Java Print Functions: Basic to Advanced Methods
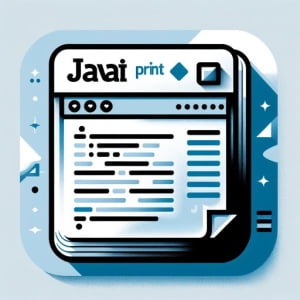
Ever wondered how to print something in Java? Like a digital printing press, Java’s print functions can output your data to the console. Whether you’re a beginner just starting out or an advanced user looking for more complex solutions, Java’s print functions are a crucial part of your toolkit.
This guide will walk you through everything you need to know about printing in Java, from the basics to more advanced techniques. We’ll cover everything from simple print statements to more complex formatting and file output. So, let’s dive in and start mastering Java print!
TL;DR: How Do I Print in Java?
To print in Java, you use the
System.out.print()
orSystem.out.println()
functions. These functions allow you to output text to the console, which is incredibly useful for debugging, displaying results, and interacting with users.
Here’s a simple example:
System.out.println('Hello, World!');
// Output:
// 'Hello, World!'
In this example, we use the System.out.println()
function to print the string ‘Hello, World!’ to the console. The println()
function automatically adds a newline character at the end, so the next output will start on a new line.
This is a basic way to print in Java, but there’s much more to learn about outputting data in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Mastering Basic Java Print Functions
In Java, the System.out.print()
and System.out.println()
functions are the basic building blocks for outputting data to the console. Let’s break down how they work, when to use each one, and what potential pitfalls you might encounter.
Understanding System.out.print()
and System.out.println()
The System.out.print()
function outputs data to the console and does not add a newline character at the end. This means any subsequent output will continue on the same line.
Here’s an example:
System.out.print('Hello, ');
System.out.print('World!');
// Output:
// 'Hello, World!'
In this example, we print ‘Hello, ‘ and ‘World!’ on the same line because we used System.out.print()
for both.
On the other hand, System.out.println()
works similarly, but it adds a newline character at the end of the output. This means any subsequent output will start on a new line.
Let’s look at an example:
System.out.println('Hello, ');
System.out.println('World!');
// Output:
// 'Hello, '
// 'World!'
In this example, ‘Hello, ‘ and ‘World!’ are printed on separate lines because we used System.out.println()
for both.
Choosing Between System.out.print()
and System.out.println()
The choice between System.out.print()
and System.out.println()
depends on how you want to format your output. If you want your output to continue on the same line, use System.out.print()
. If you want your output to start on a new line, use System.out.println()
.
Potential Pitfalls
One common mistake beginners make is forgetting that System.out.print()
does not add a newline character. This can lead to output that is all jumbled together on one line. Always remember to add a newline character or use System.out.println()
when you want your output on a new line.
Diving Deeper: Advanced Java Print Scenarios
As you become more comfortable with Java’s basic print functions, you’ll find yourself needing more advanced techniques for specific scenarios. Two such instances involve formatting output with printf()
, and printing to a file. Let’s delve into these scenarios, complete with code examples and best practices.
Formatting Output with printf()
Java’s printf()
function allows you to format your output in a variety of ways. This is especially useful when you need to display data in a specific format, such as limiting the number of decimal places in a floating-point number.
Here’s an example of how to use printf()
to format a floating-point number:
double pi = 3.14159265359;
System.out.printf('Pi is approximately %.2f.', pi);
// Output:
// 'Pi is approximately 3.14.'
In this example, the %.2f
in the printf()
function is a format specifier. It tells Java to print the floating-point number pi
with exactly two digits after the decimal point.
Printing to a File
While printing to the console is useful for debugging and simple programs, you’ll often need to print data to a file for more complex applications. Java makes this easy with the PrintWriter
class.
Here’s an example of how to print to a file in Java:
import java.io.PrintWriter;
import java.io.FileNotFoundException;
try {
PrintWriter writer = new PrintWriter('output.txt');
writer.println('Hello, World!');
writer.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
// Output:
// 'output.txt' file is created with 'Hello, World!' as its content.
