Java SimpleDateFormat Class: How-to Use with Examples
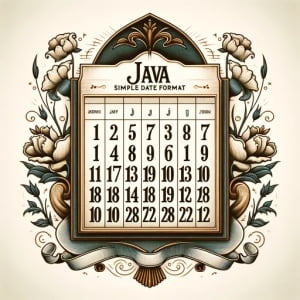
Are you struggling with date formatting in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling date formatting in Java, but we’re here to help.
Think of Java’s Simple Date Format class as a skilled craftsman – it can shape dates into any format you desire, providing a versatile and handy tool for various tasks.
This guide will walk you through the process of using Java’s Simple Date Format, from basic use to advanced techniques. We’ll cover everything from the basics of date formatting to more advanced techniques, as well as alternative approaches.
Let’s dive in and start mastering Java’s Simple Date Format!
TL;DR: How Do I Use the SimpleDateFormat Class in Java?
To use the
SimpleDateFormat
class you must first import it with the code,import java.text.SimpleDateFormat;
. Then you will need to create an instance with the syntax,SimpleDateFormat sdf = new SimpleDateFormat('dateFormat');
. This class allows you to format dates according to your needs, making it a powerful tool in your Java toolkit.
Here’s a simple example:
import java.text.SimpleDateFormat;
import java.util.Date;
SimpleDateFormat sdf = new SimpleDateFormat("MM/dd/yyyy");
String date = sdf.format(new Date());
System.out.println(date);
// Output:
// 'MM/dd/yyyy'
In this example, we’ve created an instance of SimpleDateFormat
with a date pattern ‘MM/dd/yyyy’ which is the standard format for dates in the United States. We then format the current date using this pattern and print it out. The output will be the current date in the format ‘MM/dd/yyyy’.
This is a basic way to use the Simple Date Format in Java, but there’s much more to learn about date formatting. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
Getting Started with SimpleDateFormat
The SimpleDateFormat
class in Java is used for formatting and parsing dates in a locale-sensitive manner. It allows for formatting (date -> text), parsing (text -> date), and normalization.
Let’s start with a basic example of how to use the SimpleDateFormat
class to format a date.
import java.text.SimpleDateFormat;
import java.util.Date;
public class Main {
public static void main(String[] args) {
SimpleDateFormat sdf = new SimpleDateFormat('dd/MM/yyyy');
String date = sdf.format(new Date());
System.out.println(date);
}
}
// Output:
// The current date in the format 'dd/MM/yyyy'
In this code, we first import the necessary classes. We then create a SimpleDateFormat
object sdf
and provide a pattern string ‘dd/MM/yyyy’ to the constructor. This pattern string defines the format of the date.
We then call the format
method on sdf
and pass it a new Date
object (which defaults to the current date and time). The format
method returns a String
representing the date in the specified format, which we then print out.
This is a basic usage of the SimpleDateFormat
class. It’s straightforward, but there are some important things to keep in mind. For one, SimpleDateFormat
is not thread-safe, meaning that you shouldn’t use a single instance from multiple threads without proper synchronization. Also, date patterns are case-sensitive. For example, ‘MM’ stands for month, while ‘mm’ stands for minutes.
Exploring Different Date and Time Patterns
The real power of SimpleDateFormat
lies in its flexibility. By changing the pattern string, you can manipulate the format of the date in various ways. Let’s explore how to deal with different date and time patterns using the SimpleDateFormat
class.
import java.text.SimpleDateFormat;
import java.util.Date;
public class Main {
public static void main(String[] args) {
SimpleDateFormat sdf1 = new SimpleDateFormat('dd-MM-yyyy HH:mm:ss');
SimpleDateFormat sdf2 = new SimpleDateFormat('E, MMM dd yyyy HH:mm:ss');
Date now = new Date();
System.out.println(sdf1.format(now));
System.out.println(sdf2.format(now));
}
}
// Output:
// The current date in the format 'dd-MM-yyyy HH:mm:ss'
// The current date in the format 'E, MMM dd yyyy HH:mm:ss'
In this code, we’ve created two SimpleDateFormat
objects with different pattern strings. The first pattern, ‘dd-MM-yyyy HH:mm:ss’, includes not only the date but also the time, down to seconds. The second pattern, ‘E, MMM dd yyyy HH:mm:ss’, provides a more human-readable format, including the day of the week (E
) and the month name (MMM
).
