior, or crunching numbers for a data science project, knowing how to parse JSON data in Bash can be a valuable skill.
# An example of a data processing script
data='{"users":[{"name":"John", "age":30}, {"name":"Jane", "age":25}]}'
users=$(echo "$data" | jq '.users[]')
echo "The users are:"
echo "$users"
# Output:
# The users are:
# {"name":"John", "age":30}
# {"name":"Jane", "age":25}
In this example, the script is processing a JSON string that contains user data. It uses ‘jq’ to parse the data and extract each user object.
Further Resources for Bash JSON Parsing Mastery
If you’re interested in diving deeper into JSON parsing in Bash, here are some resources that you might find helpful:
- The ‘jq’ Manual: A comprehensive guide to using ‘jq’, complete with examples and explanations for all of its features.
The Python ‘json’ Module Documentation: Detailed documentation for Python’s ‘json’ module, which you can use to parse JSON data in Bash.
The Bash Guide: An in-depth guide to Bash scripting, which can help you understand the context in which you’re parsing JSON data.
By understanding the real-world applications of JSON parsing in Bash and exploring these resources, you can take your Bash scripting skills to the next level.
Wrapping Up: Mastering JSON Parsing in Bash
In this comprehensive guide, we’ve explored the process of parsing JSON data in Bash, using various tools and techniques.
We began with the basics, learning how to parse JSON data using ‘jq’ and ‘json.tool’. We then delved into more complex scenarios, handling nested objects and arrays, and even exploring alternative methods like using Python’s ‘json’ module within a Bash script.
Along the way, we addressed common issues that you might encounter when parsing JSON in Bash, such as syntax errors and missing tools, providing you with solutions and workarounds for each problem.
We also compared the different methods of parsing JSON in Bash, giving you a sense of the strengths and weaknesses of each approach:
Method | Advantages | Disadvantages |
---|---|---|
‘jq’ | Powerful and flexible | Requires installation |
‘json.tool’ | Simple to use, pre-installed with Python | Less functionality than ‘jq’ |
Python’s ‘json’ module | Pythonic approach, ideal for Python users | Requires Python to be installed |
Whether you’re just starting out with JSON parsing in Bash or you’re looking to enhance your skills, we hope this guide has given you a deeper understanding of the process and its real-world applications.
Parsing JSON data in Bash is a valuable skill that can help you handle and manipulate data more effectively in your scripts. With this guide, you’re well on your way to mastering JSON parsing in Bash. Happy coding!