Java’s System.out.println Explained: How does it Work?
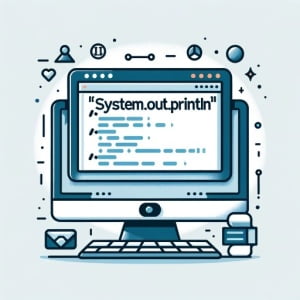
Ever found yourself puzzled about how to output a line of text to the console in Java? You’re not alone. Many developers, especially those new to Java, often find themselves scratching their heads when it comes to using the system.out.println command. Think of it as a town crier in the world of Java – it broadcasts your messages loud and clear to the console.
This guide will walk you through the ins and outs of the system.out.println command in Java. We’ll cover everything from the basics to more advanced uses, and even discuss alternative approaches. We’ll also delve into troubleshooting common issues and provide tips for best practices.
So, let’s dive in and start mastering system.out.println in Java!
TL;DR: How Do I Use system.out.println in Java?
To print a line of text to the console in Java, you use the
system.out.println
command. This command is a simple and effective way to display messages, debug your code, or understand the flow of your program.
Here’s a simple example:
System.out.println('Hello, World!');
// Output:
// 'Hello, World!'
In this example, we use the system.out.println
command to print the string ‘Hello, World!’ to the console. This is a basic use of the command, but it’s capable of much more.
If you’re interested in learning more about the
system.out.println
command, including its advanced uses and alternative approaches, keep reading. We’ll delve into all the details in the sections to come.
Table of Contents
- Basic Use of system.out.println
- Advanced Usage of system.out.println
- Exploring Alternatives to system.out.println
- Troubleshooting Common Problems with system.out.println
- Best Practices and Optimization with system.out.println
- Understanding the System Class in Java
- The out Object in Java
- The println Method in Java
- The Concept of Standard Output in Java
- Applying system.out.println in Real-World Scenarios
- Wrapping Up: Mastering system.out.println in Java
Basic Use of system.out.println
The system.out.println
command in Java is a fundamental tool for any beginner. Its main function is to print text to the console, which can be incredibly useful for debugging, displaying program outputs, or just simple greetings.
Step-by-Step Guide to Using system.out.println
Here’s a simple step-by-step guide on how to use the system.out.println
command:
- Open your Java integrated development environment (IDE).
- Start a new Java class or use an existing one.
- In your main method, type
System.out.println('Your message here');
Here’s how it looks in a code block:
public class HelloWorld {
public static void main(String[] args) {
System.out.println('Hello, World!');
}
}
// Output:
// 'Hello, World!'
In this example, we’ve created a simple Java class named HelloWorld
. Inside the main
method, we’ve used the System.out.println
command to print ‘Hello, World!’ to the console.
Pros and Cons of Using system.out.println
Like any other command, system.out.println
has its advantages and disadvantages.
Pros:
- It’s straightforward and easy to use.
- It’s a quick way to debug your code.
- It’s great for displaying program outputs.
Cons:
- It can clutter your console if overused.
- It’s not suitable for complex outputs.
- It’s not the most efficient way to output data in a production environment.
Despite these drawbacks, system.out.println
remains a vital tool in a Java developer’s arsenal, especially for beginners. As you gain more experience, you’ll learn when and how to use system.out.println
effectively.
Advanced Usage of system.out.println
As you become more comfortable with Java, you’ll discover that system.out.println
can do more than just print simple text messages. It can handle different data types and offers formatting options to display your output neatly.
Using system.out.println with Different Data Types
Java supports various data types, and system.out.println
can handle most of them. Here’s an example of how you can use it with integers, floating-point numbers, and booleans:
public class DataTypesDemo {
public static void main(String[] args) {
int number = 10;
double pi = 3.14;
boolean isJavaFun = true;
System.out.println(number);
System.out.println(pi);
System.out.println(isJavaFun);
}
}
// Output:
// 10
// 3.14
// true
In this example, we’ve declared an integer, a double, and a boolean. We’ve then used System.out.println
to print each of these values to the console.
