Date Format in Java: Techniques, Tips, and Examples
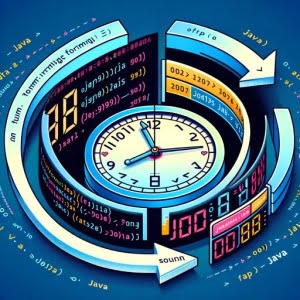
Are you wrestling with date formatting in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling date formatting in Java. Think of Java’s date formatting as a skilled watchmaker – it can precisely adjust dates to your desired format, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of formatting dates in Java, from the basics to more advanced techniques. We’ll cover everything from using the SimpleDateFormat class, handling different date and time formats, to dealing with common issues and their solutions.
So, let’s dive in and start mastering date formatting in Java!
TL;DR: How Do I Format Dates in Java?
The
SimpleDateFormat
class in Java is your go-to tool for date formatting, used with the syntax:SimpleDateFormat sdf = new SimpleDateFormat('yyyy-MM-dd');
Here’s a quick example of how you can use it:
import java.text.SimpleDateFormat;
import java.util.Date;
SimpleDateFormat sdf = new SimpleDateFormat('yyyy-MM-dd');
String date = sdf.format(new Date());
// Output:
// '2022-01-01'
In this example, we’ve created a SimpleDateFormat
object with the pattern ‘yyyy-MM-dd’. We then use the format
method to format the current date (new Date()
) according to this pattern, resulting in a string in the format ‘2022-01-01’.
This is a basic way to format dates in Java, but there’s much more to learn about handling different date and time formats, using alternative methods, and troubleshooting common issues. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Understanding SimpleDateFormat in Java
- Dealing with Different Date and Time Formats
- Exploring Alternative Methods for Date Formatting
- Troubleshooting Common Issues in Date Formatting
- Digging Deeper into Java’s Date and Time API
- The Relevance of Date Formatting in Java
- Wrapping Up: Mastering Date Formatting in Java
Understanding SimpleDateFormat in Java
In Java, the SimpleDateFormat
class is a concrete class for formatting and parsing dates in a locale-sensitive manner. It allows for formatting (date -> text), parsing (text -> date), and normalization.
How SimpleDateFormat Works
SimpleDateFormat
allows you to start by choosing any user-defined patterns for date-time formatting. However, you should be aware of the symbols used in these patterns. For instance, ‘y’ is for year, ‘M’ for month, ‘d’ for day, and so on.
Let’s see how you can use it with a simple example:
import java.text.SimpleDateFormat;
import java.util.Date;
public class Main {
public static void main(String[] args) {
SimpleDateFormat sdf = new SimpleDateFormat('yyyy-MM-dd');
String date = sdf.format(new Date());
System.out.println(date);
}
}
// Output:
// '2022-01-01'
In this example, we’ve created a SimpleDateFormat
object with the pattern ‘yyyy-MM-dd’. We then use the format
method to format the current date (new Date()
) according to this pattern. The format
method returns a string representing the date in the specified format.
Advantages and Potential Pitfalls
Using SimpleDateFormat
is straightforward and provides a good deal of flexibility in formatting dates. However, be aware that SimpleDateFormat
is not thread-safe. This means that you can’t safely use a single SimpleDateFormat
instance across multiple threads without external synchronization.
Another potential pitfall is the handling of timezone. If not explicitly set, SimpleDateFormat
uses the default timezone of the system. This can lead to unexpected results if your application is used across different timezones.
Dealing with Different Date and Time Formats
As you become more comfortable with the SimpleDateFormat
class, you’ll realize that it’s capable of handling a wide range of date and time formats. This flexibility becomes crucial when you’re dealing with different data sources that might represent dates in various ways.
Exploring Various Date and Time Formats
Let’s take a look at some different date and time formats and how you can handle them using SimpleDateFormat
:
import java.text.SimpleDateFormat;
import java.util.Date;
public class Main {
public static void main(String[] args) {
// Date format: 'yyyy-MM-dd'
SimpleDateFormat sdf1 = new SimpleDateFormat('yyyy-MM-dd');
// Date-Time format: 'dd-MM-yyyy HH:mm:ss'
SimpleDateFormat sdf2 = new SimpleDateFormat('dd-MM-yyyy HH:mm:ss');
String date1 = sdf1.format(new Date());
String date2 = sdf2.format(new Date());
System.out.println(date1);
System.out.println(date2);
}
}
// Output:
// '2022-01-01'
// '01-01-2022 00:00:00'
In this example, we’ve created two SimpleDateFormat
objects with different patterns. The first pattern, ‘yyyy-MM-dd’, is a date format. The second pattern, ‘dd-MM-yyyy HH:mm:ss’, is a date-time format that includes hours, minutes, and seconds.
