Using ‘If’ Statements in Bash | Scripting Syntax Guide
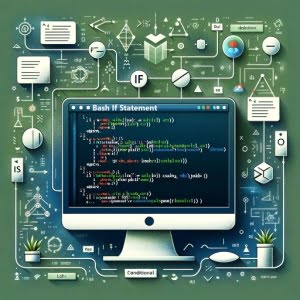
Are you finding it challenging to use if statements in Bash? You’re not alone. Many developers find themselves in a maze when it comes to controlling the flow of their Bash scripts.
Think of Bash if statements as traffic cops, directing the flow of your script just like traffic cops control the flow of vehicles on the road. They are a powerful tool to control what happens in your script and when.
In this guide, we’ll walk you through the process of using if statements in Bash, from the basics to more advanced techniques. We’ll cover everything from writing a simple if statement, using else and elif, to more complex scenarios like nested if statements.
So, let’s dive in and start mastering Bash if statements!
TL;DR: How Do I Use an If Statement in Bash?
In Bash, you use
if
statements to control the flow of your script, with the syntaxif [ {condition} ]; then {codeToExecute}
. They allow you to make decisions in your code based on conditions.
Here’s a simple example:
a=5
if [ "$a" -lt 10 ]; then
echo "Variable a is less than 10"
fi
# Output:
# Variable a is less than 10
In this example, we’ve created a variable a
and assigned it a value of 5. The if statement checks if the value of a
is less than 10. If the condition is true, which it is in this case, it echoes the string “Variable a is less than 10”.
This is a basic way to use if statements in Bash, but there’s much more to learn about controlling the flow of your scripts. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with Bash If Statements
If statements in Bash are essential for controlling the flow of your script. They allow your script to make decisions based on certain conditions. Here’s a simple example:
name="Anton"
if [ "$name" = "Anton" ]; then
echo "Hello, Anton!"
fi
# Output:
# Hello, Anton!
In this example, we’ve set a variable name
to the string “Anton”. The if statement then checks if the value of name
is equal to “Anton”. If the condition is true, it echoes the string “Hello, Anton!”.
The key elements of this if statement are:
- The
if
keyword that begins the statement. - The condition in square brackets
[]
. - The
then
keyword that indicates the start of the code to be executed if the condition is true. - The
fi
keyword that ends the if statement.
This is the basic usage of if statements in Bash. They can be used to compare numbers, strings, and files, among other things. For example, you can check if a file exists and is readable, if a string is null, or if one number is greater than another.
Understanding the basic use of if statements is the first step in mastering Bash script control flow. In the next section, we’ll explore more complex uses of if statements.
Expanding Your Knowledge: Nested If Statements and Elif
As you become more comfortable with Bash if statements, you can start exploring more complex uses. Two such advanced techniques are using else
and elif
(short for ‘else if’), and creating nested if statements.
Using Else and Elif
The else
clause in an if statement lets you specify code that will be executed if the condition is false. Here’s an example:
num=15
if [ "$num" -lt 10 ]; then
echo "Number is less than 10"
else
echo "Number is greater than or equal to 10"
fi
# Output:
# Number is greater than or equal to 10
In this example, the else
clause is executed because the condition $num -lt 10
is false.
The elif
clause allows you to specify additional conditions to check if the initial if condition is false.
num=15
if [ "$num" -lt 10 ]; then
echo "Number is less than 10"
elif [ "$num" -lt 20 ]; then
echo "Number is less than 20"
else
echo "Number is greater than or equal to 20"
fi
# Output:
# Number is less than 20
In this example, the elif
clause is executed because the initial if condition $num -lt 10
is false, but the elif
condition $num -lt 20
is true.
