Bash Regex: Shell Scripting Guide to Regular Expressions
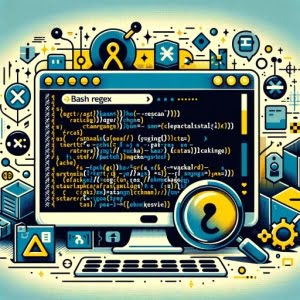
Struggling with regular expressions in Bash? You’re not alone. Many developers find themselves tangled in the web of regex in Bash, but we’re here to help. Regex is an invaluable tool that can unlock powerful string manipulation capabilities. These capabilities can then significantly enhance your scripting prowess.
This guide will walk you through the process of using regex in Bash, from the basics to more advanced techniques. We’ll cover everything from simple pattern matching to complex expressions, as well as alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering regex in Bash!
TL;DR: How Do I Use Regular Expressions in Bash?
You can use regular expressions in Bash with the
=~
operator in anif
statement. The syntax for this would be as follows:if [[ 'Hello World' =~ Hello ]]
. This operator allows you to match a string against a regular expression right within your Bash script.
Here’s a simple example:
if [[ 'Hello World' =~ Hello ]]; then echo 'Match found'; else echo 'Match not found'; fi
# Output:
# 'Match found'
In this example, we’re using the =~
operator to check if the string ‘Hello World’ contains the substring ‘Hello’. If the match is found, it echoes ‘Match found’. If not, it echoes ‘Match not found’. In this case, because ‘Hello World’ does contain ‘Hello’, the output is ‘Match found’.
This is just a basic way to use regular expressions in Bash, but there’s much more to learn about pattern matching and string manipulation. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
Getting Started with Bash Regex
Let’s start with the basics of Bash regex. The heart of regex usage in Bash lies in the =~
operator. This operator is used within an if
statement to match a string against a regular expression.
Here’s a simple example of how to use the =~
operator:
string='Bash regex is powerful'
pattern='powerful'
if [[ $string =~ $pattern ]]; then echo 'Match found'; else echo 'Match not found'; fi
# Output:
# 'Match found'
In this example, we have a string ‘Bash regex is powerful’ and a pattern ‘powerful’. We’re using the =~
operator to check if the string contains the pattern. If the pattern is found within the string, it echoes ‘Match found’. If not, it echoes ‘Match not found’. As the string does contain the pattern ‘powerful’, the output is ‘Match found’.
This is a basic usage of the =~
operator in Bash regex. It allows you to check if a string matches a certain pattern, which can be extremely useful in a variety of scripting scenarios. But remember, this is just the tip of the iceberg when it comes to the power of regex in Bash.
Exploring Complex Regular Expressions in Bash
As you get more comfortable with basic regex in Bash, it’s time to delve into more complex patterns. This includes using character classes, quantifiers, and capture groups.
Character Classes
Character classes allow you to match any character from a specific set. For instance, [a-z]
matches any lowercase letter, while [0-9]
matches any digit. Let’s see this in action:
string='My number is 12345'
pattern='[0-9]+'
if [[ $string =~ $pattern ]]; then echo 'Match found'; else echo 'Match not found'; fi
# Output:
# 'Match found'
In this example, the pattern [0-9]+
matches one or more digits in the string, thus the output is ‘Match found’.
Quantifiers
Quantifiers specify how many instances of a character, group, or character class must be present in the input for a match to be found. For example, a{3}
will match exactly three ‘a’ characters.
string='Bash regex is aaaamazing'
pattern='a{3}'
if [[ $string =~ $pattern ]]; then echo 'Match found'; else echo 'Match not found'; fi
# Output:
# 'Match found'
In this case, the pattern a{3}
matches exactly three consecutive ‘a’ characters, and since our string has ‘aaa’, the output is ‘Match found’.
Capture Groups
Capture groups are a way to treat multiple characters as a single unit. They are created by placing the characters to be grouped inside a set of parentheses ()
. For example, (abc)
is a capture group that matches the exact sequence of ‘abc’.
string='Bash regex is powerful'
pattern='(powerful)'
if [[ $string =~ $pattern ]]; then echo 'Match found'; else echo 'Match not found'; fi
# Output:
# 'Match found'
Here, the pattern (powerful)
matches the exact sequence ‘powerful’ in the string, thus the output is ‘Match found’.