Formatting Output with system.out.println
system.out.println
also allows you to format your output using string concatenation and placeholders. Here’s an example:
public class FormattingDemo {
public static void main(String[] args) {
String name = 'John';
int age = 30;
System.out.println('Hello, ' + name + '. You are ' + age + ' years old.');
}
}
// Output:
// 'Hello, John. You are 30 years old.'
In this example, we’ve used string concatenation to combine strings and variables into a single output. This allows us to create more complex and meaningful messages.
Mastering the advanced uses of system.out.println
will enable you to display a wider range of outputs and create more interactive and informative console applications.
Exploring Alternatives to system.out.println
While system.out.println
is a fundamental tool in Java, there are other related commands that can perform similar tasks. These include System.out.print
and System.out.printf
, which offer different benefits and use cases.
The System.out.print Command
The System.out.print
command is similar to system.out.println
, but it does not add a new line at the end of the output. This means that multiple System.out.print
commands will print their output on the same line.
Here’s an example:
public class PrintDemo {
public static void main(String[] args) {
System.out.print('Hello, ');
System.out.print('World!');
}
}
// Output:
// 'Hello, World!'
In this example, we’ve used two System.out.print
commands to print ‘Hello, ‘ and ‘World!’. Because System.out.print
does not add a new line, both outputs appear on the same line.
The System.out.printf Command
The System.out.printf
command allows you to format your output with placeholders. This is especially useful for formatting numbers and aligning text.
Here’s an example:
public class PrintfDemo {
public static void main(String[] args) {
String name = 'John';
int age = 30;
System.out.printf('Hello, %s. You are %d years old.', name, age);
}
}
// Output:
// 'Hello, John. You are 30 years old.'
In this example, we’ve used the %s
and %d
placeholders to insert a string and an integer into the output. This allows us to format the output neatly and efficiently.
Deciding Between system.out.println, System.out.print, and System.out.printf
Choosing between system.out.println
, System.out.print
, and System.out.printf
depends on your specific needs:
- Use
system.out.println
for simple outputs and debugging. - Use
System.out.print
when you want to print multiple items on the same line. - Use
System.out.printf
for formatted outputs and complex messages.
Understanding these alternatives to system.out.println
will give you more flexibility and control over your console output in Java.
Troubleshooting Common Problems with system.out.println
Like any command, system.out.println
can sometimes lead to unexpected results or errors. Here, we’ll discuss some common issues you might encounter and provide solutions to help you overcome them.
Null Pointer Exception with system.out.println
One common error is the NullPointerException
. This occurs when you try to print an object that has not been initialized.
public class NullDemo {
public static void main(String[] args) {
String str = null;
System.out.println(str.length());
}
}
// Output:
// Exception in thread 'main' java.lang.NullPointerException
In this example, we tried to print the length of a string that was set to null
, resulting in a NullPointerException
. To fix this, you need to ensure that your object is properly initialized before you try to use it.
Misuse of system.out.println in Multithreaded Applications
system.out.println
is thread-safe, which means it can be used safely in multithreaded applications. However, due to its thread-safety, it can slow down your application if used excessively in multithreaded environments. Consider using alternatives like System.out.print
or System.out.printf
for better performance in such cases.
Incorrect Use of system.out.println for Debugging
While system.out.println
is a useful tool for debugging, it’s not always the best choice. It can clutter your console and make it harder to find the information you need. Consider using a dedicated logging framework for more complex debugging needs.
Best Practices and Optimization with system.out.println
- Avoid Overuse: Overusing
system.out.println
can clutter your console and slow down your application. Use it sparingly and consider alternatives for complex outputs. - Initialize Your Objects: Ensure that your objects are properly initialized before you try to print them to avoid
NullPointerExceptions
. - Use Logging for Debugging: For more advanced debugging needs, consider using a dedicated logging framework instead of
system.out.println
.
Understanding these common issues and best practices will help you use system.out.println
more effectively and efficiently in your Java programs.
