Java String Methods: From Beginner to Expert
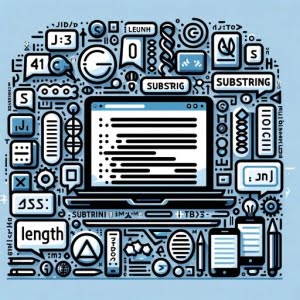
Are you finding it challenging to work with Java String methods? You’re not alone. Many developers, both beginners and experienced, often struggle with string manipulation in Java. But, think of the Java String class as a Swiss Army knife, offering a plethora of methods to manipulate strings effectively.
This guide will walk you through the most commonly used methods in the Java String class, from basic to advanced. We’ll explore the core functionality of these methods, delve into their advanced usage, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Java String methods!
TL;DR: How Do I Use Java String Methods?
Java provides a String class packed with many useful methods such as
length()
,charAt()
,substring()
,contains()
,equals()
, and more. These methods can be used to perform a variety of operations on strings.
Here’s a simple example of using the length()
method:
String str = "Hello";
int len = str.length();
System.out.println(len);
# Output:
# 5
In this example, we’ve created a string str
with the value ‘Hello’. We then use the length()
method to get the length of the string, which is 5. The length is then printed to the console.
This is just a basic way to use Java String methods, but there’s so much more to learn about manipulating and analyzing strings in Java. Continue reading for more detailed information and advanced usage examples.
Table of Contents
Exploring Basic Java String Methods
When it comes to manipulating strings in Java, the String class provides us with several powerful methods. Let’s take a closer look at some of these basic methods, their uses, and potential pitfalls.
Understanding the length()
Method
The length()
method returns the length of a specific string. Here’s how you can use it:
String str = "Hello World";
int len = str.length();
System.out.println(len);
# Output:
# 11
In this example, the string str
contains ‘Hello World’. When we apply the length()
method, it returns the length of the string, which is 11.
Working with the charAt()
Method
The charAt()
method returns the character at a specific index. Here’s an example:
String str = "Hello World";
char ch = str.charAt(0);
System.out.println(ch);
# Output:
# H
Here, we used charAt(0)
to get the first character of the string str
, which is ‘H’. Remember, string indexing starts from 0 in Java.
Using the substring()
Method
The substring()
method extracts a particular portion of a string. Let’s see it in action:
String str = "Hello World";
String subStr = str.substring(0, 5);
System.out.println(subStr);
# Output:
# Hello
In this example, substring(0, 5)
extracts the substring from index 0 to 4 (end index is exclusive), which gives us ‘Hello’.
Finding with the contains()
Method
The contains()
method checks if a string contains a specified sequence of characters. Here’s how to use it:
String str = "Hello World";
boolean result = str.contains("World");
System.out.println(result);
# Output:
# true
In this case, contains("World")
checks if ‘World’ is present in the string str
. It returns true, indicating that ‘World’ is indeed part of the string.
Comparing with the equals()
Method
The equals()
method compares two strings for equality. Let’s try it out:
String str1 = "Hello";
String str2 = "Hello";
boolean result = str1.equals(str2);
System.out.println(result);
# Output:
# true
Here, equals(str2)
compares str1
with str2
. It returns true, as both strings are indeed equal.
These are just a few of the basic Java String methods. Understanding and mastering these methods can significantly simplify your string manipulation tasks in Java. In the next section, we’ll explore some more advanced methods and their uses.
Diving Deeper: Advanced Java String Methods
After mastering the basic Java String methods, it’s time to take a step further. Let’s discuss some more complex methods that can help you carry out more advanced string manipulations.
The compareTo()
Method
The compareTo()
method compares two strings lexicographically, returning an integer based on the comparison. Let’s see it in action:
String str1 = "Apple";
String str2 = "Banana";
int result = str1.compareTo(str2);
System.out.println(result);
# Output:
# -1
In this case, compareTo(str2)
compares str1
with str2
. It returns -1, indicating that str1
is lexicographically less than str2
.
Locating with indexOf()
and lastIndexOf()
Methods
The indexOf()
method returns the index of the first occurrence of a specified character or substring, while lastIndexOf()
returns the index of the last occurrence. Let’s try them out:
String str = "Hello World, Hello Everyone";
int firstIndex = str.indexOf("Hello");
int lastIndex = str.lastIndexOf("Hello");
System.out.println(firstIndex);
System.out.println(lastIndex);
# Output:
# 0
# 13
Here, indexOf("Hello")
returns the index of the first occurrence of ‘Hello’, which is at the start of the string (index 0). On the other hand, lastIndexOf("Hello")
returns the index of the last occurrence of ‘Hello’, which is 13.
