Java Pattern Class: Mastering Guide and Examples
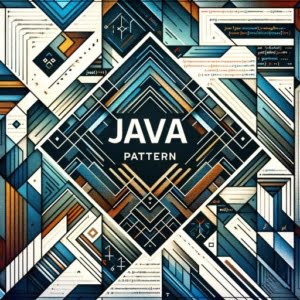
Ever felt like you’re wrestling with understanding the Java Pattern class? You’re not alone. Many developers find the Java Pattern class a bit tricky. Think of the Java Pattern class as a detective – it helps you find patterns in your data, making it an extremely powerful tool for tasks like data validation and text processing.
In this guide, we’ll walk you through the process of using the Java Pattern class, from the basics to more advanced techniques. We’ll cover everything from defining a pattern using the Pattern class, creating a Matcher object, to handling complex patterns and even troubleshooting common issues.
So, let’s dive in and start mastering the Java Pattern class!
TL;DR: What is the Java Pattern Class?
The Java Pattern class is a part of the java.util.regex package and is used to define a pattern for the regex engine. It’s a powerful tool that allows you to match sequences of characters in strings.
Here’s a simple example:
import java.util.regex.*;
Pattern pattern = Pattern.compile("\\w+");
Matcher matcher = pattern.matcher("Hello, Java Pattern!");
while (matcher.find()) {
System.out.println("Match: " + matcher.group());
}
# Output:
# Match: Hello
# Match: Java
# Match: Pattern
In this example, we create a Pattern object with the pattern ‘\w+’, which matches one or more word characters. We then create a Matcher object from this pattern and apply it to the string ‘Hello, Java Pattern!’. The find()
method of the Matcher class is used in a loop to find all matches, which are then printed out.
This is just a basic usage of the Java Pattern class. Keep reading for a more detailed guide, including advanced techniques and examples.
Table of Contents
- Defining a Pattern with the Java Pattern Class
- Creating a Matcher Object
- Finding Matches
- Advanced Pattern Matching with Java
- Using the Matcher Class
- Exploring Alternative Approaches
- Troubleshooting Common Errors in Java Pattern Matching
- Best Practices and Optimization
- Understanding Regular Expressions in Java
- Expanding the Use of Java Pattern Class
- Wrapping Up: Mastering Java Pattern Class
Defining a Pattern with the Java Pattern Class
The first step in using the Java Pattern class is to define a pattern. This pattern will be a specific sequence of characters that you want to find in a string. The pattern is defined as a string and compiled into a Pattern object using the Pattern.compile()
method. Here’s how you can do this:
import java.util.regex.*;
String regex = "\\w+";
Pattern pattern = Pattern.compile(regex);
In this code snippet, we define a pattern that matches one or more word characters. The \\w+
is a regular expression that means ‘one or more word characters’. A word character in regex is any letter, numeric digit, or the underscore character.
Creating a Matcher Object
Once you have a Pattern object, you can create a Matcher object that can match the pattern in a specific string. Here’s an example:
String input = "Hello, Java Pattern!";
Matcher matcher = pattern.matcher(input);
In this code, we create a Matcher object that will match the pattern we defined earlier against the string ‘Hello, Java Pattern!’.
Finding Matches
To find matches in the string, you can use the matcher.find()
method in a loop. Here’s a full example:
import java.util.regex.*;
String regex = "\\w+";
Pattern pattern = Pattern.compile(regex);
String input = "Hello, Java Pattern!";
Matcher matcher = pattern.matcher(input);
while (matcher.find()) {
System.out.println("Match: " + matcher.group());
}
# Output:
# Match: Hello
# Match: Java
# Match: Pattern
In this example, the matcher.find()
method returns true as long as there is a next match in the string, and false when there are no more matches. The matcher.group()
method returns the actual matched text. So, this code prints out each word in the string ‘Hello, Java Pattern!’.
Advanced Pattern Matching with Java
As you become more familiar with the Java Pattern class, you can start to explore more complex patterns. This involves using a variety of special characters and constructs in your regular expressions.
For example, you can use the .
character to match any character, the *
character to match zero or more of the preceding character, and the +
character to match one or more of the preceding character. Let’s see how this works in a code example:
import java.util.regex.*;
String regex = "H.*o";
Pattern pattern = Pattern.compile(regex);
String input = "Hello, Java Pattern!";
Matcher matcher = pattern.matcher(input);
while (matcher.find()) {
System.out.println("Match: " + matcher.group());
}
# Output:
# Match: Hello
In this code, the pattern H.*o
matches any sequence of characters that starts with ‘H’ and ends with ‘o’. The .*
in the pattern means ‘any character (.) zero or more times (*)’. So, it matches the string ‘Hello’.
