Java Scanner Class: For Beginners to Experts
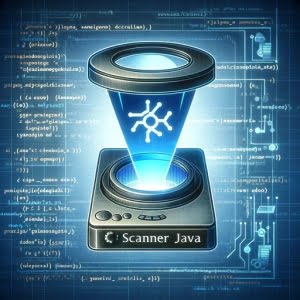
Ever found yourself struggling to read user input in Java? You’re not alone. Many developers find this task a bit challenging, but there’s a tool that can make this process a breeze.
Think of the Scanner class in Java as a real-world scanner, capable of reading and interpreting input. It’s a powerful utility that can seamlessly read different types of input, from strings and integers to floating-point numbers.
In this guide, we’ll walk you through everything you need to know about using the Scanner class in Java, from basic use to advanced techniques. We’ll cover everything from creating a Scanner object, using its methods to read input, to handling common issues and even discussing alternatives.
So, let’s dive in and start mastering the Scanner class in Java!
TL;DR: How Do I Use the Scanner Class in Java?
You can use the Scanner class to read input, created with the syntax,
Scanner scanner = new Scanner();
. It allows you to read different types of input from the user, making it a versatile tool for any Java developer.
Here’s a simple example:
Scanner scanner = new Scanner(System.in);
String input = scanner.nextLine();
System.out.println(input);
# Output:
# [Whatever the user types]
In this example, we create a Scanner object named scanner
that reads input from System.in
(the keyboard). We then use the nextLine()
method to read a line of text from the user. The input is stored in the input
variable, which we then print to the console.
This is a basic way to use the Scanner class in Java, but there’s much more to learn about handling user input effectively. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Using Scanner in Java: A Beginner’s Guide
- Advanced Scanner Usage: Delimiters, Files, and Exceptions
- Alternatives to Scanner: BufferedReader and Console
- Troubleshooting Common Scanner Issues in Java
- Understanding Java I/O: Streams and the Java I/O API
- Scanner Java: Beyond Basic Input
- Wrapping Up: The Java Scanner Class
Using Scanner in Java: A Beginner’s Guide
The Scanner class in Java is a simple text scanner which can parse primitive types and strings. It breaks the input into tokens using a delimiter which by default is a whitespace.
Reading Strings
Let’s start with the basics: reading a string. Here’s how you can do it:
Scanner scanner = new Scanner(System.in);
System.out.println("What's your name?");
String name = scanner.nextLine();
System.out.println("Hello, " + name + "!");
# Output:
# What's your name?
# [User enters their name]
# Hello, [User's name]!
In this example, we ask the user for their name and use the nextLine()
method to read the entire line of input (including any spaces).
Reading Integers
You can also use the Scanner class to read integer input. Here’s how:
Scanner scanner = new Scanner(System.in);
System.out.println("What's your favorite number?");
int number = scanner.nextInt();
System.out.println("Your favorite number is " + number + ".");
# Output:
# What's your favorite number?
# [User enters a number]
# Your favorite number is [User's number].
In this case, we use the nextInt()
method to read an integer from the user’s input.
Reading Floating-Point Numbers
Finally, you can use the Scanner class to read floating-point numbers. Here’s an example:
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a decimal number:");
float decimalNumber = scanner.nextFloat();
System.out.println("The decimal number you entered is " + decimalNumber + ".");
# Output:
# Enter a decimal number:
# [User enters a decimal]
# The decimal number you entered is [User's decimal].
In this example, we use the nextFloat()
method to read a floating-point number from the user’s input.
Advantages and Pitfalls of Using the Scanner Class
The Scanner class is a popular choice for reading input in Java because it’s easy to use and comes with a variety of methods for reading different types of input. However, it’s not without its pitfalls. For one, the Scanner class can be slower compared to other methods of reading input in Java, especially when dealing with large amounts of data. Furthermore, the Scanner class can throw exceptions if the input does not match the expected type (for example, if you use nextInt()
and the user enters a string).
Advanced Scanner Usage: Delimiters, Files, and Exceptions
Now that we’ve covered the basics, let’s delve into some of the more advanced features of the Scanner class.
Using Delimiters with Scanner
By default, Scanner uses whitespace to separate tokens. However, you can customize this using the useDelimiter()
method. This method takes a string argument, which can be a regular expression, allowing you to specify exactly how the input should be split.
Here’s an example of using a comma as a delimiter:
Scanner scanner = new Scanner("apple,banana,carrot");
scanner.useDelimiter(",");
while(scanner.hasNext()) {
System.out.println(scanner.next());
}
# Output:
# apple
# banana
# carrot
In this example, we’re reading from a string instead of System.in
. The string contains three words separated by commas. We use the useDelimiter()
method to tell Scanner to split the input on commas, not spaces.