Replacing with the replace()
Method
The replace()
method replaces all occurrences of a specified character or substring with another. Here’s an example:
String str = "Hello World";
String newStr = str.replace("World", "Everyone");
System.out.println(newStr);
# Output:
# Hello Everyone
In this case, replace("World", "Everyone")
replaces all occurrences of ‘World’ with ‘Everyone’ in the string str
.
Splitting with the split()
Method
The split()
method splits a string into an array of substrings based on a specified delimiter. Let’s see how it works:
String str = "Hello,World,Hello,Everyone";
String[] parts = str.split(",");
for(String part : parts) {
System.out.println(part);
}
# Output:
# Hello
# World
# Hello
# Everyone
Here, split(",")
splits the string str
into an array of substrings at each comma. The resulting array is then printed to the console.
These advanced Java String methods can provide you with more control and flexibility when working with strings. By understanding these methods, you can write more efficient and cleaner code.
Exploring Alternatives: StringBuilder and StringBuffer
While the Java String class provides a wide range of methods for string manipulation, there are alternative approaches to consider, especially when dealing with large amounts of data or complex operations. Let’s dive into the StringBuilder and StringBuffer classes and see how they can help us manipulate strings more effectively.
StringBuilder: An Efficient Alternative
StringBuilder is a mutable sequence of characters. Its mutable nature makes it particularly useful when dealing with large strings or performing numerous modifications on a string.
Here’s a simple example of using StringBuilder to concatenate strings:
StringBuilder sb = new StringBuilder();
sb.append("Hello");
sb.append(" ");
sb.append("World");
System.out.println(sb.toString());
# Output:
# Hello World
In this example, we create a StringBuilder object sb
and append ‘Hello’, a space, and ‘World’ to it. The toString()
method is then used to convert the StringBuilder to a String for output.
StringBuffer: Thread-Safe String Manipulation
Similar to StringBuilder, StringBuffer is also a mutable sequence of characters. The key difference is that StringBuffer is thread-safe, meaning it can be used safely in multithreaded environments.
Here’s an example of using StringBuffer to reverse a string:
StringBuffer sb = new StringBuffer("Hello World");
sb.reverse();
System.out.println(sb.toString());
# Output:
# dlroW olleH
In this case, we create a StringBuffer object sb
with ‘Hello World’. We then call the reverse()
method to reverse the string.
While both StringBuilder and StringBuffer offer similar functionality, they each have their advantages and disadvantages. StringBuilder is typically faster and recommended for single-threaded environments, while StringBuffer, being thread-safe, is suitable for multithreaded environments.
By exploring these alternative approaches, you can choose the most efficient way to manipulate strings in Java based on your specific use-case.
Troubleshooting Java String Methods
While working with Java String methods, you may encounter certain issues or exceptions. Let’s discuss some of the most common ones and how to tackle them.
Dealing with NullPointerException
A NullPointerException
occurs when you try to invoke a method or property on a null object. Here’s an example:
String str = null;
int len = str.length();
# Output:
# Exception in thread "main" java.lang.NullPointerException
In this case, we’re trying to invoke the length()
method on a null string, which throws a NullPointerException
. To avoid this, always check if a string is null before invoking any methods on it.
Handling StringIndexOutOfBoundsException
A StringIndexOutOfBoundsException
is thrown when attempting to access an index beyond the length of the string or a negative index. Let’s see an example:
String str = "Hello";
char ch = str.charAt(10);
# Output:
# Exception in thread "main" java.lang.StringIndexOutOfBoundsException: String index out of range: 10
Here, we’re trying to access the character at index 10 of the string ‘Hello’, which only has 5 characters. This throws a StringIndexOutOfBoundsException
. To avoid this, ensure that the index you’re trying to access is within the length of the string.
Other Considerations
When working with string methods, also consider the following:
- Remember that strings are immutable in Java. Any operation that modifies a string will actually create a new string.
- Be mindful of the
equals()
and==
operator. Theequals()
method checks for value equality, while the==
operator checks for reference equality.
By being aware of these common issues and considerations, you can avoid many common pitfalls when working with Java String methods.
Unraveling the Java String Class
Before we delve deeper into Java String methods, it’s crucial to understand the fundamentals of the Java String class and the concept of string immutability.
The Java String Class
In Java, strings are represented as objects of the String class. The String class provides numerous methods to perform operations on strings such as comparing strings, searching characters or substrings, changing case, concatenating strings, and more.
