Closing Files in Python: Step-by-Step Guide
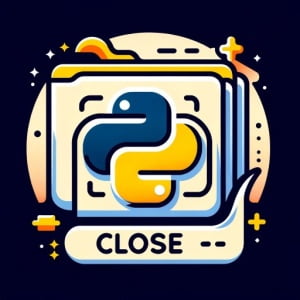
Are you finding it challenging to close files in Python? Just like closing a book after reading, it’s crucial to close files in Python once you’re done with them. Many developers overlook this step, but it’s a fundamental aspect of file handling in Python.
Think of Python’s file closing operation as the final act in a play – it signifies the end of the file’s usage in your script, freeing up system resources and preventing potential data corruption.
This guide will walk you through the process of closing files in Python, from the basic close()
method to more advanced techniques. We’ll cover everything from the basics of file closing to more advanced techniques, as well as alternative approaches.
Let’s dive in and start mastering file closing in Python!
TL;DR: How Do I Close a File in Python?
To close a file in Python, you use the
close()
method. This method is called on the file object you want to close. For example, if you have a file object namedfile
, you would close it like this:file.close()
.
Here’s a simple example:
file = open('myfile.txt', 'r')
file.close()
# Output:
# No output, but the file 'myfile.txt' is now closed and resources are freed.
In this example, we first open a file named ‘myfile.txt’ for reading, and then we close it using the close()
method. There’s no output from the close()
method, but after it’s called, the file is closed and any system resources used by the file are freed.
This is just the basic way to close a file in Python. There are more advanced techniques and considerations, especially for larger projects or more complex scenarios. Continue reading for a more detailed guide on closing files in Python.
Table of Contents
- Understanding the close() Method in Python
- Using the with Statement for Automatic File Closing
- Exploring Alternative Methods for Closing Files in Python
- Troubleshooting Common Issues in Python File Closing
- Understanding File Handling in Python
- The Role of File Handling in Larger Python Projects
- Wrapping Up: Mastering File Closing in Python
Understanding the close()
Method in Python
In Python, the close()
method is used to close a file and free up any system resources that were used during the file operation. This method is called on a file object, which is created when a file is opened.
When you’re done with a file, it’s good practice to close it. Not closing a file can lead to memory leaks, meaning your program continues to consume memory space even though it’s no longer needed. This can slow down your program and, in worst-case scenarios, cause your program to crash.
Here’s a basic example of using the close()
method:
# Open a file
file = open('myfile.txt', 'r')
# Perform some operations (like reading from or writing to the file)
# Close the file
file.close()
# Output:
# No output, but the file 'myfile.txt' is now closed and resources are freed.
In this example, we first open a file named ‘myfile.txt’ for reading. Then, we close it using the close()
method. There’s no output from the close()
method, but after it’s called, the file is closed and any system resources used by the file are freed.
Remember, not closing a file in Python might work in small scripts, but it can cause problems in larger applications. Always ensure to close your files with the close()
method after you’re done with them.
Using the with
Statement for Automatic File Closing
Python provides a more elegant and safer way to handle file closing through the with
statement. This statement creates a context where the file is automatically closed when we’re done with it, even if exceptions occur within the block. This feature is known as context management.
By using the with
statement, you’re ensuring your file is properly closed, reducing the risk of file corruption and freeing up system resources. This approach is particularly useful when working with larger files or in larger projects where manual file closing might be forgotten or overlooked.
Here’s an example of how to use the with
statement to automatically close a file in Python:
# Open and read a file using the with statement
with open('myfile.txt', 'r') as file:
content = file.read()
print(content)
# Output:
# 'Contents of the file are printed here.'
# The file 'myfile.txt' is now closed automatically.
In this example, we use the with
statement to open a file and assign it to the variable file
. We then read the content of the file with the read()
method and print it. Once the execution leaves the with
block, the file is automatically closed, even if an error occurs within the block.
The with
statement provides a reliable way for file handling in Python, ensuring your files are always closed appropriately.
Exploring Alternative Methods for Closing Files in Python
While the close()
method and the with
statement are standard ways to close files in Python, there are alternative approaches you might encounter, especially when using third-party libraries or dealing with specific use cases.
Using Third-Party Libraries
Some third-party libraries provide their own mechanisms for file handling, including file closing. For example, the popular pandas library, used for data analysis and manipulation, automatically closes files when you use its functions to read or write data.
Here’s an example of reading a CSV file with pandas:
import pandas as pd
df = pd.read_csv('myfile.csv')
# Output:
# No explicit output, but 'myfile.csv' is now closed after reading.
In this code, we use pandas’ read_csv()
function to read a CSV file and load it into a DataFrame. After the function call, pandas automatically closes the file.
The os
Module
Python’s built-in os
module also provides low-level file handling functions. While typically not as convenient as using open()
and close()
, they can give you more control in some scenarios.
Here’s an example of opening and closing a file with the os
module:
import os
# Open a file
fd = os.open('myfile.txt', os.O_RDONLY)
# Close the file
os.close(fd)
# Output:
# No explicit output, but 'myfile.txt' is now closed.
In this example, we use os.open()
to open a file and os.close()
to close it. These functions work with file descriptors, which are integers representing open files.
While these alternative methods can be useful in certain cases, they are typically more complex and less commonly used than the standard close()
method and the with
statement. For most cases, sticking to these standard methods will make your code easier to read and maintain.
Troubleshooting Common Issues in Python File Closing
While working with files in Python, you might encounter some common issues. Let’s discuss these problems and how to resolve them.
The ‘File Not Open’ Error
One common error you might encounter is trying to close a file that isn’t open. This can happen if you mistakenly call close()
on a file object that was never opened or has already been closed.
