Python Path Libraries and Functions Guide
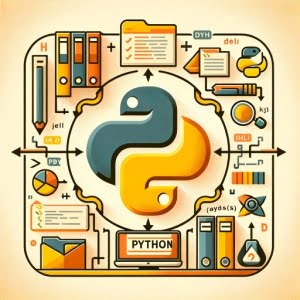
Are you finding it challenging to navigate through file paths in Python? You’re not alone. Many developers find themselves puzzled when it comes to manipulating file paths in Python. Think of Python’s file path handling as a compass – precise and essential for various tasks.
Whether you’re dealing with file I/O operations, web development, or even debugging, understanding how to manipulate file paths in Python can significantly streamline your coding process.
In this guide, we’ll walk you through the process of file path manipulation in Python, from the basics to more advanced techniques. We’ll cover everything from the os.path
module, its functions, as well as alternative approaches like the pathlib
module.
Let’s get started!
TL;DR: How Do I Manipulate File Paths in Python?
Python’s
os.path
module provides a collection of tools for manipulating file paths. Here’s a simple example:
import os
path = '/home/user/documents'
filename = 'file.txt'
full_path = os.path.join(path, filename)
print(full_path)
# Output:
# '/home/user/documents/file.txt'
In this example, we import the os
module and define a path and filename. We then use os.path.join()
to combine the path and filename into a full file path. The print()
function is used to display the full file path.
This is just a basic way to manipulate file paths in Python. There’s much more to learn about file path manipulation, including more advanced techniques. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with os.path
Python’s os.path
module is your first stop when it comes to manipulating file paths. It’s a part of Python’s standard library, which means it’s readily available for you to use. This module provides functions for creating, parsing, and manipulating file paths in a way that’s independent of the underlying operating system. This is crucial as different operating systems handle file paths differently.
Let’s start with a basic example of using os.path
to join two paths together. This is a common task you’ll perform when working with file paths. Here’s how to do it:
import os
# define the parts of the file path
path = '/home/user/documents'
filename = 'report.txt'
# use os.path.join to combine the parts
full_path = os.path.join(path, filename)
print(full_path)
# Output:
# '/home/user/documents/report.txt'
In this example, os.path.join()
is used to combine the directory path and the filename into a full file path. This function takes care of inserting the correct path separator (/
on Unix and \
on Windows) between the parts. This is one of the advantages of using os.path
– you don’t have to worry about the specifics of the underlying operating system.
However, it’s important to note that os.path.join()
doesn’t check if the resulting path actually exists or is valid. It simply combines the parts. If you need to check if a path exists, you can use os.path.exists()
.
import os
path = '/home/user/documents/report.txt'
# check if the path exists
if os.path.exists(path):
print('The path exists.')
else:
print('The path does not exist.')
# Output (depends on your file system):
# 'The path exists.'
# or
# 'The path does not exist.'
This function returns True
if the path exists and False
otherwise. It’s a handy tool to ensure that your program doesn’t crash when trying to open a file that doesn’t exist.
Advanced Path Manipulation in Python
Python’s os.path
module offers more than just basic path joining and existence checking. Let’s dive into some more advanced usage scenarios.
Joining Multiple Paths
Firstly, os.path.join()
is not limited to joining just two paths. You can join any number of paths together. This is particularly useful when you’re dealing with deeply nested directories.
import os
# define the parts of the file path
root = '/home/user'
subdirectory = 'documents/reports'
filename = 'report.txt'
# use os.path.join to combine the parts
full_path = os.path.join(root, subdirectory, filename)
print(full_path)
# Output:
# '/home/user/documents/reports/report.txt'
In this example, os.path.join()
is used to combine multiple parts into a full file path. This function handles the path separators correctly, regardless of how many parts you’re joining.
Finding the Relative Path
Another useful function is os.path.relpath()
, which calculates the relative path between two absolute paths. This is handy when you want to know the path to a file relative to a certain directory.
import os
# define the paths
path_to_file = '/home/user/documents/reports/report.txt'
start_path = '/home/user'
# use os.path.relpath to find the relative path
relative_path = os.path.relpath(path_to_file, start_path)
print(relative_path)
# Output:
# 'documents/reports/report.txt'
In this example, os.path.relpath()
calculates the path to report.txt
relative to the /home/user
directory. The resulting path is documents/reports/report.txt
.
These are just a few examples of the more advanced techniques you can use with the os.path
module. By mastering these techniques, you’ll be able to handle file paths in Python with ease.
Exploring Pathlib: A Modern Alternative
While os.path
is powerful and widely used, Python 3 introduced a new module for handling file paths: pathlib
. This module offers a more modern and object-oriented approach to path manipulation. It turns paths into ‘Path’ objects with relevant methods and attributes, making your code more readable and easier to maintain.
Let’s look at an example of how to use pathlib
to join paths:
from pathlib import Path
# define the parts of the file path
root = Path('/home/user')
subdirectory = Path('documents/reports')
filename = Path('report.txt')
# use the / operator to join the parts
full_path = root / subdirectory / filename
print(full_path)
# Output:
# '/home/user/documents/reports/report.txt'
In this example, we create Path
objects for each part of the file path. We then use the /
operator to join these parts together. This is a more intuitive and Pythonic way to join paths compared to os.path.join()
.
However, pathlib
is not without its downsides. It’s a newer module and not as widely adopted as os.path
. Some older Python codebases might not support pathlib
, and some developers might be more familiar with os.path
. Therefore, while pathlib
is a powerful tool, it’s important to consider the context in which you’re working.
In conclusion, both os.path
and pathlib
have their strengths and weaknesses. If you’re working in a modern Python 3 environment and prefer a more object-oriented style, pathlib
is a great choice. However, if you’re dealing with older codebases or prefer a more traditional approach, os.path
is still a robust and reliable option for manipulating file paths in Python.
