Java LocalDate: From Basics to Advanced Usage
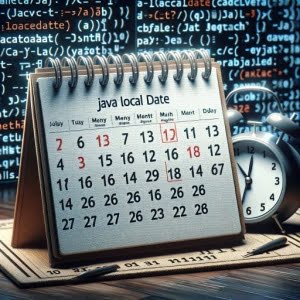
Are you wrestling with date handling in Java? You’re not alone. Many developers find themselves in a tangle when it comes to managing dates in Java, but there’s a tool that can make this process a breeze.
Think of Java’s LocalDate as a time machine – it allows you to navigate through time with ease, providing a versatile and handy tool for various tasks.
This guide will walk you through everything you need to know about using LocalDate in Java, from basic usage to advanced techniques. We’ll cover everything from creating and manipulating LocalDate instances, to dealing with different time zones, and even troubleshooting common issues.
Let’s get started and master LocalDate in Java!
TL;DR: How Do I Use LocalDate in Java?
LocalDate is a class in Java used to represent a date in ISO-8601 format (yyyy-MM-dd) and can be called simply with
LocalDate date = LocalDate.now()
. It’s a part of the Java 8 Date and Time API and is used for handling dates without time zones.
Here’s a simple example:
LocalDate date = LocalDate.now();
LocalDate tomorrow = date.plusDays(1);
System.out.println(tomorrow);
# Output:
(Assuming today's date is 2022-01-01)
# 2022-01-02
In this example, we’re using the now()
method of the LocalDate
class to get the current date. Then plusDays(1)
is used to add one day to the current date, returning the date of tomorrow. The println()
method then prints this date to the console. The output is the date of tomorrow in the format yyyy-MM-dd.
This is just a basic way to use LocalDate in Java, but there’s much more to learn about handling dates and times in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Using LocalDate: A Beginner’s Guide
- Diving Deeper: Advanced LocalDate Techniques
- Exploring Alternatives: LocalDateTime and ZonedDateTime
- Troubleshooting LocalDate: Common Issues and Solutions
- Understanding Java’s Date and Time API
- LocalDate in Real-World Applications
- Wrapping Up: Mastering LocalDate in Java
Using LocalDate: A Beginner’s Guide
The LocalDate class in Java is used to represent a date in the ISO-8601 calendar system, which is the yyyy-MM-dd format, such as ‘2022-01-01’. It’s a part of the Java 8 Date and Time API and does not include time, time-zone, or offset information.
Here’s how you can create an instance of LocalDate:
LocalDate date = LocalDate.now();
System.out.println(date);
# Output:
# 2022-01-01
In the above example, the now()
method of the LocalDate class is used to get the current date. This date is then printed to the console, resulting in the output of the current date in the yyyy-MM-dd format.
Manipulating LocalDate Instances
You can also manipulate LocalDate instances using various methods. Let’s look at an example:
LocalDate date = LocalDate.of(2022, 1, 1);
LocalDate newDate = date.plusDays(5);
System.out.println(newDate);
# Output:
# 2022-01-06
In this example, the of()
method is used to create a LocalDate instance of the date ‘2022-01-01’. We then use the plusDays()
method to add 5 days to this date, resulting in the date ‘2022-01-06’.
These methods are straightforward and easy to use, but it’s important to remember that LocalDate instances are immutable. This means that when you use methods like plusDays()
, a new LocalDate instance is created instead of modifying the original instance. This is a key feature of the LocalDate class and a fundamental concept to understand when working with dates in Java.
Diving Deeper: Advanced LocalDate Techniques
While the basic usage of LocalDate is relatively straightforward, there are more complex aspects that can be incredibly useful in your Java programming journey. Let’s delve into some of these, such as formatting and parsing dates, as well as working with different time zones.
Formatting LocalDate Instances
The LocalDate class provides a format()
method, which you can use to format dates. This method uses a DateTimeFormatter object to format the date. Here’s an example:
LocalDate date = LocalDate.of(2022, 1, 1);
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd-MM-yyyy");
String formattedDate = date.format(formatter);
System.out.println(formattedDate);
# Output:
# 01-01-2022
In this example, we’re creating a LocalDate instance for the date ‘2022-01-01’. We then create a DateTimeFormatter with the pattern ‘dd-MM-yyyy’. Using the format()
method of the LocalDate instance, we format the date according to the specified pattern. The result is the string ’01-01-2022′, which is printed to the console.
