Python With: The Resource Cleanup Statement
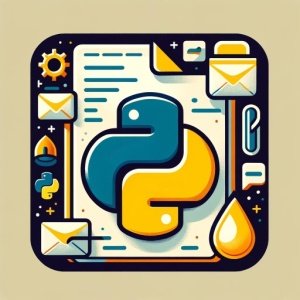
Are you grappling with resource management in Python? You’re not alone. Many developers find it challenging to handle resources efficiently and reliably in their Python programs. Think of Python’s ‘with’ statement as a diligent janitor – it ensures that cleanup is done efficiently and reliably.
Whether you’re working with files, network connections, or any other resources, understanding how to use the ‘with’ statement in Python can significantly streamline your coding process.
In this guide, we’ll walk you through the process of using the ‘with’ statement in Python, from the basics to more advanced techniques.
Let’s get started!
TL;DR: What is the ‘with’ Statement in Python and How Do I Use It?
The
with
statement in Python is a built-in function used for efficient management of resources. It ensures that resources are properly cleaned up after use, even if errors occur during the process. This is particularly useful when working with files, network connections, or any other resources that need to be properly closed or cleaned up after use.
Here’s a simple example:
with open('file.txt', 'r') as f:
contents = f.read()
print(contents)
# Output:
# 'Contents of the file.txt'
In this example, the ‘with’ statement is used to open a file named ‘file.txt’. The file is automatically closed after it is read, even if an error occurs during the reading process. This ensures that the file is properly closed and the resources are cleaned up, preventing any potential resource leaks.
This is just the tip of the iceberg when it comes to using the ‘with’ statement in Python. Continue reading for a more detailed explanation, advanced usage scenarios, and alternative approaches to resource management in Python.
Table of Contents
- Understanding Python ‘with’ Statement: Basic Use
- Advanced Resource Management with Python ‘with’ Statement
- Exploring Alternative Resource Management Techniques in Python
- Navigating Common Issues with Python’s ‘with’ Statement
- Diving Deep: The ‘with’ Statement and Context Managers in Python
- Python’s ‘with’ Statement: Beyond the Basics
- Wrapping Up: Mastering Python’s ‘with’ Statement
Understanding Python ‘with’ Statement: Basic Use
The ‘with’ statement in Python is a powerful tool that works in tandem with context managers to manage resources. But what does this mean? Let’s break it down.
A context manager in Python is a simple ‘protocol’ (or interface) that your object needs to follow so it can be used with the ‘with’ statement. It’s defined by the methods __enter__()
and __exit__()
.
Here’s a simple example of how the ‘with’ statement works:
with open('example.txt', 'r') as file:
data = file.read()
print(data)
# Output:
# 'Contents of the example.txt'
In this example, the ‘with’ statement is used to open a file named ‘example.txt’. The ‘open’ function returns a file object, and it is this object that acts as the context manager.
When the ‘with’ statement is executed, Python evaluates the expression, calls the __enter__()
method on the resulting value (the context manager), and assigns whatever __enter__()
returns to the variable given by ‘as’.
When the statements within the ‘with’ block are done, Python makes sure the __exit__()
method is called on the context manager object, even if an error occurred. This means the file is properly closed, even if an error occurs while processing the file.
This is the main advantage of using the ‘with’ statement – it ensures that clean-up code is executed. This can help prevent bugs and issues caused by forgetting to release resources or when errors occur during resource processing.
However, it’s important to note that not all objects can be used with the ‘with’ statement out of the box. The object must implement the context manager protocol (__enter__()
and __exit__()
methods), or Python will raise an error. This is one of the potential pitfalls when using the ‘with’ statement.
Advanced Resource Management with Python ‘with’ Statement
As you become more comfortable with the ‘with’ statement in Python, you can start to explore its more advanced uses. One such use is managing multiple resources at once.
Consider a scenario where you need to read data from one file and write it to another. You could do this with two separate ‘with’ statements, but Python allows you to streamline this process by using a single ‘with’ statement to manage both files.