Troubleshooting Common Issues with Python Paths
Manipulating file paths in Python can sometimes lead to unforeseen issues. Let’s discuss some common problems and their solutions.
Dealing with Different Operating Systems
One common issue arises from the differences in file path formats between Unix-based systems (like Linux and macOS) and Windows. For example, Unix-based systems use forward slashes (/
) to separate directories, while Windows uses backslashes (\
).
Luckily, Python’s os.path
and pathlib
modules handle these differences automatically. However, if you’re manually constructing file paths or using hardcoded strings, you might encounter problems.
Here’s an example of a common mistake and how to fix it using os.path
:
import os
# incorrect manual path construction
wrong_path = '/home/user' + '/' + 'documents'
# correct path construction using os.path.join
right_path = os.path.join('/home/user', 'documents')
print('Wrong:', wrong_path)
print('Right:', right_path)
# Output:
# 'Wrong: /home/user/documents'
# 'Right: /home/user/documents'
In this example, the ‘wrong’ path might not work correctly on all operating systems. The ‘right’ path, constructed using os.path.join()
, will work correctly on any system.
Handling File Permissions
Another common issue is dealing with file permissions. When you’re manipulating file paths, you might encounter files or directories that your program doesn’t have permission to access.
Python provides the os.access()
function to check if your program has access to a file or directory:
import os
path = '/home/user/documents'
# check if the program has read access to the path
if os.access(path, os.R_OK):
print('The program has read access to the path.')
else:
print('The program does not have read access to the path.')
# Output (depends on your file system):
# 'The program has read access to the path.'
# or
# 'The program does not have read access to the path.'
In this example, os.access()
is used to check if the program has read access (os.R_OK
) to the path. You can also check for write access (os.W_OK
) and execution access (os.X_OK
).
By being aware of these common issues and knowing how to handle them, you can make your Python path manipulation code more robust and reliable.
Understanding File Paths: The Basics
Before we delve deeper into Python path manipulation, it’s crucial to understand what file paths are and how they work. A file path is simply a string that specifies the location of a file or directory in a file system. It’s like an address that tells your computer where to find a specific file.
There are two types of file paths: absolute and relative.
Absolute Paths
An absolute path is a complete address to a file or directory, starting from the root directory. It’s like a full postal address, including the country, city, street, and house number.
Here’s an example of an absolute path:
/home/user/documents/report.txt
This path starts from the root directory (/
) and specifies each directory (home
, user
, documents
) leading to the file (report.txt
).
Relative Paths
A relative path, on the other hand, specifies the location of a file or directory relative to the current working directory. It’s like giving directions from your current location, such as ‘turn right at the next intersection’.
Here’s an example of a relative path:
./documents/report.txt
This path starts with ./
, which represents the current directory. It then specifies the documents
directory and the report.txt
file within that directory.
Understanding these fundamentals is crucial for manipulating file paths in Python. With this knowledge, you can now use Python’s os.path
and pathlib
modules to navigate your file system with ease.
Expanding Your Python Path Knowledge
Understanding and manipulating file paths in Python is not just a standalone skill. It has far-reaching implications in various areas of programming and web development. Let’s explore these areas a bit.
File I/O Operations
When working with File Input/Output (I/O) operations in Python, you’ll often need to specify the path to the file you want to read from or write to. This could be a simple text file, a CSV data file, or even a complex binary file. Being able to manipulate file paths allows you to locate these files, no matter where they are in your file system.
Web Development
In web development, file paths are used to locate static resources like CSS files, JavaScript files, and images. They’re also used in URL routing, where different URLs are mapped to different parts of your application. By mastering file path manipulation, you can build more robust and flexible web applications.
Exploring Related Concepts
Once you’re comfortable with manipulating file paths, you might want to explore related concepts. For example, you could learn more about file handling in Python, such as reading and writing files, handling CSV data, and dealing with binary files. You could also delve deeper into the os
module, which provides many other tools for interacting with the operating system.
Further Resources for Python Path Mastery
To help you continue your learning journey, here are some additional resources:
- IOFlood’s Python OS Module Guide explains system-specific path conventions and how “os” handles them seamlessly.
GetCurrentDirectory() Usage Guide explores the world of path management and accessing the current directory with ease.
Python “with” Statement – Learn how to use the “with” statement in Python for file handling and resource management.
Python’s official documentation on the os module is a comprehensive guide to the
os
module and theos.path
submodule.Python’s official pathlib module documentation covers the
pathlib
module, an alternative toos.path
.Real Python’s guide to file I/O covers file handling in Python, a related concept to file path manipulation.
By expanding your knowledge beyond just file path manipulation, you can become a more versatile and effective Python developer.
Wrapping Up: Mastering Python Path Manipulation
In this comprehensive guide, we’ve delved into the world of Python file paths, exploring the ins and outs of how to manipulate them effectively.
We began with the basics, introducing the os.path
module and its key functions for file path manipulation. We then ventured into more advanced topics, such as joining multiple paths, finding relative paths, and handling different operating systems and file permissions.
Along our journey, we addressed common issues you might encounter when manipulating file paths and provided solutions to overcome these hurdles. We also introduced pathlib
, a modern, object-oriented alternative to os.path
.
Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
os.path | Widely used, great for beginners | Not as intuitive as pathlib |
pathlib | More intuitive, Pythonic syntax | Newer, less widely adopted |
Whether you’re a Python beginner just starting out or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of how to manipulate file paths in Python.
With this knowledge, you’ll be able to navigate your file system with ease, making your coding process more efficient and streamlined. Happy coding!