Parsing Dates
To convert a string into a LocalDate instance, you can use the parse()
method. This method also uses a DateTimeFormatter to define the date format. Here’s how you can do it:
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd-MM-yyyy");
LocalDate date = LocalDate.parse("01-01-2022", formatter);
System.out.println(date);
# Output:
# 2022-01-01
In this example, we’re creating a DateTimeFormatter with the pattern ‘dd-MM-yyyy’. We then use the parse()
method of the LocalDate class to parse the string ’01-01-2022′ into a LocalDate instance. The result is the date ‘2022-01-01’, which is printed to the console.
Working with Different Time Zones
While LocalDate itself does not contain any time zone information, you can convert a LocalDate to a ZonedDateTime, which does include time zone information. Here’s an example:
LocalDate date = LocalDate.of(2022, 1, 1);
ZonedDateTime zonedDateTime = date.atStartOfDay(ZoneId.of("America/New_York"));
System.out.println(zonedDateTime);
# Output:
# 2022-01-01T00:00-05:00[America/New_York]
In this example, we’re creating a LocalDate instance for the date ‘2022-01-01’. We then use the atStartOfDay()
method to convert this LocalDate instance to a ZonedDateTime instance at the start of the day in the ‘America/New_York’ time zone. The result is the ZonedDateTime ‘2022-01-01T00:00-05:00[America/New_York]’, which is printed to the console.
These advanced techniques can significantly enhance your ability to handle dates in Java, making your programs more versatile and robust.
Exploring Alternatives: LocalDateTime and ZonedDateTime
While LocalDate is a powerful tool for handling dates, Java offers other date and time classes that you might find useful in certain scenarios. Let’s look at two of these: LocalDateTime and ZonedDateTime.
LocalDateTime: Dates and Times Together
LocalDateTime is a class in Java that combines date and time, but without a time zone. Here’s a simple example of using LocalDateTime:
LocalDateTime dateTime = LocalDateTime.now();
System.out.println(dateTime);
# Output:
# 2022-01-01T12:00:00
In this example, we’re using the now()
method of the LocalDateTime class to get the current date and time. The println()
method then prints this date and time to the console. The output is the current date and time in the format yyyy-MM-ddTHH:mm:ss.
ZonedDateTime: Dates, Times, and Time Zones
ZonedDateTime is a class in Java that combines date, time, and time zone. Here’s an example of how to use it:
ZonedDateTime dateTime = ZonedDateTime.now(ZoneId.of("America/New_York"));
System.out.println(dateTime);
# Output:
# 2022-01-01T12:00:00-05:00[America/New_York]
In this example, we’re using the now()
method of the ZonedDateTime class to get the current date and time in the ‘America/New_York’ time zone. The println()
method then prints this date and time to the console. The output is the current date and time in the format yyyy-MM-ddTHH:mm:ssZ[ZoneId].
Choosing the Right Class
Which class should you use? It depends on your needs. If you only need to work with dates, LocalDate is the way to go. If you need to work with dates and times, but don’t care about time zones, LocalDateTime is your best bet. And if you need to work with dates, times, and time zones, ZonedDateTime has you covered.
Remember, the key is to understand your requirements and choose the tool that best fits your needs.
Troubleshooting LocalDate: Common Issues and Solutions
While working with LocalDate, you may encounter some common issues. Let’s discuss these potential pitfalls and how to navigate around them.
Handling DateTimeParseException
When parsing a string into a LocalDate, you may encounter a DateTimeParseException if the string is not in the correct format. Here’s how you can handle this exception:
try {
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd-MM-yyyy");
LocalDate date = LocalDate.parse("01-13-2022", formatter);
} catch (DateTimeParseException e) {
System.out.println("Invalid date format.");
}
# Output:
# Invalid date format.
In this example, we’re trying to parse the string ’01-13-2022′ into a LocalDate. However, the string is not in the correct format, so a DateTimeParseException is thrown. We catch this exception and print a message to the console.
Dealing with DateTimeException
Certain methods of the LocalDate class can throw a DateTimeException. For example, the of()
method throws this exception if the values passed to it are not valid date values. Here’s an example:
try {
LocalDate date = LocalDate.of(2022, 13, 1);
} catch (DateTimeException e) {
System.out.println("Invalid date.");
}
# Output:
# Invalid date.
In this example, we’re trying to create a LocalDate instance for the date ‘2022-13-01’. However, the month value is not valid, so a DateTimeException is thrown. We catch this exception and print a message to the console.
Understanding these common issues and how to handle them can help you use LocalDate more effectively and avoid potential headaches in your Java programming journey.
Understanding Java’s Date and Time API
Java’s Date and Time API, introduced in Java 8, is a powerful toolset for handling dates and times. It’s designed to be more straightforward and intuitive than the older java.util.Date and java.util.Calendar classes, which were often seen as cumbersome and confusing.
