Printf Java: String Formatting Guide
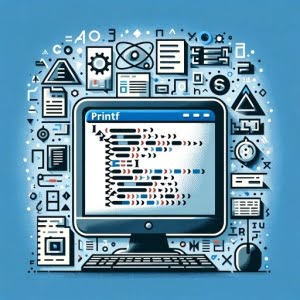
Are you finding it challenging to format your strings in Java? You’re not alone. Many developers find themselves struggling with this task, but there’s a tool that can make this process a breeze.
Like a skilled formatter, Java’s printf function can help you create beautifully formatted strings. This function can be your powerful ally in crafting precise and well-structured strings.
This guide will walk you through using the printf function in Java. We’ll explore printf’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering printf in Java!
TL;DR: How Do I Use the printf Function in Java?
The printf function in Java is used to format and print strings with the syntax,
System.out.printf("Strings", "to", "Print")
. It allows you to create formatted strings by using placeholders that get replaced by specified values.
Here’s a simple example:
System.out.printf("Hello %s", "World");
// Output:
// 'Hello World'
In this example, we use the printf function to print a string. The %s
inside the string is a placeholder that gets replaced by the value we specify, in this case, ‘World’. The result is a formatted string that says ‘Hello World’.
This is just a basic way to use the printf function in Java, but there’s much more to learn about formatting strings in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Printf Java: Basic Usage for Beginners
The printf function in Java is a powerful tool for formatting strings. It works by using placeholders, known as format specifiers, in a string. These placeholders are then replaced by the values you specify.
Printf Java: Basic String Formatting
Let’s start with a simple example:
String name = "Alice";
System.out.printf("Hello, %s", name);
// Output:
// 'Hello, Alice'
In this example, %s
is a format specifier that represents a string. It acts as a placeholder in the initial string. When the printf function is executed, it replaces %s
with the value of the variable name
.
The printf function in Java is very flexible, allowing you to format strings in different ways. For instance, you can use it to print integers, floating-point numbers, and other data types by using different format specifiers.
Printf Java: Formatting Numerical Values
Here’s an example of how to use printf to format an integer:
int age = 25;
System.out.printf("I am %d years old", age);
// Output:
// 'I am 25 years old'
In this example, %d
is the format specifier for an integer.
Using the printf function in Java can make your strings more dynamic and your code more readable. However, it’s crucial to use the correct format specifier for each data type. If you use the wrong specifier, you could end up with unexpected results or errors.
Printf Java: Advanced Techniques
As you become more comfortable with the printf function in Java, you can start to explore its more advanced features. These include using different format specifiers and flags to achieve more complex string formatting.
Printf Java: Multiple Format Specifiers
Let’s consider an example where we use multiple format specifiers:
String name = "Alice";
int age = 25;
System.out.printf("Hello, %s. You are %d years old.", name, age);
// Output:
// 'Hello, Alice. You are 25 years old.'
In this example, we use two format specifiers, %s
for a string and %d
for an integer. The printf function replaces these specifiers with the values of name
and age
, respectively.
Printf Java: Using Flags
Printf also allows you to use flags for additional formatting options. For example, you can use the -
flag to left-justify the output, or the 0
flag to pad numbers with leading zeros.
Here’s an example of using the -
flag:
int number = 25;
System.out.printf("|%10d|", number);
System.out.printf("|%-10d|", number);
// Output:
// '| 25|'
// '|25 |'
In the first printf statement, we use the %10d
format specifier to indicate that we want to print an integer with a width of 10 characters. By default, printf right-justifies the output, so the number 25 is printed with leading spaces. In the second printf statement, we use the %-10d
format specifier to left-justify the output, so the number 25 is printed with trailing spaces.
While these advanced techniques can make your code more flexible and your output more precise, they can also make your code more complex. It’s important to use these features judiciously to maintain the readability of your code.
Alternative Ways to Format Strings in Java
While the printf function is a powerful tool for string formatting in Java, it’s not the only option. Other methods, such as the String.format method and the MessageFormat class, offer alternative approaches to this task.
String.format: A Printf Alternative
The String.format method works similarly to printf, but instead of printing the formatted string, it returns it. This can be useful when you want to store the formatted string for later use.
Here’s an example:
String name = "Alice";
int age = 25;
String formattedString = String.format("Hello, %s. You are %d years old.", name, age);
System.out.println(formattedString);
// Output:
// 'Hello, Alice. You are 25 years old.'
In this example, we use String.format to create a formatted string and store it in the formattedString
variable. We then print this string using System.out.println.
MessageFormat: Advanced String Formatting
For more complex formatting needs, you can use the MessageFormat class. This class supports advanced features like formatting numbers, dates, and messages.
Here’s an example of how to use MessageFormat to format a string:
import java.text.MessageFormat;
String name = "Alice";
int age = 25;
String formattedString = MessageFormat.format("Hello, {0}. You are {1} years old.", name, age);
System.out.println(formattedString);
// Output:
// 'Hello, Alice. You are 25 years old.'
In this example, we use the {0}
and {1}
placeholders to represent the values of name
and age
, respectively.
While these alternative methods offer additional flexibility and functionality, they also come with their own complexities. It’s important to choose the method that best fits your specific needs and the complexity level you’re comfortable with.
