Java Matcher Class: Your Guide to Identifying Patterns
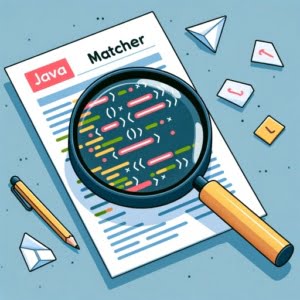
Are you finding it challenging to work with the Java Matcher class? You’re not alone. Many developers find themselves puzzled when it comes to handling pattern matching in Java, but we’re here to help.
Think of the Java Matcher class as a detective – it helps you find patterns in your data, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of mastering the Java Matcher class, from its basic usage to more advanced techniques. We’ll cover everything from the basics of pattern matching to more advanced techniques, as well as alternative approaches.
Let’s get started and start mastering the Java Matcher class!
TL;DR: What is the Java Matcher Class?
The Matcher class in Java is a powerful tool used for matching character sequences against a given pattern, instantiated with the syntax
Matcher matcher = pattern.matcher("patternToMatch");
. It’s a crucial part of the Java regular expression API and plays a significant role in pattern matching and data validation tasks.
Here’s a simple example:
Pattern pattern = Pattern.compile("abc");
Matcher matcher = pattern.matcher("abcdef");
boolean matchFound = matcher.find();
// Output:
// true
In this example, we create a Pattern
object with the pattern "abc"
. We then create a Matcher
object by invoking the matcher
method on our Pattern
object. The matcher
method takes in the string "abcdef"
that we want to search for our pattern in. Finally, we call the find
method on our Matcher
object to check if our pattern is found in the string. The find
method returns true
because our pattern "abc"
is found in the string "abcdef"
.
This is a basic way to use the Matcher class in Java, but there’s much more to learn about pattern matching and the advanced usage of the Matcher class. Continue reading for a more detailed understanding of the Matcher class and its advanced usage.
Table of Contents
- Basic Usage of Java Matcher
- Advanced Techniques with Java Matcher
- Exploring Alternative Approaches
- Overcoming Obstacles with Java Matcher
- Understanding Regular Expressions
- The Relationship Between Pattern and Matcher
- Broader Concepts
- Applying Java Matcher in Real-World Projects
- Accompanying Classes and Methods
- Wrapping Up: Mastering the Java Matcher Class
Basic Usage of Java Matcher
The Matcher class in Java is a part of the java.util.regex package and it works in conjunction with the Pattern class for pattern matching operations on text using regular expressions.
Understanding the Pattern and Matcher Classes
Firstly, we create a Pattern
object which defines the regular expression we want to search for. The Pattern
class has a method called compile(String regex)
which compiles the given regular expression into a pattern.
Next, we use the Pattern
object to create a Matcher
object. This is done using the matcher(CharSequence input)
method in the Pattern
class. This method creates a matcher that will match the given input against the pattern.
Pattern pattern = Pattern.compile("abc");
Matcher matcher = pattern.matcher("abcdefabc");
In this code block, we’re looking for the pattern "abc"
in the string "abcdefabc"
.
Finding Matches with Matcher
Once we have our Matcher
object, we can use various methods to find matches and retrieve the matched segments. The find()
method is commonly used to find the next match in the input sequence.
while (matcher.find()) {
System.out.println("Found match at: " + matcher.start() + " to " + matcher.end());
}
// Output:
// Found match at: 0 to 3
// Found match at: 6 to 9
In this example, find()
is used in a while loop to find all matches in the input sequence. For each match, it prints the start and end indices of the match in the input sequence.
The Matcher class offers a powerful and flexible way to search for patterns in text. However, it’s important to remember that the find()
method will only find non-overlapping matches. For overlapping matches, you’ll need to use different techniques, which we’ll cover in the advanced usage section.
In the next section, we’ll delve into more complex uses of the Matcher class, exploring different methods and their applications.
Advanced Techniques with Java Matcher
As you become more comfortable with the Matcher class, you’ll find there are several methods that allow for more complex pattern matching. Let’s explore three of these methods: matches()
, lookingAt()
, and find()
.
The matches() Method
The matches()
method attempts to match the entire input sequence against the pattern.
Pattern pattern = Pattern.compile("abc");
Matcher matcher = pattern.matcher("abcdef");
boolean matchFound = matcher.matches();
// Output:
// false
In this example, matches()
returns false
because it tries to match the entire string "abcdef"
against the pattern "abc"
, which is not a complete match.
The lookingAt() Method
The lookingAt()
method attempts to match the input sequence, starting at the beginning, against the pattern.
Pattern pattern = Pattern.compile("abc");
Matcher matcher = pattern.matcher("abcdef");
boolean matchFound = matcher.lookingAt();
// Output:
// true
Here, lookingAt()
returns true
because the beginning of the string "abcdef"
matches the pattern "abc"
.
The find() Method Revisited
We’ve already discussed the find()
method, but it’s worth revisiting. Unlike matches()
and lookingAt()
, find()
doesn’t require the match to be at the beginning of the string. It can find the pattern anywhere in the string.