LocalDate and the ISO-8601 Calendar System
One of the key classes in this API is LocalDate, which represents a date without time or time-zone information. LocalDate uses the ISO-8601 calendar system, a widely-used standard for representing dates and times.
The ISO-8601 calendar system represents dates in the format yyyy-MM-dd. For example, January 1, 2022, is represented as ‘2022-01-01’. This format is easy to understand and is used worldwide, making it a great choice for handling dates.
Here’s a simple example of creating a LocalDate instance in this format:
LocalDate date = LocalDate.of(2022, 1, 1);
System.out.println(date);
# Output:
# 2022-01-01
In this example, we’re using the of()
method of the LocalDate class to create an instance representing January 1, 2022. The println()
method then prints this date to the console, resulting in the output ‘2022-01-01’.
Understanding the underlying concepts of the Date and Time API, and how LocalDate fits into this picture, is fundamental to effectively handling dates in Java. With this knowledge, you can leverage the power of LocalDate and the broader Date and Time API to make your Java programming tasks easier and more efficient.
LocalDate in Real-World Applications
LocalDate is not just a theoretical concept; it has practical applications in various real-world scenarios. Understanding these applications can help you better grasp the relevance and utility of LocalDate.
Scheduling Tasks
One common use of LocalDate is in scheduling tasks. For example, you might want to schedule a task to run on a specific date. With LocalDate, you can easily represent this date and check whether the current date matches the scheduled date.
LocalDate scheduledDate = LocalDate.of(2022, 12, 31);
LocalDate currentDate = LocalDate.now();
if (currentDate.equals(scheduledDate)) {
System.out.println("Time to run the task!");
}
# Output (on December 31, 2022):
# Time to run the task!
In this example, we’re scheduling a task to run on December 31, 2022. We then check whether the current date equals the scheduled date. If the dates match, a message is printed to the console.
Formatting Dates for Display
Another common use of LocalDate is in formatting dates for display. For example, you might want to display a date in a specific format on a user interface. With LocalDate and DateTimeFormatter, you can easily achieve this.
LocalDate date = LocalDate.of(2022, 12, 31);
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("MMMM dd, yyyy");
String displayDate = date.format(formatter);
System.out.println(displayDate);
# Output:
# December 31, 2022
In this example, we’re formatting the date December 31, 2022, for display. We create a DateTimeFormatter with the desired format and use it to format the LocalDate instance. The result is the string ‘December 31, 2022’, which is printed to the console.
Further Resources for Mastering LocalDate
- Explore Java’s Date class for handling time zones and daylight saving time.
If you want to delve deeper into the Date class, click here for Java Date Insights!
Additionally, we have gathered some resources on LocalDate and related concepts that you might find helpful:
- Java Time Overview – Dive into Java Time API, a modern and comprehensive time-handling library introduced in Java 8.
Exploring Date Format Options in Java – Discover how to customize date formats in Java applications.
Java 8 Date and Time API Guide – A comprehensive guide to the Date and Time API in Java 8, including LocalDate.
Java LocalDate Tutorial – A detailed tutorial on LocalDate, including methods and examples.
Java API Documentation: LocalDate by Oracle – The official Java documentation for the LocalDate class.
Exploring these resources can provide you with a deeper understanding of LocalDate and its many applications. Happy learning!
Wrapping Up: Mastering LocalDate in Java
In this comprehensive guide, we’ve navigated the world of LocalDate, a fundamental class in Java’s Date and Time API for handling dates without time-zone information.
We began with the basics, demonstrating how to create and manipulate LocalDate instances. We then delved into more advanced topics, such as formatting and parsing dates, and working with different time zones. Along the way, we tackled common issues you might encounter when using LocalDate, such as DateTimeParseException and DateTimeException, and provided solutions to these challenges.
We also explored alternative approaches to handle dates and times in Java, introducing other classes like LocalDateTime and ZonedDateTime. These alternatives offer additional functionalities, such as handling time and time-zone information, and can be used based on your specific needs.
Here’s a quick comparison of the methods we’ve discussed:
Class | Date | Time | Time Zone |
---|---|---|---|
LocalDate | Yes | No | No |
LocalDateTime | Yes | Yes | No |
ZonedDateTime | Yes | Yes | Yes |
Whether you’re just starting out with LocalDate or you’re looking to level up your date handling skills in Java, we hope this guide has given you a deeper understanding of LocalDate and its capabilities.
With its balance of simplicity, versatility, and power, LocalDate is a valuable tool for handling dates in Java. Now, you’re well equipped to harness its power in your Java programming journey. Happy coding!