Overriding and Using the .toString() Method in Java
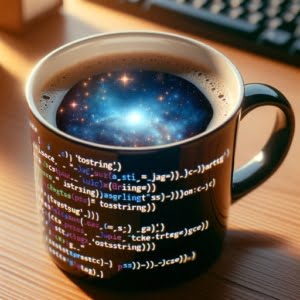
Are you finding it challenging to work with the toString() method in Java? You’re not alone. Many developers find themselves puzzled when it comes to using this method effectively.
Think of the toString() method in Java as a language interpreter. It can convert complex objects into a readable string format, making it a powerful tool for your Java toolkit.
This guide will walk you through the ins and outs of using the toString() method in Java, from the basics to more advanced techniques. We’ll cover everything from the fundamental use of the method to overriding it in user-defined classes, and even discuss alternative approaches.
So, let’s dive in and start mastering the toString() method in Java!
TL;DR: How Do I Use the toString() Method in Java?
The toString() method in Java is used to convert an object into a string, with the syntax: “. It’s a part of the Object class and can be overridden for user-defined classes to provide a meaningful string representation of an object.
Here’s a simple example:
class MyClass {
int x;
MyClass(int y) { x = y; }
public String toString() { return Integer.toString(x); }
}
MyClass myObj = new MyClass(10);
System.out.println(myObj.toString());
// Output:
// '10'
In this example, we’ve created a class MyClass
with an integer field x
. We’ve overridden the toString()
method to return the string representation of x
. When we create an object myObj
of MyClass
and print it using System.out.println(myObj.toString());
, it outputs ’10’.
This is a basic way to use the toString() method in Java, but there’s much more to learn about it. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Unveiling the Basic Use of toString() Method in Java
- Overriding toString() in User-Defined Classes
- Exploring Alternative Methods to Convert Objects to String in Java
- Troubleshooting Common Issues with toString()
- Diving Deeper into Java’s Object Class and Overriding Methods
- Exploring Beyond toString() Method in Java
- Wrapping Up: Mastering the toString() Method
Unveiling the Basic Use of toString() Method in Java
The toString()
method is an inbuilt method in Java that returns a string representation of an object. It’s typically used for debugging purposes, as it can easily convert the state of an object into a loggable string.
Let’s consider a simple example to understand how this method works.
public class Vehicle {
String color;
int speed;
Vehicle(String color, int speed) {
this.color = color;
this.speed = speed;
}
public String toString() {
return "Vehicle[color=" + color + ", speed=" + speed + "]";
}
}
public class Main {
public static void main(String[] args) {
Vehicle car = new Vehicle("Red", 100);
System.out.println(car.toString());
}
}
// Output:
// Vehicle[color=Red, speed=100]
In this code block, we’ve created a Vehicle
class with two properties: color
and speed
. We’ve overridden the toString()
method to return a string that describes the vehicle. When we create a Vehicle
object named car
and print it using System.out.println(car.toString());
, it outputs ‘Vehicle[color=Red, speed=100]’. This output gives us a clear, human-readable description of the car
object.
The toString()
method is powerful, but it’s also important to use it wisely. Overusing it can lead to performance issues, especially when dealing with large objects or complex data structures. It’s also worth noting that the default toString()
method in the Object class isn’t very helpful, as it only returns the class name and the object’s hash code. That’s why it’s common to override this method in your classes to provide more meaningful string representations.
Overriding toString() in User-Defined Classes
As we’ve seen, the toString()
method can be incredibly useful for converting objects into readable strings. But its real power emerges when you override this method in your own classes.
Overriding the toString()
method in user-defined classes allows you to provide a more meaningful string representation of your objects. Let’s consider an example to illustrate this.
public class Student {
String name;
int age;
Student(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Student[name=" + name + ", age=" + age + "]";
}
}
public class Main {
public static void main(String[] args) {
Student student = new Student("John", 20);
System.out.println(student.toString());
}
}
// Output:
// Student[name=John, age=20]
In this example, we’ve created a Student
class with two properties: name
and age
. We’ve overridden the toString()
method to return a string that describes the student. When we create a Student
object named student
and print it using System.out.println(student.toString());
, it outputs ‘Student[name=John, age=20]’.
This output provides a clear, human-readable description of the student
object, which is much more helpful than the default string representation provided by the toString()
method in the Object class.
When overriding the toString()
method, it’s a good practice to return a string that accurately represents the state of the object. This can make debugging easier and your code more understandable.
Exploring Alternative Methods to Convert Objects to String in Java
While the toString()
method is a popular choice for converting objects to strings in Java, there are alternative methods that can also get the job done. One such method is String.valueOf()
.
The String.valueOf()
method converts different types of values into string. It’s a static method that can be called directly using the class name and doesn’t throw a null pointer exception if the object is null.
Let’s illustrate this with an example:
public class Main {
public static void main(String[] args) {
Student student = null;
System.out.println(String.valueOf(student));
}
}
// Output:
// null
In this example, even though the student
object is null, String.valueOf(student)
doesn’t throw a null pointer exception and instead outputs ‘null’.
While String.valueOf()
is a handy method, it has its limitations. It doesn’t provide a meaningful string representation of user-defined objects unless you override the toString()
method in your classes.
Here’s a comparison of the toString()
method and the String.valueOf()
method:
Method | Advantages | Disadvantages |
---|---|---|
toString() | Provides a meaningful string representation of objects, Can be overridden in user-defined classes | Throws a null pointer exception if the object is null |
String.valueOf() | Doesn’t throw a null pointer exception if the object is null, Can convert different types of values into string | Doesn’t provide a meaningful string representation of user-defined objects unless the toString() method is overridden |
In conclusion, while toString()
and String.valueOf()
have their own advantages and disadvantages, they can both be effective for converting objects to strings in Java. Your choice between these methods will depend on your specific needs and circumstances.