Understanding the Differences
The key difference between the two formats is the inclusion of time in the second format. This can be useful when you need to record or display the exact time of an event, not just the date.
Best Practices
When dealing with different date and time formats, it’s essential to ensure consistency across your application. Decide on a standard date and time format and stick to it as much as possible. If you need to handle multiple formats, consider creating a utility class or method to handle date formatting. This will help you avoid errors and make your code easier to maintain.
Exploring Alternative Methods for Date Formatting
While SimpleDateFormat
is a powerful tool for date formatting, Java 8 introduced an even more robust API for date and time manipulation: the DateTimeFormatter
class.
Understanding DateTimeFormatter
DateTimeFormatter
is immutable and thread-safe, making it a significant improvement over SimpleDateFormat
in multi-threaded environments. Let’s see how you can use it:
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class Main {
public static void main(String[] args) {
DateTimeFormatter dtf = DateTimeFormatter.ofPattern('yyyy-MM-dd HH:mm:ss');
String date = dtf.format(LocalDateTime.now());
System.out.println(date);
}
}
// Output:
// '2022-01-01 00:00:00'
In this example, we’ve created a DateTimeFormatter
with the pattern ‘yyyy-MM-dd HH:mm:ss’. We then use the format
method to format the current date-time (LocalDateTime.now()
) according to this pattern.
Comparing SimpleDateFormat and DateTimeFormatter
Feature | SimpleDateFormat | DateTimeFormatter |
---|---|---|
Thread Safety | No | Yes |
API | Older, pre Java 8 | New, Java 8 and onwards |
Flexibility | Limited comparably | More flexible |
Time Zone handling | Manual | Automatic |
Making the Right Choice
While DateTimeFormatter
is more modern and thread-safe, SimpleDateFormat
is still widely used, especially in older codebases that haven’t migrated to Java 8. If you’re starting a new project or working with Java 8 and onwards, DateTimeFormatter
is recommended for better performance and ease of use.
Troubleshooting Common Issues in Date Formatting
While working with date formatting in Java, you might encounter a few common issues. Let’s discuss these problems and their solutions.
Handling ParseException
When parsing a date from a string using SimpleDateFormat
, you may encounter a ParseException
. This exception is thrown when the string cannot be parsed into a date.
import java.text.ParseException;
import java.text.SimpleDateFormat;
public class Main {
public static void main(String[] args) {
SimpleDateFormat sdf = new SimpleDateFormat('yyyy-MM-dd');
try {
Date date = sdf.parse('2022-13-01');
System.out.println(date);
} catch (ParseException e) {
e.printStackTrace();
}
}
}
// Output:
// java.text.ParseException: Unparseable date: "2022-13-01"
In this example, we tried to parse ‘2022-13-01’ into a date. However, 13 is not a valid month, so a ParseException
is thrown. To handle this exception, you can use a try-catch block as shown.
Dealing with Different Formats
Another common issue is dealing with different date formats. If you receive a date in a format different from what you expected, it can lead to incorrect results or exceptions.
To handle this, you should always validate the format of the date you receive. If you’re working with an API or a data source that can provide dates in different formats, consider using a library like Apache Commons Lang, which provides methods to parse dates in multiple formats.
Tips for Date Formatting
- Always validate your date strings before parsing.
- Be aware of the timezone. If not set explicitly,
SimpleDateFormat
uses the default system timezone. SimpleDateFormat
is not thread-safe. UseDateTimeFormatter
if you’re working with multiple threads.- Keep your date formatting consistent across your application to avoid confusion and errors.
Digging Deeper into Java’s Date and Time API
To truly master date formatting in Java, it’s essential to understand the underlying concepts of Java’s Date and Time API.