By using different patterns, you can format dates to suit your specific needs. However, you should be careful to use the correct symbols in your pattern string, as they are case-sensitive and each symbol has a specific meaning. For example, ‘MM’ stands for month, ‘mm’ stands for minutes, ‘HH’ is for 24-hour format hour, and ‘hh’ is for 12-hour format hour.
Exploring Alternative Date Formatting Methods
While SimpleDateFormat
is a powerful tool, Java provides other classes for date formatting, such as DateTimeFormatter
. This class, introduced in Java 8, is part of the new Date and Time API that aims to fix problems with the old date-time classes.
Let’s see how we can use DateTimeFormatter
to format dates:
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class Main {
public static void main(String[] args) {
DateTimeFormatter dtf = DateTimeFormatter.ofPattern('dd-MM-yyyy HH:mm:ss');
LocalDateTime now = LocalDateTime.now();
System.out.println(dtf.format(now));
}
}
// Output:
// The current date and time in the format 'dd-MM-yyyy HH:mm:ss'
In this example, we use DateTimeFormatter
to format a LocalDateTime
object. The ofPattern
method is used to create a formatter with the specified pattern.
One significant advantage of DateTimeFormatter
over SimpleDateFormat
is that it’s thread-safe. This means you can use a single instance from multiple threads without synchronization issues. However, DateTimeFormatter
is part of the new Date and Time API, so it’s not available in Java versions prior to Java 8. Therefore, if you’re working with an older Java version, SimpleDateFormat
would be your go-to class for date formatting.
The choice between SimpleDateFormat
and DateTimeFormatter
will depend on your specific needs and the Java version you’re working with. Both classes provide flexible and powerful tools for date formatting.
Troubleshooting Common Issues with Date Formatting
Like any programming tool, using SimpleDateFormat
and DateTimeFormatter
can sometimes lead to unexpected issues. Let’s discuss some common problems and how to solve them.
Dealing with ParseException
A common issue when using SimpleDateFormat
is encountering a ParseException
. This exception is thrown when parsing a string into a date fails.
import java.text.ParseException;
import java.text.SimpleDateFormat;
public class Main {
public static void main(String[] args) {
SimpleDateFormat sdf = new SimpleDateFormat('dd/MM/yyyy');
try {
sdf.parse('31-12-2020');
} catch (ParseException e) {
e.printStackTrace();
}
}
}
// Output:
// java.text.ParseException: Unparseable date: '31-12-2020'
In this example, we’re trying to parse a date string using a pattern that doesn’t match the string format. The pattern ‘dd/MM/yyyy’ expects the date parts to be separated by slashes, but the date string uses dashes. This mismatch causes a ParseException
.
To avoid this exception, ensure that the date string matches the pattern string exactly. If the date string format can vary, consider using multiple pattern strings or a more flexible date parsing method.
Handling Different Date and Time Patterns
Another common issue is dealing with different date and time patterns. The symbols used in the pattern strings are case-sensitive and each has a specific meaning. For example, ‘MM’ stands for month, ‘mm’ stands for minutes, ‘HH’ is for 24-hour format hour, and ‘hh’ is for 12-hour format hour.
Ensure you are using the correct symbols for your desired output. Consult the Java documentation for a full list of pattern string symbols and their meanings.
By understanding these common issues and their solutions, you can use SimpleDateFormat
and DateTimeFormatter
more effectively and avoid common pitfalls.
Understanding Java’s Date and Time API
Before diving deeper into date formatting, it’s essential to understand the underlying concepts and the Java Date and Time API.
Java’s Date and Time API, introduced in Java 8, is a comprehensive framework for date and time manipulation. It provides classes for date, time, date-time, timezone, period, duration, and more. This API is designed to be easier to use, more flexible, and more intuitive than the old date-time classes, such as java.util.Date
and java.util.Calendar
.