Reading Files with Scanner
Scanner can also be used to read files. Here’s an example:
try {
File file = new File("filename.txt");
Scanner fileScanner = new Scanner(file);
while (fileScanner.hasNextLine()) {
System.out.println(fileScanner.nextLine());
}
fileScanner.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
# Output:
# [Contents of filename.txt]
In this example, we create a File object for a file named filename.txt
, and then create a Scanner object that reads from this file. We use a while loop to read and print each line in the file. Note that we also need to handle the FileNotFoundException, which is checked exception.
Handling Exceptions with Scanner
When using the Scanner class, it’s important to handle exceptions properly. For example, if you’re using nextInt()
and the user enters a string, a InputMismatchException
will be thrown. Here’s how you can handle this:
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number:");
try {
int number = scanner.nextInt();
System.out.println("You entered: " + number);
} catch (InputMismatchException e) {
System.out.println("That's not a valid number!");
}
# Output:
# Enter a number:
# [User enters a non-numeric value]
# That's not a valid number!
In this example, we use a try-catch block to handle the InputMismatchException
. If the user enters a non-numeric value, the catch block will be executed and a custom error message will be printed.
While the Scanner class offers a lot of flexibility and functionality, it’s important to be aware of its limitations. For instance, it can be slower than other methods for reading large amounts of data, and it doesn’t handle encoding issues. However, for many applications, its simplicity and ease of use make it a great choice.
Alternatives to Scanner: BufferedReader and Console
While the Scanner class is a versatile tool for reading input in Java, it’s not the only option. Let’s explore some alternatives and their pros and cons.
BufferedReader: Fast and Efficient
BufferedReader is a Java class used for reading text from an input source (like a file or keyboard input) buffering characters so as to provide efficient reading of characters, arrays, and lines. Here’s a simple example of how to use it:
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Enter your name:");
String name = reader.readLine();
System.out.println("Hello, " + name + "!");
} catch (IOException e) {
e.printStackTrace();
}
# Output:
# Enter your name:
# [User enters their name]
# Hello, [User's name]!
In this example, we create a BufferedReader to read input from System.in
. We use the readLine()
method to read a line of text from the user. Note that we need to handle the IOException, which can be thrown by the readLine()
method.
The main advantage of BufferedReader is its efficiency. It’s faster than Scanner when reading large amounts of data, thanks to its internal buffer. However, it’s more complex to use, and doesn’t have the convenient methods for reading different types of input that Scanner has.
Console: Direct and Simple
The Console class is another alternative for reading input in Java. It’s less versatile than Scanner and BufferedReader, but can be simpler to use for basic needs. Here’s an example:
Console console = System.console();
System.out.println("Enter your name:");
String name = console.readLine();
System.out.println("Hello, " + name + "!");
# Output:
# Enter your name:
# [User enters their name]
# Hello, [User's name]!
In this example, we use System.console()
to get a Console object, and then use its readLine()
method to read a line of input from the user.
The advantage of Console is its simplicity. However, it’s less flexible than Scanner and BufferedReader, and it doesn’t work in some environments (for example, in an integrated development environment, or IDE).
In conclusion, while the Scanner class is a powerful tool for reading input in Java, there are alternatives that can be more suitable depending on your needs. It’s important to understand the advantages and disadvantages of each, so you can choose the right tool for the job.
Troubleshooting Common Scanner Issues in Java
While the Scanner class in Java is a powerful tool for reading input, it’s not without its challenges. Let’s delve into some common issues you might encounter when using the Scanner class and discuss how to troubleshoot them.
InputMismatchException: Handling Incorrect Input Types
One common issue is the InputMismatchException
, which occurs when the input does not match the expected type. For example, if you use nextInt()
and the user enters a string, an InputMismatchException
will be thrown.
Here’s how you can handle this:
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a number:");
try {
int number = scanner.nextInt();
System.out.println("You entered: " + number);
} catch (InputMismatchException e) {
System.out.println("That's not a valid number!");
}
# Output:
# Enter a number:
# [User enters a non-numeric value]
# That's not a valid number!
In this example, we use a try-catch block to handle the InputMismatchException
. If the user enters a non-numeric value, the catch block will be executed and a custom error message will be printed.
Resource Leaks: Closing the Scanner
Another issue to be aware of is resource leaks. When you’re done using a Scanner object, you should close it to free up system resources. However, when you close a Scanner object that reads from System.in
, it also closes System.in
, which means you can’t read from System.in
again in your program. If you need to read from System.in
again later, you should not close the Scanner.
NoSuchElementException: Reading Past the End of Input
The NoSuchElementException
is thrown by the next()
, nextInt()
, and other similar methods if no more tokens are available. To avoid this, you should always check if there’s another token available using the hasNext()
, hasNextInt()
, etc. methods.
