Learn Python: How To Loop Through a List
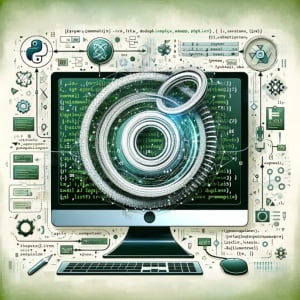
Looping through a list is a Python technique we use often when developing software for use at IOFLOOD. In our experience, looping through lists provides a structured way to process each element in a list. Today’s article explains how to loop through a list in Python, providing valuable insights and examples to help our dedicated server customers.
In this comprehensive guide, we will take you on a journey through the process of looping through lists in Python. From the basic for loop to more advanced techniques, we’ve got you covered.
So buckle up, and let’s start exploring the power of Python lists and loops!
TL;DR: How Do I Loop Through a List in Python?
You can use a
for
loop to iterate over a list in Python with the syntax,for i in sample_list:...
.
Here’s a simple example:
my_list = [1, 2, 3, 4, 5]
for i in my_list:
print(i)
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, we’ve created a list called my_list
with the numbers from 1 to 5. The for
loop goes through each item in the list (which we’ve named i
for each iteration), and then prints it. The result is each number in our list printed on a new line.
Intrigued? Keep reading for more detailed explanations and advanced techniques for looping through lists in Python.
Table of Contents
The Basics of Python List Looping
Python’s for
loop is the most common tool used to iterate over a list. Let’s look at a simple example:
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
# Output:
# apple
# banana
# cherry
In this example, we have a list called fruits
containing three string elements. The for
loop iterates over each item in the list, and the print
function displays each item. The variable fruit
represents the current item for each iteration.
Advantages of Using For Loops
The for
loop is straightforward, readable, and efficient for most use cases. It allows you to perform the same operation on each item in a list, which is a common requirement in programming.
Potential Pitfalls in Using For Loops
You should be careful not to modify the list while you’re looping over it. Python doesn’t behave well when the size of a list changes during iteration, and it can lead to unexpected results or errors.
If you need to modify the list, it’s better to create a new list or use list comprehension, which we’ll discuss later.
Advanced Strategies for List Looping
As you become more comfortable with Python, you’ll want to explore more advanced techniques for looping through lists. These techniques can make your code more efficient and easier to read.
List Comprehension
List comprehension is a concise way to create lists based on existing lists. Here’s how you can use it to loop through a list:
numbers = [1, 2, 3, 4, 5]
squares = [number**2 for number in numbers]
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In this example, we’re creating a new list called squares
that contains the square of each number in the numbers
list. The for
loop and the squaring operation are all done in one line, making the code cleaner and more readable.
Enumerate Function
[The enumerate
function is useful when](python enumerate) you need to access the index of each item in the loop:
fruits = ['apple', 'banana', 'cherry']
for i, fruit in enumerate(fruits):
print(f'{i}: {fruit}')
# Output:
# 0: apple
# 1: banana
# 2: cherry
In this example, enumerate(fruits)
generates pairs of index and value for each item in the fruits
list. The for
loop then unpacks these pairs into i
and fruit
.
Zip Function
The zip
function can be used to loop through multiple lists at the same time:
fruits = ['apple', 'banana', 'cherry']
colors = ['red', 'yellow', 'red']
for fruit, color in zip(fruits, colors):
print(f'The {fruit} is {color}.')
# Output:
# The apple is red.
# The banana is yellow.
# The cherry is red.
In this example, zip(fruits, colors)
generates pairs of items from the fruits
and colors
lists. The for
loop then unpacks these pairs into fruit
and color
.
Alternative Approaches to Looping
Python offers a variety of ways to loop through lists, each with its own advantages and potential use cases. Let’s delve into some of these alternative approaches and see how they can enhance your Python coding skills.
While Loops
While loops provide a way to loop through a list based on a condition. Here’s an example using a while loop to iterate through a list:
numbers = [1, 2, 3, 4, 5]
i = 0
while i < len(numbers):
print(numbers[i])
i += 1
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, the while loop continues to print and increment i
as long as i
is less than the length of the numbers
list. Note that while loops can be more prone to errors (like infinite loops) if not handled carefully.
Lambda and Map Functions
Lambda functions provide a way to create small, anonymous functions, and can be used in conjunction with the map function to apply a function to every item in a list:
numbers = [1, 2, 3, 4, 5]
squares = list(map(lambda x: x**2, numbers))
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In this example, the lambda function lambda x: x**2
squares each item in the numbers
list. The map function applies this lambda function to each item in the list, and the list function converts the result back into a list.