Using the Matcher Class
The Matcher class provides a number of methods that you can use to find matches and retrieve information about the matches. For example, you can use the start()
and end()
methods to get the start and end indices of a match. Here’s an example:
import java.util.regex.*;
String regex = "\\w+";
Pattern pattern = Pattern.compile(regex);
String input = "Hello, Java Pattern!";
Matcher matcher = pattern.matcher(input);
while (matcher.find()) {
System.out.println("Match: " + matcher.group() + ", Start: " + matcher.start() + ", End: " + matcher.end());
}
# Output:
# Match: Hello, Start: 0, End: 5
# Match: Java, Start: 7, End: 11
# Match: Pattern, Start: 13, End: 20
In this code, the matcher.start()
method returns the start index of the current match, and the matcher.end()
method returns the index of the character following the last character of the match. So, this code prints out each word in the string ‘Hello, Java Pattern!’, along with the start and end indices of the word in the string.
Exploring Alternative Approaches
While the Java Pattern class is a powerful tool for pattern matching, it’s not the only way to use regular expressions in Java. In fact, the String class itself provides a method called matches()
that you can use to match a regular expression against the string.
Using String’s Matches Method
Here’s an example:
String input = "Hello, Java Pattern!";
boolean matchFound = input.matches(".*Java.*");
System.out.println("Match found: " + matchFound);
# Output:
# Match found: true
In this code, the matches()
method returns true if the string matches the regular expression .*Java.*
, which matches any string that contains ‘Java’.
Benefits and Drawbacks
The matches()
method is very simple and convenient to use. However, it’s not as powerful or flexible as the Java Pattern class. For example, it doesn’t provide methods to find multiple matches, get the start and end indices of a match, or replace matches.
Making the Right Choice
When deciding whether to use the Java Pattern class or the String’s matches()
method, consider the complexity of your pattern matching needs. If you just need to check whether a string matches a simple pattern, the matches()
method may be sufficient. But if you need to find multiple matches, get detailed information about the matches, or manipulate the matches, the Java Pattern class is likely to be a better choice.
Troubleshooting Common Errors in Java Pattern Matching
While using the Java Pattern class, you might encounter some common errors or obstacles. In this section, we’ll go over these issues and provide solutions.
PatternSyntaxException
One of the most common errors you might encounter is a PatternSyntaxException
. This exception is thrown when the regular expression’s syntax is incorrect.
try {
Pattern pattern = Pattern.compile("[a-z");
} catch (PatternSyntaxException e) {
System.out.println("Invalid regex: " + e.getDescription());
}
# Output:
# Invalid regex: Unclosed character class near index 4
In this example, the regular expression [a-z
is missing the closing bracket, which causes a PatternSyntaxException
to be thrown. The exception’s getDescription()
method returns a description of the error.
To fix this error, ensure that your regular expression’s syntax is correct. In this case, the correct regular expression would be [a-z]
.
No Match Found
Another common issue is when no match is found. If you call the Matcher.group()
method without first calling the Matcher.find()
method, or if the Matcher.find()
method returns false, a IllegalStateException
is thrown.
try {
Pattern pattern = Pattern.compile("Java");
Matcher matcher = pattern.matcher("Hello, World!");
System.out.println("Match: " + matcher.group());
} catch (IllegalStateException e) {
System.out.println("No match found.");
}
# Output:
# No match found.
In this example, the string ‘Hello, World!’ doesn’t contain the word ‘Java’, so no match is found, and an IllegalStateException
is thrown.
To avoid this error, always check if a match is found by calling the Matcher.find()
method before calling the Matcher.group()
method.
Best Practices and Optimization
When using the Java Pattern class, keep these best practices in mind:
- Precompile Patterns: If you’re using a pattern multiple times, precompile it using the
Pattern.compile()
method. This can improve performance because the regular expression doesn’t need to be compiled every time it’s used. Use Non-Capturing Groups When Possible: If you don’t need to retrieve the matched text, use non-capturing groups
(?:...)
instead of capturing groups(...)
. Non-capturing groups can improve performance by avoiding unnecessary storage of matched text.Be Aware of Backtracking: Some regular expressions can cause excessive backtracking, which can slow down the matching process. Try to avoid complex regular expressions that can cause backtracking, or use a possessive quantifier
++
or atomic group(?>...)
to prevent backtracking.
Understanding Regular Expressions in Java
Regular expressions, or regex, are a powerful tool for pattern matching and manipulation of strings. In Java, regular expressions are supported by several classes in the java.util.regex
package, including the Pattern
and Matcher
classes.
A regular expression is a sequence of characters that forms a search pattern. This pattern can be used to match, locate, and manage text. Regular expressions can match literal text and can also identify patterns like digits, letters, whitespace, word boundaries, and more.
The Matcher Class
The Matcher
class works in conjunction with the Pattern
class. Once a Pattern
object is created, a Matcher
object can be created to match character sequences against the pattern. The Matcher
class provides methods to find matches (like find()
, matches()
, and lookingAt()
), to replace matches (replaceAll()
, replaceFirst()
), and to retrieve information about matches (start()
, end()
, group()
).
