NP.Zeroes | Using Zeros() In Numpy
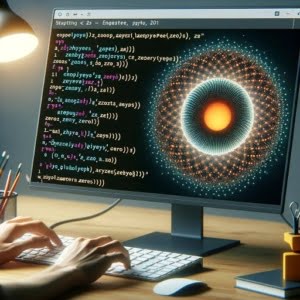
Have you ever found yourself in a situation where you needed to initialize an array with zeros in Python? If so, you’re in the right place. Just like a blank canvas waiting for an artist’s brush, the np.zeros
function in Python’s numpy library allows you to create an array filled with zeros, ready for you to paint your data on it.
In this article, we will guide you through the usage of np.zeros
, from the most basic to advanced levels. Whether you’re a beginner just starting out or an intermediate user looking to deepen your understanding, you’ll find this guide helpful. So, let’s dive into the world of np.zeros
and uncover its potential!
TL;DR: How Do I Use np.zeros in Python?
To create an array of zeros in Python, you can use the
np.zeros
function from the numpy library. Here’s a simple example:
import numpy as np
arr = np.zeros(5)
print(arr)
# Output:
# array([0., 0., 0., 0., 0.])
In the above code block, we’ve imported the numpy library as np
, and then used the np.zeros
function to create an array of five zeros. We then print this array to the console, resulting in an output of five zeros.
If you’re interested in learning more about
np.zeros
, including detailed examples and advanced usage scenarios, continue reading. We’ve got a lot more to cover!
Table of Contents
- Unveiling np.zeros: Creating Arrays Filled with Zeros
- Diving Deeper: Multi-Dimensional Arrays with np.zeros
- Exploring Alternatives: np.ones and np.empty
- Navigating Challenges: Troubleshooting np.zeros
- Unraveling the Basics: Understanding Numpy’s Array Data Type
- The Power of np.zeros in Data Analysis and Machine Learning
- Wrapping Up: np.zeros in Python
Unveiling np.zeros: Creating Arrays Filled with Zeros
The np.zeros
function in Python’s numpy library is a versatile tool for initializing arrays. It’s simple to use and incredibly efficient. Let’s explore how it works.
The np.zeros
function takes in one mandatory parameter: the shape of the array. The shape is defined as a tuple indicating the size of the array along each dimension.
import numpy as np
# Creating a 1D array of zeros
zero_array = np.zeros(3)
print(zero_array)
# Output:
# array([0., 0., 0.])
In the above example, we’ve created a 1-dimensional array of three zeros. The number 3
passed to the np.zeros
function indicates the size of the array.
The np.zeros
function is particularly useful when you have a large dataset and need to initialize an array quickly. It’s efficient and fast, saving valuable processing time.
However, there’s one potential pitfall to be aware of. By default, np.zeros
creates an array of float data type. If you need an array of integers or any other data type, you must specify it using the dtype
parameter.
import numpy as np
# Creating a 1D array of integer zeros
zero_array = np.zeros(3, dtype=int)
print(zero_array)
# Output:
# array([0, 0, 0])
In this code block, we’ve created an array of three zeros, but this time they’re integers. We achieved this by specifying dtype=int
when calling the np.zeros
function.
Diving Deeper: Multi-Dimensional Arrays with np.zeros
While creating a single-dimensional array with np.zeros
is straightforward, this function truly shines when we start working with multi-dimensional arrays. Let’s dive into the creation of 2D and 3D arrays with np.zeros
.
Creating a 2D Array
To create a 2D array, we pass a tuple to the np.zeros
function, specifying the number of rows and columns.
import numpy as np
# Creating a 2D array of zeros
zero_2d_array = np.zeros((3, 4))
print(zero_2d_array)
# Output:
# array([[0., 0., 0., 0.],
# [0., 0., 0., 0.],
# [0., 0., 0., 0.]])
In the above code block, we’ve created a 2D array with three rows and four columns, all initialized with zeros.