Here’s a simple example of creating a string in Java:
String str = "Hello World";
System.out.println(str);
# Output:
# Hello World
In this example, we create a string str
with the value ‘Hello World’ and then print it to the console.
Immutability of Strings in Java
One of the key characteristics of the Java String class is that strings are immutable. This means that once a string is created, it cannot be changed. Any operation that seems to modify a string actually creates a new string.
Let’s see this in action:
String str1 = "Hello";
String str2 = str1;
str1 = str1 + " World";
System.out.println(str1);
System.out.println(str2);
# Output:
# Hello World
# Hello
In this example, we first create a string str1
with the value ‘Hello’ and assign it to str2
. We then append ‘ World’ to str1
. However, this operation does not modify the original ‘Hello’ string. Instead, it creates a new string ‘Hello World’ and assigns it to str1
. The str2
still points to the original ‘Hello’ string, demonstrating the immutability of strings in Java.
The Impact of Immutability on String Methods
The immutability of strings affects how string methods work in Java. Since strings cannot be changed, any string method that modifies a string will actually create a new string. This is important to remember when working with string methods as it can impact performance, especially when dealing with large amounts of data or performing numerous string operations.
Understanding these fundamentals of the Java String class and the concept of string immutability is crucial to effectively using and interpreting the results of Java String methods.
Java String Methods: The Bigger Picture
Java String methods are not just useful for basic string manipulations. Their relevance extends to larger projects and more complex tasks. Let’s explore how these methods play a role in broader contexts and related concepts you might want to explore.
String Methods in Data Parsing
Java String methods are often used in data parsing tasks. For instance, the split()
method can be used to parse CSV files, and the trim()
method can help clean up data by removing extra spaces.
String line = "John,Doe,25";
String[] data = line.split(",");
for(String datum : data) {
System.out.println(datum.trim());
}
# Output:
# John
# Doe
# 25
In this example, we use the split()
method to parse a line of CSV data into an array. We then use the trim()
method to remove any extra spaces around the data.
String Methods in File Handling
When working with files, Java String methods like replace()
, substring()
, and contains()
can be used to manipulate file paths, extract file extensions, or check if a path contains a specific directory.
Exploring Related Concepts
Beyond Java String methods, there are several related concepts that can further enhance your string manipulation skills. Regular expressions in Java, for instance, allow you to perform complex pattern matching and string manipulations. String formatting can help you create neatly formatted strings for output.
Further Resources for Mastering Java String Methods
To deepen your understanding of Java String methods and related concepts, consider exploring the following resources:
- Advanced Techniques for Using Strings in Java – Learn about the Java String class’s methods for substring comparison.
Using Matchers in Java – Master using Matcher for advanced pattern matching and text manipulation tasks in Java.
String Length in Java – Understand how to determine the length of a string in Java using built-in methods.
Java String Documentation – The official Java documentation on all String methods.
Java Regular Expressions Tutorial – A guide to using regular expressions in Java.
Java String Formatting Guide provides various examples of string formatting in Java.
By understanding the broader applications of Java String methods and exploring related concepts, you can take your Java skills to the next level.
Wrapping Up: Mastering Java String Methods
In this comprehensive guide, we’ve explored the fascinating world of Java String methods. These methods offer a robust toolkit for string manipulation, making it easier to perform everyday tasks and complex operations alike.
We began with the basics, understanding how to use fundamental Java String methods like length()
, charAt()
, substring()
, contains()
, and equals()
. Each of these methods plays a crucial role in string manipulation, providing the building blocks for more advanced operations.
We then ventured into more advanced territory, diving deeper into the Java String class. We explored methods like compareTo()
, indexOf()
, lastIndexOf()
, replace()
, and split()
, each offering more complex functionality for string manipulation. We also discussed alternative approaches, such as using the StringBuilder and StringBuffer classes for more efficient string handling.
Along the way, we tackled common issues that you might encounter when using Java String methods, such as NullPointerException
and StringIndexOutOfBoundsException
, providing solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Use Case | Pitfalls |
---|---|---|
Basic String Methods (length() , charAt() , etc.) | Basic string operations | None |
Advanced String Methods (compareTo() , indexOf() , etc.) | More complex string operations | May throw exceptions if used incorrectly |
StringBuilder and StringBuffer | Efficient string manipulation in large-scale or multithreaded applications | None |
Whether you’re just starting out with Java or looking to level up your string manipulation skills, we hope this guide has given you a deeper understanding of Java String methods and their capabilities.
With its balance of simplicity, flexibility, and power, Java’s String class is an indispensable tool for any programmer. Now, you’re well equipped to make the most of it. Happy coding!