Pattern pattern = Pattern.compile("abc");
Matcher matcher = pattern.matcher("abcdefabc");
while (matcher.find()) {
System.out.println("Found match at: " + matcher.start() + " to " + matcher.end());
}
// Output:
// Found match at: 0 to 3
// Found match at: 6 to 9
In this example, find()
finds two matches for the pattern "abc"
in the string "abcdefabc"
.
Each of these methods has its uses, and understanding which one to use in a given situation can greatly enhance your pattern matching abilities with the Matcher class. In the next section, we’ll discuss alternative approaches and when they might be more appropriate.
Exploring Alternative Approaches
While the Java Matcher class is a powerful tool for pattern matching, there are other methods and classes that can be used for similar tasks. Let’s explore some of these alternatives.
Using Pattern.split()
The Pattern.split(CharSequence input)
method splits the given input sequence around matches of the pattern.
Pattern pattern = Pattern.compile("a");
String[] result = pattern.split("abcabc");
for (String str : result) {
System.out.println(str);
}
// Output:
// ''
// 'bc'
// 'bc'
In this example, the input string "abcabc"
is split around matches of the pattern "a"
. The split()
method returns an array of strings computed by splitting the input around matches of the pattern.
Using String.matches()
The String.matches(String regex)
method tells whether or not the string matches the given regular expression.
boolean matchFound = "abcdef".matches("abc.*");
// Output:
// true
In this example, matches()
returns true
because the string "abcdef"
matches the regular expression "abc.*"
.
Using Apache Commons Lang
Third-party libraries like Apache Commons Lang offer utilities for working with regular expressions. For example, StringUtils.containsAny(CharSequence sequence, CharSequence... searchSequences)
checks if any of the searchSequences
are found in the sequence
.
boolean matchFound = StringUtils.containsAny("abcdef", "abc", "xyz");
// Output:
// true
In this example, containsAny()
returns true
because the sequence "abcdef"
contains the search sequence "abc"
.
These alternative approaches can be more suitable in certain scenarios. For example,
Pattern.split()
can be used for tokenizing a string,String.matches()
can be used for simple pattern matching tasks, and Apache Commons Lang can be used for more complex pattern matching tasks. Understanding these alternatives and when to use them can greatly enhance your pattern matching abilities.
Overcoming Obstacles with Java Matcher
While using the Matcher class, you might encounter some common errors or obstacles. Let’s discuss these issues and their solutions, along with some best practices for optimization.
Dealing with No Match Found Exception
A common error occurs when you try to use match information methods (like start()
, end()
, group()
) without first ensuring that a match exists. This will result in a IllegalStateException
.
try {
Pattern pattern = Pattern.compile("abc");
Matcher matcher = pattern.matcher("def");
System.out.println(matcher.group());
} catch (IllegalStateException e) {
System.out.println("No match found.");
}
// Output:
// No match found.
In this example, we’re trying to get the matched group before calling a method like find()
or matches()
to ensure a match exists. To avoid this error, always ensure a match exists before trying to retrieve match information.
Considerations for Pattern Complexity
The complexity of your pattern can significantly impact the performance of your matching operation. Avoid overly complex patterns with excessive quantifiers or unnecessary groups. If performance is a concern, consider using simpler patterns or alternative methods for string processing.
Using find() Correctly
Remember that the find()
method resets its internal state each time it’s called. If you call find()
again after finding a match, it will continue searching from where it left off, not from the beginning of the input sequence.
Pattern pattern = Pattern.compile("abc");
Matcher matcher = pattern.matcher("abcdefabc");
if (matcher.find()) {
System.out.println("Found match at: " + matcher.start() + " to " + matcher.end());
}
if (matcher.find()) {
System.out.println("Found match at: " + matcher.start() + " to " + matcher.end());
}
// Output:
// Found match at: 0 to 3
// Found match at: 6 to 9
In this example, we call find()
twice. Each call to find()
continues searching from where the last match ended, not from the beginning of the string.
Understanding these common obstacles and their solutions, along with these considerations, can help you use the Matcher class more effectively and efficiently.
Understanding Regular Expressions
Regular expressions, often abbreviated as regex, are sequences of characters that form a search pattern. These patterns are used to match character combinations in strings, making them a crucial tool in text processing.
In Java, regular expressions are implemented through the Pattern
and Matcher
classes in the java.util.regex
package. The Pattern
class encapsulates the compiled version of a regular expression, while the Matcher
class interprets the pattern and performs match operations on a character sequence.
Pattern pattern = Pattern.compile("abc");
Matcher matcher = pattern.matcher("abcdefabc");
while (matcher.find()) {
System.out.println("Found match at: " + matcher.start() + " to " + matcher.end());
}
// Output:
// Found match at: 0 to 3
// Found match at: 6 to 9
In this example, we’re creating a Pattern
object with the pattern "abc"
, then creating a Matcher
object from that pattern. The find()
method is used in a loop to find all occurrences of the pattern in the input string.