These are just a few examples of the more complex regular expressions you can use in Bash. By understanding and combining these elements, you can create powerful and flexible patterns that can match almost any string.
Alternative Ways to Use Bash Regex
While the =~
operator is a powerful tool for using regex in Bash, it’s not the only way. Two other common methods for using regular expressions in Bash are the grep
command and the sed
command. Let’s explore these alternatives.
Using Grep for Regex in Bash
Grep is a command-line utility used for searching plain-text data for lines that match a regular expression. It’s a powerful tool for regex in Bash. Here’s an example:
echo 'Bash regex is powerful' | grep -E 'powerful'
# Output:
# 'Bash regex is powerful'
In this example, we’re using grep
with the -E
flag (which enables extended regex support) to search for the pattern ‘powerful’ in our string. If the pattern is found, grep
outputs the line that contains the match.
Benefits of using grep
include its flexibility and its ability to handle large amounts of data efficiently. However, as it’s a separate command rather than a built-in Bash operator, it may not be as fast as using =~
for simple pattern matching.
Using Sed for Regex in Bash
Sed, or stream editor, is another command-line utility. It can perform a lot of functions on file data, including regex pattern matching. Here’s how you can use sed
for regex in Bash:
echo 'Bash regex is powerful' | sed -n '/powerful/p'
# Output:
# 'Bash regex is powerful'
In this example, we’re using sed
with the -n
flag (which suppresses automatic printing) and the p
command (which prints the line) to search for the pattern ‘powerful’. If the pattern is found, sed
outputs the line.
While sed
is a very powerful tool, it can be overkill for simple pattern matching. Its syntax can also be more complex than grep
or =~
. However, for complex string manipulation tasks, sed
is an excellent choice.
In conclusion, while the =~
operator is a great tool for regex in Bash, grep
and sed
offer alternative approaches that can be more suitable depending on your specific needs.
Common Issues with Bash Regex
While regex in Bash is a powerful tool, it’s not without its challenges. Two common issues are dealing with special characters and handling whitespace. Let’s explore these issues and their solutions.
Escaping Special Characters
Special characters such as *
, .
, ?
, and others have special meanings in regex. If you want to match these characters literally, you need to escape them using a backslash \
.
Here’s an example:
string='Bash regex is *powerful*'
pattern='\*powerful\*'
if [[ $string =~ $pattern ]]; then echo 'Match found'; else echo 'Match not found'; fi
# Output:
# 'Match found'
In this case, we want to match the pattern ‘powerful‘ in the string. To do this, we escape the *
characters in the pattern with \
. As a result, the output is ‘Match found’.
Handling Whitespace
Whitespace characters like spaces and tabs can sometimes cause unexpected behavior in regex. One way to handle this is by using the [:space:]
character class, which matches any whitespace character.
Here’s an example:
string='Bash regex is powerful'
pattern='Bash regex is[[:space:]]+powerful'
if [[ $string =~ $pattern ]]; then echo 'Match found'; else echo 'Match not found'; fi
# Output:
# 'Match found'
In this example, the pattern ‘Bash regex is[[:space:]]+powerful’ matches ‘Bash regex is’ followed by one or more spaces, followed by ‘powerful’. As a result, even though there are multiple spaces in the string, the output is ‘Match found’.
These are just a couple of the common issues you might encounter when working with regex in Bash. By understanding these issues and how to solve them, you can use regex more effectively in your Bash scripts.
Understanding Regular Expressions
To fully grasp the power of regex in Bash, it’s essential to understand what regular expressions are and how they work at a fundamental level.
What are Regular Expressions?
Regular expressions, or regex, are a sequence of characters that form a search pattern. This pattern can be used to match, locate, and manage text. Regex patterns can range from simple characters to complex expressions for matching IP addresses, email addresses, and much more.
Here’s a simple regex example:
string='Bash regex is powerful'
pattern='powerful'
if [[ $string =~ $pattern ]]; then echo 'Match found'; else echo 'Match not found'; fi
# Output:
# 'Match found'
In this example, the pattern ‘powerful’ is a simple regex that matches the exact sequence of characters ‘powerful’. When used with the =~
operator in Bash, it checks if the string ‘Bash regex is powerful’ contains this sequence.