Here’s an example of using the Matcher
class:
import java.util.regex.*;
Pattern pattern = Pattern.compile("Java");
Matcher matcher = pattern.matcher("Hello, Java Pattern!");
if (matcher.find()) {
System.out.println("Match found at index " + matcher.start());
} else {
System.out.println("No match found.");
}
# Output:
# Match found at index 7
In this example, the Pattern.compile()
method compiles the regular expression ‘Java’ into a Pattern
object. The pattern.matcher()
method creates a Matcher
object that matches the pattern against the string ‘Hello, Java Pattern!’. The matcher.find()
method returns true if a match is found, and false otherwise. The matcher.start()
method returns the start index of the match.
The PatternSyntaxException Class
The PatternSyntaxException
class is a subclass of the IllegalArgumentException
class. It’s an unchecked exception that indicates a syntax error in a regular expression pattern. This exception is thrown by the Pattern.compile()
method when a pattern’s syntax is invalid.
Here’s an example of a PatternSyntaxException
:
try {
Pattern pattern = Pattern.compile("[a-z");
} catch (PatternSyntaxException e) {
System.out.println("Invalid regex: " + e.getDescription());
}
# Output:
# Invalid regex: Unclosed character class near index 4
In this example, the regular expression ‘[a-z’ is missing the closing bracket, which causes a PatternSyntaxException
to be thrown. The exception’s getDescription()
method returns a description of the error.
Expanding the Use of Java Pattern Class
As you become more comfortable with the Java Pattern class, you’ll start seeing its potential in larger projects. The Pattern class is not just for simple pattern matching – it’s a powerful tool that can significantly enhance your Java applications.
Data Validation with Java Pattern
One common use of the Pattern class is data validation. For instance, you can use it to check if a string matches a specific format, like an email address or a phone number. Here’s an example of using the Pattern class to validate email addresses:
import java.util.regex.*;
Pattern emailPattern = Pattern.compile("\\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\\.[A-Za-z]{2,}\\b");
Matcher matcher = emailPattern.matcher("[email protected]");
if (matcher.matches()) {
System.out.println("Valid email address.");
} else {
System.out.println("Invalid email address.");
}
# Output:
# Valid email address.
In this code, the regular expression \\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\\.[A-Za-z]{2,}\\b
matches any string that is a valid email address. The matches()
method returns true if the string matches the pattern exactly, making it perfect for data validation.
Text Processing with Java Pattern
Another application of the Pattern class is text processing. For example, you can use it to split a string into tokens, extract substrings, or replace certain parts of a string. Here’s an example of using the Pattern class to split a string into words:
import java.util.regex.*;
Pattern wordPattern = Pattern.compile("\\w+");
Matcher matcher = wordPattern.matcher("Hello, Java Pattern!");
while (matcher.find()) {
System.out.println("Word: " + matcher.group());
}
# Output:
# Word: Hello
# Word: Java
# Word: Pattern
In this example, the find()
method finds each word in the string, and the group()
method retrieves the matched word. This makes the Pattern class a powerful tool for text processing.
Further Resources for Mastering Java Pattern Class
If you’re interested in diving deeper into the Java Pattern class and related topics, here are some resources that you might find useful:
- Mastering Java Strings: A Comprehensive Guide – Explore Java’s String class for efficient string manipulation.
Regular Expressions in Java – Explore regular expressions (regex) in Java for pattern matching and text manipulation.
Matcher in Java – Explore the Matcher class in Java for applying regular expressions to text input.
Java’s Official Regex Tutorial from Oracle provides a detailed introduction to regular expressions in Java.
Java Regex Tutorial covers Java’s regular expression syntax in detail, with plenty of examples.
Baeldung’s Guide to Java Regex provides an overview of Java’s regex capabilities, including topics like lookaheads and lookbehinds.
Wrapping Up: Mastering Java Pattern Class
In this comprehensive guide, we’ve explored the depths of the Java Pattern class, an integral part of Java’s regular expression API for pattern matching and text processing.
We began with the basics, understanding how to define a pattern using the Pattern class and use it to create a Matcher object. We then delved into more advanced usage, discussing complex patterns and the use of the Matcher class to find and analyze matches. We also explored alternative approaches to pattern matching in Java, such as using the String class’s matches method.
Along the way, we navigated through common challenges that you may encounter while using the Java Pattern class, such as PatternSyntaxException and no match found issues, providing you with solutions and best practices for each problem.
To give you a broader perspective, here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
Java Pattern Class | High | High | Multiple matches, detailed match information |
String’s matches() method | Low | Low | Simple pattern matching |
Whether you’re just starting out with the Java Pattern class or you’re looking to enhance your pattern matching skills, we hope this guide has equipped you with a deeper understanding of the Java Pattern class and its capabilities.
With its balance of flexibility and power, the Java Pattern class is a robust tool for pattern matching in Java. Now, you’re well prepared to handle any pattern matching task that comes your way. Happy coding!