The Relationship Between Pattern and Matcher
The Pattern
and Matcher
classes work closely together to perform regex operations. The Pattern
class represents the regular expression, while the Matcher
class uses a Pattern
object to perform matching operations on an input string.
It’s important to note that a Matcher
object can only work with one pattern and one input sequence. If you need to match against a different pattern or on a different input sequence, you’ll need to create a new Matcher
object.
Broader Concepts
Understanding regular expressions and the Pattern
and Matcher
classes is just the tip of the iceberg when it comes to text processing in Java. Other related classes, like String
, StringBuilder
, and StringBuffer
, offer additional methods for manipulating and processing text.
Furthermore, third-party libraries like Apache Commons Lang and Google Guava provide even more powerful tools for text processing. But no matter what tools you use, the fundamental concepts of pattern matching and regular expressions remain the same.
Applying Java Matcher in Real-World Projects
The Java Matcher class is not just a theoretical concept, but a practical tool that can be used in various real-world applications.
Data Validation
One of the most common uses of the Matcher class is in data validation. By defining a pattern for valid data, you can use a Matcher to check if a given input matches the pattern. This can be used, for example, to validate user inputs such as email addresses, phone numbers, or passwords.
Pattern pattern = Pattern.compile("^[a-zA-Z0-9._%-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,6}$");
Matcher matcher = pattern.matcher("[email protected]");
if (matcher.matches()) {
System.out.println("Valid email address.");
} else {
System.out.println("Invalid email address.");
}
// Output:
// Valid email address.
In this example, we use a regular expression to define a pattern for a valid email address. The Matcher then checks if the input string matches this pattern.
Text Parsing
The Matcher class can also be used for text parsing tasks. For example, you can use it to extract specific information from a larger text, such as finding all URLs in a webpage.
Pattern pattern = Pattern.compile("(http://|https://)[a-zA-Z0-9.-]+(/[a-zA-Z0-9.%&=]*)?");
Matcher matcher = pattern.matcher("Visit us at http://www.example.com or at https://blog.example.com");
while (matcher.find()) {
System.out.println("URL found: " + matcher.group());
}
// Output:
// URL found: http://www.example.com
// URL found: https://blog.example.com
In this example, we define a pattern for URLs and use the Matcher to find all URLs in a text.
Accompanying Classes and Methods
The Matcher class often doesn’t work alone. It’s usually used together with other classes and methods from the java.util.regex
package or even from third-party libraries. For example, the Pattern
class is almost always used together with the Matcher class. Other related classes include PatternSyntaxException
and MatchResult
.
Further Resources for Mastering Java Matcher
To continue your journey in mastering the Java Matcher class and related topics, here are some resources that offer more in-depth information:
- Mastery Step-by-Step: Strings in Java – Discover techniques for encoding and decoding strings in Java.
Java Pattern Basics – Understand how to create Pattern objects and use them for pattern matching operations.
Java String Functions – Learn about various string operations such as concatenation, and substring extraction.
Oracle’s Java Documentation on Regular Expressions – Direct insights on working with regular expressions in Java.
Baeldung’s Guide on Java Regex explores navigating regular expressions in Java.
GeeksforGeeks’ Java Regex Tutorial teaches how to implement Java regular expressions efficiently.
These resources offer comprehensive guides on Java’s regular expressions, including the Matcher and Pattern classes, and provide a deeper understanding of their usage and applications.
Wrapping Up: Mastering the Java Matcher Class
In this comprehensive guide, we’ve delved into the depths of the Java Matcher class, a powerful tool for pattern matching in Java.
We began with the basics, understanding how to use the Matcher class in conjunction with the Pattern class to find patterns in data. We then explored more advanced techniques, such as using different methods like matches()
, lookingAt()
, and find()
, each with its own unique use case.
We also discussed alternative approaches, such as using Pattern.split()
, String.matches()
, or third-party libraries like Apache Commons Lang. Each of these alternatives offers unique advantages and can be more suitable in certain scenarios.
Along the way, we tackled common obstacles and their solutions when using the Matcher class, providing tips for best practices and optimization. We also delved into the background and fundamentals of regular expressions, the relationship between the Pattern and Matcher classes, and broader concepts related to text processing in Java.
Here’s a quick comparison of the methods we’ve discussed:
Method | Uses | Pros | Cons |
---|---|---|---|
matches() | Matches the entire input sequence | Precise | Not flexible |
lookingAt() | Matches the beginning of the input sequence | Flexible | Limited scope |
find() | Finds the pattern anywhere in the sequence | Most flexible | Might require more processing power |
Whether you’re just starting out with the Matcher class or looking to level up your pattern matching skills, we hope this guide has given you a deeper understanding of the Matcher class and its capabilities.
With its balance of flexibility, power, and precision, the Matcher class is a valuable tool for pattern matching in Java. Now, you’re well equipped to harness its power. Happy coding!