Crafting a 3D Array
Creating a 3D array follows a similar pattern. This time, we pass a tuple specifying the number of matrices, rows, and columns.
import numpy as np
# Creating a 3D array of zeros
zero_3d_array = np.zeros((2, 3, 4))
print(zero_3d_array)
# Output:
# array([[[0., 0., 0., 0.],
# [0., 0., 0., 0.],
# [0., 0., 0., 0.]],
#
# [[0., 0., 0., 0.],
# [0., 0., 0., 0.],
# [0., 0., 0., 0.]]])
In this example, we’ve created a 3D array with two matrices, each containing three rows and four columns.
The ability to create multi-dimensional arrays with np.zeros
opens up a world of possibilities in data manipulation and analysis. Whether you’re working with image data in machine learning or handling large datasets in data analysis, np.zeros
provides an efficient way to initialize your multi-dimensional arrays.
Exploring Alternatives: np.ones and np.empty
While np.zeros
is a powerful function in the numpy library for initializing arrays, it’s not the only tool at your disposal. Let’s explore two alternative methods for creating arrays in numpy: np.ones
and np.empty
.
The np.ones Function
The np.ones
function works similarly to np.zeros
, but instead of filling the array with zeros, it fills the array with ones.
import numpy as np
# Creating a 1D array of ones
ones_array = np.ones(5)
print(ones_array)
# Output:
# array([1., 1., 1., 1., 1.])
In the above code block, we’ve created a 1D array with five ones using the np.ones
function. This function is particularly useful when you need to initialize an array with a value other than zero.
The np.empty Function
The np.empty
function, on the other hand, does not initialize the array elements to any particular values. It simply allocates the requested space in memory and returns an array without initializing entries.
import numpy as np
# Creating an empty array
empty_array = np.empty(5)
print(empty_array)
# Output:
# array([6.23042070e-307, 4.67296746e-307, 1.69121096e-306, 1.06811422e-306, 1.42417221e-306])
In the code block above, we’ve created an ’empty’ array. The values you see in the output are garbage values, which is whatever values were already in memory at that location. The np.empty
function is faster than np.zeros
and np.ones
as it does not have to initialize the array elements, but it should only be used when you are certain that you will be overwriting all elements of the array.
Each of these functions — np.zeros
, np.ones
, and np.empty
— has its own advantages and is suited to different scenarios. Understanding their differences and when to use each one is key to effectively leveraging the power of the numpy library.
While np.zeros
is a powerful tool for initializing arrays, like any function, it’s not without its quirks. Let’s discuss some common issues you may encounter and how to solve them.
Data Type Issues
By default, np.zeros
creates an array of float data type. If you need an array of integers or any other data type, you must specify it using the dtype
parameter.
import numpy as np
# Creating a 1D array of integer zeros
zero_array = np.zeros(3, dtype=int)
print(zero_array)
# Output:
# array([0, 0, 0])
In this code block, we’ve created an array of three zeros, but this time they’re integers. We achieved this by specifying dtype=int
when calling the np.zeros
function.
Memory Considerations
When working with large arrays, memory management becomes crucial. The np.zeros
function is memory-efficient and only allocates the necessary space for the array. However, if you’re creating extremely large arrays and running into memory issues, you may want to consider using the np.empty
function, which does not initialize the array elements and thus saves on memory.
import numpy as np
# Creating a large empty array
large_array = np.empty(1000000)
print(large_array)
# Output: array with uninitialized entries
In the above code block, we’ve created an ’empty’ array with one million entries. The np.empty
function is faster and more memory-efficient than np.zeros
as it does not have to initialize the array elements.
Remember, each function in the numpy library has its own strengths and weaknesses. Understanding these can help you choose the right tool for your specific needs.
Unraveling the Basics: Understanding Numpy’s Array Data Type
Before we delve deeper into the np.zeros
function, it’s crucial to understand the fundamental data type at the heart of numpy: the array. The numpy library in Python primarily revolves around this versatile data type.