Here’s an example:
file = open('myfile.txt', 'r')
file.close()
file.close()
# Output:
# Traceback (most recent call last):
# File "<stdin>", line 1, in <module>
# ValueError: I/O operation on closed file.
In this example, we’re trying to close the file ‘myfile.txt’ twice. The second call to close()
raises a ValueError
because the file is already closed.
To fix this error, you can check whether a file is closed before trying to close it. The closed
attribute of a file object tells you whether the file is closed (True
) or not (False
).
Here’s how you can use it:
file = open('myfile.txt', 'r')
if not file.closed:
file.close()
# Output:
# No output, but the file 'myfile.txt' is now closed and resources are freed.
In this code, we check if the file is not closed before we try to close it. This prevents the ValueError
from being raised.
Best Practices for Closing Files in Python
When working with files in Python, here are some best practices to follow:
- Always close your files when you’re done with them. This frees up system resources and prevents potential data corruption.
- Use the
with
statement for file handling. This ensures your files are properly closed, even if an error occurs. - Check if a file is closed before trying to close it. This can prevent errors in your code.
By following these best practices, you can avoid common issues and write more robust code when working with files in Python.
Understanding File Handling in Python
Python’s file handling system is a key aspect of the language that allows us to interact with external files on our system. This includes operations like opening files, reading data from them, writing data to them, and importantly, closing them when we’re done.
Opening, Reading, and Writing Files
Before you can read from or write to a file in Python, you need to open it using the open()
function. This function returns a file object, which you can then use to read data from the file or write data to it.
Here’s an example:
# Open a file for writing
file = open('myfile.txt', 'w')
# Write some data to the file
file.write('Hello, Python!')
# Close the file
file.close()
# Output:
# No output, but 'Hello, Python!' is now written to 'myfile.txt', and the file is closed.
In this example, we open a file named ‘myfile.txt’ for writing, write a string to it, and then close it.
The Importance of Closing Files
Closing a file in Python is just as important as opening it. When a file is closed, Python frees up the system resources that were used during the file operation. If a file is not properly closed, it can lead to memory leaks, which can slow down your program or even cause it to crash.
Moreover, not closing a file can cause data corruption, especially when you’re writing data to the file. Until a file is properly closed, the changes you make might not be saved correctly.
Potential Issues from Not Closing Files
If you don’t close a file, you might encounter unexpected behavior in your program. For example, if you open a file multiple times without closing it, you might exceed the maximum number of file descriptors that your system allows for a process, causing your program to crash.
In conclusion, proper file handling, including closing files when you’re done with them, is crucial when working with files in Python. It keeps your program running efficiently and prevents potential issues.
The Role of File Handling in Larger Python Projects
File handling is not limited to small scripts or basic Python programs. In fact, it plays a critical role in larger Python projects, where efficient and correct file handling can significantly impact the performance and reliability of your code.
Whether you’re developing a web application with Django, a data analysis program with pandas, or a machine learning model with scikit-learn, you’ll likely need to interact with files. You might need to read configuration data, load machine learning models, process large datasets, or write logs or outputs to disk.
In all these scenarios, understanding how to properly close files in Python is crucial. It ensures your program runs efficiently, prevents potential data corruption, and helps maintain the integrity of your data.
Applying File Closing Concepts in Different Contexts
The concepts discussed in this article, such as using the close()
method or the with
statement, are not limited to basic file operations. They can be applied in different contexts and with different types of files.
For example, when working with binary files, you can still use the close()
method to close the file after you’re done with it. Similarly, when working with text or CSV files in pandas, even though pandas automatically closes files, understanding how and why it does so can help you debug issues or optimize your code.
Further Resources for Mastering File Handling in Python
To further deepen your understanding of file handling in Python, including file closing, here are some additional resources:
- Navigating Python’s OS Module – Tips and tricks on file and directory manipulation with Python’s “os” module.
Working with os.path Module – Learn how to leverage the Python “os.path” module for path-related operations.
Python File Checking – Learn how to check if a file exists in Python using various techniques.
Python’s official documentation on file handling provides a comprehensive understanding of reading and writing Python files.
Real Python’s file I/O guide offers practical examples and techniques for file input and output operations in Python.
Python for Beginners’ file handling tutorial provides an intro to handling operations such as reading and writing Python files.
These resources provide in-depth tutorials and explanations on file handling in Python, including opening, reading, writing, and closing files.
Wrapping Up: Mastering File Closing in Python
In this comprehensive guide, we’ve navigated through the essential process of closing files in Python, a fundamental aspect of file handling in Python that often gets overlooked yet holds great importance.
We began with the basics, understanding how to use the close()
method to manually close files once we’re done with them. We then delved into the advanced usage of the with
statement, a more elegant and safer way to handle file closing, which automatically closes the file even if exceptions occur within the block.
Along the way, we tackled common issues and errors that you might encounter when closing files, such as the ‘file not open’ error, and provided solutions to help you overcome these challenges.
We also explored alternative approaches to file closing, such as using third-party libraries like pandas for automatic file closing, or using Python’s built-in os
module for more control over file operations.
Here’s a rundown of the various methods we discussed:
Method | Pros | Cons |
---|---|---|
close() method | Direct control over closing | Manual, can be forgotten |
with statement | Automatic, safer | None |
Third-party libraries | Automatic, part of library’s functions | Depends on the library |
os module | More control | Less convenient |
Whether you’re a beginner just starting out with Python or an experienced developer looking to refine your file handling skills, we hope this guide has deepened your understanding of the importance of closing files in Python and how to do it effectively.
Remember, proper file handling, including closing files when you’re done with them, is crucial for efficient and reliable Python programming. It prevents potential data corruption and helps maintain the integrity of your data. Happy coding!