In this example, we create a PrintWriter
object and use it to write ‘Hello, World!’ to a file named ‘output.txt’. If the file doesn’t exist, Java will create it for us. If the file cannot be created or opened (for example, due to a permissions issue), a FileNotFoundException
will be thrown.
Remember, always close your PrintWriter
when you’re done with it to free up system resources and ensure that all your data gets written to the file.
Exploring Alternatives: PrintWriter
and PrintStream
Java provides alternative approaches to printing, such as using a PrintWriter
or PrintStream
. These classes are more flexible and powerful than the basic System.out.print()
and System.out.println()
, but they also require a bit more understanding of Java’s I/O system.
Harnessing the Power of PrintWriter
The PrintWriter
class is a character-oriented class, as opposed to byte-oriented. This means it’s better for writing text data, such as characters and strings.
Here’s an example of how to use PrintWriter
to print to the console:
import java.io.PrintWriter;
PrintWriter writer = new PrintWriter(System.out);
writer.println('Hello, World!');
writer.flush();
// Output:
// 'Hello, World!'
In this example, we create a PrintWriter
object and use it to print ‘Hello, World!’ to the console. The flush()
method is called to ensure that all data is actually written out. If you forget to call flush()
, some of your data may not be written.
Utilizing PrintStream
for Advanced Printing
The PrintStream
class is byte-oriented and is suitable for outputting binary data. However, it can also be used for printing text. In fact, System.out
is actually a PrintStream
.
Here’s an example of how to use PrintStream
to print to the console:
import java.io.PrintStream;
PrintStream stream = new PrintStream(System.out);
stream.println('Hello, World!');
// Output:
// 'Hello, World!'
In this example, we create a PrintStream
object and use it to print ‘Hello, World!’ to the console. The usage is very similar to PrintWriter
.
Making the Right Choice
When choosing between System.out.print()
, PrintWriter
, and PrintStream
, consider what you’re trying to accomplish. If you’re just printing text to the console, System.out.print()
is the simplest and most straightforward option. If you need to write text to a file or a network socket, PrintWriter
is a good choice. If you need to output binary data, PrintStream
is the way to go.
Like any programming task, printing in Java can sometimes present challenges. Two common issues involve problems with character encoding and optimization of print performance. Let’s explore these issues, their solutions, and some best practices.
Tackling Character Encoding Issues
Java uses Unicode for its character set, which can cause issues when you’re trying to print characters that are not part of the ASCII character set. For example, accented characters or characters from non-Latin scripts might not print correctly.
Here’s an example of a character encoding issue:
System.out.println('Jalapeño');
// Output:
// 'Jalape?o'
In this example, the ‘ñ’ character is not part of the ASCII character set, so it prints as a question mark.
To solve this issue, make sure your Java environment is configured to use the correct character encoding. You can set the default character encoding using the file.encoding
system property:
java -Dfile.encoding=UTF-8 MyProgram
Optimizing Print Performance
If you’re printing a lot of data, the performance of your print operations can become a concern. One way to optimize print performance is to use a BufferedWriter
in combination with a PrintWriter
:
import java.io.BufferedWriter;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
PrintWriter writer = new PrintWriter(new BufferedWriter(new OutputStreamWriter(System.out)));
writer.println('Hello, World!');
writer.flush();
// Output:
// 'Hello, World!'
In this example, we wrap System.out
in a BufferedWriter
before passing it to the PrintWriter
. The BufferedWriter
improves performance by buffering the output, which means it writes the data in larger chunks instead of one character at a time.
Understanding Java’s I/O System
Java’s I/O (Input/Output) system is an integral part of the language, enabling communication between your program and the outside world. This communication could be with the console, a file, a network socket, or even another program. Understanding this system is fundamental to mastering Java print functions.