In conclusion, while the Scanner class is a handy tool for reading input in Java, it’s important to be aware of potential pitfalls and how to handle them. By understanding these issues and how to troubleshoot them, you can use the Scanner class more effectively in your Java programs.
Understanding Java I/O: Streams and the Java I/O API
To fully grasp the role and utility of the Scanner class, it’s crucial to first understand how input and output (I/O) work in Java. This involves a discussion on streams and the Java I/O API.
Java I/O: A Brief Overview
In Java, I/O is performed through streams. A stream represents a sequence of data. There are two main types of streams: byte streams (which read and write data byte by byte) and character streams (which read and write data character by character).
The Java I/O API provides classes for system input and output through data streams. These classes can be found in the java.io
package.
The Role of Scanner in Java I/O
The Scanner class is a part of the java.util
package. It’s a simple text scanner which can parse primitive types and strings using regular expressions. While it’s not a part of the Java I/O API, the Scanner class works with streams to provide a convenient interface for reading input.
Here’s a basic example of how the Scanner class works with Java I/O:
Scanner scanner = new Scanner(System.in);
System.out.println("Enter your name:");
String name = scanner.nextLine();
System.out.println("Hello, " + name + "!");
# Output:
# Enter your name:
# [User enters their name]
# Hello, [User's name]!
In this example, System.in
is an instance of InputStream
, which is a kind of byte stream. The Scanner class takes this stream as input and provides methods like nextLine()
to read from this stream in a more convenient way.
In conclusion, the Scanner class plays a significant role in Java I/O. It provides a high-level, user-friendly interface for reading input, making it a valuable tool for any Java developer.
Scanner Java: Beyond Basic Input
The Scanner class in Java is not just for simple user input. It can also be used in larger programs, such as interactive applications and file processing programs. Let’s explore these applications in more detail.
Interactive Applications with Scanner
In interactive applications, the Scanner class can be used to read user commands. For example, you could create a text-based game where the user enters commands to control their character. The Scanner class makes it easy to read these commands and respond appropriately.
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a command:");
String command = scanner.nextLine();
// Process the command...
# Output:
# Enter a command:
# [User enters a command]
In this example, we read a command from the user and then process it. The processing would depend on the specific commands your application supports.
File Processing with Scanner
The Scanner class can also be used for file processing. For example, you could write a program that reads a CSV file and prints out each record.
try {
File file = new File("records.csv");
Scanner fileScanner = new Scanner(file);
while (fileScanner.hasNextLine()) {
String record = fileScanner.nextLine();
System.out.println(record);
}
fileScanner.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
# Output:
# [Contents of records.csv]
In this example, we create a Scanner that reads from a file, and then use a while loop to read and print each line in the file.
Further Resources for Mastering Scanner in Java
If you want to learn more about the Scanner class in Java and how to use it effectively, here are some resources that you might find helpful:
- IOFlood’s Java User Input Guide explains how to parse and convert user input into various data types.
printf in Java – Explore printf in Java for formatted output.
System.out.println in Java Usage – Learn about System.out.println for outputting text in Java.
Java Documentation: Scanner – The official Java documentation on the Scanner class and its methods.
JavaTpoint’s Java Scanner Class provides a detailed explanation of the Scanner class.
GeeksforGeeks’ Java.util.Scanner class in Java provides a detailed overview of the Scanner class.
Remember, practice is key when it comes to mastering any new concept in programming. So, don’t forget to experiment with the Scanner class and try to incorporate it into your own Java projects.
Wrapping Up: The Java Scanner Class
In this comprehensive guide, we’ve delved into the intricacies of the Scanner class in Java, a versatile and user-friendly tool for reading and interpreting user input.
We kicked off our exploration with the basics, understanding how to create a Scanner object and use its various methods to interpret different types of input, including strings, integers, and floating-point numbers. We then ventured into more advanced territory, discussing the use of delimiters, reading from files, and handling exceptions with the Scanner class.
Along the way, we tackled common issues that you might encounter when using the Scanner class, such as InputMismatchException
and resource leaks, providing solutions and workarounds for each challenge. We also looked at alternatives to the Scanner class for reading input in Java, such as BufferedReader and Console, equipping you with a broader understanding of the landscape of input tools in Java.
Here’s a quick comparison of these input methods:
Method | Flexibility | Speed | Ease of Use |
---|---|---|---|
Scanner | High | Moderate | High |
BufferedReader | Moderate | High | Moderate |
Console | Low | High | High |
Whether you’re just starting out with the Scanner class or you’re looking to deepen your understanding of Java input methods, we hope this guide has been a valuable resource. The Scanner class, with its flexibility and ease of use, is a powerful tool in any Java developer’s toolkit. Now, you’re well-equipped to harness its capabilities in your Java programs. Happy coding!