Understanding Java’s Date and Time API
Java’s Date and Time API, introduced in Java 8, is a comprehensive framework for date and time manipulation. It includes classes for date, time, date-time, timezone, periods, duration, and more.
The key classes in the API are:
LocalDate
: Represents a date (year, month, day).LocalTime
: Represents a time (hours, minutes, seconds, nanoseconds).LocalDateTime
: Represents both a date and a time.DateTimeFormatter
: Used for formatting and parsing dates and times.
These classes are immutable and thread-safe, making them a significant improvement over the old Date
and Calendar
classes.
Grasping Different Date and Time Formats
When working with dates and times, you’ll encounter various formats. Here are some common ones:
- ‘yyyy-MM-dd’: A date format with year, month, and day.
- ‘HH:mm:ss’: A time format with hours, minutes, and seconds.
- ‘yyyy-MM-dd HH:mm:ss’: A date-time format.
In these formats, ‘y’ stands for year, ‘M’ for month, ‘d’ for day, ‘H’ for hour, ‘m’ for minute, and ‘s’ for second.
Let’s see a simple example of how to use these formats with DateTimeFormatter
:
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class Main {
public static void main(String[] args) {
DateTimeFormatter dtf = DateTimeFormatter.ofPattern('yyyy-MM-dd HH:mm:ss');
String date = dtf.format(LocalDateTime.now());
System.out.println(date);
}
}
// Output:
// '2022-01-01 00:00:00'
In this example, we’ve formatted the current date-time into the ‘yyyy-MM-dd HH:mm:ss’ format using DateTimeFormatter
.
Understanding these formats and the Date and Time API is fundamental to mastering date formatting in Java.
The Relevance of Date Formatting in Java
Date formatting in Java is not just an academic exercise. It plays a crucial role in various real-world applications, such as data processing, web applications, and more.
Date Formatting in Data Processing
In data processing, date formatting is essential for sorting, filtering, and aggregating data based on time. It allows you to transform raw data into meaningful insights.
Date Formatting in Web Applications
In web applications, date formatting is used to display dates and times in a user-friendly manner. It also helps to handle user input in various date formats.
Exploring Related Concepts
Beyond date formatting, there are other related concepts you might want to explore. For instance, understanding time zones and locale settings can help you handle dates and times for users around the world.
Time zones are crucial when you’re dealing with users in different geographical locations. Locale settings allow you to format dates according to the cultural norms of a specific region.
Further Resources for Mastering Date Formatting in Java
To deepen your knowledge of date formatting in Java, consider exploring these resources:
- Exploring Java Date Handling: A Comprehensive Guide – Explains how to handle dates in Java with ease.
Exploring LocalDate in Java – Learn how to work with dates using LocalDate.
Java SimpleDateFormat Overview – Explore SimpleDateFormat in Java for parsing and formatting dates.
Java 8 Date and Time API Guide – A comprehensive guide to the Date and Time API introduced in Java 8
Java SimpleDateFormat Tutorial – A tutorial on using
SimpleDateFormat
for date formatting in Java.DateFormat Format Method in Java provides a detailed tutorial on the ‘DateFormat’ class and format method in Java.
Wrapping Up: Mastering Date Formatting in Java
In this comprehensive guide, we’ve explored the process of date formatting in Java. We’ve seen how Java provides powerful tools like SimpleDateFormat
and DateTimeFormatter
to handle a wide range of date and time formats.
We began with the basics, learning how to use SimpleDateFormat
to format dates. We then delved into advanced usage, handling different date and time formats and troubleshooting common issues, such as ParseException
and dealing with different formats.
We also looked at alternative approaches, exploring the DateTimeFormatter
class introduced in Java 8. This class offers several advantages over SimpleDateFormat
, including thread-safety and improved timezone handling.
Here’s a quick comparison of the methods we’ve discussed:
Method | Thread Safety | API | Flexibility | Time Zone handling |
---|---|---|---|---|
SimpleDateFormat | No | Older, pre Java 8 | Limited comparably | Manual |
DateTimeFormatter | Yes | New, Java 8 and onwards | More flexible | Automatic |
Whether you’re just starting out with date formatting in Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of how to format dates in Java.
Mastering date formatting is a crucial skill for any Java developer. With this knowledge, you’re now well-equipped to handle a wide range of date formatting tasks. Happy coding!