What is a Numpy Array?
A numpy array, often referred to as a ndarray (n-dimensional array), is a grid of values, all of the same type. It’s indexed by a tuple of non-negative integers. The dimensions are defined as axes, and the number of axes is the rank.
import numpy as np
# Creating a 1D numpy array
np_array = np.array([1, 2, 3])
print(np_array)
# Output:
# array([1, 2, 3])
In the above code block, we’ve created a 1D numpy array with three elements.
How Does np.zeros Fit In?
The np.zeros
function leverages the power of numpy arrays. It creates a new array of the specified size, all initialized to zero. This is particularly useful when you need to create a ‘clean slate’ array before filling it with data.
Understanding the fundamentals of numpy arrays is crucial to grasp the full potential of the np.zeros
function. With this understanding, you can better appreciate the efficiency and utility of np.zeros
in initializing arrays in Python.
The Power of np.zeros in Data Analysis and Machine Learning
The np.zeros
function is more than just a tool for initializing arrays. It plays a vital role in data analysis and machine learning, areas where handling large amounts of data efficiently is paramount.
In data analysis, np.zeros
is often used to initialize arrays before filling them with data for processing. It provides a quick and efficient way to create a ‘starting point’ for your data.
In machine learning, np.zeros
is frequently used in the initialization of weights in algorithms. For example, in neural networks, the weights of the nodes are often initialized to zero before training begins.
import numpy as np
# Initializing weights for a neural network layer
weights = np.zeros((10, 5))
print(weights)
# Output:
# array([[0., 0., 0., 0., 0.],
# [0., 0., 0., 0., 0.],
# [0., 0., 0., 0., 0.],
# [0., 0., 0., 0., 0.],
# [0., 0., 0., 0., 0.],
# [0., 0., 0., 0., 0.],
# [0., 0., 0., 0., 0.],
# [0., 0., 0., 0., 0.],
# [0., 0., 0., 0., 0.],
# [0., 0., 0., 0., 0.]])
In the above code block, we’ve created a 2D array of zeros to represent the weights of a neural network layer with ten nodes and five inputs each. This array will then be updated during the training process.
Exploring Further
While np.zeros
is a powerful tool in the numpy library, it’s just the tip of the iceberg. There are other related concepts like array operations and broadcasting that are worth exploring. To deepen your understanding of numpy and its capabilities, consider exploring resources like the following:
- This Ultimate Numpy Resource for Python developers explains NumPy’s integration with other popular Python libraries for data analysis and visualization.
np.linspace() Python Tutorial – Explains how to create evenly spaced numeric arrays with the “np.linspace” function.
Generating Sequences: numpy np.arange() – Learn about generating numeric sequences with custom values in Python.
The Official Numpy Documentation offers a detailed overview of the module and its functionalities.
Python Numpy Articles – A series of GeeksForGeeks articles centered around the usage of Numpy in Python.
This downloadable Numpy Book PDF from MIT offers comprehensive knowledge about Numpy.
With a solid grasp of numpy and its functions, you’ll be better equipped to handle complex data manipulation tasks in Python.
Wrapping Up: np.zeros in Python
Throughout this guide, we’ve explored the np.zeros
function in Python’s numpy library. This function is a powerful tool for initializing arrays, offering an efficient way to create ‘blank slate’ data structures ready for your data.
We’ve discussed the basic usage of np.zeros
, demonstrating how to create a single-dimensional array filled with zeros. We also delved into more advanced usage, explaining how to create multi-dimensional arrays.
We also covered some potential issues you might encounter with np.zeros
, such as data type and memory considerations, and how to navigate them.
Moreover, we explored alternative methods for creating arrays in numpy, such as np.ones
and np.empty
, each with its own strengths and suited to different scenarios.
By understanding these different methods and when to use each one, you can leverage the full power of the numpy library for your data manipulation tasks in Python.