Exploring Nested If Statements
Nested if statements are if statements within if statements. They allow you to check multiple conditions and execute different code blocks based on those conditions. Here’s an example:
num=15
if [ "$num" -gt 10 ]; then
if [ "$num" -lt 20 ]; then
echo "Number is between 10 and 20"
fi
fi
# Output:
# Number is between 10 and 20
In this example, the inner if statement is only checked if the outer if statement’s condition is true. This allows us to confirm that the number is between 10 and 20.
Understanding these advanced techniques will give you more control over your Bash scripts and allow you to write more complex and flexible code.
Exploring Alternative Control Flow Techniques
While if statements are a fundamental part of Bash scripting, they are not the only way to control the flow of your scripts. Two powerful alternatives are case
statements and the ?:
ternary operator.
Using Case Statements in Bash
A case
statement is a powerful tool for matching several specific values. It’s similar to a series of if-elif-else statements, but can be more concise and easier to read. Here’s an example:
animal="dog"
case "$animal" in
"cat") echo "Furry and aloof"
;;
"dog") echo "Friendly and loyal"
;;
*) echo "Unknown animal"
;;
esac
# Output:
# Friendly and loyal
In this example, the case
statement checks the value of the animal
variable. If it’s “cat”, it echoes “Furry and aloof”; if it’s “dog”, it echoes “Friendly and loyal”. The *)
case is a catch-all that matches anything not caught by the other cases.
Leveraging the Ternary Operator
The ?:
ternary operator is a compact way to write simple if-else statements. It takes three arguments: a condition, a result for if the condition is true, and a result for if the condition is false. Here’s an example:
num=15
[ "$num" -lt 20 ] && echo "Number is less than 20" || echo "Number is greater than or equal to 20"
# Output:
# Number is less than 20
In this example, the condition is $num -lt 20
. If it’s true, it echoes “Number is less than 20”; if it’s false, it echoes “Number is greater than or equal to 20”.
While these alternative methods can be useful, it’s important to choose the right tool for the job. If statements are simple and versatile, making them a good choice for many situations. However, for more complex scenarios, case
statements and the ?:
ternary operator can provide more concise and readable solutions.
Troubleshooting Common Bash If Statement Issues
Even the most experienced Bash scripters run into issues when using if statements. Let’s discuss some common problems, their solutions, and best practices to avoid these issues.
Syntax Errors
One of the most common issues when writing if statements in Bash is syntax errors. These can be caused by missing or misplaced keywords, brackets, or spaces.
For example, consider the following code:
num=15
if ["$num" -lt 20]
then
echo "Number is less than 20"
fi
# Output:
# bash: [: missing `]'
This script results in a syntax error because there are no spaces between the brackets and the condition. In Bash, spaces are crucial in if statements. The correct syntax would be [ "$num" -lt 20 ]
.
Unexpected Behavior
Another common issue is unexpected behavior, which can be caused by misunderstandings about how Bash evaluates conditions. For example, consider the following script:
str=""
if [ "$str" ]; then
echo "Non-empty string"
else
echo "Empty string"
fi
# Output:
# Empty string
This script might surprise you by outputting “Empty string”. This is because in Bash, a non-empty string evaluates to true, while an empty string or null value evaluates to false.
Best Practices
To avoid these issues, follow these best practices:
- Always place spaces around your conditions within brackets.
- Use double quotes around variables to prevent word splitting and pathname expansion.
- Understand how Bash evaluates conditions, especially when dealing with strings and null values.
By understanding these common issues and best practices, you can write more robust and reliable Bash if statements.
Understanding Control Flow in Scripting
Control flow is a fundamental concept in scripting and programming. It refers to the order in which the individual statements, instructions, or function calls of an imperative or a declarative program are executed or evaluated.
In Bash scripting, control flow is primarily managed using conditional statements, loops, and function calls. Among these, if statements play a crucial role in decision making.
The Power of If Statements
If statements in Bash, and in programming in general, allow your script to make decisions based on conditions. They form the backbone of any complex script and provide flexibility in handling different situations.
