Numpy’s np.arange Function Guide (With Examples)
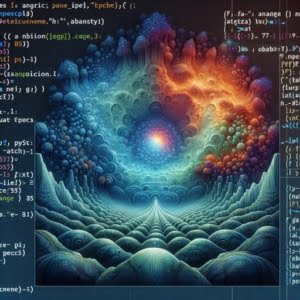
Are you stuck trying to create arrays in Python? Just like a skilled craftsman, np.arange can shape your data into the perfect array.
This guide will walk you through the np.arange function in numpy, a powerful tool for creating numerical sequences in Python.
Whether you’re a beginner or an experienced programmer, this guide will help you understand and master the np.arange function in numpy.
TL;DR: How Do I Use np.arange in Numpy?
To create an array using np.arange in numpy, you specify the start, stop, and step values, such as
array = np.arange(start=0, stop=10, step=2)
. This function generates a sequence of numbers within the specified range, which can be incredibly useful in data analysis and scientific computing.
Here’s a simple example:
import numpy as np
array = np.arange(start=0, stop=10, step=2)
print(array)
# Output:
# array([0, 2, 4, 6, 8])
In this example, we import the numpy module and use the np.arange function to create an array. The start value is 0, the stop value is 10, and the step value is 2. This generates an array of numbers starting from 0, ending before 10, and incrementing by 2.
This is a basic way to use np.arange in numpy, but there’s much more to learn about creating and manipulating arrays in numpy. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Exploring np.arange: Basic Use
The np.arange function in numpy is a versatile tool used to create arrays. It’s like a craftsman’s chisel, shaping your data into the perfect array. Let’s delve into how this function works.
At its most basic, np.arange creates a sequence of numbers within a specified range. You control this range by defining the start, stop, and step values.
Here’s a simple example:
import numpy as np
array = np.arange(start=0, stop=10, step=1)
print(array)
# Output:
# array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
In this example, we’ve created an array that starts at 0, ends at 9, and increments by 1. The np.arange function generates a sequence of numbers, starting from the ‘start’ value (0 in this case), and ending just before the ‘stop’ value (10 in this case), with each number in the sequence incremented by the ‘step’ value (1 in this case).
The advantages of using np.arange are numerous. It allows you to create arrays quickly and efficiently, which is essential in data analysis and scientific computing. However, be aware of potential pitfalls. For instance, when using non-integer step values, the results might be inconsistent due to the finite floating point precision. We’ll explore this in more detail in the advanced use section.
Advanced np.arange: Beyond Basics
As your familiarity with numpy and np.arange grows, you’ll find that this function offers much more than creating simple numerical sequences. Let’s explore some of these advanced uses.
Non-Integer Step Values
First, np.arange allows for non-integer step values. This means that you can generate sequences of floating-point numbers. Here’s how it works:
import numpy as np
array = np.arange(start=0, stop=1, step=0.1)
print(array)
# Output:
# array([0. , 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9])
In this example, the step value is 0.1, which is a floating-point number. This generates a sequence of numbers from 0 to 0.9, incrementing by 0.1 each time.
However, be aware that using non-integer step values can sometimes lead to unexpected results due to the finite precision of floating point numbers. Always check your output to ensure it’s what you expect.
Creating Multi-Dimensional Arrays
Another advanced use of np.arange is creating multi-dimensional arrays. This is especially useful in scientific computing where multi-dimensional data is common. Here’s an example:
import numpy as np
array = np.arange(12).reshape(3, 4)
print(array)
# Output:
# array([[ 0, 1, 2, 3],
# [ 4, 5, 6, 7],
# [ 8, 9, 10, 11]])
In this example, np.arange creates a sequence of 12 numbers. The reshape function then rearranges this sequence into a 3×4 multi-dimensional array.
Diversifying Array Creation: Alternative Approaches
While np.arange is a powerful tool for creating arrays, numpy offers other functions that can be more suitable depending on your specific needs. Two such alternatives are np.linspace and np.logspace.
Using np.linspace
The np.linspace function is similar to np.arange, but instead of defining the ‘step’ value, you define the total number of items you want in your array. Here’s an example:
import numpy as np
array = np.linspace(start=0, stop=1, num=10)
print(array)
# Output:
# array([0. , 0.11111111, 0.22222222, 0.33333333, 0.44444444,
# 0.55555556, 0.66666667, 0.77777778, 0.88888889, 1. ])
In this example, np.linspace creates an array with 10 equally spaced numbers between 0 and 1.
Exploring np.logspace
The np.logspace function, on the other hand, creates an array with numbers that are evenly spaced on a log scale. Here’s how it works:
import numpy as np
array = np.logspace(start=1, stop=3, num=3)
print(array)
# Output:
# array([ 10., 100., 1000.])
In this example, np.logspace creates an array with 3 numbers, evenly spaced on a log scale between 10^1 (10) and 10^3 (1000).
Each of these methods has its own advantages and can be more suitable than np.arange depending on the situation. np.linspace is useful when you need a specific number of items in your array, while np.logspace is perfect for creating arrays with values spread over several orders of magnitude. As always, the best tool depends on your specific needs, so don’t be afraid to experiment and find the method that works best for you.
Troubleshooting np.arange: Common Pitfalls
While using np.arange, you might encounter a few common issues. Understanding these potential pitfalls can save you time and frustration. Let’s explore some of these common problems and their solutions.