Here’s an example:
with open('source.txt', 'r') as source, open('destination.txt', 'w') as destination:
data = source.read()
destination.write(data)
In this example, the ‘with’ statement is used to open two files – ‘source.txt’ for reading and ‘destination.txt’ for writing. The data is read from the source file and written to the destination file. Both files are automatically closed at the end of the ‘with’ block, even if an error occurs while processing the files.
This not only makes the code more concise and readable, but it also ensures that both resources are properly managed and cleaned up, regardless of what happens during the execution of the block.
Remember, the ‘with’ statement in Python is a powerful tool for resource management, and understanding how to use it effectively can greatly enhance your programming skills.
Exploring Alternative Resource Management Techniques in Python
While the ‘with’ statement is an effective way to manage resources in Python, it’s not the only method available. Let’s explore some alternative approaches that you might find useful, especially when dealing with more complex scenarios.
Manually Closing Resources
One straightforward approach is to manually close resources once you’re done with them. This can be done using the ‘close’ method provided by many resource types.
Here’s an example:
file = open('file.txt', 'r')
data = file.read()
file.close()
print(data)
# Output:
# 'Contents of the file.txt'
In this example, the ‘open’ function is used to open a file named ‘file.txt’, and the ‘close’ method is called to close the file after reading its content. While this method is simple, it’s also risky. If an error occurs before the ‘close’ method is called, the resource might not be properly released.
Using try/finally Blocks
To ensure resources are always properly released, you can use a ‘try/finally’ block. The ‘finally’ block is executed no matter how the ‘try’ block is exited, ensuring that cleanup code is always run.
Here’s an example:
file = open('file.txt', 'r')
try:
data = file.read()
finally:
file.close()
print(data)
# Output:
# 'Contents of the file.txt'
In this example, the ‘open’ function is used to open a file named ‘file.txt’, and the ‘close’ method is called in the ‘finally’ block to ensure the file is closed even if an error occurs while reading its content.
While these methods can be effective, they require more manual management and are more prone to errors than using the ‘with’ statement. Therefore, it’s generally recommended to use the ‘with’ statement for resource management in Python, especially for beginners and intermediate-level programmers.
While Python’s ‘with’ statement is a powerful tool for resource management, it’s not without its quirks. Let’s discuss some common issues you might encounter and how to navigate them.
Forgetting to Use ‘with’ Statement
One common mistake is forgetting to use the ‘with’ statement when dealing with a resource that needs cleanup. This can lead to resource leaks, as the resource might not be properly released.
Consider the following code:
file = open('file.txt', 'r')
data = file.read()
print(data)
# Output:
# 'Contents of the file.txt'
In this example, the file is opened and read, but it’s not properly closed. If this code is part of a larger program that opens many files without closing them, it can lead to a resource leak and potentially crash the program.
The solution is simple: always use the ‘with’ statement when dealing with resources that need cleanup. This ensures that the resources are properly released, even if an error occurs.
Using Objects That Don’t Support Context Management
Another common issue is trying to use the ‘with’ statement with an object that doesn’t support context management. Remember, to use an object with the ‘with’ statement, the object must define __enter__()
and __exit__()
methods.
If you try to use an object that doesn’t support context management with the ‘with’ statement, Python will raise a TypeError. To avoid this, always ensure that the object you’re using with the ‘with’ statement supports context management.
Python’s ‘with’ statement is a powerful tool, but like any tool, it takes practice to use effectively. Keep these considerations in mind, and you’ll be well on your way to mastering resource management in Python.
Diving Deep: The ‘with’ Statement and Context Managers in Python
To fully understand the power of the ‘with’ statement in Python, it’s crucial to delve into its inner workings and the concept of context managers.
The Mechanics of the ‘with’ Statement
The ‘with’ statement is a control-flow structure whose basic structure is: with EXPRESSION as VARIABLE:
. Here’s a simple example:
with open('file.txt', 'r') as file:
data = file.read()
print(data)
# Output:
# 'Contents of the file.txt'
When the ‘with’ statement is executed, Python evaluates the EXPRESSION
, calls the __enter__()
method on the resulting value, and assigns whatever __enter__()
returns to VARIABLE
. When the ‘with’ block is exited, Python calls the __exit__()
method on the value that __enter__()
returned.