Consider a simple real-world scenario: If it’s raining, you’ll take an umbrella; otherwise, you won’t. This decision-making process is what if statements bring to your Bash scripts.
Here’s an example:
weather="rain"
if [ "$weather" = "rain" ]; then
echo "Take an umbrella"
else
echo "No need for an umbrella"
fi
# Output:
# Take an umbrella
In this script, we have a variable weather
that holds the string “rain”. The if statement checks if weather
equals “rain”. If it’s true, it echoes “Take an umbrella”; otherwise, it echoes “No need for an umbrella”.
This is a simple demonstration of how if statements control the flow of a script based on conditions. In more complex scripts, you could have multiple if statements, nested if statements, and if statements combined with loops and functions.
Understanding control flow and the role of if statements is essential for writing effective Bash scripts. It allows you to create scripts that can handle a wide range of scenarios and conditions.
Broadening Your Bash Horizons
If statements in Bash are not just for small scripts. They are an integral part of larger Bash projects, helping to control the flow of execution and making your scripts dynamic and responsive to different conditions.
Applying If Statements in Larger Scripts
In larger Bash scripts, you might find yourself using if statements to control which functions are called and when, to validate input, or to handle errors. For instance, you could write a script that takes a filename as an argument and uses an if statement to check if the file exists before attempting to open it.
filename="$1"
if [ -f "$filename" ]; then
cat "$filename"
else
echo "File does not exist"
fi
# Output:
# Content of the file if it exists
# File does not exist if it doesn't
In this script, $1
is the first command-line argument, which is the filename. The if statement uses the -f
test to check if the file exists and is a regular file. If it is, the script displays its content using the cat
command. Otherwise, it prints a message saying the file does not exist.
Expanding Your Knowledge: Loops and Debugging
Once you’re comfortable with if statements, you can explore other control structures in Bash, like loops. Loops allow you to repeat a block of code multiple times, which is useful for tasks like processing the lines in a file or the elements in an array.
Another valuable skill is debugging. Bash provides several options for debugging scripts, including the -v
(verbose) and -x
(xtrace) options, which display detailed information about what the script is doing.
Further Resources for Bash Proficiency
Ready to dive deeper into Bash scripting? Here are some resources that can help you expand your knowledge:
- Advanced Bash-Scripting Guide: This guide goes beyond the basics to explore topics like arrays, string manipulation, and process management.
Bash Scripting if Statement: This GeeksforGeeks article delves into the if statement in Bash scripting.
Bash Pitfalls: This page lists common mistakes and misconceptions about Bash scripting, and how to avoid them.
Wrapping Up: Mastering Bash If Statements
In this comprehensive guide, we’ve delved into the world of Bash if statements, a fundamental tool for controlling the flow of your Bash scripts.
We started with the basics, learning how to write a simple if statement and understand its syntax. We then moved on to more advanced techniques, exploring the use of else
and elif
clauses and nested if statements. We also discussed common issues you might encounter when writing if statements in Bash, such as syntax errors and unexpected behavior, and provided solutions and best practices to avoid these pitfalls.
We didn’t stop at if statements. We also looked at alternative ways to control the flow of your scripts, such as case
statements and the ?:
ternary operator. These methods can provide more concise and readable solutions in some scenarios, although if statements remain a versatile and powerful tool.
Control Flow Method | Flexibility | Complexity | Versatility |
---|---|---|---|
If Statements | High | Low-Moderate | High |
Case Statements | Moderate | Moderate | Moderate |
Ternary Operator | Low | High | Low |
Whether you’re a beginner just starting out with Bash scripting or an experienced developer looking to refine your skills, we hope this guide has helped you to deepen your understanding of if statements and control flow in Bash.
With the knowledge you’ve gained from this guide, you’re well-equipped to write robust and efficient Bash scripts. Remember, practice is key when it comes to mastering any programming concept. So, keep scripting, keep experimenting, and most importantly, have fun while doing it. Happy scripting!