Date and Time Patterns
A crucial part of date formatting is understanding date and time patterns. In SimpleDateFormat
and DateTimeFormatter
, a date and time pattern is a string consisting of special symbols that represent different parts of a date, such as year, month, day, hour, minute, and second.
Here are some common symbols used in date and time patterns:
- ‘y’: year
- ‘M’: month in year
- ‘d’: day in month
- ‘H’: hour in day (0-23)
- ‘h’: hour in am/pm (1-12)
- ‘m’: minute in hour
- ‘s’: second in minute
These symbols can be repeated to represent different formats. For example, ‘yy’ represents a two-digit year, while ‘yyyy’ represents a four-digit year. ‘MM’ represents a two-digit month, while ‘MMM’ represents a three-letter month abbreviation.
Here’s an example of a date formatted with a custom pattern:
import java.text.SimpleDateFormat;
import java.util.Date;
public class Main {
public static void main(String[] args) {
SimpleDateFormat sdf = new SimpleDateFormat('dd-MMM-yyyy HH:mm:ss');
String date = sdf.format(new Date());
System.out.println(date);
}
}
// Output:
// The current date and time in the format 'dd-MMM-yyyy HH:mm:ss'
In this code, we use the pattern string ‘dd-MMM-yyyy HH:mm:ss’ to format the current date and time. The output will be the current date and time in the specified format, such as ’01-Jan-2022 13:45:30′.
The Importance of Date Formatting in Java
Understanding and mastering Java’s date formatting is not just an academic exercise. It’s a crucial skill that has practical applications in many areas of software development.
Date Formatting in Logging
One common use of date formatting is in logging. Logs often include timestamps to help developers understand when certain events occurred. Using SimpleDateFormat
or DateTimeFormatter
, you can format these timestamps to be easily readable and provide the necessary level of detail.
Date Formatting in Database Applications
Another area where date formatting is essential is in database applications. Databases often store dates in a specific format, and when retrieving this data, you may need to format it in a way that’s understandable and useful for the end-user.
Exploring Related Concepts
While SimpleDateFormat
and DateTimeFormatter
are powerful tools for date formatting, Java provides other classes and methods for dealing with dates and times. For example, the Calendar
class provides methods for manipulating dates based on the calendar system. Another important concept is time zones, which you can handle in Java using the TimeZone
and ZonedDateTime
classes.
Further Resources for Mastering Java Date Formatting
To deepen your understanding of date formatting in Java, here are some resources for further reading:
- Java Date Article on IOFlood explains how to parse, format, and compare dates using Java’s Date class.
Date Format in Java – Learn about date formatting in Java and its importance for displaying dates as strings.
Time Handling in Java explains LocalDate, LocalTime, and LocalDateTime classes for working with dates and times.
Java Date and Time Tutorial on Baeldung, covers the new Date and Time API introduced in Java 8.
Java SimpleDateFormat Tutorial on JavaTpoint, provides a deep dive into
SimpleDateFormat
with many examples.Official Java Documentation on SimpleDateFormat provides an extensive look at the SimpleDateFormat class in Java.
Wrapping Up: SimpleDateFormat Class
In this comprehensive guide, we’ve explored the ins and outs of Java’s Simple Date Format, a versatile tool for formatting dates in Java.
We began with the basics, learning how to use the SimpleDateFormat
class to format dates. We then ventured into more advanced territory, exploring different date and time patterns and how to handle them with SimpleDateFormat
. Along the way, we tackled common issues you might encounter when using SimpleDateFormat
, such as ParseException
and dealing with different date and time patterns, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to date formatting, comparing SimpleDateFormat
with DateTimeFormatter
, a newer date formatting class introduced in Java 8. Here’s a quick comparison of these classes:
Class | Thread Safety | Availability | Flexibility |
---|---|---|---|
SimpleDateFormat | Not thread-safe | Available in all Java versions | High |
DateTimeFormatter | Thread-safe | Available in Java 8 and later | High |
Whether you’re just starting out with Java’s Simple Date Format or you’re looking to level up your date formatting skills, we hope this guide has given you a deeper understanding of date formatting in Java and its practical applications.
With its balance of flexibility and ease of use, Java’s Simple Date Format is a powerful tool for handling dates in Java. Happy coding!