The Role of Streams in Java
In Java, a stream is a sequence of data. There are two types of streams: input streams, which read data into your program, and output streams, which write data from your program. The System.out
object we’ve been using to print data is an output stream.
Here’s an example of using an output stream to write data:
System.out.println('Hello, World!');
// Output:
// 'Hello, World!'
In this example, System.out
is an output stream that writes data to the console.
Byte Streams vs Character Streams
Java has two types of streams: byte streams and character streams. Byte streams (InputStream
and OutputStream
) are used for binary input and output of 8-bit bytes. They’re useful for binary data, like images.
Character streams (Reader
and Writer
), on the other hand, are used for character input and output. They’re based on the Unicode character set and are useful for text data, like strings.
The PrintWriter
and PrintStream
we discussed earlier are character streams, making them ideal for printing text data in Java.
By understanding Java’s I/O system, you can better understand how the print functions work and how to use them effectively in your programs.
Extending Your Java Print Knowledge
Printing in Java isn’t just about displaying messages on the console or writing data to a file. It’s a fundamental part of larger Java applications, playing a crucial role in areas such as logging and debugging. Let’s explore these applications and discuss some related topics you might find interesting.
Java Print for Logging
In larger applications, logging is essential for tracking events and diagnosing problems. Java’s print functions can be used to write log messages to the console or a file. These messages can then be analyzed to understand the application’s behavior and identify any issues.
Here’s an example of using System.out.println()
for logging:
System.out.println('Starting application...');
// Output:
// 'Starting application...'
In this example, we print a message to the console indicating that the application is starting. This could be useful when debugging startup issues.
Debugging with Java Print
Java’s print functions are also useful for debugging. By printing out variable values, you can understand how your program’s state changes over time and identify any unexpected behavior.
Here’s an example of using System.out.println()
for debugging:
int x = 5;
System.out.println('x = ' + x);
// Output:
// 'x = 5'
In this example, we print the value of the variable x
to the console. This can help us understand what x
is at this point in the program.
Further Resources for Mastering Java Print
If you’re interested in diving deeper into Java’s print functions and related topics, here are some resources you might find useful:
- Beginner’s Guide to Java User Input – Master the art of prompting users for input and validating their responses in Java applications.
Using printf for Formatted Output – Learn how to use printf for precise control over output formatting.
Exploring System.out.println Usage – Master System.out.println for debugging and logging in Java applications.
Programiz’s Basic Input Output in Java is a guide about handling input and output in Java.
JavaTpoint’s How to Print in Java shares different methods and formats to display output in Java with examples.
W3Schools’ Java Print Variables is a detailed tutorial on how to print variable values in Java.
By exploring these resources and experimenting with Java’s print functions, you can become a master of printing in Java and enhance your overall Java programming skills.
Wrapping Up: Mastering Java Print Functions
In this comprehensive guide, we’ve explored the ins and outs of printing in Java, from the basic print functions to more advanced techniques and considerations.
We began with the basics, understanding how to use System.out.print()
and System.out.println()
to output data to the console. We then delved into more advanced techniques, such as formatting output with printf()
, and printing to a file using PrintWriter
. We also discussed alternative approaches to printing in Java, such as using PrintStream
and PrintWriter
for more complex scenarios.
Along the way, we tackled common issues you might encounter when printing in Java, such as character encoding problems and performance considerations, providing you with solutions and tips for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
System.out.print() | Simple, easy to use | Does not add a newline character |
System.out.println() | Adds a newline character | Less control over formatting |
printf() | Allows for complex formatting | More complex to use |
PrintWriter and PrintStream | More flexible and powerful | Requires understanding of Java’s I/O system |
Whether you’re just starting out with Java or you’re looking to enhance your Java print skills, we hope this guide has given you a deeper understanding of Java’s print functions and their capabilities.
With its balance of simplicity and power, Java’s print functions are a crucial part of any Java programmer’s toolkit. Happy coding!