The Role of Context Managers
In Python, a context manager is any object that defines methods __enter__()
and __exit__()
. These methods are used to set up and tear down a context for a block of code, hence the name ‘context manager’.
When a context manager is used in a ‘with’ statement, the __enter__()
method is called at the beginning of the block, and the __exit__()
method is called at the end of the block. This ensures that resources are properly set up and cleaned up, regardless of how the block is exited.
Why is the ‘with’ Statement Important for Resource Management?
The ‘with’ statement is crucial for efficient resource management in Python. It ensures that resources, like files or network connections, are properly cleaned up after use, even if errors occur during their use. This can help prevent bugs and crashes caused by resource leaks, making your Python programs more robust and reliable.
Understanding the ‘with’ statement and context managers is a fundamental part of mastering resource management in Python. With this knowledge, you can write cleaner, more efficient, and more reliable Python code.
Python’s ‘with’ Statement: Beyond the Basics
While we’ve covered the basics and some advanced uses of the ‘with’ statement in Python, it’s important to understand its relevance in larger scripts or projects. This can include working with databases, network connections, and other resources that require efficient management.
The ‘with’ Statement in Larger Projects
In larger projects, the ‘with’ statement can significantly streamline your code by ensuring that resources are properly managed. For instance, when working with databases, the ‘with’ statement can be used to ensure that the database connection is properly closed after use, preventing potential resource leaks.
Exploring Related Concepts
If you’re interested in diving deeper into resource management in Python, you might want to explore related concepts like the ‘contextlib’ module. This module provides utilities for creating and working with context management functions, giving you more control and flexibility over your resource management.
For instance, the ‘contextlib’ module provides the ‘closing()’ function, which returns a context manager that closes the object upon exit, even if it doesn’t implement a proper __exit__()
method. This can be useful when working with objects that don’t natively support the ‘with’ statement.
Further Resources for Mastering Python’s ‘with’ Statement
To further enhance your understanding of the ‘with’ statement and resource management in Python, here are a few external resources that you might find helpful:
- This Python OS module tutorial by IOFlood explores practical use cases and projects involving “os” in Python.
This Python Path Module guide explains the details of creating, manipulating, and validating file paths.
Python “open()” for File Creation can help you create files dynamically to store data in Python programs.
Real Python’s ‘with’ statement and context managers guide offers a detailed understanding on ensuring resources are efficiently managed during Python programming.
Python’s official ‘contextlib’ module documentation offers insights into the tools for creating and working with context managers.
Python’s official ‘with’ statement documentation explores ‘with’ statement fundamentals and its usage in Python programming.
Remember, mastering a programming concept like the ‘with’ statement in Python is a journey, not a destination. Don’t be afraid to explore, experiment, and learn at your own pace. Happy coding!
Wrapping Up: Mastering Python’s ‘with’ Statement
In this comprehensive guide, we’ve explored the ins and outs of the ‘with’ statement in Python, a built-in feature for efficient and reliable resource management.
We began with the basics, understanding the ‘with’ statement’s purpose and its simple use cases. We then delved into more advanced usage, managing multiple resources simultaneously, and even examined alternative approaches for handling resources in Python.
Along the way, we’ve tackled common issues and considerations when using the ‘with’ statement, such as forgetting to use it or attempting to use it with objects that don’t support context management. We provided solutions and workarounds for these potential pitfalls, equipping you with the knowledge to avoid them in your coding journey.
We also took a deep dive into the mechanics of the ‘with’ statement and the concept of context managers in Python, understanding how they work under the hood to ensure efficient resource management.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
‘with’ Statement | Efficient resource management, handles errors | Requires objects to support context management |
Manually Closing Resources | Straightforward, no special requirements | Risk of forgetting to release resources |
try/finally Blocks | Ensures cleanup code is always run | More manual management required |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your resource management skills, we hope this guide has given you a deeper understanding of the ‘with’ statement and its capabilities.
With its efficient and reliable approach to resource management, the ‘with’ statement is a powerful tool in Python programming. Happy coding!