The Theory Behind Regex
The power of regex comes from its use of metacharacters – special characters that have unique meanings in a regex pattern. For example, the .
metacharacter matches any character except a newline, while the *
metacharacter matches zero or more of the preceding element.
By combining metacharacters with regular characters, you can create complex patterns that can match almost any kind of text.
The Importance of String Manipulation in Bash
String manipulation is a crucial aspect of Bash scripting. Whether you’re renaming files, extracting data from text, or validating user input, being able to manipulate and analyze strings is key to creating effective scripts. And regex, with its ability to match and manage complex patterns, is an invaluable tool for string manipulation in Bash.
In conclusion, understanding the fundamentals of regex and string manipulation can significantly enhance your Bash scripting skills. With this knowledge, you’re well on your way to mastering regex in Bash.
Broadening Bash Regex Applications
Now that we’ve covered the basics and some advanced techniques of Bash regex, let’s discuss how you can apply these skills in larger scripts or projects. Regex can play a critical role in various tasks, such as data processing and log file analysis.
Data Processing with Bash Regex
Imagine you have a large dataset that you need to clean and process. Bash regex can be a powerful tool for this task. You can use regex to find and replace certain patterns, remove unnecessary characters, and format your data in a way that’s useful for your specific needs.
# Let's assume we have a dataset with inconsistent date formats, and we want to standardize them to 'YYYY-MM-DD'.
data='Date: 2020/12/31, Date: 31-12-2020'
# We can use regex to find and replace the dates to the desired format.
processed_data=$(echo $data | sed -E 's|([0-9]{4})/([0-9]{2})/([0-9]{2})|\1-\2-\3|g' | sed -E 's|([0-9]{2})-([0-9]{2})-([0-9]{4})|\3-\2-\1|g')
echo $processed_data
# Output:
# 'Date: 2020-12-31, Date: 2020-12-31'
In this example, we’re using the sed
command with regex to find dates in the formats ‘YYYY/MM/DD’ and ‘DD-MM-YYYY’ and replace them with the format ‘YYYY-MM-DD’. This is just one of the many ways you can use Bash regex for data processing.
Log File Analysis with Bash Regex
Another common use of Bash regex is log file analysis. If you’re dealing with large log files, regex can help you find errors, track user activity, or extract important information.
# Let's say we want to find all ERROR logs in our log file.
log_file='log.txt'
# We can use grep with regex to find all lines that contain 'ERROR'.
grep 'ERROR' $log_file
# This will output all lines in the log file that contain 'ERROR'.
In this example, we’re using the grep
command with the regex pattern ‘ERROR’ to find all error logs in our log file. This can be extremely useful for troubleshooting and debugging.
Further Resources for Bash Regex Mastery
To continue your journey in mastering Bash regex, here are some additional resources that you might find useful:
- GNU Bash Manual: The official manual for Bash, including a section on pattern matching and string manipulation.
- Regular-Expressions.info: A comprehensive resource on regular expressions, with detailed explanations and tutorials.
- The Art of Command Line: A GitHub repository with practical tips and tricks for using the command line effectively, including using regex.
Wrapping Up: Mastering Bash Regex
In this comprehensive guide, we’ve delved into the world of regular expressions, or regex, in Bash. We’ve explored how this powerful tool can be used for pattern matching and string manipulation, enhancing your Bash scripting prowess.
We began with the basics, learning how to use the =~
operator for simple pattern matching. We then moved on to more complex regular expressions, exploring character classes, quantifiers, and capture groups. We’ve also looked at alternative approaches to using regex in Bash, such as the grep
and sed
commands.
Along the way, we’ve tackled common challenges you might face when using Bash regex, such as escaping special characters and handling whitespace. We’ve provided solutions and workarounds for these issues, equipping you with the knowledge to overcome these obstacles.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity |
---|---|---|
=~ Operator | Moderate | Low |
grep Command | High | Moderate |
sed Command | High | High |
Whether you’re just starting out with Bash regex or you’re looking to upskill, we hope this guide has given you a deeper understanding of regex in Bash and its capabilities. With this knowledge in hand, you’re well equipped to tackle any string manipulation task in Bash. Happy scripting!