While powerful, lambda and map functions can be harder to read and understand for beginners, so use them judiciously.
Troubleshooting Issues in List Iteration
While looping through lists in Python is generally straightforward, you might encounter some common issues that can trip up even experienced programmers. Let’s discuss these problems and how to resolve them.
Handling Index Errors
One of the most common issues when looping through lists is the IndexError
. This error typically occurs when you’re trying to access an index that doesn’t exist in the list. Here’s an example:
fruits = ['apple', 'banana', 'cherry']
print(fruits[3])
# Output:
# IndexError: list index out of range
In this example, we’re trying to print the item at index 3 of the fruits
list. However, since Python list indices start at 0, there is no index 3 in a list of three items. To avoid this error, make sure you’re not trying to access an index greater than len(list) - 1
.
Modifying Lists During Iteration
Another common pitfall is trying to modify a list while iterating over it. This can lead to unexpected behavior. For example, consider the following code:
numbers = [1, 2, 3, 4, 5]
for i in numbers:
if i == 3:
numbers.remove(i)
print(numbers)
# Output:
# [1, 2, 4, 5]
In this example, we’re trying to remove the number 3 from the list while we’re looping over it. The output is as expected, but if you try to remove multiple items, you might skip some due to the way Python handles list resizing during iteration.
It’s generally safer to create a new list or use list comprehension to modify a list based on some condition.
Understanding Lists and Iteration
Before we delve deeper into looping through lists in Python, it’s essential to grasp two fundamental concepts: Python’s list data type and the concept of iteration.
Python Lists: A Quick Recap
In Python, a list is a collection of items that are ordered and changeable. Lists are written with square brackets, and they can contain any type of data: numbers, strings, other lists, and so on.
# a list of numbers
numbers = [1, 2, 3, 4, 5]
# a list of strings
fruits = ['apple', 'banana', 'cherry']
# a list of mixed data types
mixed = [1, 'banana', [2, 3, 4]]
Iteration: The Heart of Loops
Iteration is a general term for taking each item of something, one after another. When you use a loop to go through each item in a list, that’s iteration. The item that is being used during each loop is called the iterator
.
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
# Output:
# apple
# banana
# cherry
In this example, fruit
is the iterator. For each loop (or iteration), fruit
takes on the value of the next item in the fruits
list.
Understanding these concepts is crucial for mastering list looping in Python. With this knowledge, you can better understand how loops work and how to use them effectively in your Python programming.
Further Resources for Python
If you’re interested in diving deeper into Python lists, there are plenty of related concepts to explore. List manipulation techniques (like sorting, slicing, and reversing) and advanced list comprehension techniques can all add to your Python toolkit.
Here are a few other resources that you might find helpful:
- Python Lists: Streamlining Your Programming Workflow: Learn how to streamline your programming workflow by harnessing the power of Python lists, and explore their wide range of applications in various coding scenarios.
Python List Extend Method: Usage and Examples: IOFlood’s guide explains how to use the extend() method in Python to add multiple elements to a list, providing usage examples and scenarios where it can be useful.
Removing Duplicates from a List in Python: IOFlood’s tutorial explores different techniques to remove duplicates from a list in Python, including using set(), list comprehension, and the built-in remove() method.
Python Documentation on Data Structures: The official Python documentation provides a comprehensive tutorial on the various data structures available in Python, such as lists, dictionaries, and sets.
Python for Everybody: This Coursera course, taught by Charles Severance, provides a beginner-friendly introduction to Python programming and its applications in various fields.
Introduction to Python Programming: Offered by edX, this course covers the fundamentals of Python programming, including syntax, data structures, and algorithms.
Real Python: Real Python is an online platform that offers a wide range of Python tutorials, articles, and videos covering various topics and skill levels. It provides practical examples and tips for Python enthusiasts and developers.
Wrapping Up: Python List Iteration
In this guide, we’ve journeyed through the world of Python list iteration, starting from the basics and moving up to more advanced techniques. We’ve seen how the humble for
loop can unlock the potential of Python lists, and how other constructs like while
loops and map
and lambda
functions offer alternative ways to loop through lists.
We’ve also delved into potential pitfalls, such as IndexError
and the risks of modifying a list while iterating over it. But with the right knowledge and a bit of caution, these issues are easily avoided.
So whether you’re a newcomer to Python or an experienced developer looking to brush up on your skills, I hope you’ve found this guide to Python list iteration helpful. Remember, the best way to learn is by doing, so don’t be afraid to get your hands dirty and start looping!