Type Errors
One common issue is type errors. For instance, if you inadvertently pass a string argument to np.arange instead of a numeric one, you’ll encounter a TypeError. Here’s an example:
import numpy as np
try:
array = np.arange(start='0', stop=10, step=2)
except TypeError as e:
print(e)
# Output:
# ufunc 'subtract' did not contain a loop with signature matching types (dtype('<U21'), dtype('<U21')) -> dtype('<U21')
In this example, we’ve passed a string ‘0’ as the start value, which causes a TypeError. The solution is simple: always ensure that the arguments to np.arange are numeric.
Unexpected Results Due to Floating Point Precision
Another issue you might encounter is unexpected results due to floating point precision. When using non-integer step values, the results might be inconsistent due to the finite precision of floating point numbers. Here’s an example:
import numpy as np
array = np.arange(0.5, 0.8, 0.1)
print(array)
# Output:
# array([0.5, 0.6, 0.7, 0.8])
In this example, you might expect the array to stop at 0.7, but it includes 0.8 due to floating point precision. One workaround is to use np.linspace when you need to include the end value, or when working with non-integer step values.
These are just a few examples of issues you might encounter when using np.arange. By understanding these potential pitfalls and their solutions, you can use np.arange more effectively and avoid common mistakes.
Unpacking Numpy and Array Creation
To fully grasp the power and utility of np.arange, it’s important to understand the basics of numpy and array creation in Python. Let’s dive into these fundamental concepts.
Understanding Numpy
Numpy, short for ‘Numerical Python’, is a powerful library that supports large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays. It’s a cornerstone in the world of scientific computing with Python.
import numpy as np
print(np.__version__)
# Output:
# '1.21.0'
In this example, we import numpy and print its version. This is the first step in any numpy-related task.
Array Creation in Python
Arrays are a central data structure in numpy, offering a way to represent and manipulate numerical data efficiently. They are similar to lists in Python, but can have any number of dimensions, and all elements are of the same type, typically a numeric one.
Here’s an example of creating a simple one-dimensional array:
import numpy as np
array = np.array([1, 2, 3, 4, 5])
print(array)
# Output:
# array([1, 2, 3, 4, 5])
In this example, we create a one-dimensional array with five elements. This is the simplest form of array creation in numpy.
Understanding these fundamentals of numpy and array creation is crucial to mastering the use of np.arange and other numpy functions. With this foundation, you’ll be better equipped to understand and leverage the power of np.arange.
The Bigger Picture: np.arange and Data Analysis
The np.arange function is more than just a tool for creating arrays. It plays a crucial role in data analysis and scientific computing, where the manipulation and analysis of numerical data is key.
Array Manipulation and Operations in Numpy
Beyond creating arrays, numpy offers a wide range of operations to manipulate and analyze these arrays. For instance, you can perform arithmetic operations, apply mathematical functions, calculate statistical measures, and much more, all in a fast and efficient manner.
Here’s an example of array manipulation using np.arange:
import numpy as np
array = np.arange(10)
squared_array = array ** 2
print(squared_array)
# Output:
# array([ 0, 1, 4, 9, 16, 25, 36, 49, 64, 81])
In this example, we create an array of numbers from 0 to 9 using np.arange, and then square each element of the array. This illustrates how you can easily perform operations on arrays in numpy.
Further Resources for Numpy Proficiency
If you’re interested in diving deeper into numpy and array creation, here are some resources that you might find useful:
- IOFlood’s Numpy Tutorial covers essential tips and techniques for mastering the art of indexing and slicing NumPy arrays for efficient data extraction.
NumPy’s np.zeros() – Simplify array initialization by using “np.zeros” to allocate memory efficiently for data storage.
np.where() in NumPy – Dive into the details of using “np.where” to filter and modify array values based on conditions.
Numpy’s Official Documentation provides a comprehensive resource covering all aspects of numpy.
Python Numpy Tutorial – This tutorial from Stanford provides a practical introduction to numpy.
DataCamp’s Numpy Tutorial is a beginner-friendly tutorial that covers the basics of numpy, including array creation and manipulation.
These resources offer a wealth of information that can help you master numpy and its array creation functions, including np.arange.
Wrapping Up: The Power of np.arange in Numpy
In this comprehensive guide, we’ve explored how to use the np.arange function in numpy to create arrays efficiently.
We delved into the basic usage of the function, showing how you can generate a sequence of numbers within a specified range by defining the start, stop, and step values. Common issues, such as type errors and unexpected results due to floating point precision, were discussed, along with their solutions.
We also explored advanced uses of np.arange
, such as creating multi-dimensional arrays and using non-integer step values. In addition, we introduced alternative approaches to array creation in numpy, including np.linspace
and np.logspace
, each with their own advantages depending on the situation.
Approach | Description | Use Case |
---|---|---|
np.arange | Creates a sequence of numbers within a specified range | When you need to generate a sequence of numbers with a specific step value |
np.linspace | Generates a specified number of evenly spaced values between two numbers | When you need a specific number of items in your array |
np.logspace | Creates an array with numbers that are evenly spaced on a log scale | When you need values spread over several orders of magnitude |
Finally, we discussed the relevance of array creation in data analysis and scientific computing, suggesting further resources for those interested in deepening their understanding of numpy and its array creation functions.
Whether you’re a beginner or an experienced programmer, mastering the np.arange
function in numpy can be a significant asset in your data analysis and scientific computing toolkit.