Understanding the System Class in Java
In Java, System
is a final class present in java.lang
package. It contains several useful class fields and methods. It cannot be instantiated, which means you cannot create an object of the System
class. Instead, all its fields and methods are static, making it easier to access them.
System.out.println('Hello, World!');
// Output:
// 'Hello, World!'
In this code, System
is the class name.
The out Object in Java
The out
object is an instance of the PrintStream
class and provides many methods to write data to various outputs. This object is part of the System
class and is made available to all Java programs.
System.out.println('Hello, World!');
// Output:
// 'Hello, World!'
In this code, out
is the object of the PrintStream
class.
The println Method in Java
The println
method prints data to the console and moves the cursor to a new line. It can take arguments of various data types, including int
, char
, long
, float
, boolean
, double
, String
, and more.
System.out.println('Hello, World!');
// Output:
// 'Hello, World!'
In this code, println
is the method of the PrintStream
class.
The Concept of Standard Output in Java
The term ‘standard output’ (stdout) refers to the default data stream where a program writes its output data. In Java, standard output is typically the console or screen. The System.out.println
command prints the given data to the standard output stream (the console, in most cases).
Understanding these fundamental concepts is crucial to mastering system.out.println
and other similar commands in Java. It allows you to comprehend not only what these commands do, but also why they work the way they do.
Applying system.out.println in Real-World Scenarios
The system.out.println
command is not just a tool for beginners learning Java. It’s a versatile command that finds its place in larger projects and real-world scenarios as well. Whether it’s for debugging a complex application, logging important information, or displaying output to the user, system.out.println
is a handy command to have in your toolkit.
Related Commands and Functions
In real-world applications, system.out.println
often works in tandem with other related commands and functions. For instance, you might use system.out.println
with System.err.println
for error messages, or with System.in
for reading user input.
Here’s an example of how you might use system.out.println
and System.in
together in a real-world application:
import java.util.Scanner;
public class InputDemo {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println('Enter your name: ');
String name = scanner.nextLine();
System.out.println('Hello, ' + name + '!');
}
}
// Output:
// Enter your name:
// [user enters 'John']
// Hello, John!
In this example, we’ve used System.in
to read user input and system.out.println
to display a greeting to the user. This is a common use case in interactive console applications.
Further Resources for Mastering Java Console Output
If you’re interested in learning more about system.out.println
and related topics, Click Here to learn how to implement user-friendly input prompts and error messages in Java applications.
Additionally, here are a few resources that offer more in-depth information:
- Scanner in Java: Basics – Explore the Scanner class in Java for user input processing.
Using Print Statements in Java – Learn different print methods available in Java for displaying data.
Java Documentation: Class PrintStream – The official Java documentation provides detailed information about the
PrintStream
class, which includessystem.out.println
.Java Console Input and Output – This tutorial from W3Schools covers console input and output in Java.
Java for Beginners – This interactive course from Codecademy covers various topics, including
system.out.println
.
Wrapping Up: Mastering system.out.println in Java
In this comprehensive guide, we’ve explored the ins and outs of system.out.println
, a fundamental command in Java for outputting text to the console.
We began with the basics, explaining how to use system.out.println
to print simple messages. We then delved into more advanced usage, highlighting its ability to handle different data types and format outputs. We also introduced alternative commands, such as System.out.print
and System.out.printf
, and discussed when to use each one.
Along the way, we tackled common issues you might encounter when using system.out.println
, such as NullPointerExceptions
and performance issues in multithreaded applications, providing solutions and best practices for each challenge.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
system.out.println | Simple, versatile, good for debugging | Can clutter console if overused |
System.out.print | Prints on same line, good for compact outputs | Does not move cursor to new line |
System.out.printf | Allows formatted outputs, good for complex messages | Slightly more complex than other methods |
Whether you’re just starting out with Java or you’re looking to deepen your understanding of its console output commands, we hope this guide has been a valuable resource.
Mastering system.out.println
and its alternatives gives you the tools to display a wide range of outputs and create more interactive and informative console applications. Happy coding!