Troubleshooting Printf Java: Common Issues and Solutions
While using printf in Java, you might encounter some common issues, especially related to format specifier errors. Let’s go over these potential pitfalls and their solutions.
Issue: Incorrect Format Specifier
Using an incorrect format specifier is a common mistake. For example, using %d
for a string or %s
for an integer will result in an IllegalFormatConversionException
.
String name = "Alice";
try {
System.out.printf("Hello, %d", name);
} catch (Exception e) {
e.printStackTrace();
}
// Output:
// java.util.IllegalFormatConversionException: d != java.lang.String
In this example, we intentionally used %d
(which is for integers) for a string variable. As a result, we got an IllegalFormatConversionException
.
Solution: Match Format Specifiers with Correct Data Types
Ensure that you match the format specifiers with the correct data types. For instance, use %s
for strings, %d
for integers, and %f
for floating-point numbers.
String name = "Alice";
System.out.printf("Hello, %s", name);
// Output:
// 'Hello, Alice'
In this corrected example, we used %s
for a string variable, and the code executed without any exceptions.
Best Practices and Optimization Tips
- Always ensure that your format specifiers match the data types of your variables.
- Try to keep your format strings simple and readable. Avoid overly complex format strings that can make your code hard to understand.
- When working with multiple format specifiers, ensure that they are in the correct order. The order of the specifiers should match the order of the variables in the argument list.
- Remember to use the printf function’s advanced features judiciously. While these features can provide powerful formatting options, they can also make your code more complex.
Java’s String Data Type and Printf
Java’s string data type is more than just a simple sequence of characters. It’s a powerful data type that comes with a rich set of built-in methods for manipulating text. One such method is the printf function, which fits into the larger picture of string formatting in Java.
Understanding Format Specifiers and Flags
At the heart of the printf function are format specifiers, which are placeholders for the values you want to format into the string. Each specifier starts with a %
character, followed by a character that indicates the type of data to be formatted. For example, %s
is used for strings, %d
for integers, and %f
for floating-point numbers.
Here’s a simple example:
System.out.printf("%s is %d years old", "Alice", 25);
// Output:
// 'Alice is 25 years old'
In this example, %s
is replaced by the string ‘Alice’, and %d
is replaced by the integer 25.
In addition to format specifiers, printf also supports flags that modify the output in various ways. For example, you can use the -
flag to left-justify the output, or the 0
flag to pad numbers with leading zeros. These flags provide additional control over the formatting of your output, allowing you to create precisely formatted strings.
Understanding the string data type and how the printf function fits into its functionality is crucial to mastering string formatting in Java. With this knowledge, you can create more dynamic and readable code, enhancing both your productivity and the quality of your software.
Printf Java: Beyond Basic Formatting
The printf function in Java is not just a tool for string formatting, but a fundamental part of larger Java applications. Its relevance extends beyond simple tasks, playing a crucial role in data presentation, debugging, and logging.
Exploring Related Concepts
As you master the printf function, it’s worthwhile to explore related concepts in Java. String manipulation, for instance, is a broad area that covers various methods for modifying, splitting, joining, and comparing strings. Understanding these concepts can significantly enhance your ability to work with text data in Java.
Additionally, there are other methods for formatting strings in Java, such as the String.format method and the DecimalFormat class. These methods offer different features and capabilities, giving you more options for handling string formatting tasks.
Further Resources for Mastering Printf in Java
To deepen your understanding of printf and related concepts in Java, consider exploring these resources:
- IOFlood’s Java User Input Guide – Learn how to parse and convert user input into appropriate data types.
Exploring Java Scanner – Master Scanner techniques for interactive Java programs.
Java Print Basics – Understand Java print statements for outputting text.
Oracle’s Java Documentation is an excellent resource for understanding the printf function and other formatting options.
Baeldung’s Guide to Java String Format provides a comprehensive overview of ‘printf’ in Java.
Digital Ocean’s Java Printf Method Tutorial offers a deep dive into the printf method, covering basic usage and flags.
Exploring these resources can help you master not only printf, but also the broader area of string manipulation in Java. By continually learning and practicing, you can become more proficient and efficient in your Java programming tasks.
Wrapping Up
In this comprehensive guide, we’ve explored the printf function in Java, a powerful tool for formatting strings. We’ve seen how it can be used to format and print strings, and how it fits into the larger picture of string manipulation in Java.
We began with the basics, understanding how printf works with different data types using format specifiers. We then delved into more advanced usage, exploring how the use of multiple format specifiers and flags can achieve complex string formatting.
Along the way, we tackled common issues that you might encounter when using printf, such as incorrect format specifier usage, and provided solutions to help you overcome these challenges. We also looked at alternative approaches to handle string formatting, such as the String.format method and the MessageFormat class, giving you a sense of the broader landscape of tools for string formatting in Java.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity |
---|---|---|
Printf | High | Moderate |
String.format | Moderate | Low |
MessageFormat | High | High |
Whether you’re just starting out with printf in Java or you’re looking to level up your string formatting skills, we hope this guide has given you a deeper understanding of printf and its capabilities.
With its balance of flexibility and precision, printf is a powerful tool for string formatting in Java. Now, you’re well equipped to enjoy those benefits. Happy coding!