Troubleshooting Common Issues with toString()
Like any other method in Java, using toString()
comes with its own set of challenges. Let’s discuss some common issues you might encounter and how to resolve them.
Dealing with Null Pointer Exceptions
One of the most common issues with toString()
is it can throw a Null Pointer Exception if the object is null. Here’s an example:
public class Main {
public static void main(String[] args) {
Student student = null;
System.out.println(student.toString());
}
}
// Output:
// Exception in thread "main" java.lang.NullPointerException
In this example, we’re trying to call toString()
on a null student
object, which results in a Null Pointer Exception.
To avoid this, you can check if the object is null before calling toString()
, like so:
public class Main {
public static void main(String[] args) {
Student student = null;
if (student != null) {
System.out.println(student.toString());
} else {
System.out.println("student is null");
}
}
}
// Output:
// student is null
Handling Complex Objects
Another issue arises when dealing with complex objects. The default toString()
method doesn’t provide a meaningful string representation for these objects. Overriding toString()
in your classes can help solve this problem.
public class ComplexObject {
// ... complex data structures ...
@Override
public String toString() {
// return a meaningful string representation of the object
}
}
In this example, we’re overriding toString()
in the ComplexObject
class to return a meaningful string representation of the object.
Remember, toString()
is a powerful tool, but it must be used wisely. Always check for null before calling toString()
and override toString()
in your classes to provide meaningful string representations of your objects.
Diving Deeper into Java’s Object Class and Overriding Methods
To fully grasp the toString()
method in Java, it’s crucial to understand the fundamentals of Java’s Object class and the concept of method overriding.
The Object Class in Java
In Java, the Object class is the root of the class hierarchy. Every class in Java is a direct or indirect subclass of the Object class, meaning that every Java object is an instance of this class or one of its subclasses.
The Object class provides a few standard methods that are available to all objects, including the toString()
method. The default toString()
method returns a string consisting of the name of the class, the ‘@’ symbol, and the unsigned hexadecimal representation of the hash code of the object.
Object obj = new Object();
System.out.println(obj.toString());
// Output:
// java.lang.Object@15db9742
In this code block, we’re calling toString()
on a new Object instance. The output is the default string representation provided by the Object class.
Overriding Methods in Java
In Java, a subclass can provide a specific implementation of a method that’s already provided by its parent class. This is known as method overriding. Overriding is done using the @Override
annotation.
When the toString()
method is called on an object, the JVM checks if the method has been overridden in the object’s class. If it has, the JVM uses the overridden method. If not, it uses the default method from the Object class.
The Importance of String Representation of Objects
Providing a meaningful string representation of your objects is crucial for debugging and logging purposes. It can help you understand the state of your objects at a glance, without having to inspect each field individually.
By overriding the toString()
method in your classes, you can provide a string representation that’s tailored to your needs and makes your code easier to understand and debug.
Exploring Beyond toString() Method in Java
The toString()
method in Java is not just a tool for converting objects to strings. It plays a vital role in several other areas of Java programming, including debugging and logging.
Debugging and Logging with toString()
When debugging or logging, it’s often necessary to print out the state of an object. The toString()
method provides a convenient way to get a readable string representation of an object, which can be extremely useful when you’re trying to figure out what’s going wrong in your code.
Exploring Related Concepts: equals() and hashCode() Methods
If you’re interested in delving deeper into Java’s Object class, two related methods you might want to explore are the equals()
and hashCode()
methods.
The equals()
method is used to compare two objects for equality. It checks if the two objects are the same object or if they are ‘equal’ in some class-specific way.
The hashCode()
method returns a hash code value for the object. This method is supported for the benefit of hash tables such as those provided by HashMap
.
Just like toString()
, the equals()
and hashCode()
methods can be overridden in your classes to provide class-specific behavior.
Further Resources for Mastering Java’s Object Class
There many other ways to handle Objects in Java. To learn more about this topic, Click Here for a complete guide on Casting Java Objects!
If you’re interested in learning more about the toString()
, equals()
, and hashCode()
methods, as well as other features of Java’s Object class, here are a few resources that you might find helpful:
- Java Char to String Conversion – Master converting char values to strings for enhanced string manipulation in Java.
JSON to Java Object – Effortlessly deserialize JSON data into Java objects for seamless integration.
Oracle’s Java Documentation – The official documentation for the Object class in Java and all the methods it provides.
GeeksforGeeks’ Java Library of Java tutorials and articles covers a wide range of topics.
Java Code Geeks offers a vast collection of Java tutorials and guides.
Wrapping Up: Mastering the toString() Method
In this comprehensive guide, we’ve explored the toString()
method in Java, a powerful tool for converting objects into readable strings.
We began with the basics, learning how to use the toString()
method in a simple Java class. We then delved into more advanced usage, such as overriding the toString()
method in user-defined classes to provide a meaningful string representation of objects.
Along the way, we tackled common issues you might encounter when using the toString()
method, such as null pointer exceptions and challenges with complex objects, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to converting objects to strings in Java, such as the String.valueOf()
method. Here’s a quick comparison of these methods:
Method | Advantages | Disadvantages |
---|---|---|
toString() | Provides a meaningful string representation of objects, Can be overridden in user-defined classes | Throws a null pointer exception if the object is null |
String.valueOf() | Doesn’t throw a null pointer exception if the object is null, Can convert different types of values into string | Doesn’t provide a meaningful string representation of user-defined objects unless the toString() method is overridden |
Whether you’re just starting out with the toString()
method in Java or you’re looking to deepen your understanding, we hope this guide has been a valuable resource.
The toString()
method is a powerful tool for any Java developer, and with this guide, you’re well equipped to use it effectively. Happy coding!